原博地址http://blog.csdn.net/facedream/article/details/7934923
一、准备工作
当在页面表单中选择本地文件之后点击“提交“按钮将文件上传到服务器时该Http请求正文的数据类型是multipart/form-data型的。在Servlet中,该请求会被Servlet容器(如Tomcat等)包装成HttpServletRequest对象,再由端所请求的相应Servlet进行处理。
Apache FileUpload 包是Apache的开源软件包,它令文件上传功能的实现变得简单易行。我们需要在我们的工程里同时引入commons-fileupload包和commons-io包。
本篇使用的两个包的版本分别是commons-fileupload-1.3.jar和commons-io-2.4.jar。
二、程序代码
1、JSP页面
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>Insert title here</title> </head> <body> <form action="upload" method="post" enctype="multipart/form-data"> 上传文件<input type="file" name="file" /><br /><br /> <input type="submit" value="上 传" /> </form> </body> </html>
页面很简单,只有一个文件选择框和一个提交按钮。
2、UploadServlet文件
/** * Servlet implementation class AddCssServlet */ @WebServlet(name = "UploadServlet", urlPatterns = { "/upload" }) public class UploadServletextends HttpServlet { private static final long serialVersionUID = 1L; /** * @see HttpServlet#HttpServlet() */ public UploadServlet() { super(); // TODO Auto-generated constructor stub } /** * @see HttpServlet#doGet(HttpServletRequest request, HttpServletResponse * response) */ protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { // TODO Auto-generated method stub request.setCharacterEncoding("utf-8"); response.setCharacterEncoding("utf-8"); response.setContentType("text/html"); PrintWriter out = response.getWriter(); // 设置上传文件保持路径 String filepath = this.getServletContext().getRealPath("/upload"); System.out.println(filepath); File uploadDir = new File(filepath); // 如果该文件夹不存在则创建 if (!uploadDir.exists()) { uploadDir.mkdirs(); } // 获取服务器默认的临时文件夹 String temp = System.getProperty("java.io.tmpdir"); File tempDir = new File(temp); // 如果该文件夹不存在,则创建 if (!tempDir.exists()) { tempDir.mkdirs(); } // 创建文件项目工厂对象 DiskFileItemFactory factory = new DiskFileItemFactory(); // 设置临时文件夹 factory.setRepository(new File(temp)); // 设置缓冲区大小为 1M factory.setSizeThreshold(1024 * 1024); ServletFileUpload serveltFileUpload = new ServletFileUpload(factory); // 解析文件上传请求,解析结果放在 List中 try { List<FileItem> list = serveltFileUpload.parseRequest(request); for (FileItem item : list) { // 判断某项是否是普通的表单类型。 if (item.isFormField()) { //该表单项是普通类型 // 忽略简单form字段而不是上传域的文件域 continue; } else { // 否则文件域 // 获取上传文件名 String fileName = item.getName(); // 此时的文件名包含了完整的路径,得注意加工一下 System.out.println("完整的文件名:" + fileName); int index = fileName.lastIndexOf("\\"); fileName = fileName.substring(index + 1, fileName.length()); // 创建文件输入流 InputStream in = item.getInputStream(); // 创建一个指向 File 对象所表示的文件中写入数据的文件输出流 FileOutputStream outs = new FileOutputStream(new File( filepath, fileName)); int len = 0; // 创建字节数组 ,大小位 1024字节 byte[] buffer = new byte[1024]; out.println("上传文件名称:" + fileName + "<br/>"); out.println("上传文件大小:" + item.getSize() / 1024 + " KB(" + item.getSize() + " 字节)<br/>"); while ((len = in.read(buffer)) != -1) { // 字节数组从偏移量 0 到len 个字节写入文件输出流 outs.write(buffer, 0, len); } // 关闭输入流 in.close(); // 关闭输出流 outs.close(); } } } catch (FileUploadException e) { // TODO Auto-generated catch block e.printStackTrace(); } finally { } } /** * @see HttpServlet#doPost(HttpServletRequest request, HttpServletResponse * response) */ protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { // TODO Auto-generated method stub doGet(request, response); } }
三、查看上传
上传的文件的将存储在你的服务器的临时目录下。
Tomcat运行后的存储路径为:\workspace\.metadata\.plugins\org.eclipse.wst.server.core\tmp0\wtpwebapps\你的项目名称\upload
当然,我们一般更愿意将我们的文件存放在当前项目所在的WEB服务器下,此时我们就需要更改项目部署时的路径。
在Eclipse中集成好Tomcat之后,发布我们的项目,然后菜单栏选择Window-->show view-->services,查看服务面板,双击Tomcat进入配置界面修改Server Locations。
默认选项为:Use workspace metadata(dose not modify Tomcat installation)
修改选项为:Use Tomcat installation(takes control of Tomcat installation)
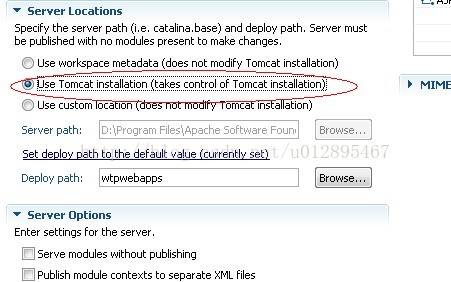
更改配置后,我们的文件上传路径就变为C:\你的Tomcat路径\wtpwebapps\你的项目名称\upload
另外有两点值得注意:
1、若修改配置时显示灰色无法更改,此时我们需要右击选择clean将Tomcat下的所有项目一处方可设置。
2、Eclipse每次部署发布项目时会将上一次部署的所有内容删除,然后再重新部署。所以,若上传文件时保存路径指向的是Tomcat中部署的文件内,每次重新部署发布就会将之前上传的文件全部清空。