java 时间 pt
For software engineers that are aiming to become full-stack or backend developers, it is best to know languages that are Object Oriented such as Ruby, JavaScript, Python, Go, Elixir, and Java. Of course there are many more object oriented programming, but the main focus today will be Java. I wanted to learn it because, I had the need to learn a different backend language and I wanted to see if I can create a few fun projects with them! Those projects will come later, but for this reading I want to talk about Java basics.
对于打算成为全栈或后端开发人员的软件工程师,最好了解面向对象的语言,例如Ruby,JavaScript,Python,Go,Elixir和Java。 当然,还有更多面向对象的编程,但是今天的主要焦点将是Java。 我想学习它,因为我需要学习另一种后端语言,并且想知道是否可以与他们一起创建一些有趣的项目! 这些项目将在以后发布,但是对于本阅读,我想谈谈Java基础。
你需要知道的事情 (Things you need to know)
For an upcoming technical interview, it is best to know some of Java’s vocabulary. You should understand these basics words at least: abstraction, encapsulation, polymorphism, and inheritance. JavaScript also has these vocabularies, but they may contain different meanings.
对于即将进行的技术面试,最好了解Java的一些词汇。 您至少应该了解以下基本词汇:抽象,封装,多态和继承。 JavaScript也具有这些词汇,但是它们可能包含不同的含义。
Abstraction: Is knowing what the outcome is, but you don’t know how the process works. Think of putting a flat piece of paper into one side of the box and the box produces an origami at the other side. I don’t know how it folds the paper, but I do know that the flat piece of paper comes out as an origami.
抽象:知道结果是什么,但是您不知道该过程如何工作。 考虑将一张扁平的纸放入盒子的一侧,盒子的另一侧产生折纸。 我不知道它是如何折叠纸张的,但我确实知道平纸可以作为折纸出现。
Encapsulation: Is hiding a variable from other classes and methods. In Java we use the key reserved word “private” to prevent the variable from leaking out.
封装:正在向其他类和方法隐藏变量。 在Java中,我们使用关键字保留字“ private”来防止变量泄漏。
Polymorphism: In a generic sense, it is the ability to take on many forms. So a reference type in Java can change it’s form according to the user.
多态性:从一般意义上讲,它是具有多种形式的能力。 因此,Java中的引用类型可以根据用户更改其形式。
Inheritance: a subclass inheriting properties/values from the main class. In Java and every other object oriented programming language, it allows an object to take a similar property to that of it’s parents.
继承:从主类继承属性/值的子类。 在Java和其他所有面向对象的编程语言中,它允许对象采用与其父对象相似的属性。
资料类型 (Data Types)
Java has 8 primitive data types that hold a specific amount of space in memory.
Java有8种原始数据类型,它们在内存中保留特定数量的空间。

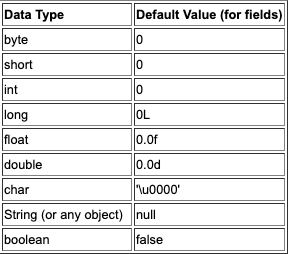
In order to assign a value to a variable, you are required to assign a data type first, name the variable second, and give the value third. For example, I want to create a variable age. In order to write that in Java, you must declare the variable as an int then the name of our variable will be age and the value will be 24.
为了给变量赋值,您需要首先赋值数据类型,然后给变量命名,然后给值赋第三。 例如,我要创建一个可变年龄。 为了用Java编写代码,必须将变量声明为int,然后变量的名称将为age,值将为24。
int age = 24; (like this)
年龄= 24; (像这样)
You are also allowed to assign this variable to another variable. int herAge = age;
您也可以将此变量分配给另一个变量。 int herAge =年龄;
When you print out the variable herAge, the terminal will show 24. Quick thing to note, primitive types are copied by their value and these values are all independent from each other. So if I were to change the value of herAge to 48, that would also be valid.
当您打印出变量herAge时,终端将显示24。值得注意的是,基本类型按其值复制,并且这些值彼此独立。 因此,如果我将herAge的值更改为48,那也是有效的。
弦乐 (Strings)
In Java, strings are considered objects and they are immutable because this object is backed internally by a char array (sequence of characters). You are not allowed to mutate the size of an array, thus a string cannot be mutated. There are two ways that we can create a string which will be shown on Example 1.
在Java中,字符串被视为对象,并且它们是不可变的,因为此对象在内部由char数组(字符序列)支持。 您不允许更改数组的大小,因此不能更改字符串。 创建字符串的方法有两种,如示例1所示。


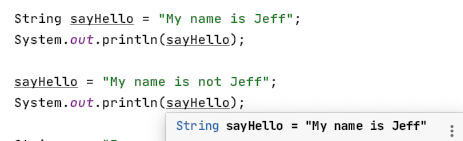
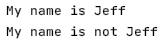
We are declaring the object type (String), then we are naming the object (a), and finally we are creating a value to a.
我们先声明对象类型(String),然后再命名对象(a),最后为a创建一个值。
The other way to declare a string is to specify the object type and name then use the keyword new for the value (were allocating memory for this object).
声明字符串的另一种方法是指定对象类型和名称,然后使用关键字new作为值(正在为此对象分配内存)。
I did mention before that we cannot mutate the string, but we can do it locally. On example 2, I have a variable called sayHello which points to “My name is Jeff”, then I change the value to “My name is not Jeff.” When I print out the variables it shows that I have changed the string object sayHello (locally). Java uses pass-by-value, not pass-by-reference. When I assign a new value to sayHello in my variable it only modifies the local sayHello, not the original sayHello in the calling code.
我之前曾提到过,我们无法对字符串进行突变,但可以在本地进行。 在示例2中,我有一个名为sayHello的变量,它指向“我的名字叫Jeff”,然后将值更改为“我的名字不是Jeff”。 当我打印出变量时,它表明我已经更改了字符串对象sayHello(本地)。 Java使用按值传递,而不是按引用传递。 当我在变量中为sayHello分配新值时,它仅修改本地sayHello,而不修改调用代码中的原始sayHello。
参考类型 (Reference Types)
In Java, reference type is a data type that’s based on a class rather than on one of the primitive types. The class can be a class that is provided as part of the Java API class library or a class that you write yourself. When you create an object from a class, Java allocates the amount of memory the object requires to store the object. Then, if you assign the object to a variable, the variable is actually assigned a reference to the object, not the object itself. This reference is the address of the memory location where the object is stored.
在Java中,引用类型是基于类而不是原始类型之一的数据类型。 该类可以是作为Java API类库的一部分提供的类,也可以是您自己编写的类。 从类创建对象时,Java会分配该对象存储该对象所需的内存量。 然后,如果将对象分配给变量,则实际上为变量分配了对对象的引用,而不是对象本身。 该引用是存储对象的存储位置的地址。
To declare a variable using a reference type, you simply list the class name as the data type. For example, the following statement defines a variable that can reference objects created from a class called Date.
要使用引用类型声明变量,只需将类名称列出为数据类型。 例如,以下语句定义了一个变量,该变量可以引用从名为Date的类创建的对象。
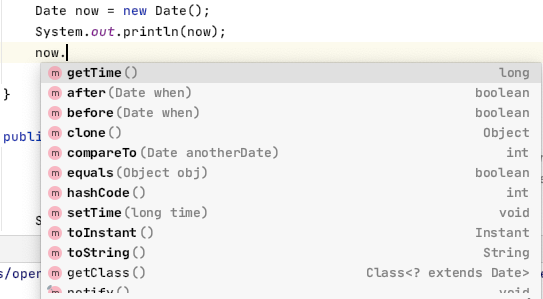

Date is part of the Java API class libraries that allows us to have access to some methods for this class of Date. Our variable now is an object from the Date class and we now have reference to the object through the variable.
Date是Java API类库的一部分,它使我们能够访问此Date类的某些方法。 现在,我们的变量是Date类中的对象,并且现在可以通过变量引用该对象。
最终最终确定 (Final, Finally, Finalize)
These three words in the english dictionary have a similar term to one another, but in Java they all have a different meaning. It is also great to know about these three terms for a technical interview. Final is used to apply restrictions on class, method and variable (keyword). Final class can’t be inherited, final method can’t be overridden and final variable value can’t be changed. Finally is used to place important code, it will be executed whether exception is handled or not (block). Last but not least, finalize is used to perform clean up processing just before object is garbage collected (method).
英语词典中的这三个词彼此具有相似的术语,但是在Java中,它们都有不同的含义。 知道这三个术语以进行技术面试也很棒。 Final用于对类,方法和变量(关键字)施加限制。 不能继承final类,不能覆盖final方法,并且不能更改final变量值。 最后用于放置重要代码,无论是否处理异常(块),都会执行该代码。 最后但并非最不重要的一点是, finalize用于在对象被垃圾回收(方法)之前执行清理处理。
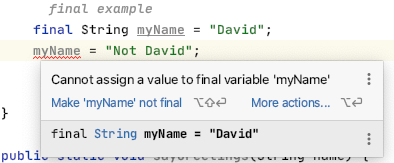
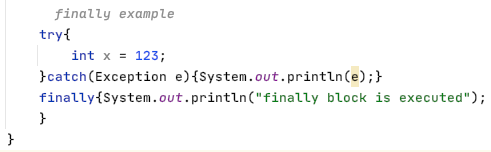
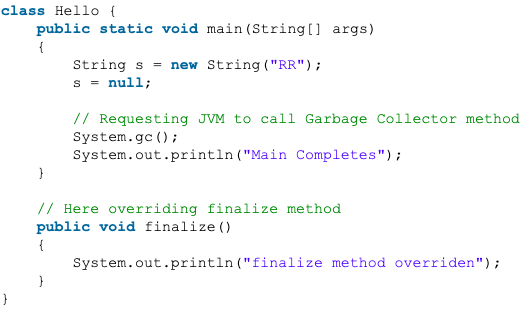
There is so much we can do with Java and I will be discussing about the other types of functionality Java provides. I hope this clarifies some of the basic with you!
Java可以做很多事情,我将讨论Java提供的其他功能。 我希望这能使您了解一些基本知识!
翻译自: https://medium.com/@littlesadtea/interacting-with-java-pt-1-308dd246b49c
java 时间 pt