java中的组合与继承
Java中的继承是什么?(What is Inheritance in Java?)
Inheritance is a process of defining a new class by using already defined class so that newly defined class can use data members(attributes) and member functions (methods) of the already defined class.
继承是通过使用已定义的类来定义新类的过程,以便新定义的类可以使用已定义的类的数据成员(属性)和成员函数(方法)。
Already defined class is called as Parent class or Base Class or Super class .
已经定义的类称为父类或基类或超类。
Newly defined class is called as Child class or derived class or Sub class .
新定义的类称为Child类或派生类或Sub类。
继承的优点: (Advantages of Inheritance :)
- Newly created class (child class) can use the attributes(instance variables ) and methods (instance methods ) of already defined class (Parent class ) without re-defining them in child class. This way code re-usability is improved. 新创建的类(子类)可以使用已定义的类(父类)的属性(实例变量)和方法(实例方法),而无需在子类中重新定义它们。 这样,代码的可重用性得到了改善。
- A child class can have its own instance variables and instance methods. 子类可以具有自己的实例变量和实例方法。
- A child class can override the parent class instance methods. 子类可以覆盖父类的实例方法。
NOTE : When child class inherits parent class , all the instance variables and instance methods are accessible with child class object depending on access modifiers (private/default/protected/public ) used for instance variable and methods in parent class.Basically one copy of variables and methods of parent class is created in child class [just for understanding].
注:当子类继承父类,所有实例变量和实例方法与根据访问修饰符子类的对象使用实例变量和变数父class.Basically一个复制方法(私有/默认/保护/公共)访问父类的方法是在子类中创建的(仅供理解)。
NOTE :When instance variables and methods of parent class have public or protected access modifier then child class object can directly access them but if they are declared as private then child object won’t be able to access them directly and hence public getters and setters are required for such private variables .
注意:当父类的实例变量和方法具有公共或受保护的访问修饰符时,子类对象可以直接访问它们,但是如果将它们声明为私有,则子对象将无法直接访问它们,因此公共获取器和设置器是这种私有变量必需的。
private variables are visible to the class in which they are declared.
私有变量对于声明它们的类是可见的。
继承的语法: (The syntax for inheritance :)
public class Parent {//instance variables of parent class//instance methods of parent class}public class Child extends Parent{//instance variables of child class//instance methods of child class}
Let’s see an example of Inheritance.
让我们来看一个继承的例子。
Animal class as parent class.
动物类为父类。
package com.vikram;
public class Animal {
//instance variables of Animal class
public String name;
public String body;
public boolean brain;
//parameterized constructor to initialized instance variables
public Animal(String name, String body, boolean brain) {
this.name = name;
this.body = body;
this.brain = brain;
}
//public getters and setters for instance variables
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getBody() {
return body;
}
public void setBody(String body) {
this.body = body;
}
public boolean getBrain() {
return brain;
}
public void setBrain(boolean brain) {
this.brain = brain;
}
//instance methods of Animal class
public void move() {
System.out.println("Animal move() called...");
}
public void eat() {
System.out.println("Animal eat() called...");
}
}
Dog as a child class of Animal class.
狗作为动物类的子类。
package com.vikram;
public class Dog extends Animal{
//instance variables of Dog class
public int legs;
public String color;
//parameterized constructor to initialize instance variables
public Dog(String name, String body, boolean brain, int legs, String color) {
super(name, body, brain);
this.legs = legs;
this.color = color;
}
//getters and setters for instance variables
public int getLegs() {
return legs;
}
public void setLegs(int legs) {
this.legs = legs;
}
public String getTail() {
return color;
}
public void setTail(String tail) {
this.color = tail;
}
//instance methods of Dog class
public void run(){
System.out.println("Dog run() called... ");
}
//overriding move method of Animal class in dog class
@Override
public void move() {
System.out.println("Dog move() called... ");
super.move();//calling move() of Animal class.
}
}
Main as driver class
主要作为驾驶员
package com.vikram;
class Main {
public static void main(String args[]) {
//creating object of Animal class and calling instance methods
Animal animal = new Animal("Elephant","Big",true);
animal.eat();
animal.move();
//creating object of Dog class and calling Animal and dog instance methods
Dog dog = new Dog("Jeffy","Medium",true,4,"Black");
dog.run();//calling run() method of Dog class
dog.move();//calling move() method of Dog class
dog.eat();//calling eat() method of Animal class
}
}
In the above example, Animal is parent class and Dog is child class. Now we can say that all the instance variables (attribute) and instance methods of parent class are part of child class as well. Hence we can say that every Dog is an Animal. So in the case of inheritance, there is always an IS-A relationship between subclass and superclass.
在上面的示例中, Animal是父类, Dog是子类。 现在我们可以说父类的所有实例变量(属性)和实例方法也都是子类的一部分。 因此,我们可以说每只狗都是动物。 因此,在继承的情况下,子类和超类之间始终存在IS-A关系。
构造函数如何与继承一起使用? (How do constructors work with Inheritance?)
When a child class object is created it invokes a constructor and this child class constructor invokes the parent constructor. Let’s see an example to understand this …
创建子类对象时,它将调用构造函数,而此子类构造函数将调用父构造函数。 让我们看一个例子来理解这个……
package com.vikram;
//parent class
class Person{
public Person() {
System.out.println("Parent constructor called!!!");
}
}
//child class inheriting parent class
class Employee extends Person{
public Employee() {
/*
here super() call is implicit but we can explicitly write it when
we want to call parent class parameterized constructor using
super(param).
*/
System.out.println("Child constructor called!!!");
}
}
//driver class
class Main {
public static void main(String args[]) {
Employee employee = new Employee();
}
}
输出:(OUTPUT :)
Parent constructor called!!!
Child constructor called!!!
You can see that when a Child class object is created then the child’s no-argument constructor is called but internally the first statement in this constructor is a super call to parent class constructor which is hidden. So first parent class is loaded and its constructor is executed then child class is loaded and its constructor is executed in JVM.
您可以看到,当创建Child类对象时,将调用该子级的无参数构造函数,但是在内部,该构造函数中的第一条语句是对父类构造函数的超级调用,该父调用已隐藏。 因此,首先加载父类并执行其构造函数,然后加载子类并在JVM中执行其构造函数。
有哪些不同类型的继承? (What are the different types of Inheritance?)
- Single Inheritance 单继承
- Multilevel Inheritance多级继承
- Multiple Inheritance多重继承
- Hierarchical Inheritance层次继承
- Hybrid Inheritance混合继承
1.单一继承:(1. Single Inheritance :)
One class extends other class eg. class B extends class A.
一类扩展了另一类,例如。 B级扩展了A级。
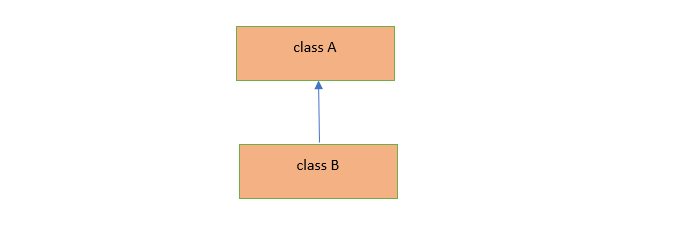
2,多级继承(2.Multilevel Inheritance :)
In this type of inheritance, class B extends class A and class C extends class B.
在这种继承中,类B扩展了类A,而类C扩展了类B。
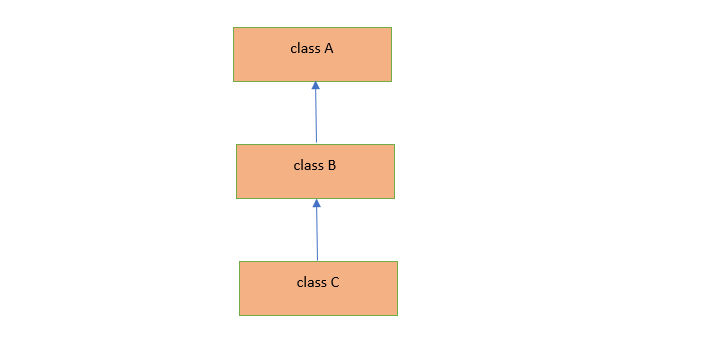
3,多重继承(3.Multiple Inheritance :)
In this type of inheritance one class A extends two classes B and C.
在这种继承类型中,一个类A扩展了两个类B和C。
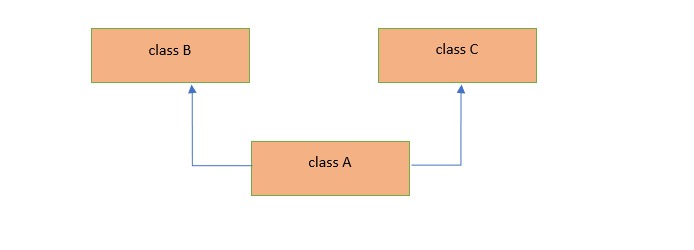
Note: Java doesn’t support multiple inheritances.
注意:Java不支持多重继承。
4,层次继承 (4.Hierarchical Inheritance :)
In this type, more than one class extends the same parent class.
在这种类型中,一个以上的类扩展了相同的父类。
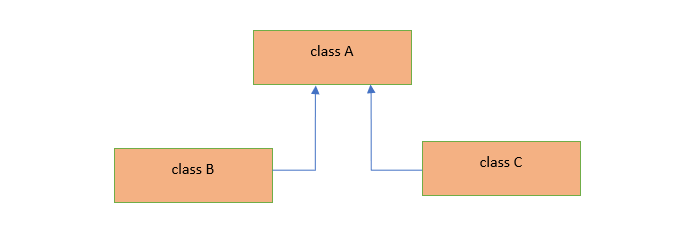
5,混合继承(5.Hybrid Inheritance :)
Combining two or more types of above inheritance will be a hybrid inheritance.
组合两种或多种上述继承将成为混合继承。
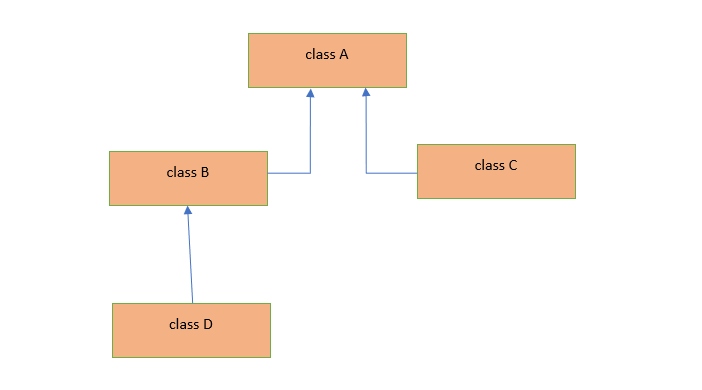
Java中的成分是什么?(What is Composition in Java?)
The composition is another OOPs feature in Java and there exists “HAS-A” a relationship between the classes .Eg. Let's say we have two classes Car and Engine. Then there is a relationship of “HAS a” between Car and Engine. So we can say Car has an Engine.
组合是Java中的另一种OOPs功能,并且类之间存在“ HAS-A ”关系。 假设我们有Car和Engine这两个类。 在汽车和发动机之间存在“ HAS a ”的关系。 所以我们可以说Car有引擎。
So there will be two classes Car and Engine and Car class will have an instance variable of Engine class.
因此将有Car和Engine这两个类,而Car类将具有Engine类的实例变量。
Let’s see the program for the same.
让我们来看看相同的程序。
Engine class
引擎等级
public class Engine {
public String fuelType;
public int cc;
public int maxTorque;
public int maxPower;
public Engine(String fuelType, int cc, int maxTorque, int maxPower) {
this.fuelType = fuelType;
this.cc = cc;
this.maxTorque = maxTorque;
this.maxPower = maxPower;
}
public String getFuelType() {
return fuelType;
}
public void setFuelType(String fuelType) {
this.fuelType = fuelType;
}
public int getCc() {
return cc;
}
public void setCc(int cc) {
this.cc = cc;
}
public int getMaxTorque() {
return maxTorque;
}
public void setMaxTorque(int maxTorque) {
this.maxTorque = maxTorque;
}
public int getMaxPower() {
return maxPower;
}
public void setMaxPower(int maxPower) {
this.maxPower = maxPower;
}
@Override
public String toString() {
return "Engine{" +
"fuelType='" + fuelType + '\'' +
", cc=" + cc +
", maxTorque=" + maxTorque +
", maxPower=" + maxPower +
'}';
}
}
Car class
车类
public class Car {
public String brandName;
public String color;
public Engine engine;
public String model;
public Car(String brandName, String color, Engine engine, String model) {
this.brandName = brandName;
this.color = color;
this.engine = engine;
this.model = model;
}
public String getBrandName() {
return brandName;
}
public void setBrandName(String brandName) {
this.brandName = brandName;
}
public String getColor() {
return color;
}
public void setColor(String color) {
this.color = color;
}
public Engine getEngine() {
return engine;
}
public void setEngine(Engine engine) {
this.engine = engine;
}
public String getModel() {
return model;
}
public void setModel(String model) {
this.model = model;
}
@Override
public String toString() {
return "Car{" +
"brandName='" + brandName + '\'' +
", color='" + color + '\'' +
", engine=" + engine +
", model='" + model + '\'' +
'}';
}
}
Main as driver class
主要作为驾驶员
public class Main {
public static void main(String args[]){
Engine engine = new Engine("Petrol",1498,98,200);
Car car = new Car("Honda","Silver",engine,"2020");
System.out.println("Car Object is : "+car);
}
}
输出:(OUTPUT :)
Car Object is : Car{brandName=’Honda’, color=’Silver’, engine=Engine{fuelType=’Petrol’, cc=1498, maxTorque=98, maxPower=200}, model=’2020'}
The advantage of using composition is that we can control the visibility/accessibility of instance variable engine in the Car class.
使用组合的好处是我们可以控制Car类中实例变量引擎的可见性/可访问性。
Both Inheritance and Composition improve code re-usability.
继承和组合都可以提高代码的可重用性。
继承和组合有什么区别? (What are the differences between Inheritance and Composition?)
- Inheritance is the “IS-A” relationship between the child and parent class whereas the composition is the “HAS-A” relationship between two classes. 继承是子类和父类之间的“ IS-A”关系,而构成是两个类之间的“ HAS-A”关系。
2. In the case of an inheritance child class can override parent class instance methods but this is not applicable in the case of composition.
2.在继承的情况下,子类可以覆盖父类的实例方法,但这不适用于组合的情况。
So this is the end of the article and I hope you have understood the concept of Inheritance and Composition in Java. If you find this article useful then share it with your friends. Follow me for similar articles.
所以这是本文的结尾,希望您理解Java中的继承和组合的概念。 如果您发现本文有用,请与您的朋友分享。 跟我来类似的文章。
Read my other helpful articles.
阅读其他有用的文章。
java中的组合与继承