javascript 过滤
Umm…
嗯...
So, what exactly are map(), filter() and reduce()?
那么,map(),filter()和reduce()到底是什么?
They are JavaScript methods of ECMAScript 5 version used to manipulate data in an array.
它们是ECMAScript 5版本JavaScript方法,用于处理数组中的数据。
These methods help in managing complexity and improving readability.
这些方法有助于管理复杂性并提高可读性。
Let’s Buckle up and land on ECMAScript 5 planet to know more about these methods.
让我们紧扣并着陆在ECMAScript 5星球上,以了解有关这些方法的更多信息。
格式 (Format)
Here, I will go over each method in the following format
在这里,我将以以下格式介绍每种方法
- The What (with an orange analogy😉)什么(用橙色比喻😉)
- Input and Output 输入输出
- Syntax句法
- Examples (with comic heroes 😍)例子(漫画英雄😍)
- Takeaways 外卖
- Conclusion结论
地图()(Map())
什么啊 (The What?)
map() is a method applied on arrays which return a new array after performing given action on each element without disturbing the original array.
map()是一种应用于数组的方法,该方法在对每个元素执行给定操作后不干扰原始数组的情况下返回一个新数组。
Imagine you have a basket full of oranges and want to peel each one of them. Also, you want to place peeled oranges in a new basket.
想象一下,您有一个装满橙子的篮子,想剥每个橙子。 另外,您想将去皮的橘子放在新的篮子里。
I know, such a tedious task. Ta-da!! you do have an assistant named map. Now, what’s the plan?
我知道,这是一个乏味的任务。 - 您确实有一个名为map的助手。 现在有什么计划?
You give him a basket full of oranges and ask him to peel. You tell him how to peel too. Easy!!
您给他装满橙子的篮子,请他剥皮。 你也告诉他怎么剥皮。 简单!!
He peels each orange and gives a new basket full of peeled ones.
他给每个橘子去皮,然后给一个装满去皮的新篮子。
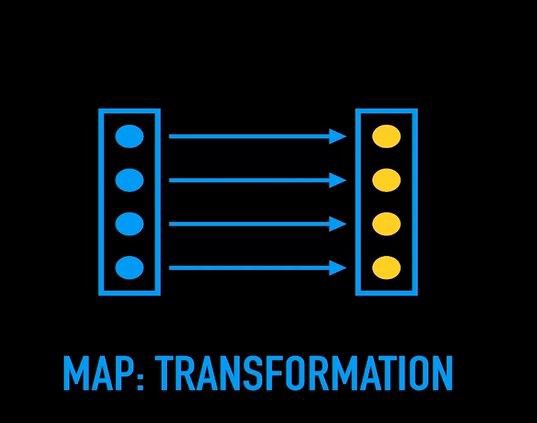
输入输出 (Input and Output)
input is an array
输入是一个数组
output is a new array with the provided actions performed
输出是执行了提供的操作的新数组
何时使用(When to use)
- Perform an action on every value of an iterable对可迭代的每个值执行操作
- When you want a whole new array without disturbing the original array while performing a set of actions on the array当您想要一个全新的数组而又不干扰原始数组同时在该数组上执行一组操作时
句法(Syntax)
Let’s go through each parameter,
让我们浏览每个参数,
function(): a callback function
function() :一个回调函数
currentValue: It’s value of the current element (required parameter)
currentValue :当前元素的值(必需参数)
index: It’s index of the current element (optional parameter)
index :当前元素的索引(可选参数)
arr: It’s the array the map was called upon ie.., the original array (optional parameter)
arr :这是地图被调用的数组,即原始数组(可选参数)
thisArg: Value to use as this inside the callback (optional parameter)
thisArg :在回调中用作此值的值(可选参数)
例子 (Examples)
- First, let’s go over the basic example of doubling integer values in an array首先,让我们看一下将数组中的整数值加倍的基本示例
- And deal with objects并处理对象
So, first, let me explain how we could solve a basic problem without using map and then why map is a better option.
因此,首先让我解释一下如何在不使用map的情况下解决基本问题,然后解释为什么map是更好的选择。
Code to double integers in an array using for, forEach, and map
代码使用for,forEach和map将数组中的整数加倍
Using for
用于
Using forEach
使用forEach
Using map
使用地图
Takeaways:
外卖:
map vs for/forEach: We don’t need to create an empty array and push the new values into it while using map()
map vs for / forEach :使用map()时,我们不需要创建一个空数组并将新值推送到其中
map vs for: We don’t need to maintain the index of the item
map vs for :我们不需要维护商品的索引
对象和地图(Objects and Map)
- Without map没有地图
- We don’t need to create a whole new array when we use map.使用map时,我们不需要创建一个全新的数组。
过滤() (filter())
什么啊 (The What?)
Filter() is a method applied on arrays which returns a new array with all elements that pass the test implemented by the provided function. It returns an empty array if none of the elements pass the test. It doesn’t change the original array.
Filter()是应用于数组的方法,该方法返回一个新数组,该数组包含所有通过提供的函数实现的测试的元素。 如果没有任何元素通过测试,它将返回一个空数组。 它不会更改原始数组。
Imagine you have a basket full of oranges and want to peel those which are in good condition. Also, you want to place the peeled ones in a new basket.
想象一下,您有一个装满橙子的篮子,想剥掉状况良好的篮子。 另外,您想将去皮的面包放在新的篮子里。
Again, you have an assistant named filter. So, what’s the plan?
同样,您有一个名为filter的助手。 那么,有什么计划?
You give him the basket and ask him to peel those which are in good condition (ie.., filter in). You tell him how to find an orange which is in a good condition.
您给他篮子,请他去掉那些状况良好的东西(即过滤)。 您告诉他如何找到状况良好的橙子。
Work was done!! he peels those which are in good condition and returns a new basket filled with them.
工作完成了!! 他将状况良好的果皮去皮,并返回装满它们的新篮子。
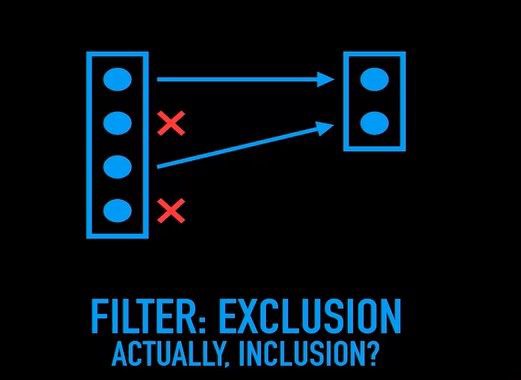
Remember, we filter-in the values, not filter-out ie.., the elements which evaluate to true will be added to the array.
记住,我们过滤掉值,而不是过滤掉,即,将评估为true的元素添加到数组中。
输入输出 (Input and Output)
input is an array
输入是一个数组
output is a new array with elements that pass the condition.
输出是一个具有通过条件的元素的新数组。
何时使用 (When to use)
- When you want a whole new array with desired values.当您想要一个具有所需值的全新数组时。
句法 (Syntax)
- It’s the same as map和地图一样
Just like map parameters,
就像地图参数一样
currentValue: It’s the value of the current element (required parameter)
currentValue :当前元素的值(必需参数)
index: It’s the index of the current element (optional parameter)
index :当前元素的索引(可选参数)
arr: It’s the array the map was called upon ie.., the original array (optional parameter)
arr :这是地图被调用的数组,即原始数组(可选参数)
thisArg: Value to use as this inside the callback (optional parameter)
thisArg :在回调中用作此值的值(可选参数)
例子 (Examples)
- First, let’s go over the basic example of finding even numbers in an array首先,让我们看一下在数组中查找偶数的基本示例
- And deal with objects并处理对象
Finding Even numbers in an array
在数组中查找偶数
Takeaways:
外卖:
- The above problem can be solved using for or forEach. But, we need to create a new empty array and push the values into it 可以使用for或forEach解决以上问题。 但是,我们需要创建一个新的空数组并将值推入其中
对象和过滤器(Objects and filter)
Let’s take the following example
让我们来看下面的例子
- Let’s find those who don’t belong to DC让我们找到那些不属于DC的人
Without filter
不带过滤器
With filter
带过滤器
Takeaways:
外卖:
- With filter, we can write less complex and more readable code 使用过滤器,我们可以编写更简单,更易读的代码
- Also, we don’t need to create a whole new array另外,我们不需要创建一个全新的数组
减少()(Reduce())
什么啊 (The What?)
Reduce() is a method applied on arrays which executes a reducer function on each element of the array and returns a single output. It won’t execute the function if the array is empty.
Reduce()是应用于数组的一种方法,该方法在数组的每个元素上执行reducer函数并返回单个输出。 如果数组为空,它将不会执行该函数。
Imagine you have a basket full of oranges. You want to make a jar full of juice with all of them.
假设您有一个装满橙子的篮子。 您想用所有这些制成一个装满果汁的罐子。
Again, you have an assistant named reducer. What are you waiting for? 😃
同样,您有一个名为reducer的助手。 你在等什么? 😃
You give him a basket full of oranges and ask him to make juice. You tell him how to make it.
您给他装满橙子的篮子,请他做果汁。 你告诉他怎么做。
He takes all oranges and gives you a jar of juice (a good assistant 😜)
他拿走了所有的橘子,给了你一罐果汁(好助手😜)
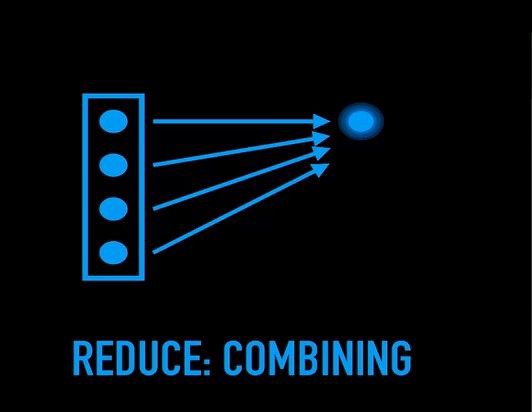
输入输出(Input and Output)
- Input is an array输入是一个数组
- Output is a single value of the desired type输出是所需类型的单个值
何时使用(When to use)
- When you want to deal with each element of an array to get a single desired output当您要处理数组的每个元素以获取单个所需的输出时
句法(Syntax)
Let’s go through each parameter,
让我们浏览每个参数,
accumulator: The initialValue or the previously returned value of the function (Required parameter)
累加器:函数的initialValue或先前返回的值(必需参数)
currentValue: The current element of the array (Optional parameter)
currentValue :数组的当前元素(可选参数)
currentIndex: Current index of the element (Optional parameter)
currentIndex :元素的当前索引(可选参数)
arr: The original array (Optional parameter)
arr :原始数组(可选参数)
initialValue: Initial value of the accumulator. If nothing is passed, it used the first element of the array as the initial accumulator value (Optional parameter)
initialValue :累加器的初始值。 如果未传递任何内容,它将使用数组的第一个元素作为初始累加器值(可选参数)
例子 (Examples)
- Let’s go over a basic example of summing up the values of an array让我们来看一个汇总数组值的基本示例
- And deal with objects并处理对象
Summing up the values of an array
总结一个数组的值
Objects and Reduce
目标与归约
- To get heroes who belong to DC获得属于DC的英雄
Takeaways:
外卖:
- When you want to deal with every element in an array desiring a single value output 当您要处理数组中的每个元素并需要单个值输出时
- Also, if you want to get a single value output passing a condition, use the filter, and reduce together.另外,如果要获得通过条件的单个值输出,请使用过滤器,然后一起减少。
结论 (Conclusion)
- Use map(), filter() when you want to perform actions but, don’t want to disturb the original array当您想执行操作但不想干扰原始数组时,请使用map(),filter()
- map(), filter() return a whole new array map(),filter()返回一个新数组
- Use reduce() when you want to use every element of an array to get a single desired value当您要使用数组的每个元素以获取单个所需值时,请使用reduce()
- Using map(), filter() and reduce() make your code less complex and more readable 使用map(),filter()和reduce()可使您的代码更简单,更易读
Thanks to Kyle Simpson, javaScript Teacher and FreeCodeCamp!
感谢Kyle Simpson,javaScript Teacher和FreeCodeCamp!
翻译自: https://medium.com/swlh/javascript-map-filter-reduce-illustrated-83897f144613
javascript 过滤