本来打算去上课的,但给我一觉睡过去了
。。。。然后就有了这篇题解。。。
250pts
Problem Statement |
| Fox Ciel thinks that the number 41312432 is interesting. This is because of the following property: There is exactly 1 digit between the two 1s, there are exactly 2 digits between the two 2s, and so on. Formally, Ciel thinks that a number X is interesting if the following property is satisfied: For each D between 0 and 9, inclusive, X either does not contain the digit D at all, or it contains exactly two digits D, and there are precisely D other digits between them. You are given a string x that contains the digits of a positive integer. Return "Interesting" if that integer is interesting, otherwise return "Not interesting". |
Definition |
|
Class: | InterestingNumber | Method: | isInteresting | Parameters: | string | Returns: | string | Method signature: | string isInteresting(string x) | (be sure your method is public) | |
Limits |
|
Time limit (s): | 2.000 | Memory limit (MB): | 256 | |
Constraints |
- | x will correspond to an integer between 1 and 1,000,000,000, inclusive. |
- | x will not start with a '0'. |
Examples |
0) | |
|
| Returns: "Interesting" |
There are 0 digits between the two 0s, and 2 digits between the two 2s, so this is an interesting number. | | |
1) | |
|
| Returns: "Not interesting" |
There should be 1 digit between the two 1s, but there are 2 digits between them. Hence, this number is not interesting. | | |
2) | |
|
| Returns: "Interesting" |
This is the number in the statement. | | |
3) | |
|
| Returns: "Not interesting" |
There is only one digit 6 in this number, so it's not interesting. | | |
4) | |
|
|
5) | |
|
| Returns: "Not interesting" |
This number contains the digit 1 three times. | | |
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2003, TopCoder, Inc. All rights reserved.
int cnt[12];
int pos[12][5];
string InterestingNumber::isInteresting(string x)
{
fill(cnt,cnt+12,0);
memset(pos,0,sizeof(pos));
int n=x.size();
for(int i=0;i<n;i++)
{
cnt[x[i]-'0']++;
pos[x[i]-'0'][cnt[x[i]-'0']]=i;
if(cnt[x[i]-'0']>2) return "Not interesting";
}
for(int i=0;i<=9;i++)
{
if(!cnt[i]) continue;
if(pos[i][2]-pos[i][1]-1==i) continue;
return "Not interesting";
}
return "Interesting";
}
500pts:去掉不能整除的数,然后求lcm
Problem Statement |
| For any non-empty sequence of positive integers s1, s2, ..., sK their least common multiple is the smallest positive integer that is divisible by each of the given numbers. We will use "lcm" to denote the least common multiple. For example, lcm(3) = 3, lcm(4,6) = 12, and lcm(2,5,7) = 70. You are given a vector <int> S and an int x. Find out whether we can select some elements from S in such a way that their least common multiple will be precisely x. Formally, we are looking for some s1, s2, ..., sK, K >= 1, such that each si belongs to S, and x=lcm(s1, s2, ..., sK). Return "Possible" if such elements of S exist, and "Impossible" if they don't. |
Definition |
|
Class: | LCMSetEasy | Method: | include | Parameters: | vector <int>, int | Returns: | string | Method signature: | string include(vector <int> S, int x) | (be sure your method is public) | |
Limits |
|
Time limit (s): | 2.000 | Memory limit (MB): | 256 | |
Constraints |
- | S will contain between 1 and 50 elements, inclusive. |
- | Each element in S will be between 1 and 1,000,000,000, inclusive. |
- | Elements in S will be distinct. |
- | x will be between 2 and 1,000,000,000, inclusive. |
Examples |
0) | |
|
| Returns: "Possible" |
We can obtain 20 in multiple ways. One of them: 20 = lcm(4, 5). | | |
1) | |
|
| Returns: "Impossible" |
If S={2,3,4}, the only values we can obtain are 2, 3, 4, 6, and 12. | | |
2) | |
|
|
3) | |
|
{1,2,3,4,5,6,7,8,9,10} | 24 | | Returns: "Possible" |
| |
4) | |
|
{100,200,300,400,500,600} | 2000 | | Returns: "Possible" |
| |
5) | |
|
{100,200,300,400,500,600} | 8000 | | Returns: "Impossible" |
| |
6) | |
|
{1000000000,999999999,999999998} | 999999999 | | Returns: "Possible" |
| |
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2003, TopCoder, Inc. All rights reserved
int gcd(int a,int b)
{
return b?gcd(b,a%b):a;
}
int lcm(int a,int b)
{
return b/gcd(a,b)*a;
}
string LCMSetEasy::include(vector <int> S,int x)
{
vector<int> vk;
for(int i=0,n=S.size();i<n;i++)
{
if(x%S[i]==0)
{
vk.push_back(S[i]);
}
}
int lc=1;
for(int i=0,n=vk.size();i<n;i++)
{
lc=lcm(lc,vk[i]);
}
if(lc==x) return "Possible";
else return "Impossible";
}
1000pts:鉴于TC的机器很快,所以直接枚举所有的可能(100w而已。。。
roblem Statement |
| There is an n times n field. Some of the cells of the field contain water. You are given a map of the field encoded as a vector <string> field. There are n elements in field and each of them has n characters. The character field[i][j] is 'Y' if there is water in the cell (i,j). Otherwise, the corresponding character is 'N'. There are 4n elephants around the field: one per each cell boundary, as shown in the pictures below. The elephants can use their trunks to drink water. Each elephant can only extend its trunk straight into the field. So, for example, the elephants that are on the left side of the field can only extend their noses towards the right. The trunks are long enough to reach the opposite end of the field. 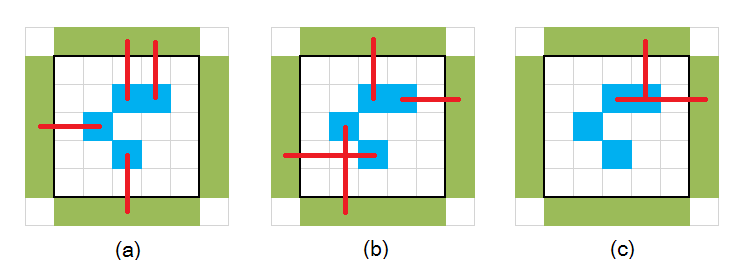 There are two additional restrictions: The trunks of elephants are not allowed to intersect. For each cell with water, there can be at most one elephant drinking from that cell. For example, figure (a) below shows a valid configuration. Cells with water are blue, elephants are green, and their trunks are red. In the figure there are four elephants that drink. Figures (b) and (c) show invalid configurations. In both of them the trunks intersect. Your task is to return the maximal number of elephants who can drink at the same time. |
Definition |
|
Class: | ElephantDrinkingEasy | Method: | maxElephants | Parameters: | vector <string> | Returns: | int | Method signature: | int maxElephants(vector <string> map) | (be sure your method is public) | |
Limits |
|
Time limit (s): | 2.000 | Memory limit (MB): | 256 | |
Constraints |
- | n will be between 2 and 5, inclusive. |
- | field will contain exactly n elements, inclusive. |
- | Each element in field will contain exactly n characters, inclusive. |
- | Each character in field will be 'Y' or 'N'. |
Examples |
0) | |
|
{"NNNNN",
"NNYYN",
"NYNNN",
"NNYNN",
"NNNNN"} | | Returns: 4 |
This is the field shown in the figure in the problem statement. As shown in figure (a), four elephants can drink at the same time. And as we only have four cells with water, this is clearly optimal. | | |
1) | |
|
| Returns: 8 |
It is possible for the elephants to drink from 8 cells at the same time. For example, a suitable set of 8 elephants can drink from all cells except for the one in the center. It is not possible for the elephants to drink from all 9 cells at the same time. | | |
2) | |
|
{"YNYN",
"NNYY",
"YYNN",
"YYYY"} | | Returns: 10 |
| |
3) | |
|
{"YNYN",
"YNYY",
"YYNN",
"YYYY"} | | Returns: 10 |
| |
4) | |
|
{"YYNYN",
"NYNNY",
"YNYNN",
"YYNYY",
"YYNNN"} | | Returns: 12 |
| |
5) | |
|
{"YYNYN",
"NYNYY",
"YNYYN",
"YYNYY",
"YYNNN"} | | Returns: 13 |
| |
6) | |
|
| Returns: 0 |
There can be no water at all. | | |
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2003, TopCoder, Inc. All rights reserved.
int G[10][10];
int getone(int x)
{
int t=0;
while(x)
{
t++;
x&=(x-1);
}
return t;
}
int ElephantDrinkingEasy::maxElephants(vector <string> mp)
{
int n=mp.size(),m=4*n;
int ans=0;
for(int k=0;k<(1<<m);k++)
{
int tt=getone(k);
bool ok=true;
if(k<ans) continue;
memset(G,0,sizeof(G));
///down
for(int i=0;i<n;i++)
{
if(!(k&(1<<i))) continue;
bool water=false;
for(int j=0;j<n;j++)
{
if(G[j][i]) {ok=false; break; }
G[j][i]=1;
if(mp[j][i]=='Y') {water=true; break;}
}
if(water==false) ok=false;
if(ok==false) break;
}
if(!ok) continue;
///up
for(int i=0;i<n;i++)
{
if(!(k&(1<<(i+n)))) continue;
bool water=false;
for(int j=n-1;j>=0;j--)
{
if(G[j][i]) {ok=false;break;}
G[j][i]=1;
if(mp[j][i]=='Y') {water=true; break;}
}
if(water==false) ok=false;
if(ok==false) break;
}
if(!ok) continue;
///left
for(int i=0;i<n;i++)
{
if(!(k&(1<<(i+2*n)))) continue;
bool water=false;
for(int j=0;j<n;j++)
{
if(G[i][j]) {ok=false;break;}
G[i][j]=1;
if(mp[i][j]=='Y') {water=true; break;}
}
if(water==false) ok=false;
if(ok==false) break;
}
if(!ok) continue;
///right
for(int i=0;i<n;i++)
{
if(!(k&(1<<(i+3*n)))) continue;
bool water=false;
for(int j=n-1;j>=0;j--)
{
if(G[i][j]) {ok=false;break;}
G[i][j]=1;
if(mp[i][j]=='Y') {water=true; break;}
}
if(water==false) ok=false;
if(ok==false) break;
}
if(!ok) continue;
ans=max(ans,tt);
}
return ans;
}