This tutorial will teach you how to create a dynamic HTML table through the use of Javascript and Document Object Model (DOM) manipulation. The number of table rows and cell content will vary depending on your data.
本教程将教您如何通过使用Javascript和文档对象模型(DOM)操作来创建动态HTML表。 表行数和单元格内容将根据您的数据而变化。
In this tutorial, I will be creating a scoreboard for a Javascript video game and the data will be coming from a fetch request. The source of the data for your application may be different, but the logic should still apply. This tutorial will include screenshots for the code, website rendering, and the full HTML at each step in the tutorial.
在本教程中,我将为Javascript视频游戏创建一个记分板,并且数据将来自获取请求。 您的应用程序的数据源可能有所不同,但逻辑仍然适用。 本教程将在本教程的每个步骤中包括代码,网站渲染和完整HTML的屏幕截图。
The copy-paste-friendly code is at the end. 🔥
复制粘贴友好的代码位于末尾。 🔥
The data for this HTML table comes from an SQLite database table. The number of rows will vary depending on the number of records in the scores table.
该HTML表的数据来自SQLite数据库表。 行数将根据分数表中的记录数而变化。
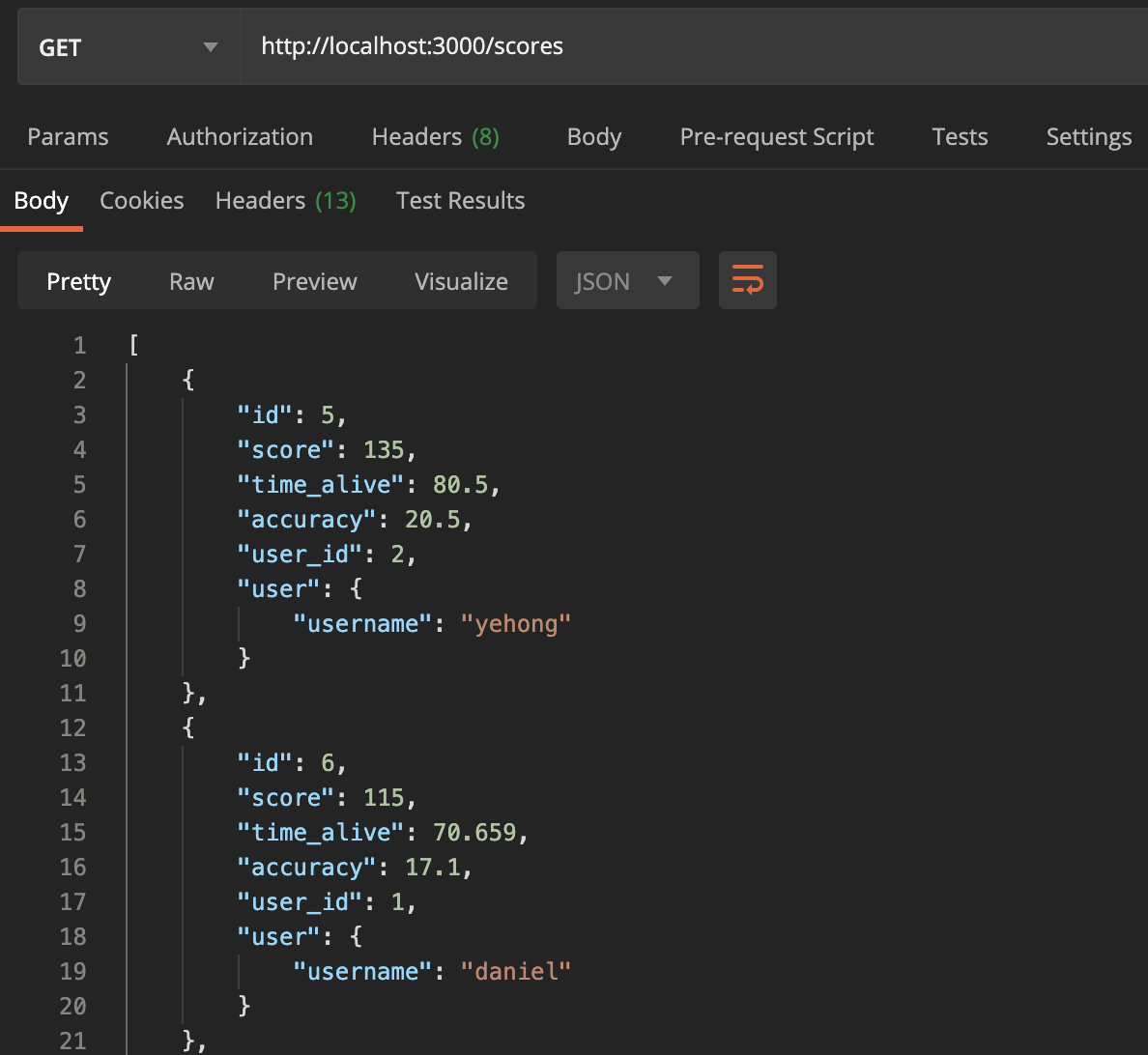
HTML Table Tags:
HTML表格标签:
An HTML table consists of one <table>
element and one or more <tr>, <th>, and <td> elements.
HTML表由一个<table>
元素和一个或多个<tr> , <th>和<td>元素组成。
This HTML table will also include the optional <thead> and <tbody> elements for additional styling options.
该HTML表还将包含可选的<thead>和<tbody>元素,以用于其他样式选项。
Tag Definitions:
标签定义:
The <table>
tag defines an HTML table.
<table>
标签定义一个HTML表。
The <tr>
tag defines a row in an HTML table.
<tr>
标记定义HTML表中的一行。
The <th>
tag defines a header cell in an HTML table.
<th>
标记定义HTML表中的标题单元格。
The <td>
tag defines a standard data cell in an HTML table.
<td>
标记在HTML表中定义标准数据单元。
The <thead>
tag is used to group header content in an HTML table.
<thead>
标记用于将HTML表中的标题内容分组。
The <tbody>
tag is used to group the body content in an HTML table.
<tbody>
标记用于将HTML表格中的正文内容分组。
We will be creating all the tags that are defined above through document method document.createElement(tagName) and we will append them to a <div> tag in our html file.
我们将通过文档方法document.createElement(tagName)创建上面定义的所有标记,并将它们附加到html文件中的<div>标记中。
Most of the ‘classNames’ that you will see in the code are there for styling purposes and are completely optional.
您将在代码中看到的大多数“ className”都是出于样式目的,并且是完全可选的。
The CSS styling will not be discussed, but it will be available for you to reference and test on your own.
不会讨论CSS样式,但是您可以自己参考和测试。
教程: (The Tutorial:)
The <div> that will contain our table is the following:
包含我们的表的<div>如下:
<div class='scoreboard'></div>
Steps to create the table:
创建表的步骤:
Find the ‘scoreboard’ div with the document method document.querySelector(selectors).
使用文档方法document.querySelector(selectors)查找'scoreboard'div 。
- Create an array of strings that holds the header values for the data that you are interested in displaying. I believe these are more representative of the data I am going to display and it allowed me to include units in brackets. (This is an optional step since you can use the object keys as the headers) 创建一个字符串数组,其中包含要显示的数据的标题值。 我相信这些可以更好地代表我将要显示的数据,并且可以在括号中包含单位。 (这是一个可选步骤,因为您可以将对象键用作标题)
let tableHeaders = [“Global Ranking”, “Username”, “Score”, “Time Alive [seconds]”, “Accuracy [%]”]
- Creating the <table>. 创建<table>。
- Creating and appending the <thead> element to the table. This <thead> contains the first <tr> element with all 5 of the strings in the ‘tableHeaders’ variable displayed above as the values inside the respective <th> cell elements. 创建<thead>元素并将其附加到表中。 此<thead>包含第一个<tr>元素,上面显示的'tableHeaders'变量中的所有5个字符串均作为各个<th>单元格元素内的值。
- Creating and appending the <tbody> element to the table. This will allow us to append the <tr> tags that correspond to each score record in our scores table later. 创建<tbody>元素并将其附加到表中。 这将允许我们稍后在分数表中附加与每个分数记录相对应的<tr>标记。
- Appending the <table> onto the ‘scoreboard’ <div>. 将<table>附加到“记分板” <div>上。
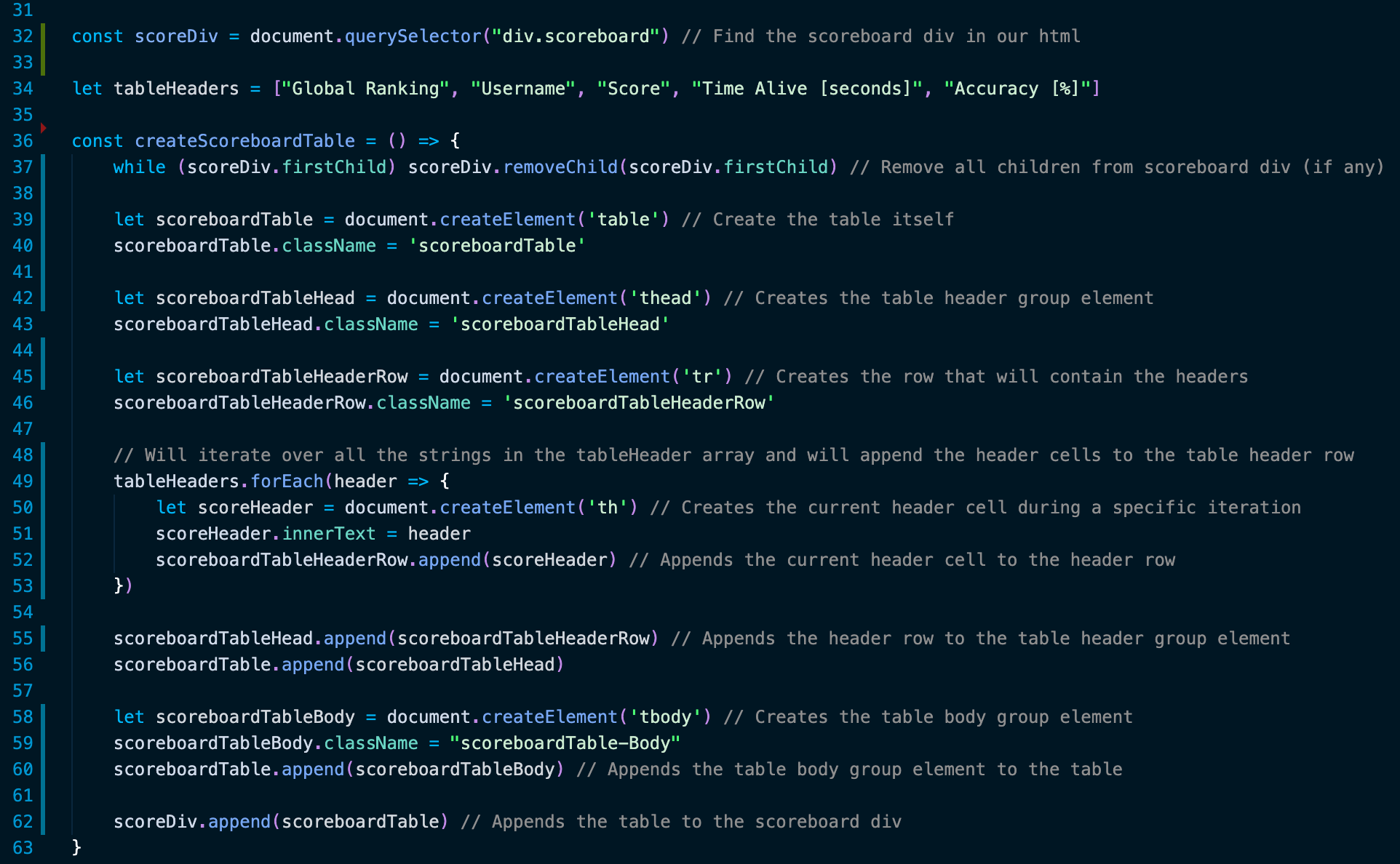

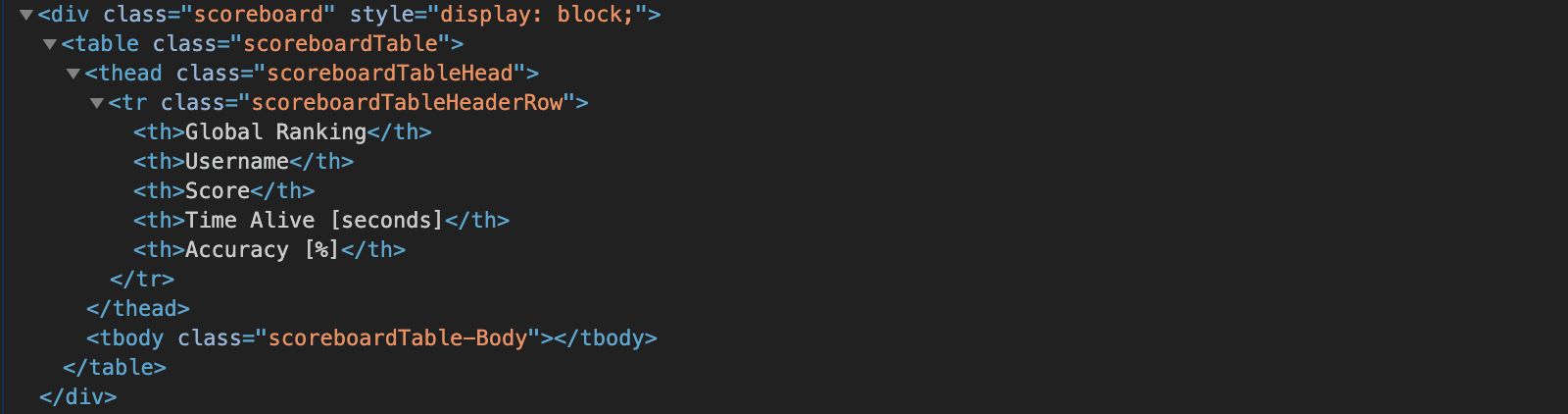
Find the HTML table we created above with the document method document.querySelector(selectors).
使用文档方法document.querySelector(selectors)查找我们在上面创建HTML表。
- Create all of the table body rows that represent each high score. These will be <tr> tags that will hold a <td> tag for each one of the columns in your table. The following function will create a new row when given a score object. 创建代表每个高分的所有表主体行。 这些将是<tr>标记,将为表中的每一列保留一个<td>标记。 给定得分对象时,以下函数将创建一个新行。
Example of the singleScore object that is being passed into the next function:
传递给下一个函数的singleScore对象的示例:
singleScore = { "id": 6, "score": 115, "time_alive": 70.659, "accuracy": 17.1, "user_id": 1, "user": { "username": "daniel" }}
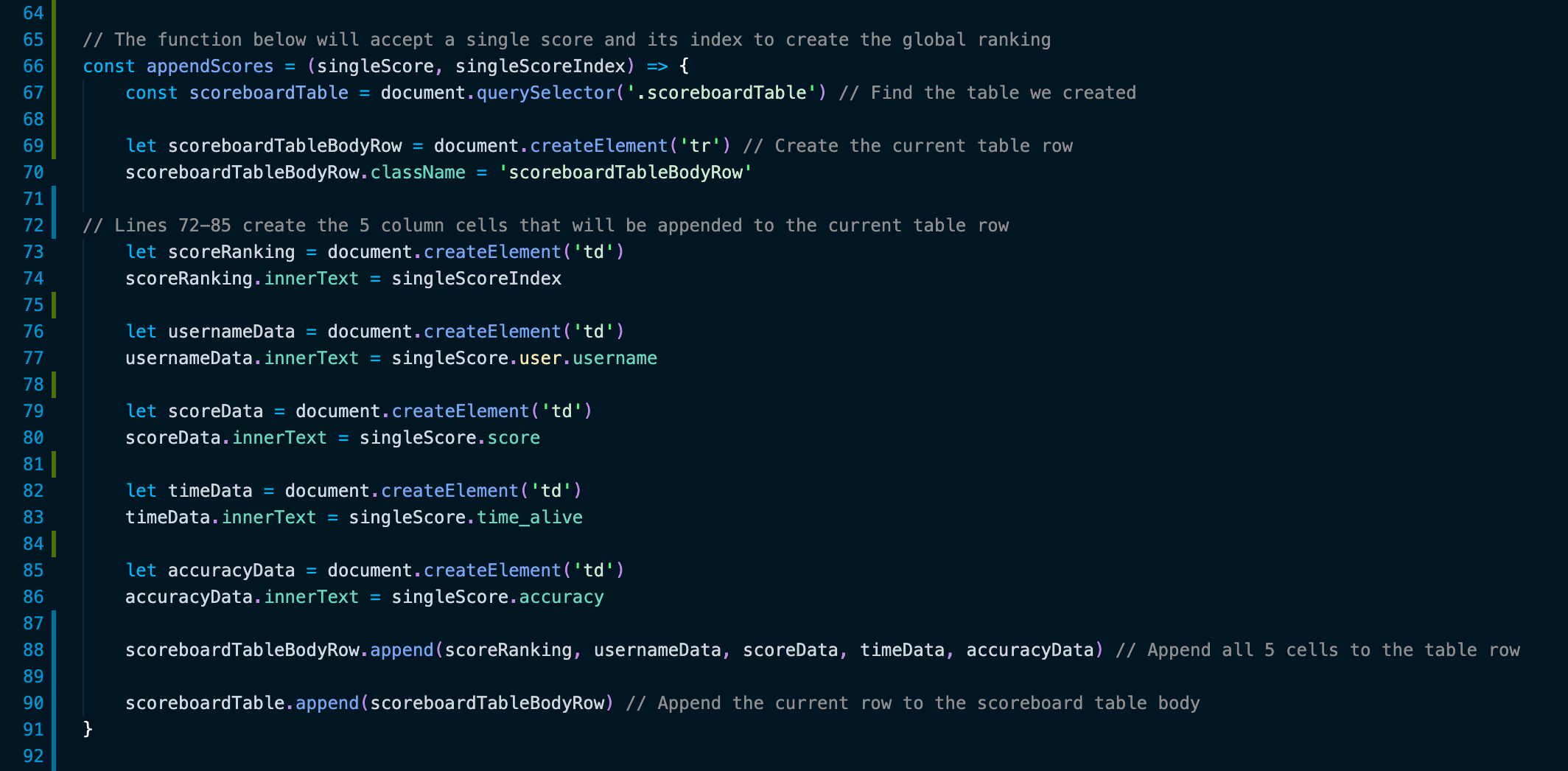
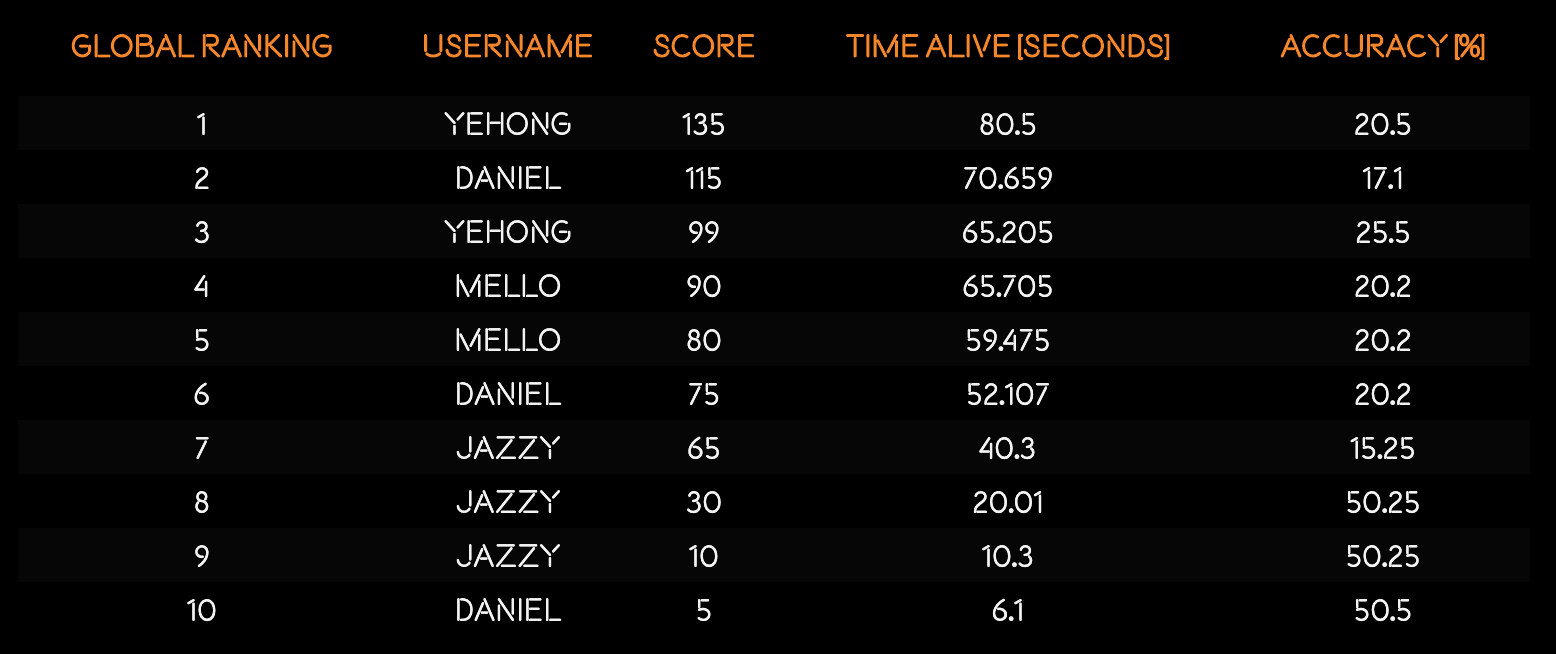
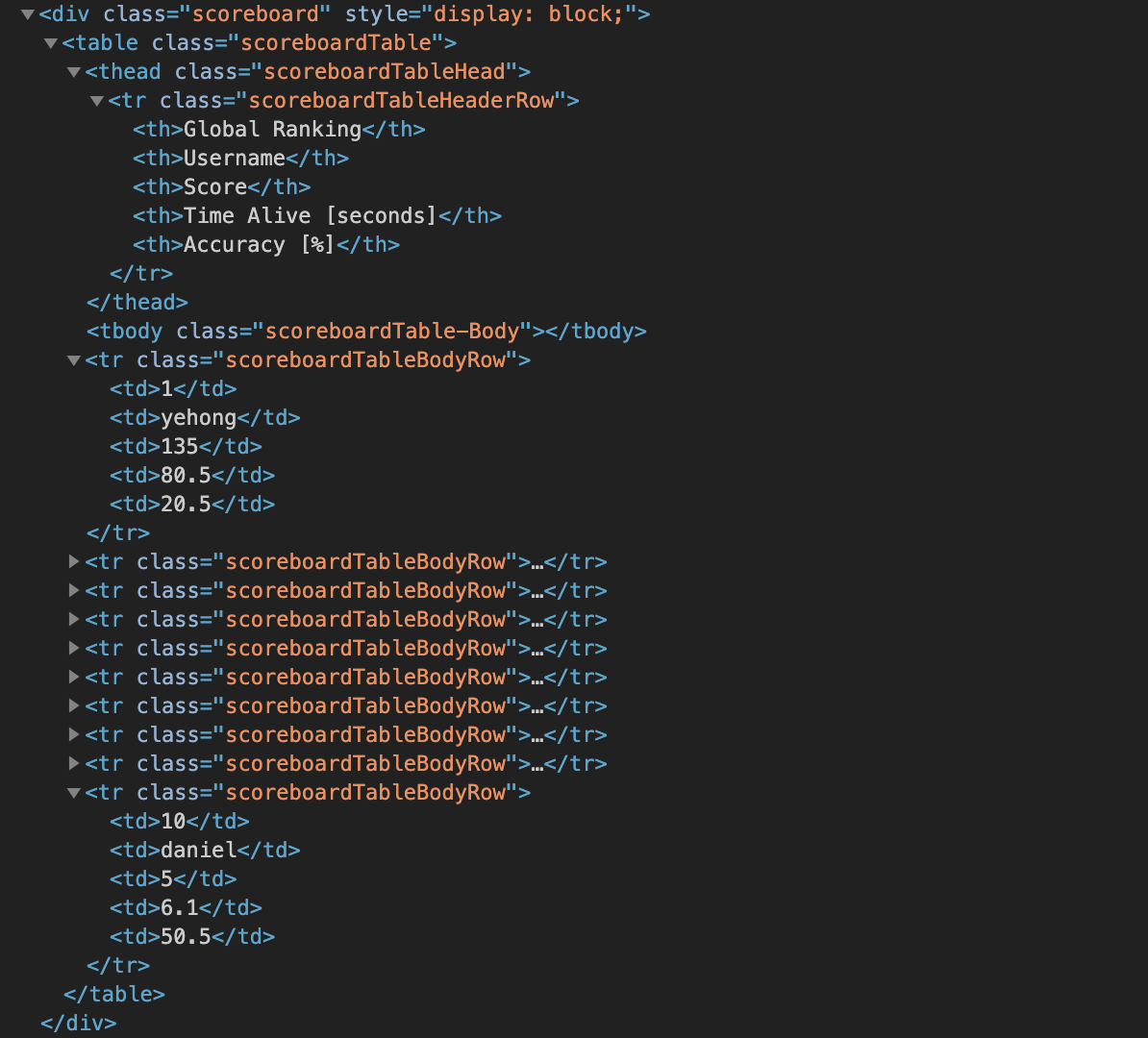
Lastly, I mentioned that this example table relied on a fetch to receive its data. Here is the code for the fetch that supplies all the individual scores to the ‘appendScores’ function:
最后,我提到这个示例表依赖于获取来接收其数据。 这是将所有单独分数提供给'appendScores'函数的获取代码:

I hope you have found this tutorial helpful. If you have any questions, feel free to ask them below.
希望本教程对您有所帮助。 如果您有任何疑问,请随时在下面提问。
Here is a short video of the application that I created this specific table for!
All of the code in the snippets:
片段中的所有代码:
const scoreDiv = document.querySelector("div.scoreboard") // Find the scoreboard div in our htmllet tableHeaders = ["Global Ranking", "Username", "Score", "Time Alive [seconds]", "Accuracy [%]"]const createScoreboardTable = () => {while (scoreDiv.firstChild) scoreDiv.removeChild(scoreDiv.firstChild) // Remove all children from scoreboard div (if any)let scoreboardTable = document.createElement('table') // Create the table itselfscoreboardTable.className = 'scoreboardTable'let scoreboardTableHead = document.createElement('thead') // Creates the table header group elementscoreboardTableHead.className = 'scoreboardTableHead'let scoreboardTableHeaderRow = document.createElement('tr') // Creates the row that will contain the headersscoreboardTableHeaderRow.className = 'scoreboardTableHeaderRow'// Will iterate over all the strings in the tableHeader array and will append the header cells to the table header rowtableHeaders.forEach(header => {let scoreHeader = document.createElement('th') // Creates the current header cell during a specific iterationscoreHeader.innerText = headerscoreboardTableHeaderRow.append(scoreHeader) // Appends the current header cell to the header row})scoreboardTableHead.append(scoreboardTableHeaderRow) // Appends the header row to the table header group elementscoreboardTable.append(scoreboardTableHead)let scoreboardTableBody = document.createElement('tbody') // Creates the table body group elementscoreboardTableBody.className = "scoreboardTable-Body"scoreboardTable.append(scoreboardTableBody) // Appends the table body group element to the tablescoreDiv.append(scoreboardTable) // Appends the table to the scoreboard div}// The function below will accept a single score and its index to create the global rankingconst appendScores = (singleScore, singleScoreIndex) => {const scoreboardTable = document.querySelector('.scoreboardTable') // Find the table we createdlet scoreboardTableBodyRow = document.createElement('tr') // Create the current table rowscoreboardTableBodyRow.className = 'scoreboardTableBodyRow'// Lines 72-85 create the 5 column cells that will be appended to the current table rowlet scoreRanking = document.createElement('td')scoreRanking.innerText = singleScoreIndexlet usernameData = document.createElement('td')usernameData.innerText = singleScore.user.usernamelet scoreData = document.createElement('td')scoreData.innerText = singleScore.scorelet timeData = document.createElement('td')timeData.innerText = singleScore.time_alivelet accuracyData = document.createElement('td')accuracyData.innerText = singleScore.accuracyscoreboardTableBodyRow.append(scoreRanking, usernameData, scoreData, timeData, accuracyData) // Append all 5 cells to the table rowscoreboardTable.append(scoreboardTableBodyRow) // Append the current row to the scoreboard table body}const getScores = () => {fetch('http://localhost:3000/scores') // Fetch for all scores. The response is an array of objects that is sorted in decreasing order.then(res => res.json()).then(scores => {createScoreboardTable() // Clears scoreboard div if it has any children nodes, creates & appends the table// Iterates through all the objects in the scores array and appends each one to the table bodyfor (const score of scores) {let scoreIndex = scores.indexOf(score) + 1 // Index of score in score array for global ranking (these are already sorted in the back-end)appendScores(score, scoreIndex) // Creates and appends each row to the table body}})}
All the CSS styling for the table in this tutorial:
本教程中表格的所有CSS样式:
.scoreboardTable {padding: 0;margin: auto;border-collapse: collapse;width: 80%;text-align: center;color: whitesmoke;}.scoreboardTableHeaderRow {color: darkorange;font-weight: bold;height: 50px;}.scoreboardTableBodyRow:nth-child(odd){background-color: rgba(128, 128, 128, 0.050);}#latestUserScore {background-color: crimson;}.scoreboardTableBodyRow:hover{background-color: darkorange;}.scoreboardTable tr td {height: 25px;}