flutter 数据加载
Fetching data from a database and displaying it on a list is a very common task in app development. One crucial task that’s often neglected when doing this, especially by new developers, is lazy loading the data to avoid fetching a whole collection, making your app slower and racking up your bill.
从数据库中获取数据并将其显示在列表上是应用程序开发中非常常见的任务。 执行此操作时经常被忽略的一项关键任务,尤其是对于新开发人员而言,是懒惰地加载数据以避免获取整个集合,这会使您的应用程序变慢并增加费用。
Paginating or lazy loading your data using Firestore is relatively simple — that is if you’re getting the documents once. When you attempt to lazy load your data using streams to keep your data updated in real time, it’s a whole other story.
使用Firestore分页或延迟加载数据相对简单-也就是说,一次获取文档即可。 当您尝试使用流来延迟加载数据以保持数据实时更新时,这完全是另一回事了。
In this article, I’m going to teach you a technique to lazy load your data from Firestore in real time using the Bloc pattern. If you don’t know anything about the Bloc pattern, I suggest you read their documentation to get an idea of how it works.
在本文中,我将教您一种使用Bloc模式从Firestore实时延迟加载数据的技术。 如果您对Bloc模式一无所知,建议您阅读他们的文档,以了解其工作原理。
我们正在建设 (What We’re Building)
We’ll build a simple app that lazy loads posts from a Firestore collection and displays them on a ListView
.
我们将构建一个简单的应用程序,以延迟加载Firestore集合中的帖子并将其显示在ListView
。
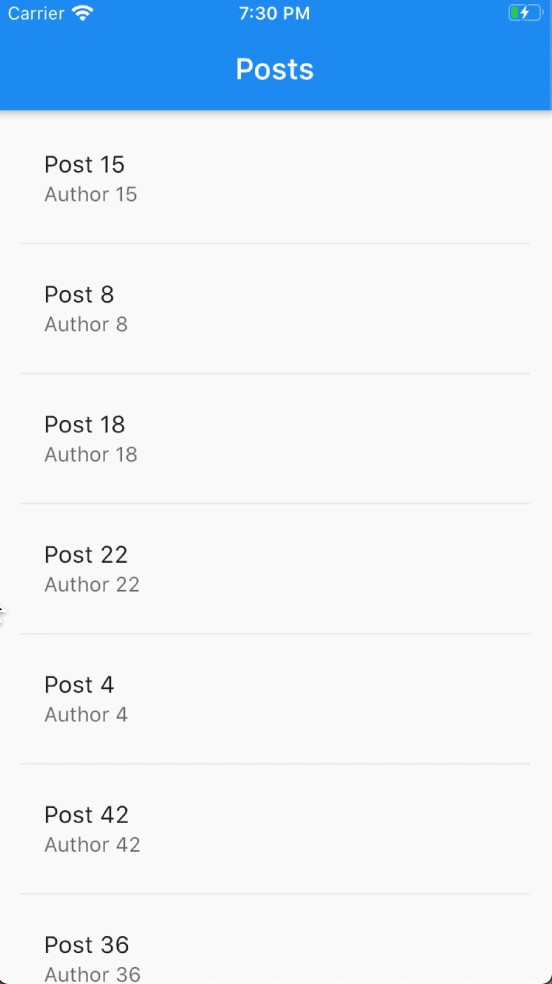
项目创建 (Project Creation)
Start by creating a new Flutter project:
首先创建一个新的Flutter项目:
$ flutter create lazy_loading_demo$ cd lazy_loading_demo
Add the following dependencies to your pubspec.yaml
:
将以下依赖项添加到您的pubspec.yaml
:
firebase_core: ^0.5.0
cloud_firestore: ^0.14.0+2
flutter_bloc: ^6.0.5
equatable: ^1.2.5
Next, go to the Firebase console, and create a new project. Once the project is created, enable Firestore, and follow the instructions to add it to your app.
接下来,转到Firebase控制台,然后创建一个新项目。 创建项目后,启用Firestore,然后按照说明将其添加到您的应用中。
帖子库 (Post Repository)
We’re going to need to create a repository that our Bloc can use to fetch data from Firestore. Create a new folder named supplemental
, and then create post_repository.dart
inside of it. Add the following code:
我们将需要创建一个存储库,Bloc可以使用该存储库从Firestore提取数据。 创建一个名为supplemental
的新文件夹,然后在其中创建post_repository.dart
。 添加以下代码:
import 'package:cloud_firestore/cloud_firestore.dart';
class PostRepository {
// Singleton boilerplate
PostRepository._();
static PostRepository _instance = PostRepository._();
static PostRepository get instance => _instance;
// Instance
final CollectionReference _postCollection = FirebaseFirestore.instance.collection('posts');
Stream<QuerySnapshot> getPosts() {
return _postCollection
.limit(15)
.snapshots();
}
Stream<QuerySnapshot> getPostsPage(DocumentSnapshot lastDoc) {
return _postCollection
.startAfterDocument(lastDoc)
.limit(15)
.snapshots();
}
}
Now we have a simple repository we can use to get our data. The code is relatively easy to understand: getPosts
returns a stream containing the first 15 documents of the posts
collection. getPostsPage
returns a stre