算法符号
I wrote an article the other day about the programming profession and the qualities & skills you needed. One of those skills was math. I then wrote a second article on the algorithms and again mentioned math. And well I thought it might be a good time to actually write about math for a change.
前几天,我写了一篇有关编程专业以及您需要的素质和技能的文章。 这些技能之一是数学。 然后,我写了第二篇有关算法的文章,并再次提到了数学。 好吧,我认为这可能是时候写一些有关改变的数学了。
So here we go. Now there is a fair bit of math in computer science, although I would argue most of it reasonably well-grounded. I mentioned base theory, boolean logic, geometry, matrices and percentages as candidates. But I don’t want to talk about any those right now. I want to talk about this math thing called the big O notation. Big O notation is a means of measuring the performance of algorithms.
所以我们开始。 现在,计算机科学领域中有相当一部分数学,尽管我认为其中大部分都是有充分根据的。 我提到了基础理论,布尔逻辑,几何,矩阵和百分比作为候选。 但是我现在不想谈论这些。 我想谈谈这个叫做大O符号的数学问题。 大O表示法是一种衡量算法性能的方法。
It is a notation invented by Paul Bachmann and Edmund Landau. The individual you won’t be surprised to learn were mathematicians. Although you may be taken back by the knowledge that they were both born long before computers were invented in the eighteenth century.
这是Paul Bachmann和Edmund Landau发明的一种表示法。 您不会感到惊讶的是数学家。 尽管您可能会回想起它们都早在18世纪发明计算机之前就知道了。
The notation is used as a standard way to compare how algorithms perform as your data input grows. Here is a cheat sheet that shows you a graph of what is what.
该符号用作比较算法随着数据输入的增长如何执行的标准方法。 这是一份备忘单,向您显示什么是图形。
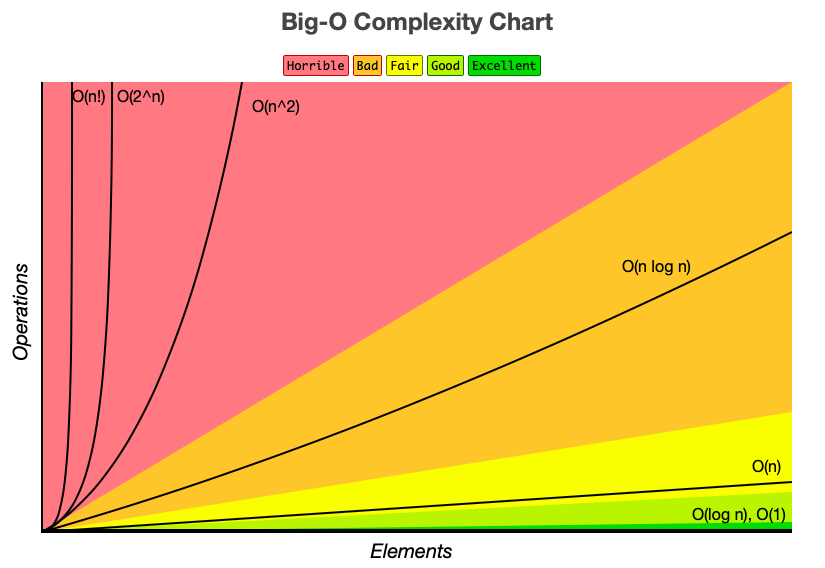
In short, you’re looking for algorithms that sit in the green band and want to avoid those in the red. So what does all this means practically?
简而言之,您正在寻找位于绿色区域的算法,并希望避免使用红色的算法。 那么,这实际上意味着什么?
O(1) (O(1))
This denotes a constant performance. So it doesn’t matter how much data you add to your set, the time it takes is always the same. An example of this might be a function that calculates something, so pure math if you like. It won’t matter what sort of numbers you send to the function, the time it takes will always be constant. This is the green zone.
这表示性能稳定 。 因此,无论向集合中添加多少数据都没有关系,花费的时间始终是相同的。 这样的一个例子可能是一个计算东西的函数,如果您愿意,可以使用纯数学运算。 您发送给函数什么样的数字都没有关系,它花费的时间将始终是恒定的。 这是绿色区域。
上) (O(n))
This is also known as linear time. This means the amount of time it takes for your method to run is directly linked to the amount of data that you add to it. So a CS example would look like this.
这也称为线性时间。 这意味着您的方法运行所花费的时间直接与您添加到其中的数据量有关。 因此,一个CS示例将如下所示。
for t in 0...n {
print("n ",n)
}
Now as can you see, the bigger you make n, the longer it will take for the method to complete. But the time is taken will be uniform. Plot it on a graph and it will be a straight line. On the chart above we’re in the yellow zone.
现在可以看到,n越大,完成该方法所花费的时间就越长。 但是花费的时间将是统一的。 将其绘制在图形上将是一条直线。 在上面的图表中,我们位于黄色区域。
O(n2) (O(n2))
This is also known as quadratic time. This means the amount of time it takes for your method to run will increase dramatically as the amount of data you send in increases. A simple example of this looks like this.
这也称为二次时间。 Ť他的手段所花费的时间为你的方法来运行将大大增加数据的你增加发量的量。 一个简单的例子如下。
for t1 in 0...n {
for t2 in 0...n {
print("t1 t2 ",t1,t2)
}
}
You can appreciate I am sure that the greater the value of n the longer it will take. Here we’re in the red zone.
您可以理解,我确信n的值越大,花费的时间就越长。 这是红色区域。
O(log n) (O(log n))
This is known as logarithmic time, this means the amount of time it takes for a method to run will increase in proportion to a logarithmic on the input data size. The classic example of a logarithmic algorithm is a binary search. The code looks like this.
这称为对数时间 ,这意味着方法运行所花费的时间将与输入数据大小的对数成正比。 对数算法的经典示例是二进制搜索。 代码看起来像这样。
O(nlog n) (O(nlog n))
This is also as logarithmic time, only this time is multiplied by n [which is the amount of data]. A binary sort is a classic example of an algorithm with a big O of nlog n. The code for that looks like this.
这也称为对数时间 ,仅此时间乘以n [这是数据量]。 二进制排序是nlog n的O大的算法的经典示例。 该代码看起来像这样。
Which covers our five main cases. To be a better programmer you need to be at the top of this list. You don’t want to build an app with loops with a Big O complexity at the bottom unless of course, you like being roasted alive in your code reviews.
其中涵盖了我们的五个主要案例。 要成为一个更好的程序员,您需要在此列表的顶部。 除非您希望在代码审查中活着,否则您不希望在底部构建具有O大复杂性的循环的应用程序。
O(1)O(n)O(log n)O(nlog n)O(n2)
O(1)O(n)O(log n)O(nlog n)O(n2)
Now, it isn’t just about the time taken for your function to run. Because you can use the same idea when talking about the memory used by your application. That said obviously the same heuristic applies, you want O(1) in an ideal world and you definitively don’t want O(n2).
现在,这不仅是函数运行所花的时间。 因为在谈论应用程序使用的内存时,您可以使用相同的想法。 也就是说,显然有相同的启发式方法,您希望在理想世界中使用O(1),而您绝对不希望使用O(n2)。
As a closing note here is the source of the graphic I used. An excellent reference should you be interested in learning/having more examples than I gave in this super short article on the subject.
作为结束语, 这里是我使用的图形的来源。 如果您有兴趣学习/拥有比我在本主题的超短文章中提供的示例更多的示例,那么它是一个很好的参考。
Keep calm, keep coding
保持冷静,保持编码
翻译自: https://medium.com/beautiful-programming/algorithms-and-big-o-notation-f93fb66e7093
算法符号