Image lazy loading defers the loading of an image that isn’t currently visible in the viewport. Such an image will be loaded only when the user scrolls and the image becomes visible. Using this technique, we can gain better performance and load time.
图像延迟加载会延迟加载当前在视口中不可见的图像。 仅当用户滚动并且图像变为可见时,才会加载此类图像。 使用此技术,我们可以获得更好的性能和加载时间。
Today modern browsers added native support for lazy loading images, and we can benefit from it by adding one simple attribute to our img
element:
今天,现代浏览器增加了对延迟加载图像的本机支持,我们可以通过在img
元素中添加一个简单的属性来从中受益:
<img src="src.png" alt="Angular" loading="lazy">
The loading
attribute supports three options — auto
, eager
, and lazy
. Setting it to lazy
will defer the loading of the resource until it reaches a calculated distance from the viewport.
loading
属性支持三个选项auto
, eager
和lazy
。 将其设置为lazy
将延迟资源的加载,直到达到距视口的计算距离为止。
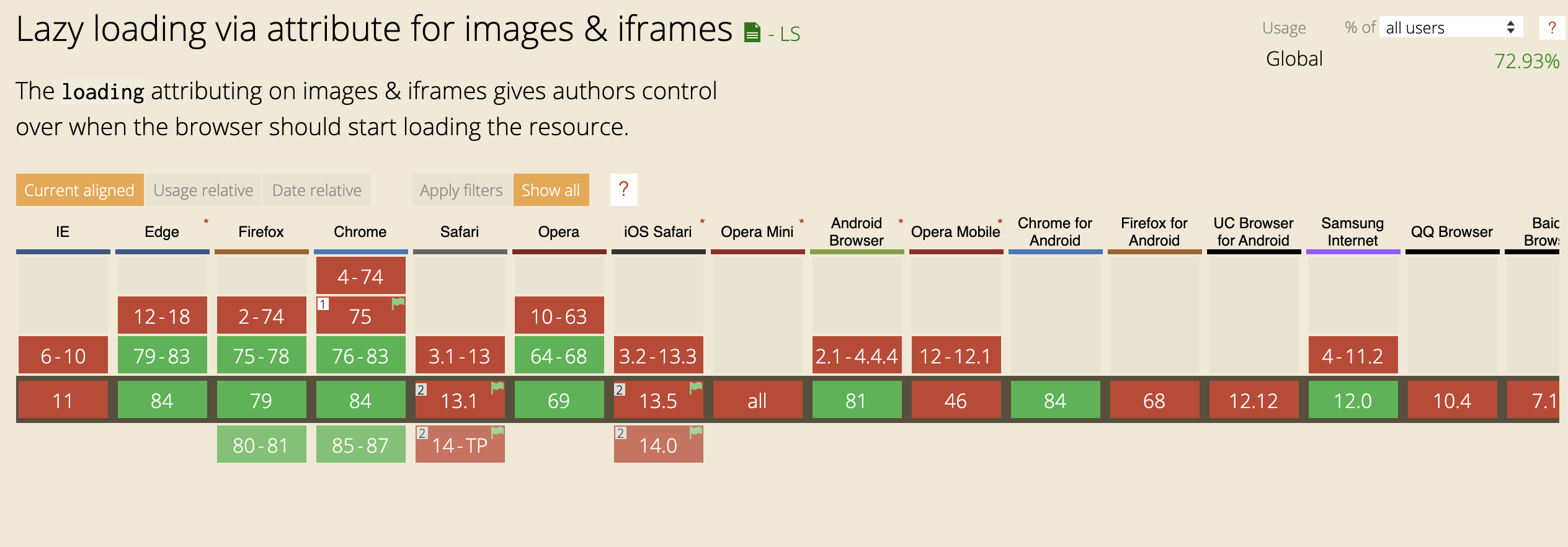
Let’s create a directive that seamlessly adds this attribute if the browser supports it:
让我们创建一个指令,如果浏览器支持的话,可以无缝添加该属性:
import { Directive, ElementRef } from '@angular/core';
@Directive({ selector: 'img' })
export class LazyImgDirective {
constructor({ nativeElement }: ElementRef<HTMLImageElement>) {
const supports = 'loading' in HTMLImageElement.prototype;
if (supports) {
nativeElement.setAttribute('loading', 'lazy');
}
}
}
We check if the browser supports this feature. If that’s the case, we add the loading attribute; Otherwise, we leave the default behavior.
我们检查浏览器是否支持此功能。 如果是这样,我们添加loading属性; 否则,我们保留默认行为。
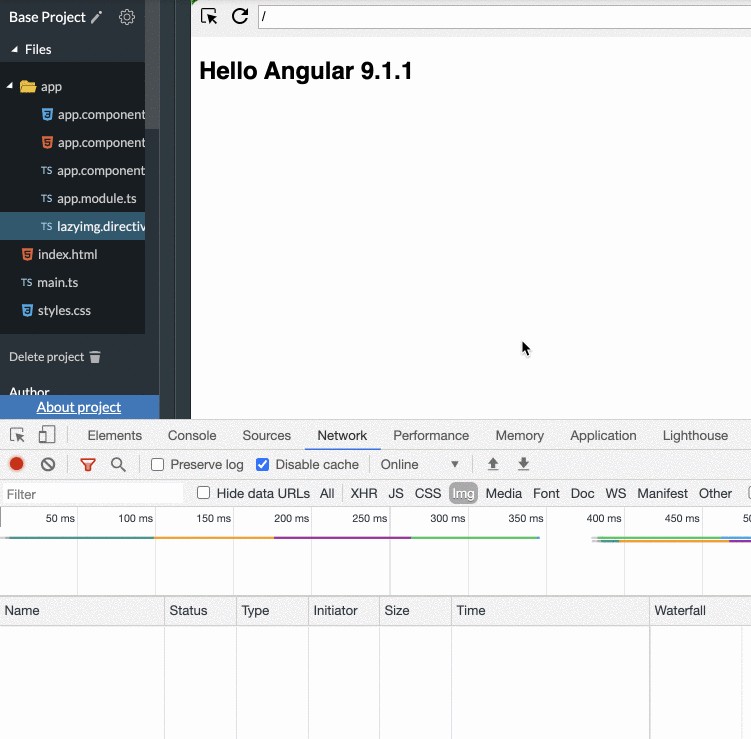
We can also take it one step further and fallback to an IntersectionObserver implementation, which is supported by all browsers (except IE).
我们还可以更进一步,回退到IntersectionObserver实现,所有浏览器(IE除外)都支持该实现。
import { Directive, ElementRef } from '@angular/core';
@Directive({ selector: 'img' })
export class LazyImgDirective {
constructor({ nativeElement }: ElementRef<HTMLImageElement>) {
const supports = 'loading' in HTMLImageElement.prototype;
if (supports) {
nativeElement.setAttribute('loading', 'lazy');
} else {
// fallback to IntersectionObserver
}
}
}
🚀万一您错过了 (🚀 In Case You Missed It)
Here are a few of my open source projects:
这是我的一些开源项目:
Akita: State Management Tailored-Made for JS Applications
秋田 :针对JS应用量身定制的国家管理
Spectator: A Powerful Tool to Simplify Your Angular Tests
Spectator :简化角度测试的强大工具
Transloco: The Internationalization library Angular
Transloco : Angular国际化图书馆
Forms Manager: The Foundation for Proper Form Management in Angular
表单管理器 :Angular中正确表单管理的基础
Cashew: A flexible and straightforward library that caches HTTP requests
腰果 :一个灵活而直接的库,用于缓存HTTP请求
Error Tailor — Seamless form errors for Angular applications
错误裁缝 -Angular应用程序的无缝形式错误
And many more!
和许多更多!
Follow me on Medium or Twitter to read more about Angular, Akita and JS!
翻译自: https://netbasal.com/lazy-load-images-in-angular-with-two-lines-of-code-beb13cd5a1c4