杰克逊对象映射器(Jackson Object Mapper)
Introducing, the black magic that is the objectMapper. This library is the go to, state of the art, hands down #1 place to go for translating Json to a java class and vis-versa.
引入的黑魔法就是objectMapper。 这个库是最先进的,是将Json转换为Java类(反之亦然)的第一要务。
Here is the link to the library if you want to dive deep in all the things you can do with ObjectMapper.
如果您想深入了解ObjectMapper可以做的所有事情,这是库的链接。
In this short tutorial, we will show how powerful the ObjectMapper is by serializing to and from a Classroom java object — which itself will contain a List of Person Objects — so there will be a decent level of complication since our Classroom is composed of Persons.
在这个简短的教程中,我们将通过在Classroom Java对象之间进行序列化来展示ObjectMapper的功能-该Java对象本身将包含一个Person对象列表-因此,由于我们的Classroom由Persons组成,因此复杂度很高。
将对象存储为Json (Storing Objects as Json)
Java类: (The Java Classes:)
Say we have a classroom object
说我们有一个教室对象
public class ClassRoom {
private String className;
private List<Person> members;
public ClassRoom() {
}
public ClassRoom(String className, List<Person> members) {
this.className = className;
this.members = members;
}
public String getClassName() {
return className;
}
public void setClassName(String className) {
this.className = className;
}
public List<Person> getMembers() {
return members;
}
public void setMembers(List<Person> members) {
this.members = members;
}
}
In this ClassRoom object📚👨🏻🏫📎, we have a String for the name of the class, and a list of members in the class. The members are of the object type Person🙋🏻♂️.
在这个ClassRoom对象中,我们有一个String作为类的名称,并包含该类的成员列表。 成员属于对象类型Person🙋🏻♂️。
public class Person {
private String name;
private int age;
public Person() {
}
public Person(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
}
A Person has a name and an age 👦🏼.
一个人有一个名字和年龄👦🏼。
Now imagine we have a classroom as shown below
现在假设我们有一个教室,如下所示
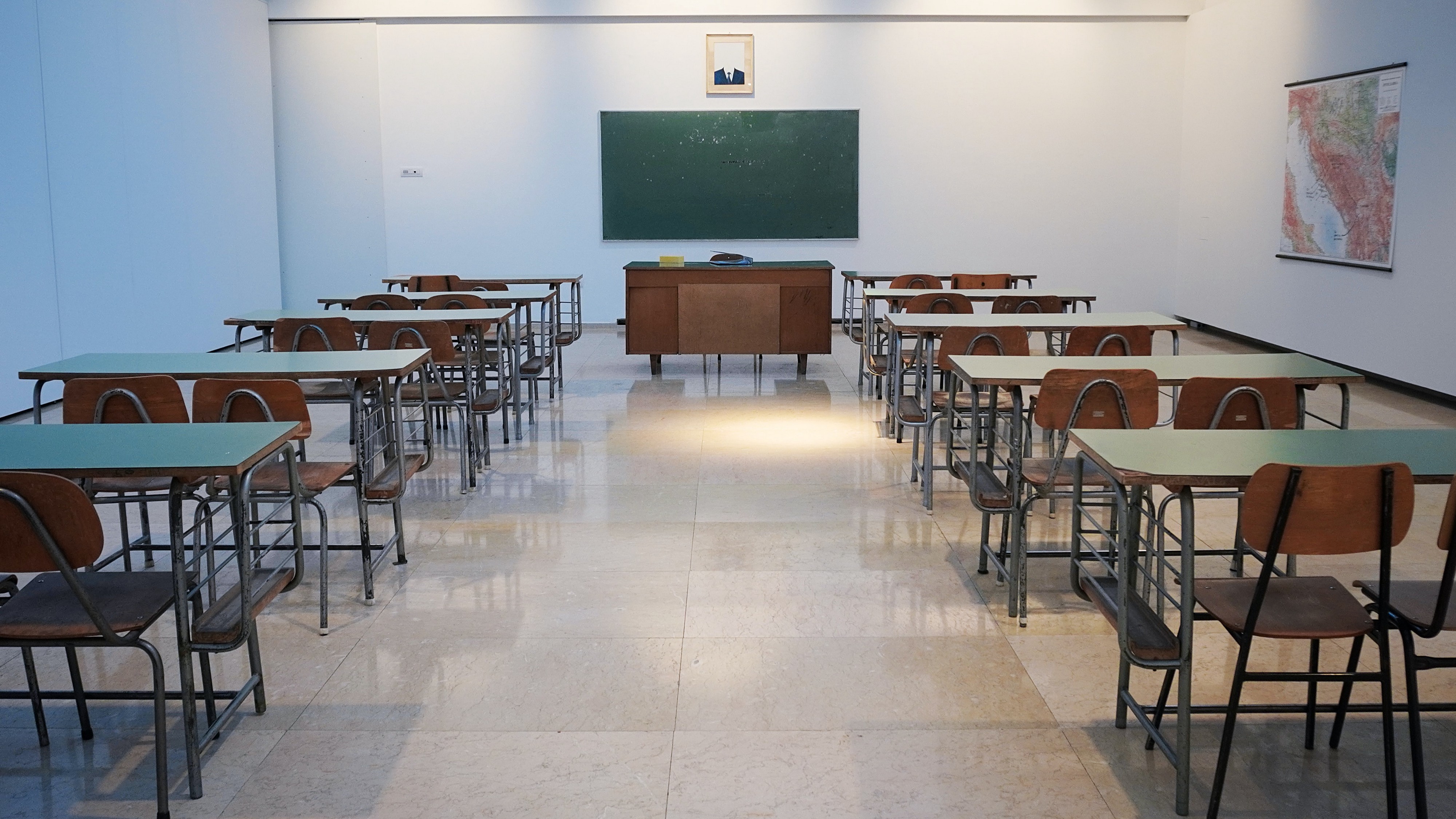
We also have a bunch of students that belong to this classroom, we want to put this Classroom and all of its students 🧑🏽 into a file, but how should we store them? In Json of course!🙌🏻
我们还有一群属于这个教室的学生,我们想将这个Classroom及其所有学生🧑🏽成一个文件,但是我们应该如何存储它们呢? 当然是在杰森!🙌🏻
给Json编写课程: (Writing Our Classes to Json:)
public class ObjectToJson {
public static void main(String[] args) throws IOException {
ObjectMapper objectMapper = new ObjectMapper();
ClassRoom classRoom1 = new ClassRoom("Math 101", Arrays.asList(new Person("Bill", 12), new Person("John", 15), new Person("Matt", 10)));
ClassRoom classRoom2 = new ClassRoom("English 101", Arrays.asList(new Person("Bob", 13), new Person("Alex", 16), new Person("Matt", 10)));
ClassRoom classRoom3 = new ClassRoom("French 101", Arrays.asList(new Person("Jill", 9), new Person("John", 15), new Person("John", 15)));
//write it
String classRoomJson = objectMapper.writeValueAsString(new ClassRoom[]{classRoom1, classRoom2, classRoom3});
System.out.println(classRoomJson);
}
}
Here we create a few classroom objects with three members each on lines 4–6. Then we write the array of classes as Json and store it in a String
在这里,我们在第4-6行中创建了几个教室对象,每个教室都有三个成员。 然后我们将类数组写为Json并将其存储在String中
Below is what we should get on execution.😮⬇️
以下是我们应该执行的内容.😮⬇️
[
{
"className": "Math 101",
"members": [
{
"name": "Bill",
"age": 12
},
{
"name": "John",
"age": 15
},
{
"name": "Matt",
"age": 10
}
]
},
{
"className": "English 101",
"members": [
{
"name": "Bob",
"age": 13
},
{
"name": "Alex",
"age": 16
},
{
"name": "Matt",
"age": 10
}
]
},
{
"className": "French 101",
"members": [
{
"name": "Jill",
"age": 9
},
{
"name": "John",
"age": 15
},
{
"name": "John",
"age": 15
}
]
}
]
从Json读取对象 (Reading Objects From Json)
To do this, 🤓📚 we need every object we will be creating from the Json to have a no params constructor and getter and setters for all fields.
为此,🤓📚我们需要将要从Json创建的每个对象都具有无参数的构造函数以及所有字段的getter和setter。
将我们的Json重新读入Java对象: (Reading our Json back into Java Objects:)
public class ObjectToJson {
public static void main(String[] args) throws IOException {
ObjectMapper objectMapper = new ObjectMapper();
ClassRoom classRoom1 = new ClassRoom("Math 101", Arrays.asList(new Person("Bill", 12), new Person("John", 15), new Person("Matt", 10)));
ClassRoom classRoom2 = new ClassRoom("English 101", Arrays.asList(new Person("Bob", 13), new Person("Alex", 16), new Person("Matt", 10)));
ClassRoom classRoom3 = new ClassRoom("French 101", Arrays.asList(new Person("Jill", 9), new Person("John", 15), new Person("John", 15)));
//write it
String classRoomJson = objectMapper.writeValueAsString(new ClassRoom[]{classRoom1, classRoom2, classRoom3});
//System.out.println(classRoomJson);
//read back
List<ClassRoom> classRooms = objectMapper.readValue(classRoomJson, new TypeReference<List<ClassRoom>>(){});
classRooms.forEach((classRoom) -> {
System.out.println(classRoom.getClassName());
classRoom.getMembers().forEach((person) -> {
System.out.println(String.format("\t%s %d", person.getName(), person.getAge()));
});
});
}
}
Here, we will read back the Json we just made and store it into objects. On line 14 we read the value we created as a List of ClassRooms 📚✏️📏, we need to specify what we expect from the Json by passing the second argument
在这里,我们将读回刚刚制作的Json并将其存储到对象中。 在第14行,我们读取了作为ClassRooms列表创建的值,我们需要通过传递第二个参数来指定对Json的期望
new TypeReference<List<ClassRoom>>(){}
on lines 15,16 for each classroom we print out its name, then on lines 17,18 for each member of a classroom we print out that member’s name and age.
在第15,16行,为每个教室打印我们的名字,然后在第17,18行,为每个教室成员打印我们的名字和年龄。
We should get the following output.🎉
我们应该得到以下输出。
Math 101
Bill 12
John 15
Matt 10
English 101
Bob 13
Alex 16
Matt 10
French 101
Jill 9
John 15
John 15
Done! You have just stored an object to json and read it back like a pro 😎.
做完了! 您刚刚将对象存储到json并像pro😎一样将其读回。