程序员往基础和底层走
Learning a programming language takes research. Each one is merely a tool used to perform a specific task and different languages offer benefits over others which makes it important to know exactly what you want to achieve before choosing an option.
大号赚一门编程语言需要研究。 每个选项仅是用于执行特定任务的工具,不同的语言比其他语言具有更多的好处,因此在选择选项之前准确了解要实现的目标非常重要。
Artificial Intelligence is recommended with Python because of built-in libraries like NumPy and Pandas that offer functionality for A.I. development out of the box. If you’re working with Embedded Systems, more than likely you would use a language like C due to its power and low-level access.
一个rtificial智能推荐使用Python,因为内置的像NumPy这样和熊猫库,用于AI开发提供实用的开箱即用。 如果您使用的是嵌入式系统,则由于C的强大功能和低级访问权限,您很有可能会使用C之类的语言。
Now don’t get me wrong — depending on the community — you can accomplish almost anything nowadays, no matter the language chosen. There are a number of package managers composed of libraries — created by maintainers and community members — that will get the job done but to fully optimize your project, selecting the right language makes for a stronger architecture.
ñOW不要误会我的意思-这取决于社区-你现在可以完成几乎所有的东西,不管选择的语言。 由维护人员和社区成员创建的由库组成的许多程序包管理器可以完成工作,但要完全优化您的项目,选择正确的语言可以使体系结构更强大。
G o is a powerful language. It was built on the premise of efficiency and ease of programming. The syntax can be written loosely or precise as you intend. The language has differentiated itself from others with features that highlight concurrency but no matter your purpose, executes at speeds more performant than any syntax to come before it. It might not be a language that I would recommend to a beginner but I was able to grasp the concepts after learning JavaScript — although, you may want to check out TypeScript before making the leap. Nonetheless, let’s explore the basic fundamentals of Go.
G o是一门强大的语言。 它是在高效且易于编程的前提下构建的。 可以根据需要随意或精确地编写语法。 该语言与众不同,其特色在于突出了并发性,但无论您的目的如何,其执行速度都比其之前的任何语法都要高。 它可能不是我会推荐给初学者的语言,但是我在学习JavaScript之后能够掌握其中的概念,尽管您可能想在飞跃之前先检查一下TypeScript。 尽管如此,让我们探索Go的基本原理。
种类 (Types)
Types dominate the Go programming language. They specify the underlying data type of a variable. There are four different classifications of a type:
类型占主导地位的Go编程语言。 它们指定变量的基础数据类型。 一个类型有四种不同的分类:
Basic types
基本类型
- Numbers号码
- Strings弦乐
- Boolean布尔型
2. Aggregate/Composite types
2.集合/复合类型
- Arrays — aggregate: groups values of same type数组-聚合:将相同类型的值分组
- Structs — composite: groups values of different types结构—复合:将不同类型的值分组
3. Reference types
3.参考类型
- Pointers指针
- Slices切片
- Maps地图
- Functions功能
- Channels* ( not covered )渠道*(未涵盖)
4. Interface type
4.接口类型
基本类型(Basic Types)
号码(Numbers)
Number types are separated into 3 sub-categories: Integers, Floating-Points, and Complex Numbers.
数字类型分为3个子类别:整数,浮点数和复数。
弦乐 (Strings)
String types represent a sequence of Unicode characters.
字符串类型表示Unicode字符序列。
布尔型 (Boolean)
Boolean types are represented by either a true or false value.
布尔类型由true或false值表示。
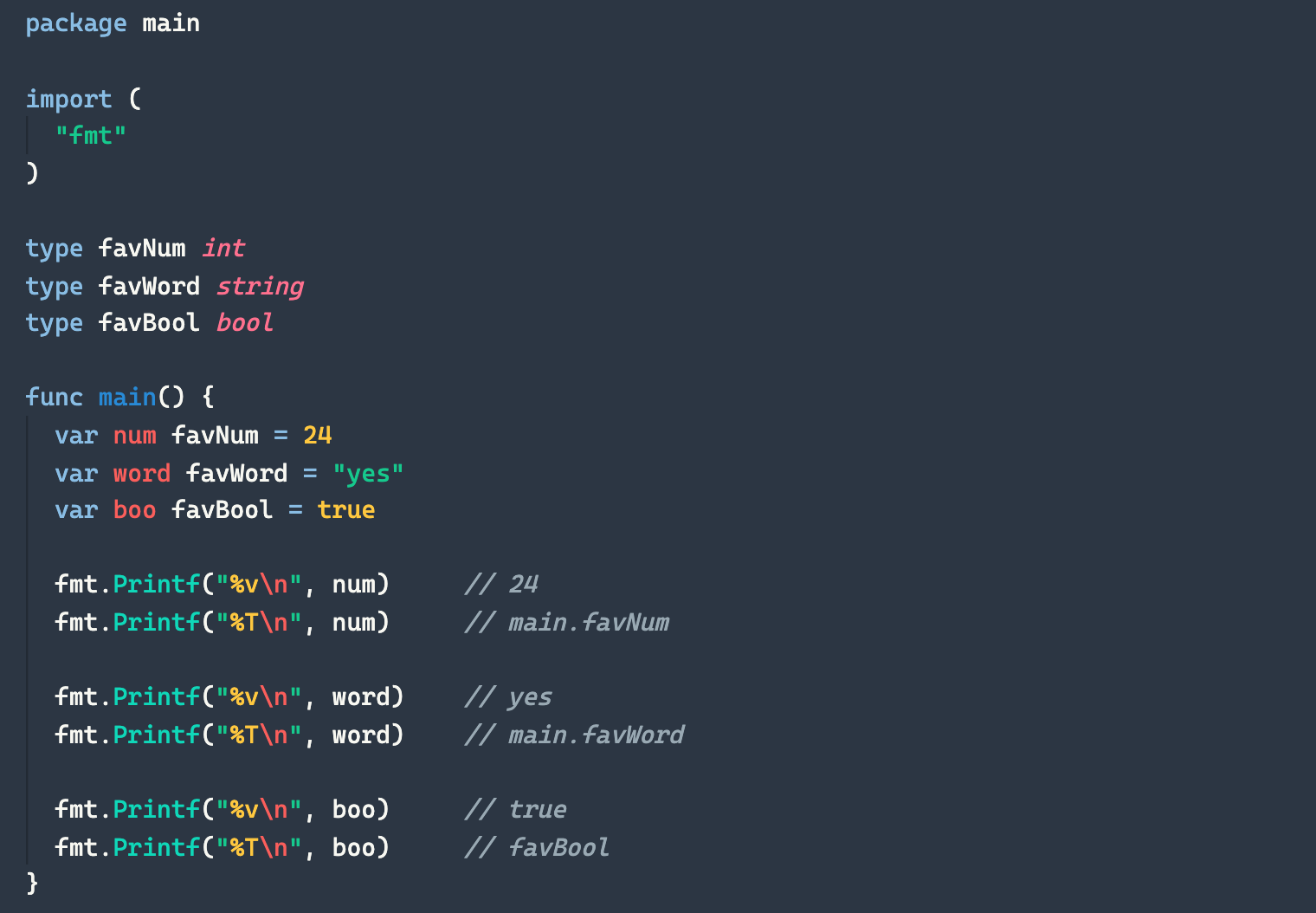
变数 (Variables)
There are three primary ways of declaring variables in Go. The first way is by using the var keyword before its identifier followed by the variable type. You can then set the variable to a value of your choosing. If a value is not provided, the variable will be set to its zero value.
Go中有三种声明变量的主要方法。 第一种方法是在变量标识符之前使用var关键字,后跟变量类型。 然后可以将变量设置为您选择的值。 如果未提供值,则变量将设置为零值。
The second way to declare a variable is by way of the shorthand syntax — which can only be used inside of a function — and uses type inference like that of a dynamically-typed programming language. Shorthand variables are declared with the :=
operator — which is said to resemble that of a gopher — and requires the compiler to interpret its type by the value set to it.
声明变量的第二种方法是通过简写语法-只能在函数内部使用-并使用类型推论,就像动态类型的编程语言一样。 速记变量是使用:=
运算符声明的-据说类似于地鼠-并要求编译器通过为其设置的值来解释其类型。
The third way is by using the keyword const
which will set an immutable value that cannot be changed.
第三种方式是使用关键字const
,它将设置一个不可更改的不可变值。
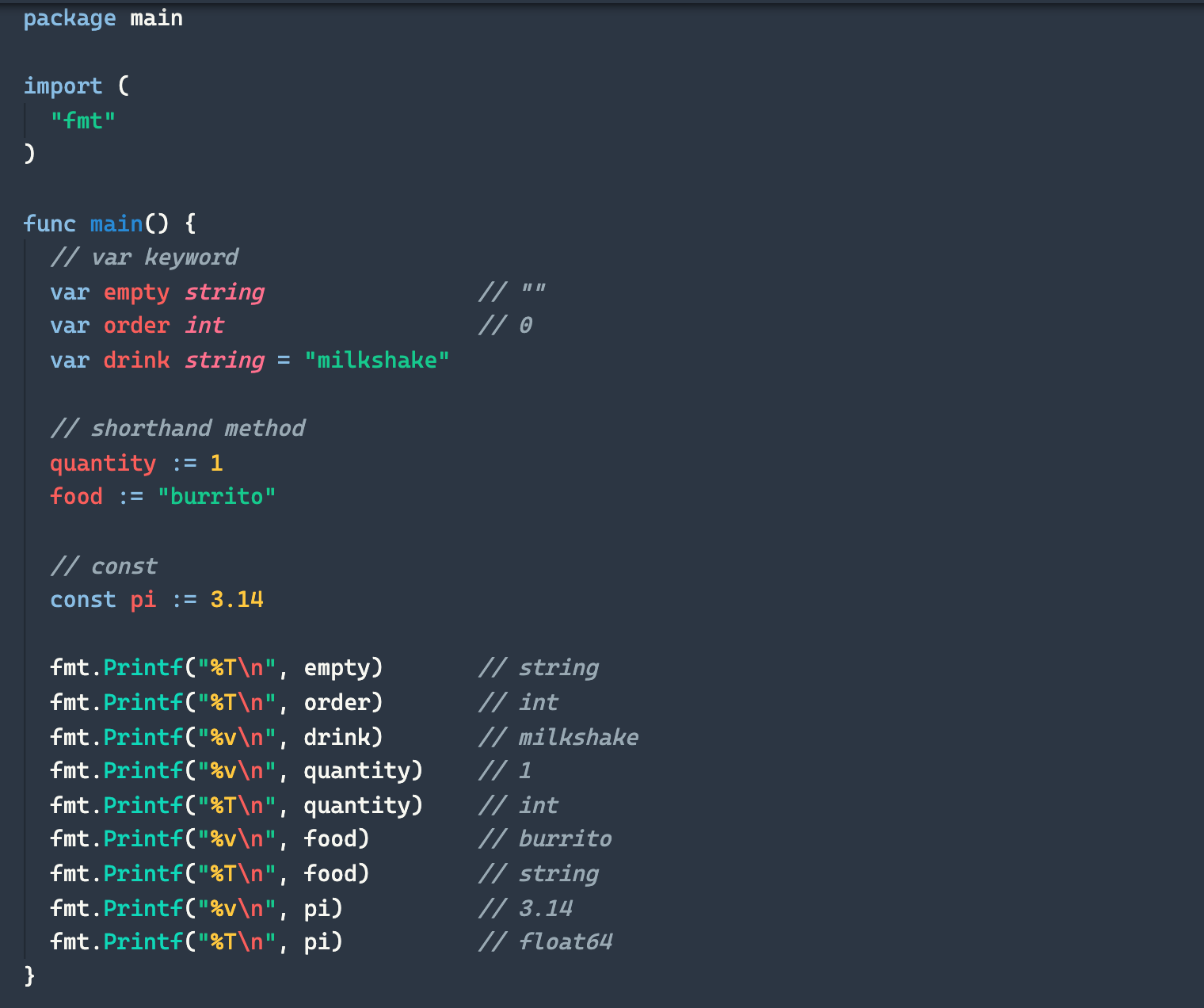
功能 (Functions)
Each program written in Go has — at minimum — one function with an identifier of main()
. This is the entry point of a Go application. A function declaration provides the compiler information about the function including its identifier ( name ), return type and parameters. Functions in Go can also be referred to as methods, subroutines, or procedures.
用Go编写的每个程序至少都有一个带有main()
标识符的函数。 这是Go应用程序的入口点。 函数声明提供了有关函数的编译器信息,包括其标识符(名称),返回类型和参数。 Go中的函数也可以称为方法,子例程或过程。
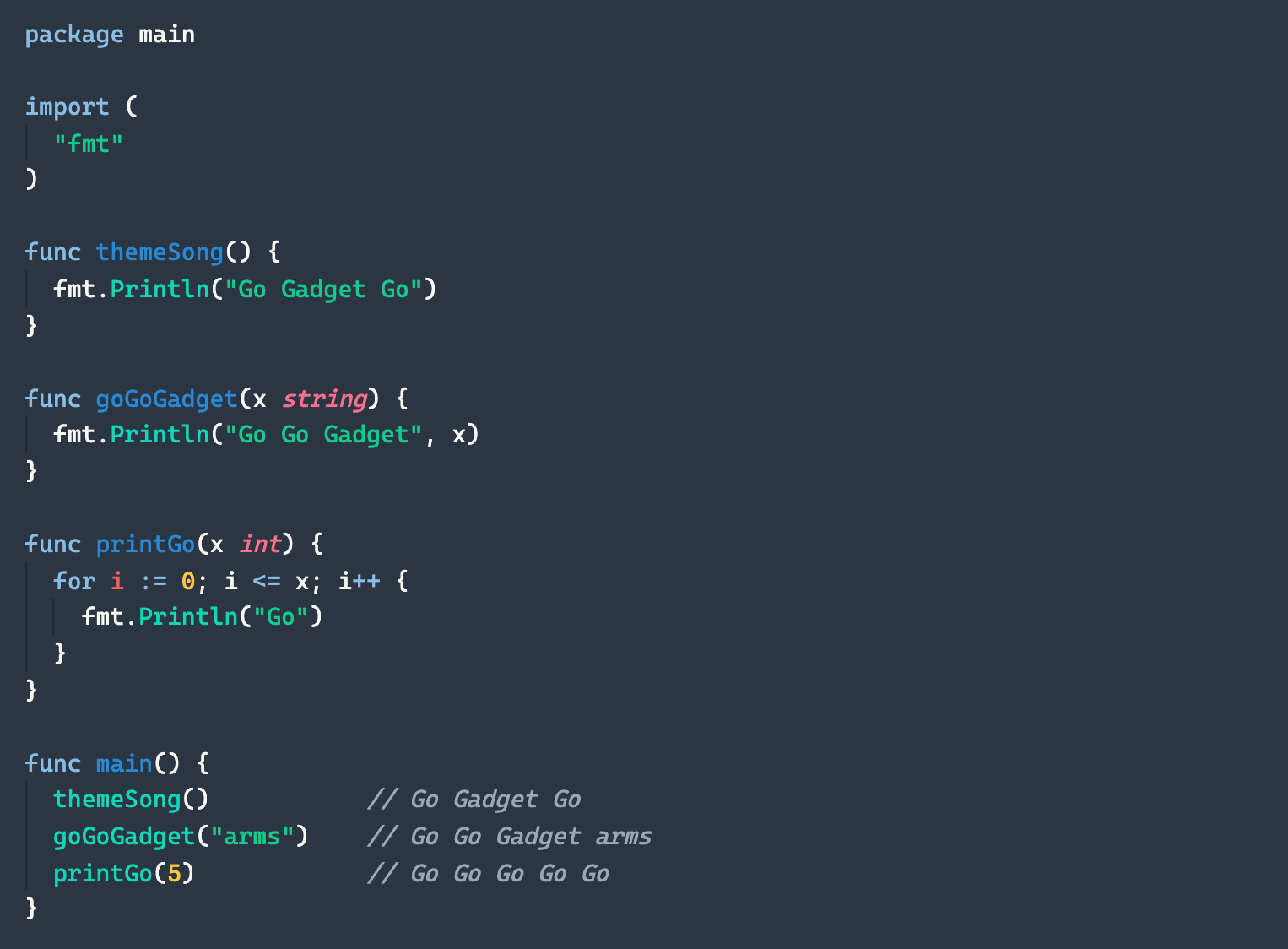
You can also return multiple values with functions in Go.
您还可以使用Go中的函数返回多个值。
数组 (Arrays)
Arrays in Go are different from that of dynamically types languages such as JavaScript or Python. In Go, an array is a collection of a fixed-size and its elements all share the same type. An array in Go also consists of neighboring memory locations with the lowest address corresponding to the first element. You declare an array similarly to how you would a variable in Go and subsequently, access an element of an array in the standard, programmatic form:
Go中的数组与动态类型的语言(如JavaScript或Python)不同。 在Go中,数组是固定大小的集合,并且其元素都共享相同的类型。 Go中的数组还包括具有最低地址的相邻内存位置,该地址对应于第一个元素。 声明数组的方式与在Go中定义变量的方式类似,随后以标准的编程形式访问数组的元素:
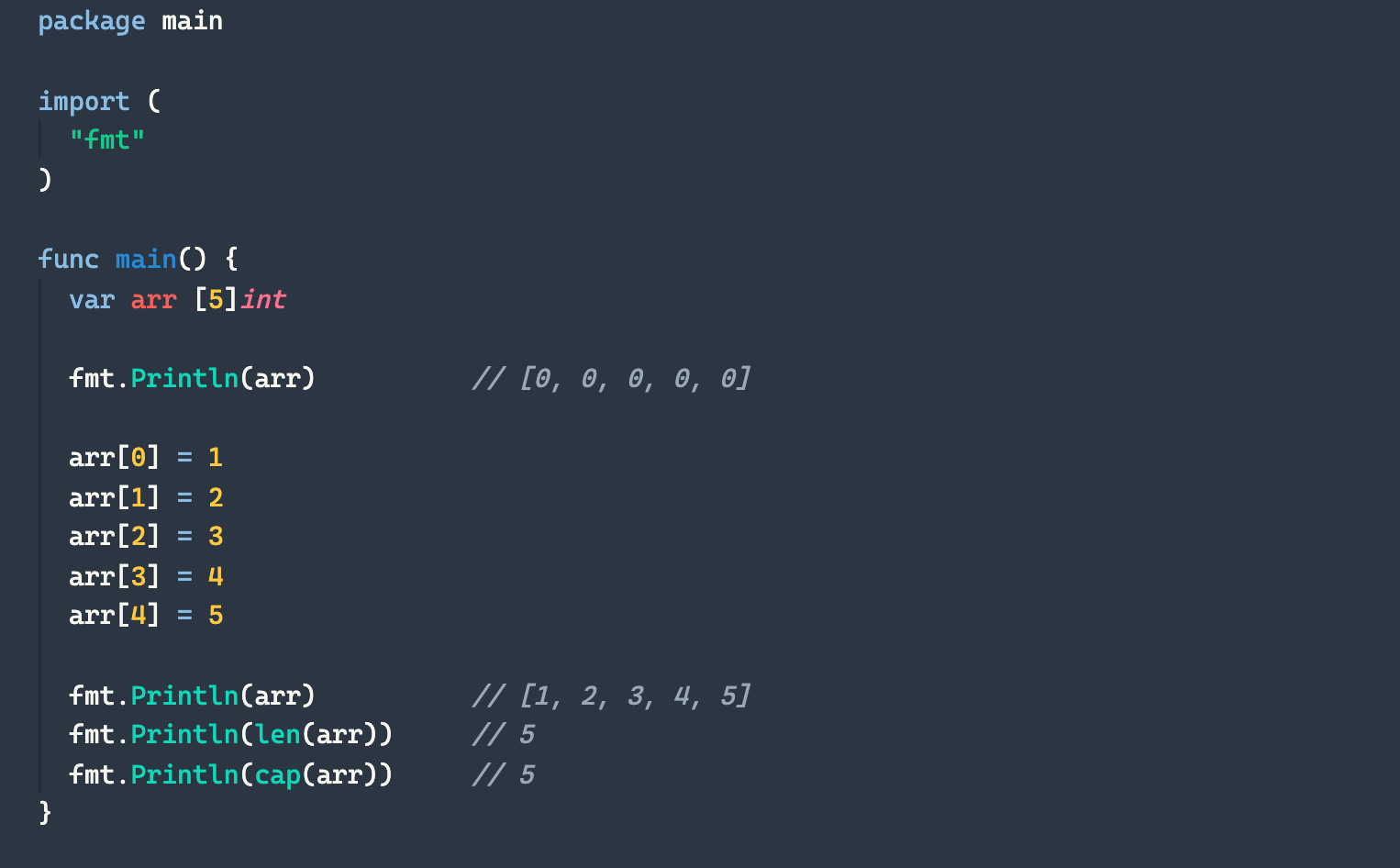
An array is also the underlying structure of a slice.
数组也是切片的基础结构。
切片 (Slices)
A slice is an abstraction that is built on top of an array in Go. It allows for an array to increment in size and can access utility functions that are otherwise called on an array like len()
— to output the length of the slice — and cap()
which outputs its capacity.
切片是一种构建在Go数组顶部的抽象。 它允许数组增加大小,并可以访问在len()
等数组上调用的实用程序函数,以输出切片的长度,而cap()
输出其容量。
There are two ways to declare a slice. The first sets an identifier to a pair of empty square brackets followed by the type of slice — int, string, bool. To initialize a slice with values, add a pair of curly braces after the slice type and input whatever you wish. Slices that are declared without inputs are initialized to nil by default.
声明切片有两种方法。 第一个将标识符设置为一对空方括号,其后是slice的类型— int , string , bool 。 要使用值初始化切片,请在切片类型后添加一对大括号,然后输入所需的内容。 默认情况下,声明为不带输入的切片将初始化为nil。
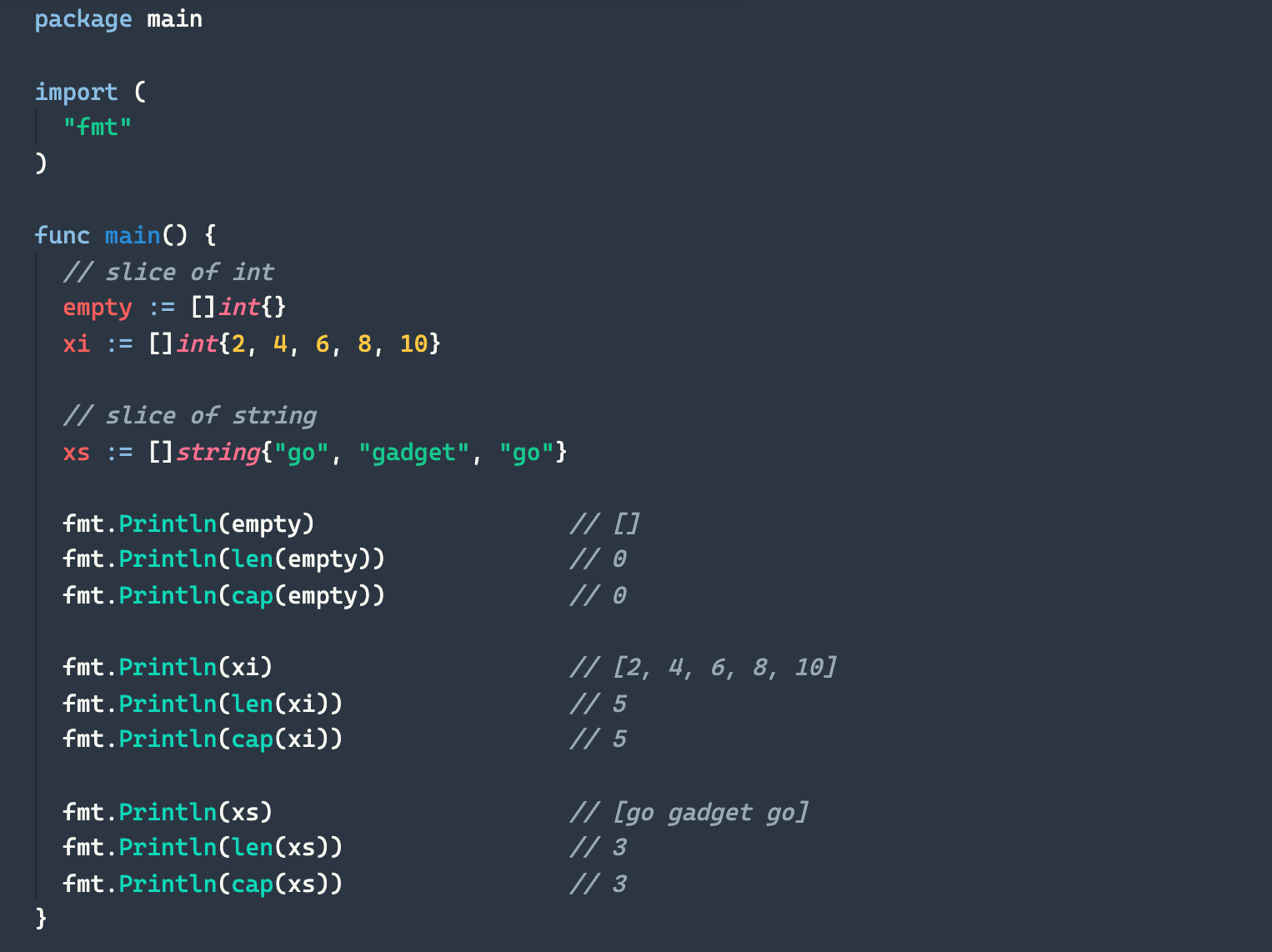
You can add items to a slice with the built-in append( ) method.
您可以使用内置的append()方法将项目添加到切片中。
结构 (Structs)
A struct in Go is a data structure that stores items of different types, similar to objects in OOP languages. They can be used to create models which help structure real world objects. To declare a struct, use the type keyword followed by its identifier, a struct statement and a pair of curly braces.
Go中的结构是一种数据结构,用于存储不同类型的项目,类似于OOP语言中的对象。 它们可用于创建有助于构造现实世界对象的模型。 要声明一个结构,请使用type关键字,后跟其标识符,一个struct语句和一对花括号。
To access items from a struct, use the member access operator, which is to Go what dot notation is to JavaScript.
要从结构访问项目,请使用成员访问运算符,该运算符是JavaScript的点符号。
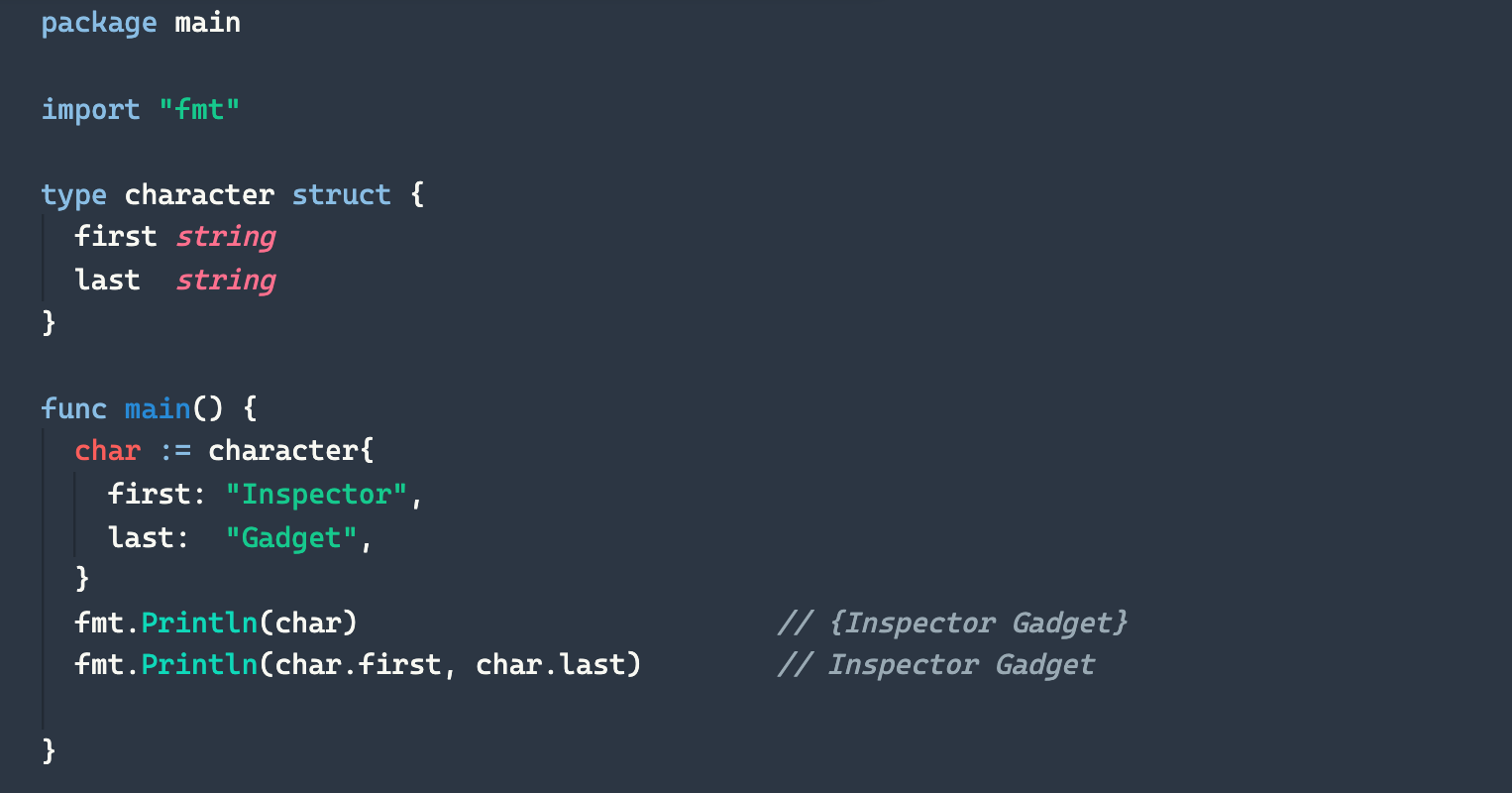
地图 (Maps)
A map is a data type which relates unique keys to values. You can declare a map one of two ways. The first way is by using the var keyword and setting the identifier to the keyword map followed by the type of key inside of a pair of square brackets; outside of the brackets, specify which type of value corresponds to the key.
映射是将唯一键与值相关联的数据类型。 您可以使用以下两种方法之一声明地图。 第一种方法是使用var关键字,并将标识符设置为关键字映射,然后在一对方括号内设置键的类型。 在方括号之外,指定与键对应的值类型。
You can also utilize the make method to declare a map.
您也可以利用make方法来声明地图。
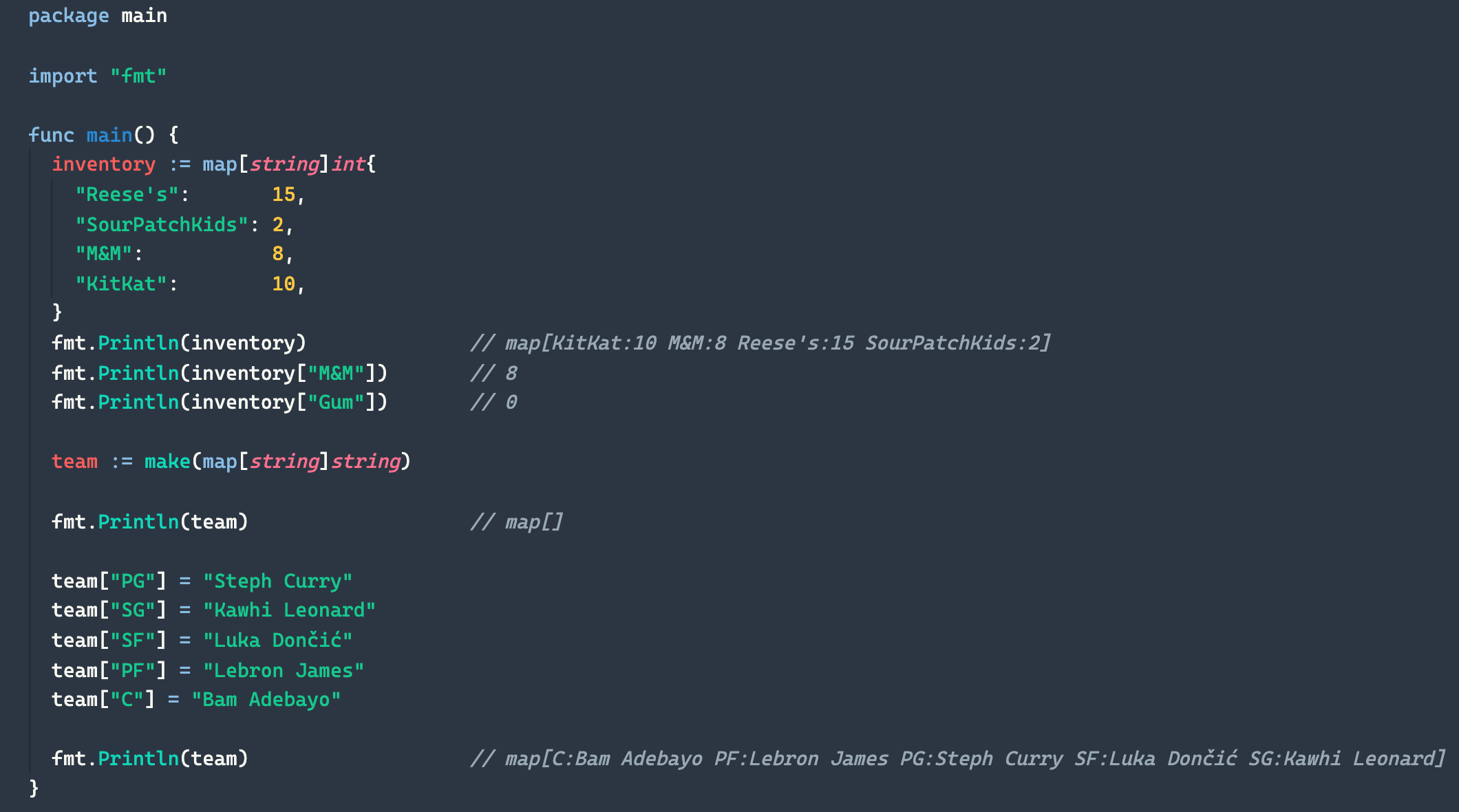
指针 (Pointers)
A variable is nothing more than an address in memory that stores data. A pointer is a variable whose value points to another variable’s location in space. It is defined using a var keyword followed by an identifier set to a data type that’s preceded by an asterisk. Pointers are crucial in performing tasks such as call by reference.
变量不过是存储数据的内存中的地址。 指针是一个变量,其值指向另一个变量在空间中的位置。 它是使用var关键字定义的,后跟一个标识符,该标识符设置为带有星号的数据类型。 指针对于执行诸如引用调用之类的任务至关重要。
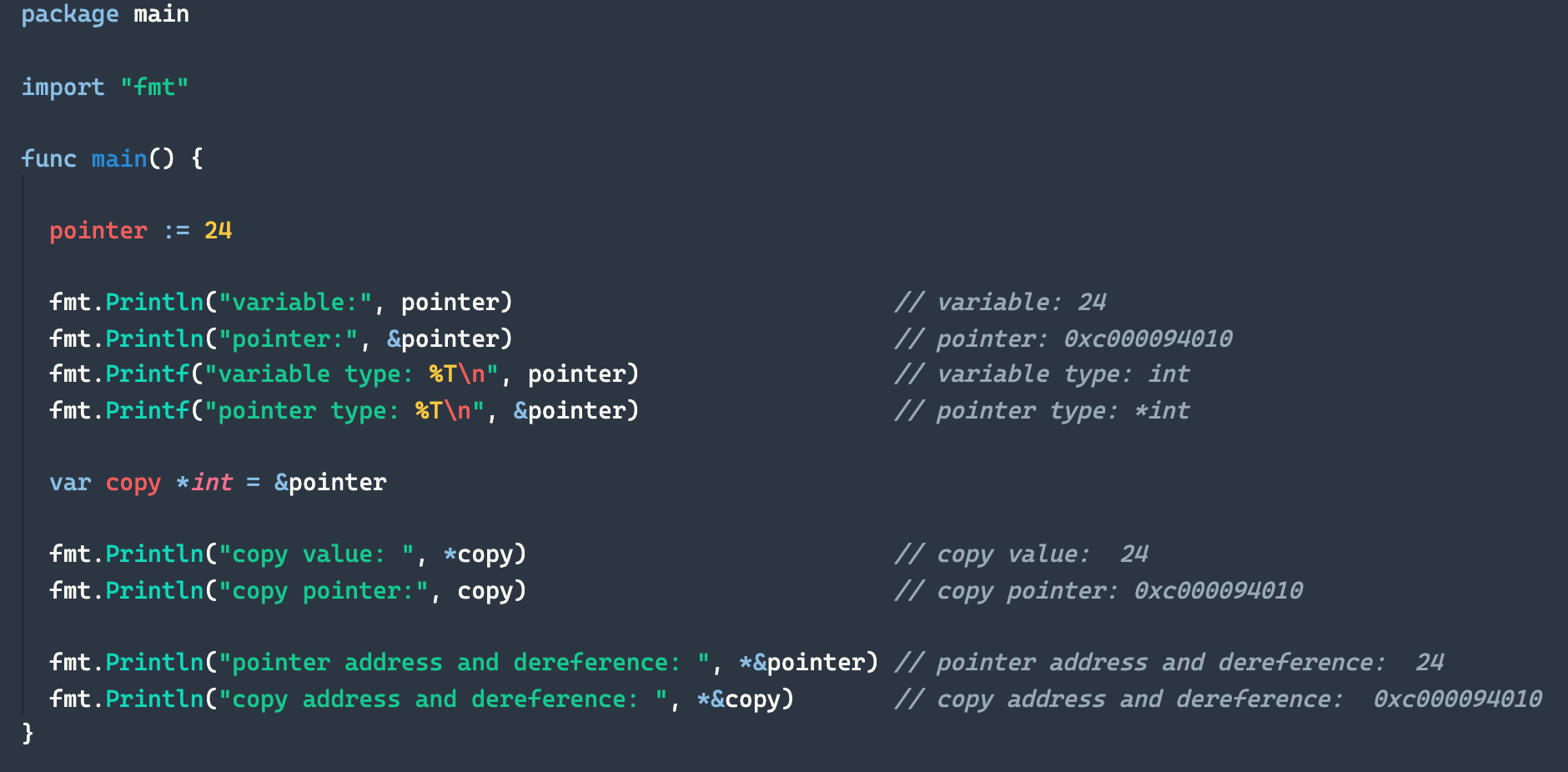
介面 (Interfaces)
An interface is a data type that consists of a list of method signatures that allow for structs of the same type to access whatever functionality is explicitly defined.
接口是一种数据类型,由一系列方法签名组成,这些方法签名允许相同类型的结构访问任何显式定义的功能。
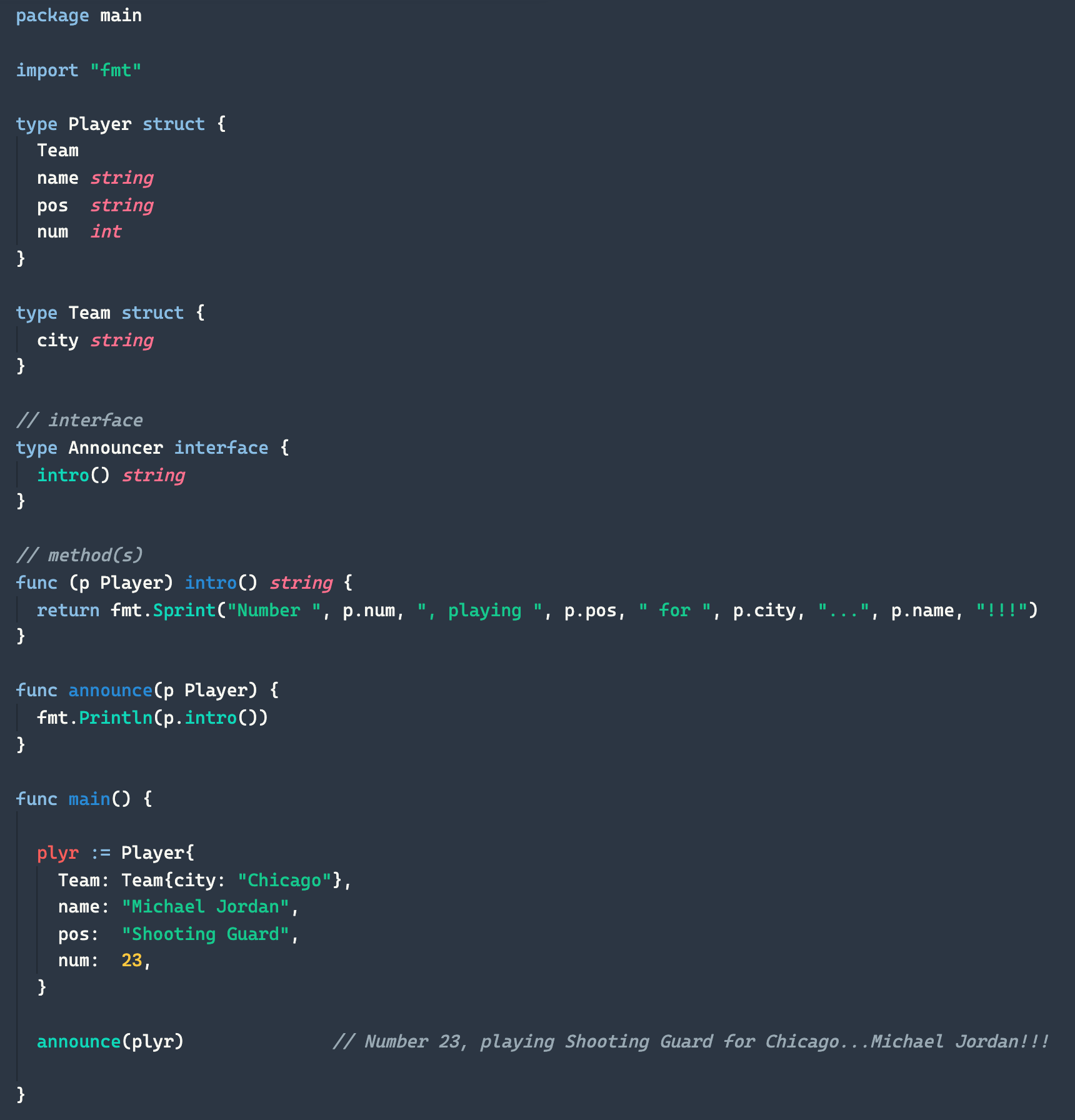
结论 (Conclusion)
The above was meant to serve as an introduction of the syntax and to familiarize you with the data structures of the Go programming language. There are a number of resources available to learn Go, some of which I have outlined in a previous overview of the language. Once you’ve taken the time to explore the core concepts, I implore you to venture out into the Standard Library to truly understand what Go has to offer.
以上内容旨在作为语法的介绍,并使您熟悉Go编程语言的数据结构。 有许多可供学习围棋的资源,其中一些我在前面所概述的概述语言。 一旦您花时间探索了核心概念,我恳请您冒险进入标准库,以真正了解Go所提供的功能。
What are you waiting for? Go!
你在等什么? 走!
翻译自: https://medium.com/@ckakos/go-fundamentals-1c173c2b5fa7
程序员往基础和底层走