aws terraform
We are going to deploy a WordPress application on top of Kubernetes cluster using Kubernetes service of Google Cloud Platform (GCP) along with Load Balancer and for database, we will be creating a MySQL db instance using RDS of Amazon Web Services (AWS). All of these using Terraform.
我们将使用Google Cloud Platform(GCP)的Kubernetes服务以及负载均衡器在Kubernetes集群之上部署WordPress应用程序,并针对数据库,我们将使用Amazon Web Services(AWS)的RDS创建MySQL数据库实例。 所有这些都使用Terraform。
So, these are the steps -
因此,这些步骤-
- Creating a VPC, subnet and firewall rule inside a project on GCP. 在GCP上的项目内创建VPC,子网和防火墙规则。
- Create a Kubernetes cluster, deploy WordPress on top of it with LoadBalancer service. 创建一个Kubernetes集群,并使用LoadBalancer服务在其之上部署WordPress。
- Using Relational Database Service (RDS) of AWS and creating a MySQL db instance for connecting it to the WordPress site. 使用AWS的关系数据库服务(RDS)并创建一个MySQL数据库实例以将其连接到WordPress站点。
Every resource on GCP needs to be created inside a project, so first we should have a project. We can create or delete projects using the cloud shell as shown in the image. ( the project should be connected with billing account and also required APIs should be enabled)
GCP上的每个资源都需要在一个项目中创建,因此首先我们应该有一个项目。 如图所示,我们可以使用云外壳创建或删除项目。 (该项目应与结算帐户关联,并且还应启用必需的API)
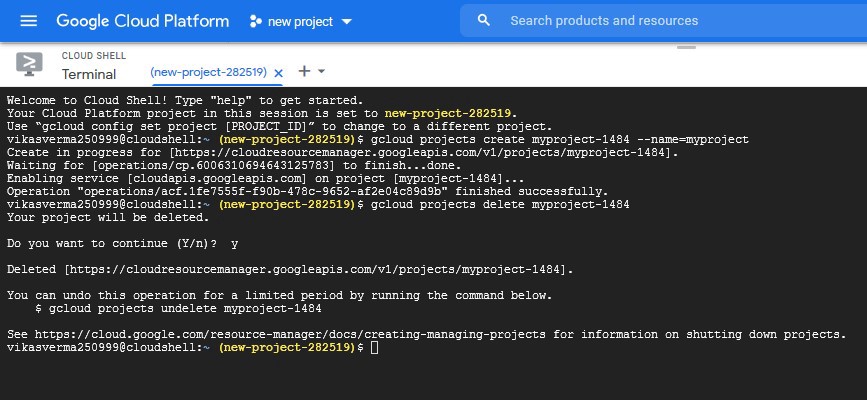
For connecting Terraform with your GCP account, you can create a key from your service account and download that json format key.
为了将Terraform与您的GCP帐户连接,您可以从服务帐户中创建一个密钥,然后下载该json格式密钥。
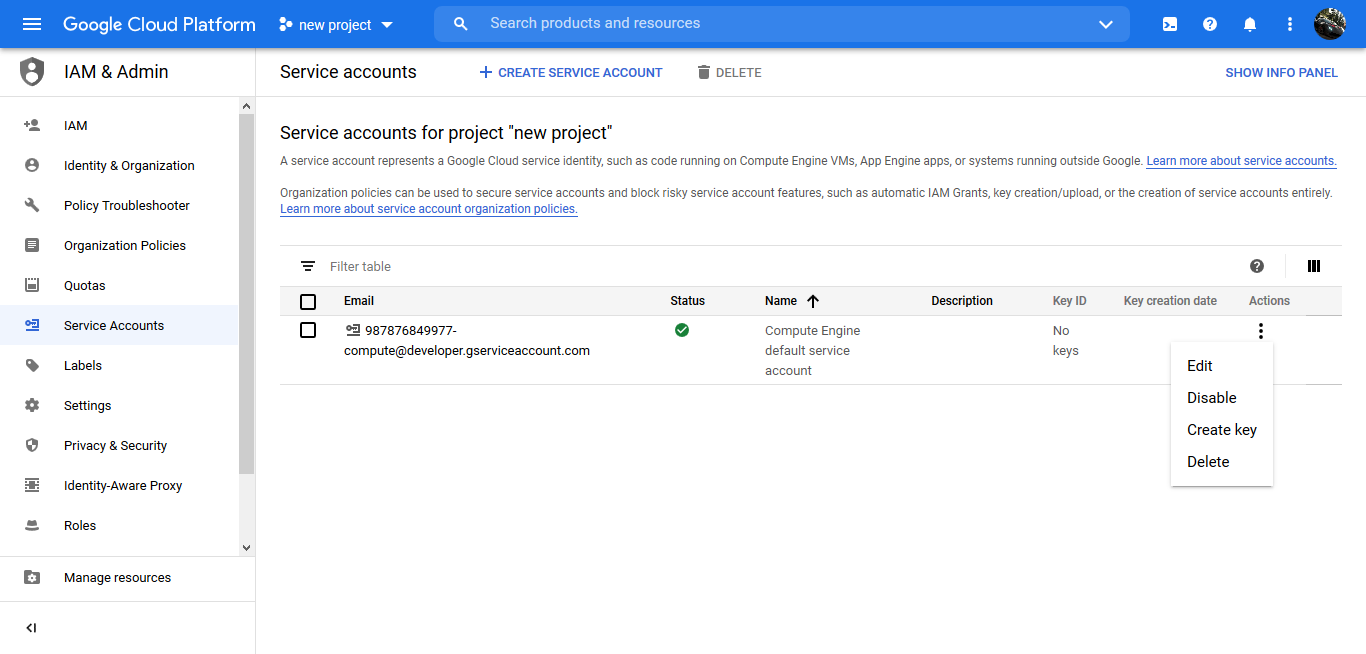
Now, let’s start writing Terraform code.
现在,让我们开始编写Terraform代码。
First we need the provider which is google, give the credential (the downloaded key of the service account) and also write the project in which we are going to create the resources.
首先,我们需要提供者google,提供凭据(服务帐户的下载密钥),并编写要在其中创建资源的项目。
provider "google" {
credentials = file("new-project.json")
project = var.project
}
Creating a VPC and subnet,
创建一个VPC和子网,
resource "google_compute_network" "myvpc" {
name = var.vpc_gcp
auto_create_subnetworks = false
}
resource "google_compute_subnetwork" "network" {
name = var.lab
ip_cidr_range = "10.0.1.0/24"
region = var.gcp_region
network = google_compute_network.myvpc.id
}
Adding firewall rule to our created vpc,
将防火墙规则添加到我们创建的vpc中,
resource "google_compute_firewall" "rule" {
name = "myfirewall"
network = google_compute_network.myvpc.name
allow {
protocol = "icmp"
}
allow {
protocol = "tcp"
ports = ["80"]
}
}
Now creating a Kubernetes cluster in our vpc,
现在在我们的vpc中创建一个Kubernetes集群,
resource "google_container_cluster" "primary" {
name = "myk8scluster"
location = var.gcp_region
initial_node_count = 1
network = google_compute_network.myvpc.name
subnetwork = google_compute_subnetwork.network.name
node_config {
oauth_scopes = [
"https://www.googleapis.com/auth/logging.write",
"https://www.googleapis.com/auth/monitoring",
]
metadata = {
disable-legacy-endpoints = "true"
}
}
}
We are now using Kubernetes provider and connect with the created cluster, creating a deployment using WordPress docker image .
我们现在使用Kubernetes提供程序并连接到创建的集群,并使用WordPress docker image创建部署。
data "google_client_config" "provider" {}
provider "kubernetes" {
load_config_file = false
host = "https://${google_container_cluster.primary.endpoint}"
token = data.google_client_config.provider.access_token
cluster_ca_certificate = base64decode(
google_container_cluster.primary.master_auth[0].cluster_ca_certificate,
)
}
resource "kubernetes_deployment" "wp" {
metadata {
name = "wordpress"
labels = {
App = "frontend"
}
}
spec {
replicas = 1
selector {
match_labels = {
App = "frontend"
}
}
template {
metadata {
labels = {
App = "frontend"
}
}
spec {
container {
image = "wordpress"
name = "wordpress"
port {
container_port = 80
}
}
}
}
}
}
Using the LoadBalancer service of Kubernetes, it will create an external Load Balancer using the Load balancing service provided by GCP.
使用Kubernetes的LoadBalancer服务,它将使用GCP提供的负载平衡服务创建一个外部负载平衡器。
resource "kubernetes_service" "lb" {
metadata {
name = "wordress"
}
spec {
selector = {
App = "frontend"
}
port {
port = 80
target_port = 80
}
type = "LoadBalancer"
}
}
Now, let’s move to AWS, (you should have configured aws cli with your credentials of the profile you are using)
现在,让我们转到AWS,(您应该使用正在使用的配置文件的凭据配置aws cli)
provider "aws" {
region = var.aws_region
profile = var.profile
}
Creating a security group to allow inbound or ingress to port number 3306 (default port on which MySQL work),
创建一个安全组,以允许入站或入站到端口号3306(MySQL工作所在的默认端口),
resource "aws_security_group" "rds" {
name = "terraform_rds_security_group"
description = "Terraform example RDS MySQL server"
ingress {
from_port = 3306
to_port = 3306
protocol = "tcp"
cidr_blocks = ["0.0.0.0/0"]
}
# Allow all outbound traffic.
egress {
from_port = 0
to_port = 0
protocol = "-1"
cidr_blocks = ["0.0.0.0/0"]
}
tags = {
Name = "terraform-example-rds-security-group"
}
}
We are creating a MySQL db instance, publicly accessible so that WordPress can connect to it.
我们正在创建一个可公开访问MySQL数据库实例,以便WordPress可以连接到它。
resource "aws_db_instance" "default" {
allocated_storage = 20
storage_type = "gp2"
engine = "mysql"
engine_version = "5.7"
instance_class = "db.t2.micro"
name = var.name
username = var.username
password = var.password
parameter_group_name = "default.mysql5.7"
skip_final_snapshot = true
backup_retention_period = 0
apply_immediately = true
publicly_accessible = true
vpc_security_group_ids = [aws_security_group.rds.id]
}
This is just to print the output at the end with all the things required by us.
这只是在最后输出我们需要的所有内容。
output "lb_ip" {
value = kubernetes_service.lb.load_balancer_ingress.0.ip
}
output "dns" {
value = aws_db_instance.default.address
}
output "name" {
value = aws_db_instance.default.name
}
output "username" {
value = aws_db_instance.default.username
}
output "password" {
value = aws_db_instance.default.password
}
So, time to run the code…
因此,该运行代码了……
First we do terraform init to download all the providers used (google, terraform and aws)
首先,我们进行terraform init下载所有使用的提供程序(google,terraform和aws)
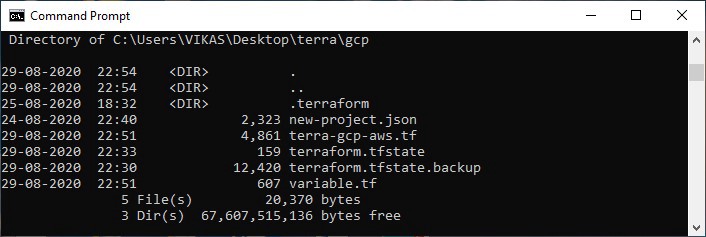
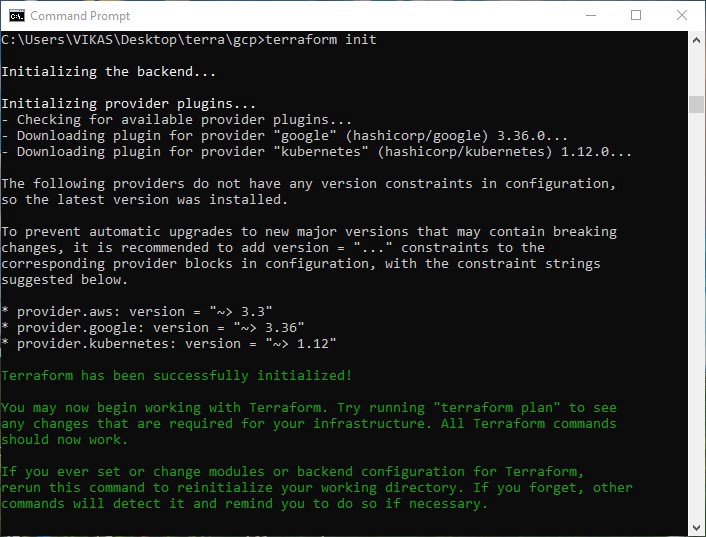
Now, terraform plan to see what resources will be created actually after apply
现在,terraform计划查看应用后将实际创建哪些资源
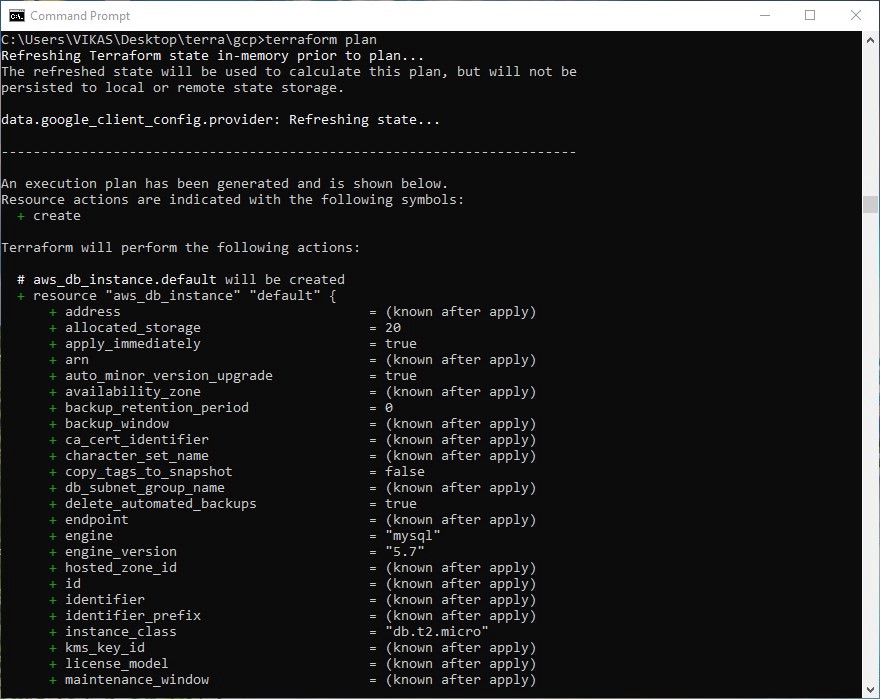
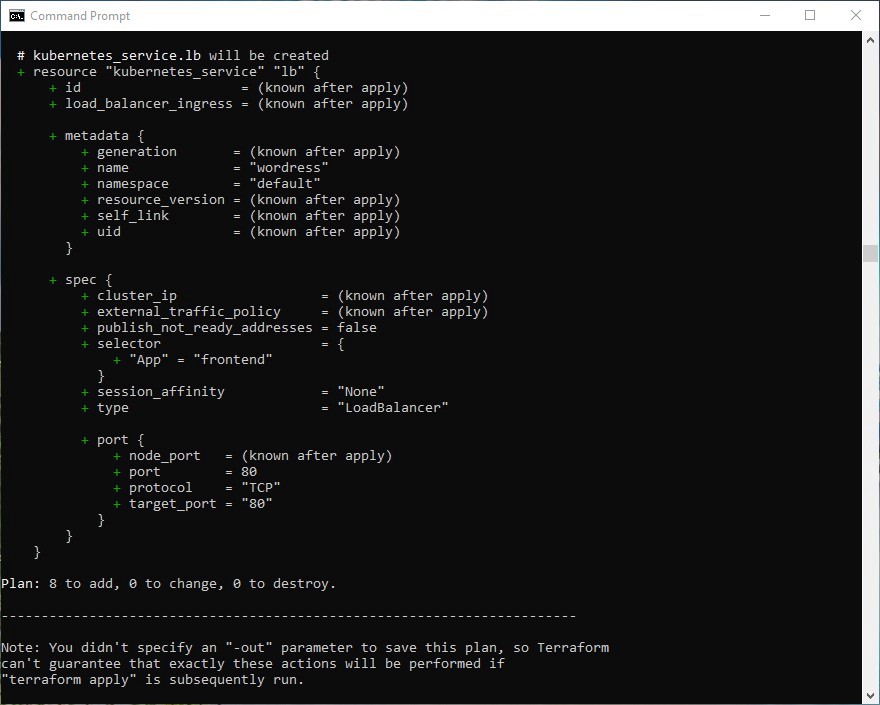
Finally, apply to create all the resources.
最后,申请创建所有资源。
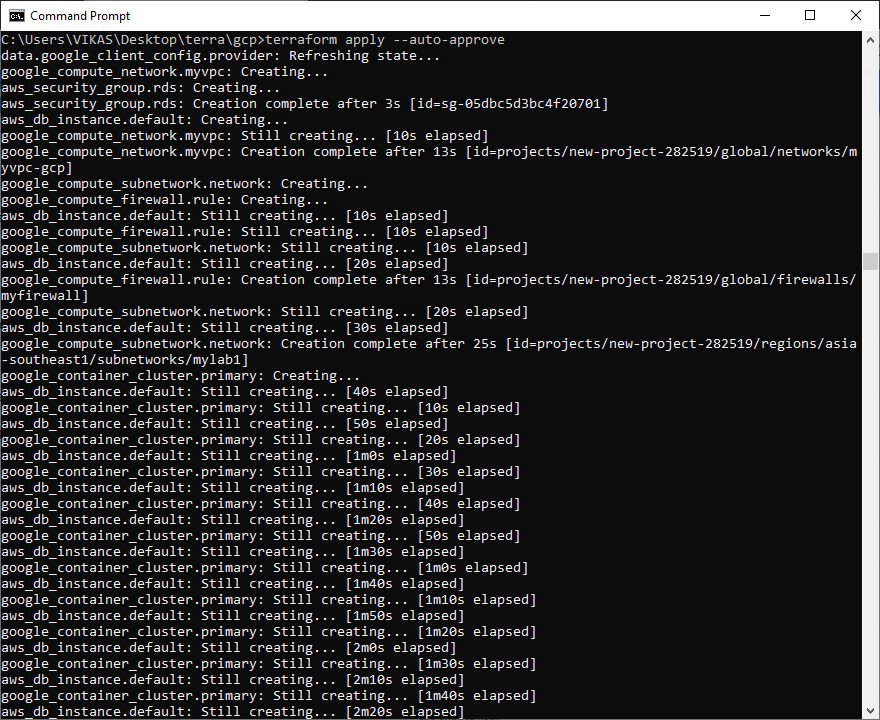
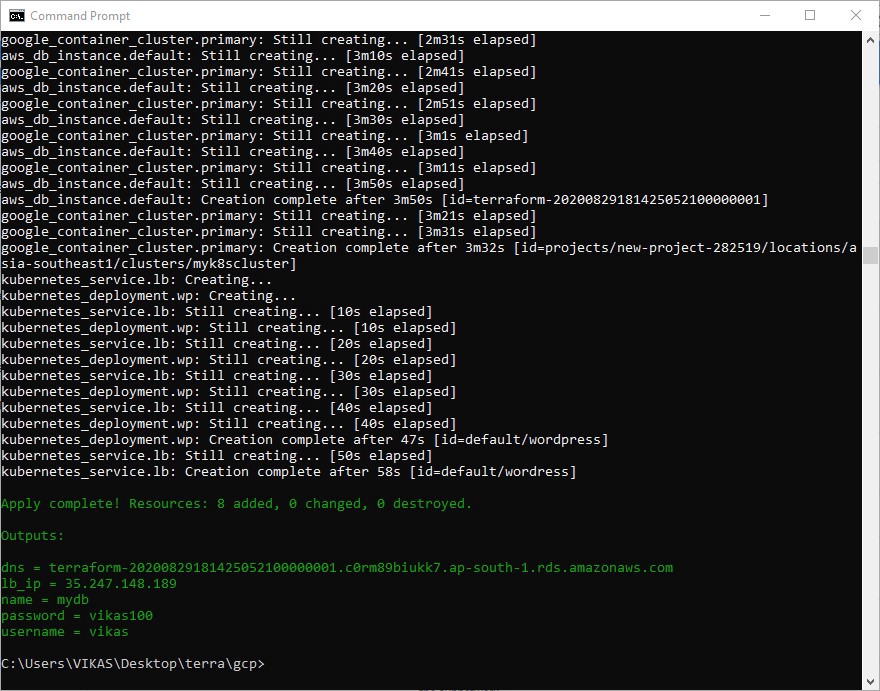
We can go to GCP console and see all the resourecs are created there (VPC, kubernetes cluster, wordpress deployment, Load balancer)
我们可以转到GCP控制台,查看在那里创建的所有资源(VPC,kubernetes集群,wordpress部署,负载均衡器)
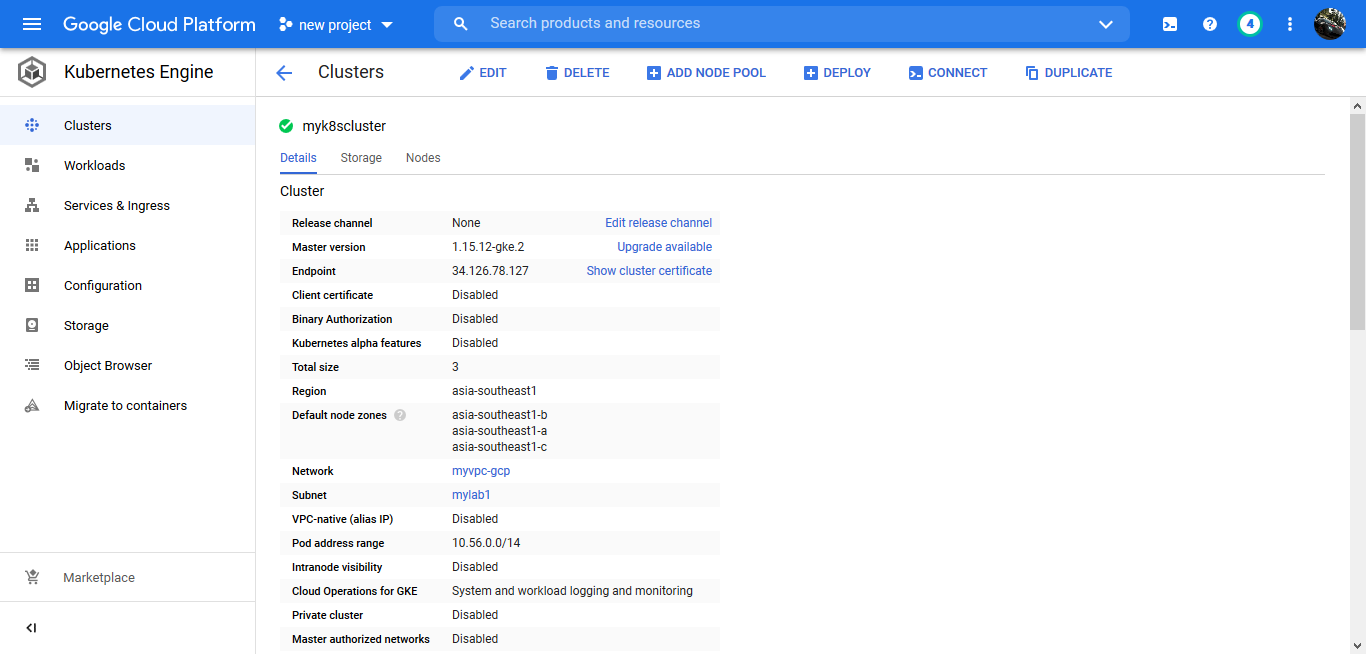
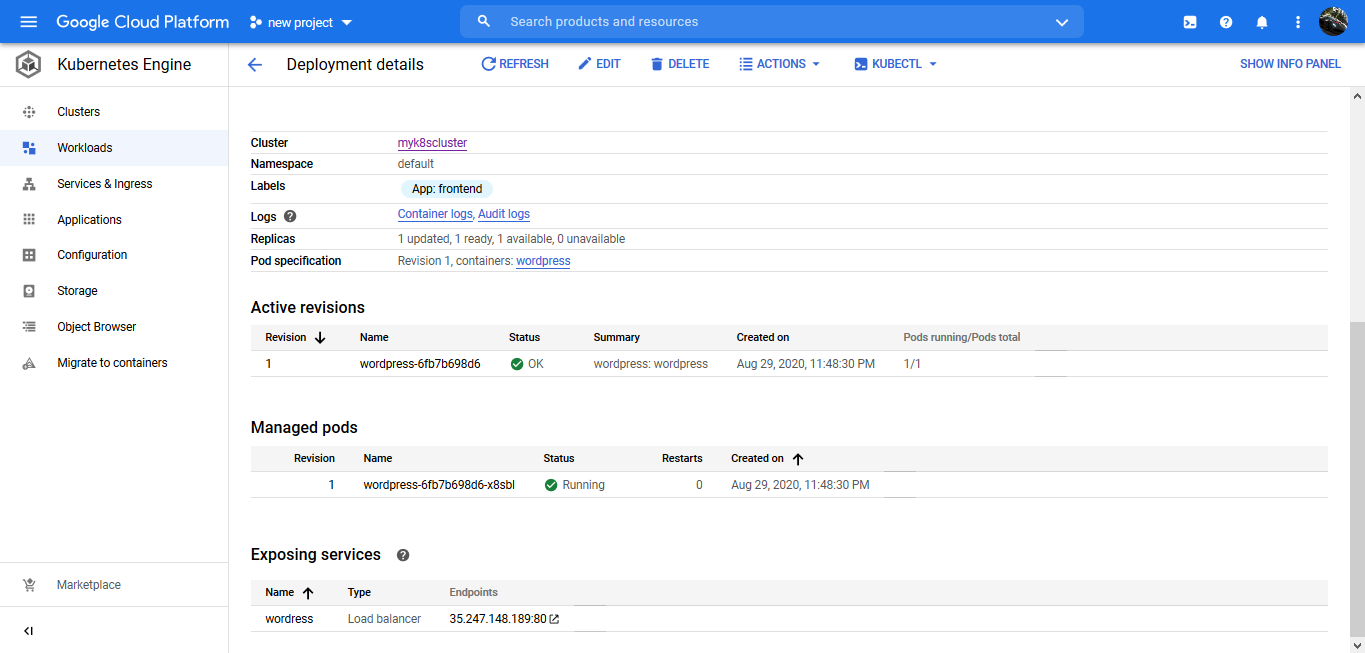
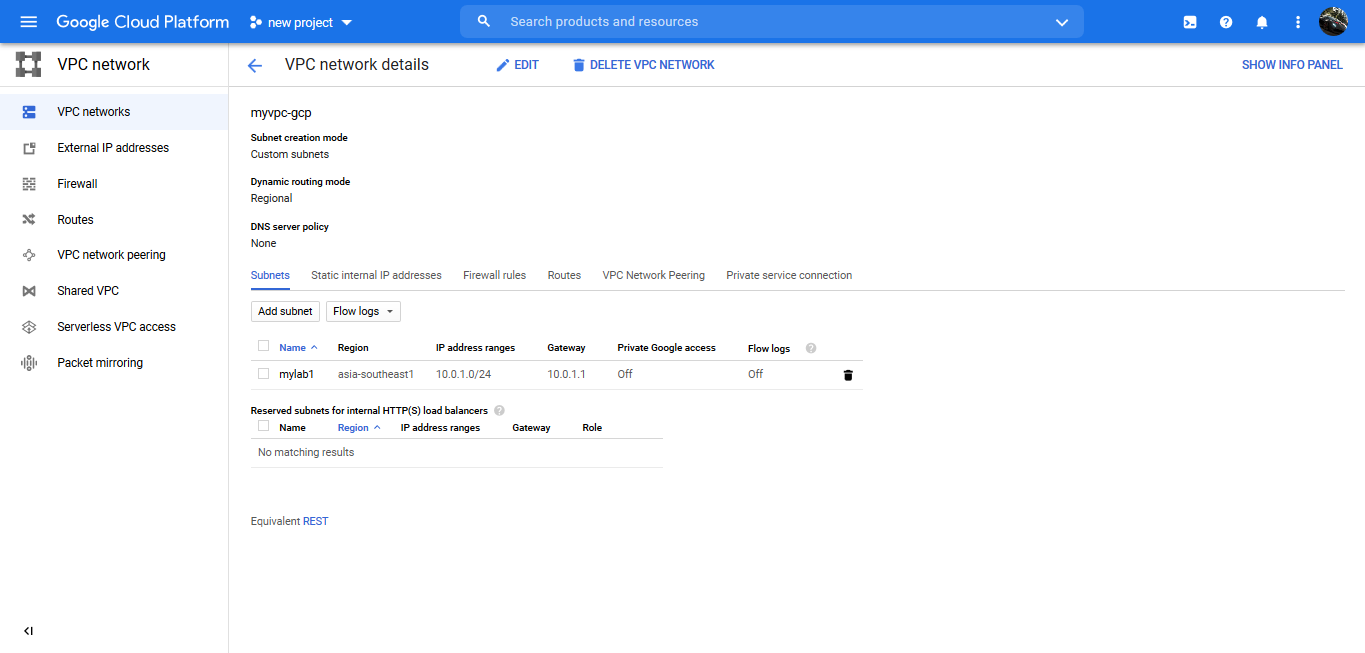
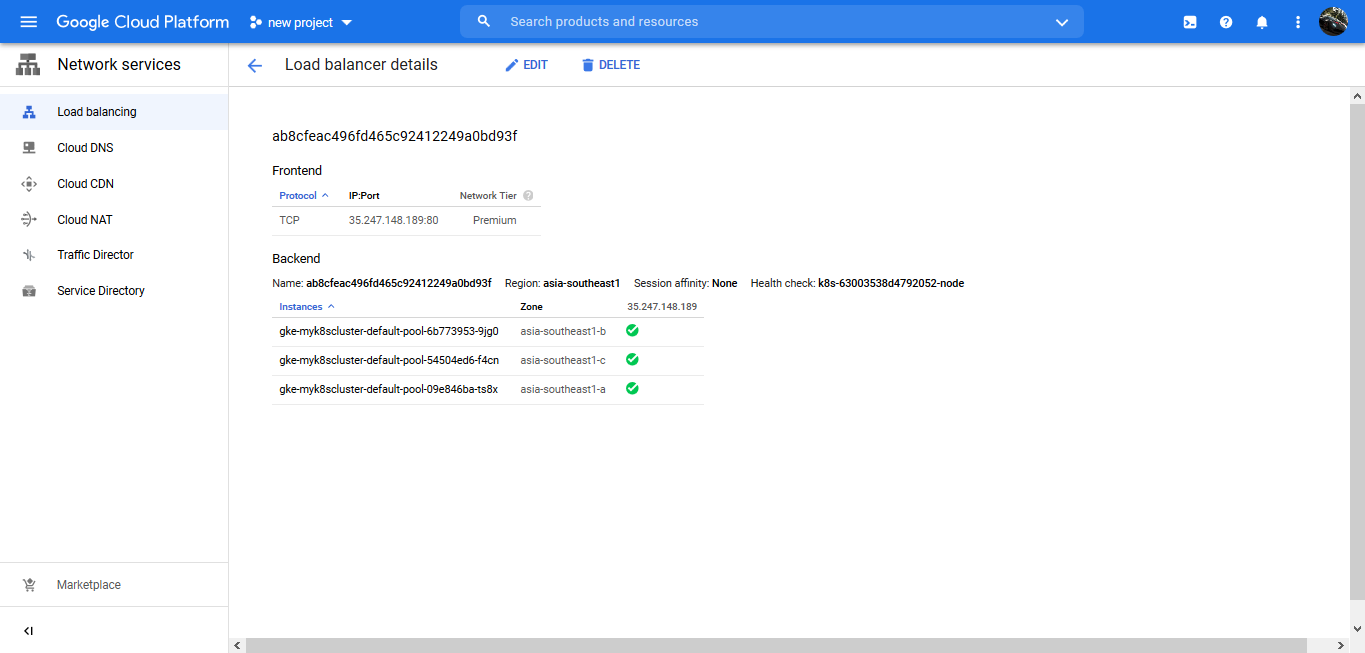
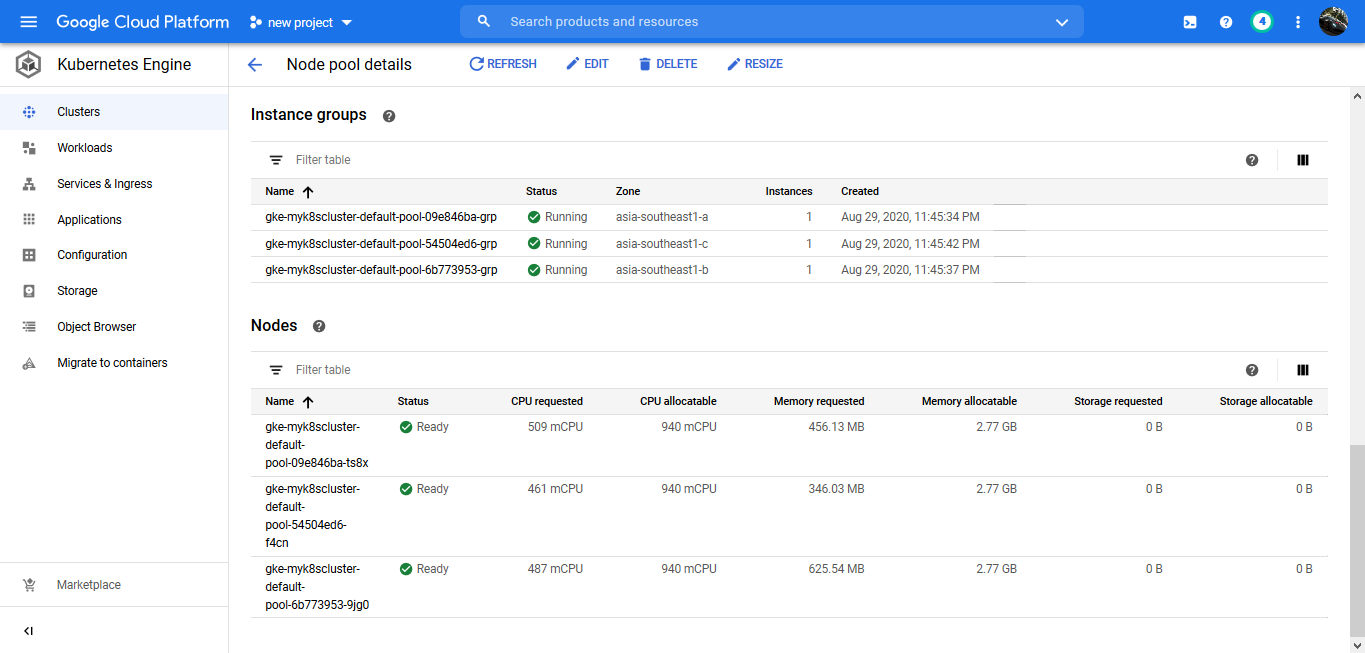
Also, in AWS our MySQL database instance is created
此外,在AWS中,我们还创建了MySQL数据库实例
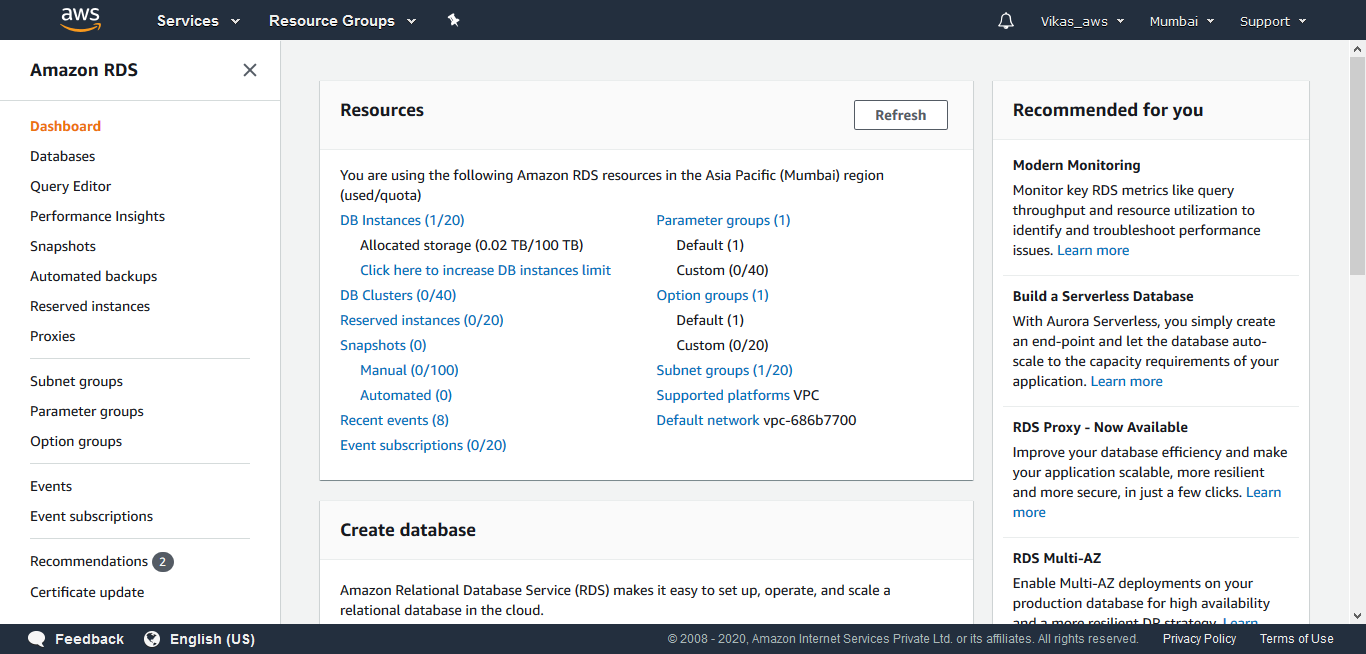
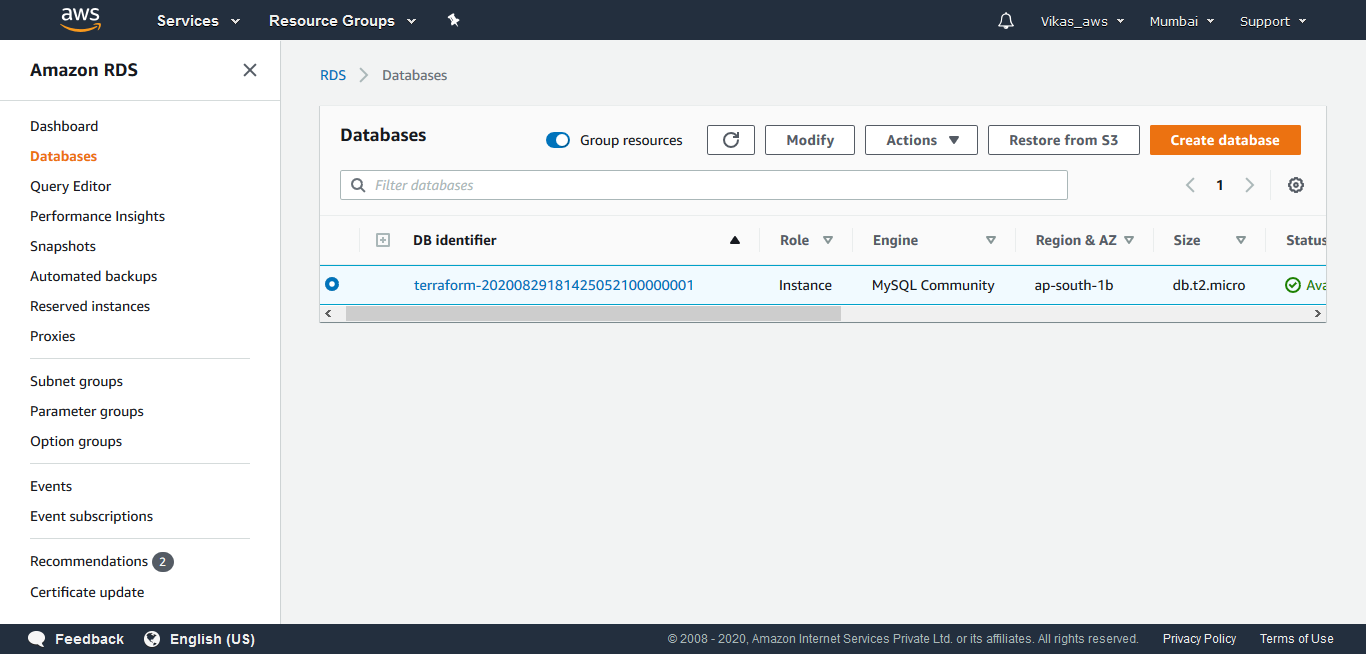
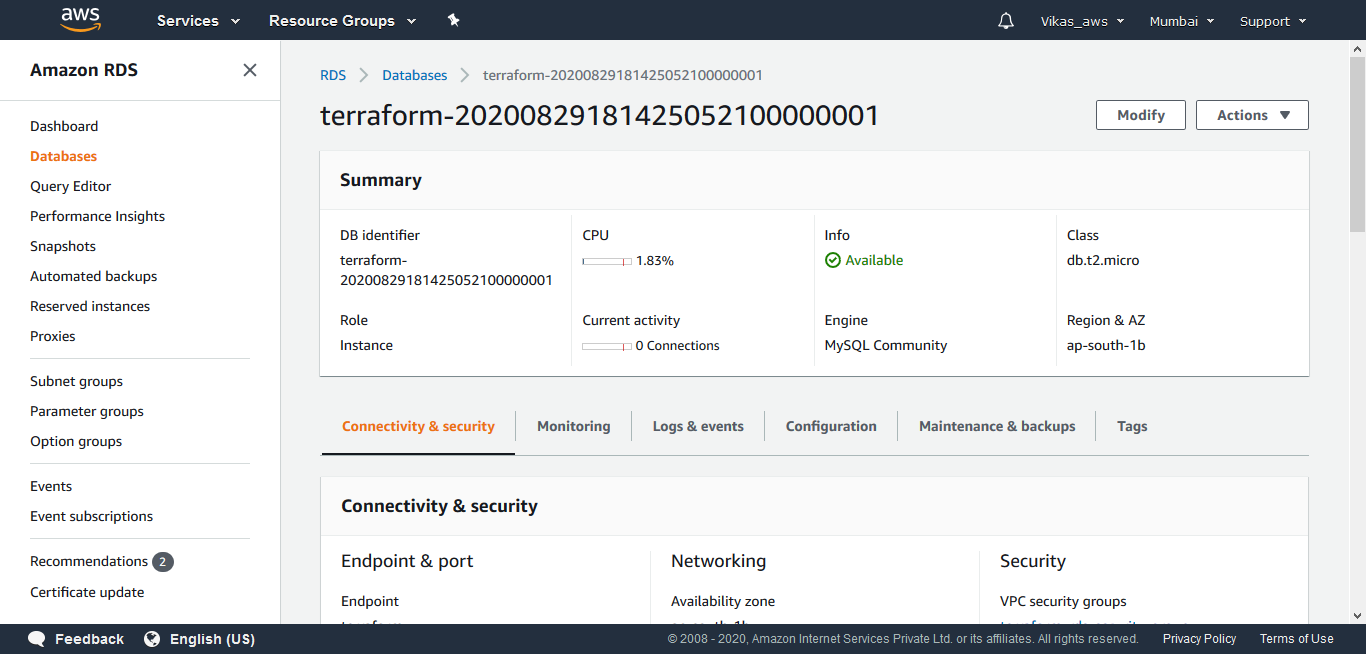
Let’s connect to our WordPress site using the load balancer ip address, connect to the database and complete the setup.
让我们使用负载均衡器ip地址连接到我们的WordPress网站,连接到数据库并完成设置。
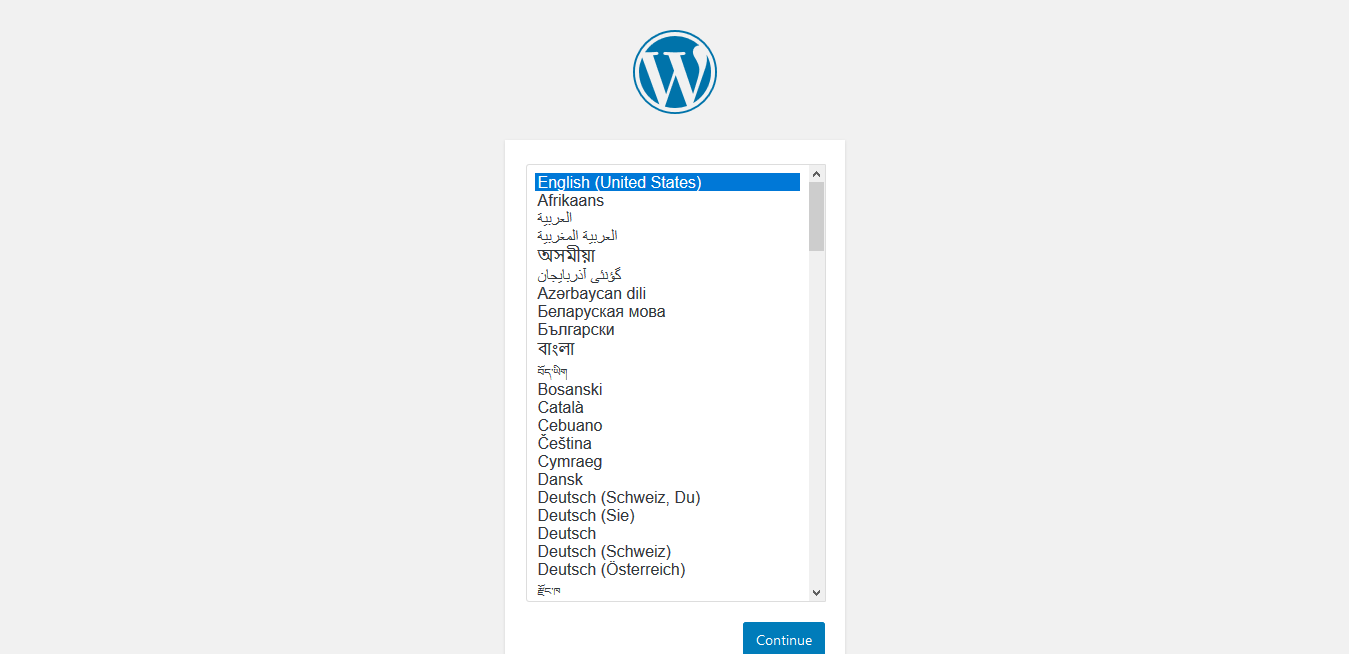
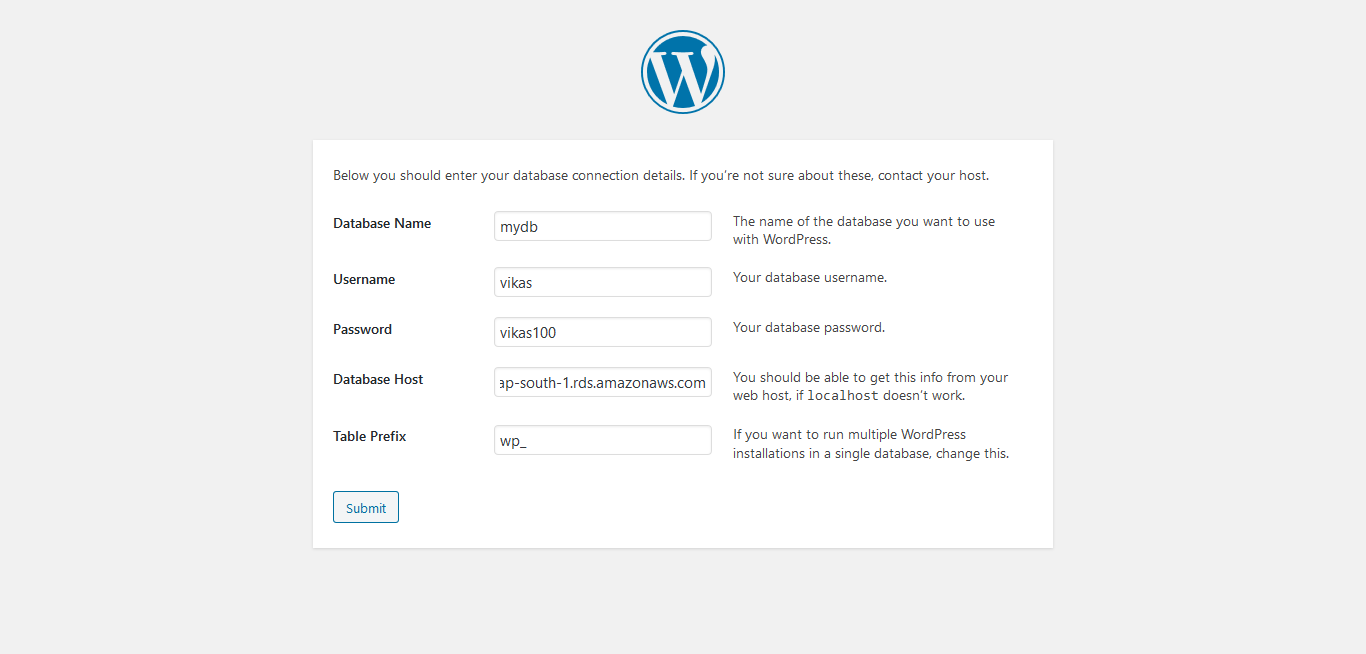
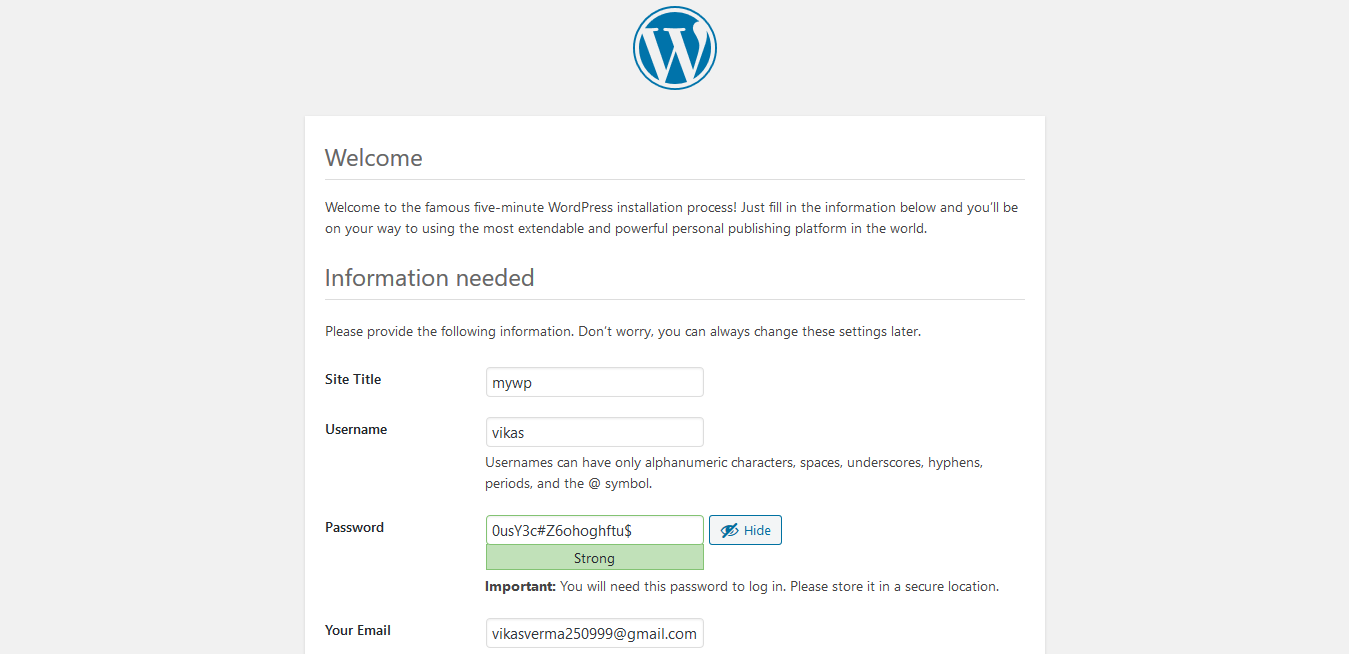
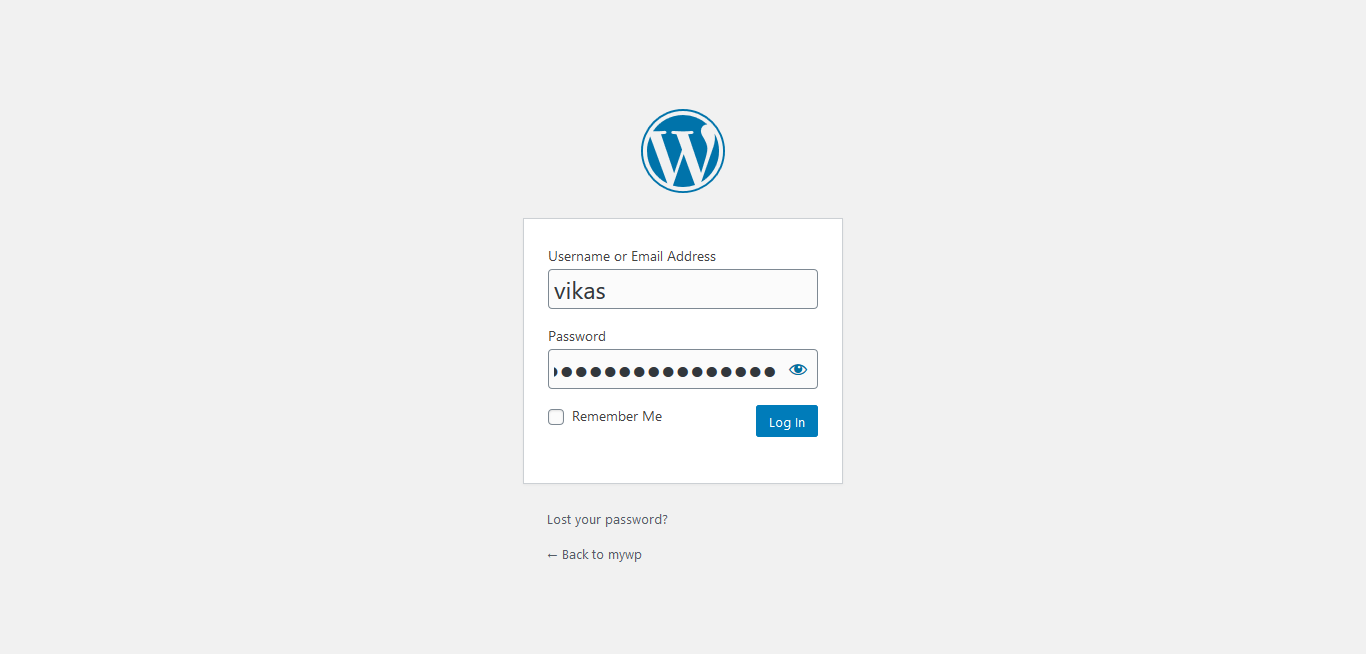
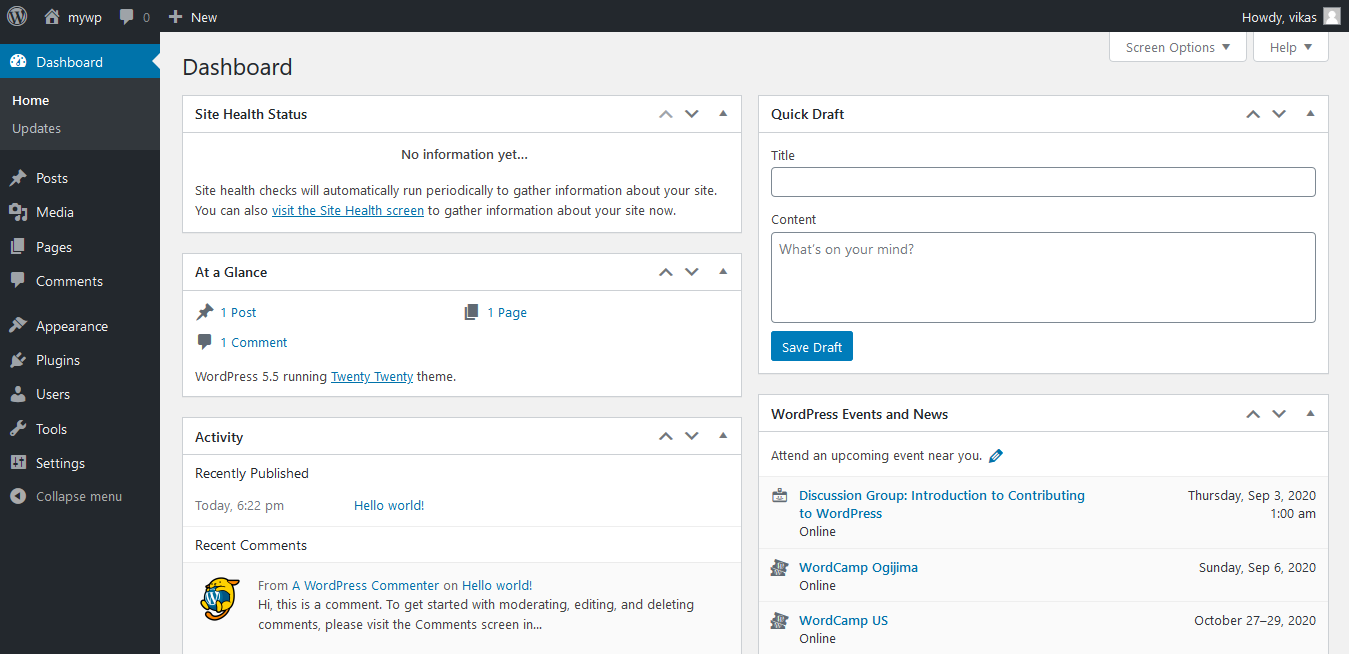
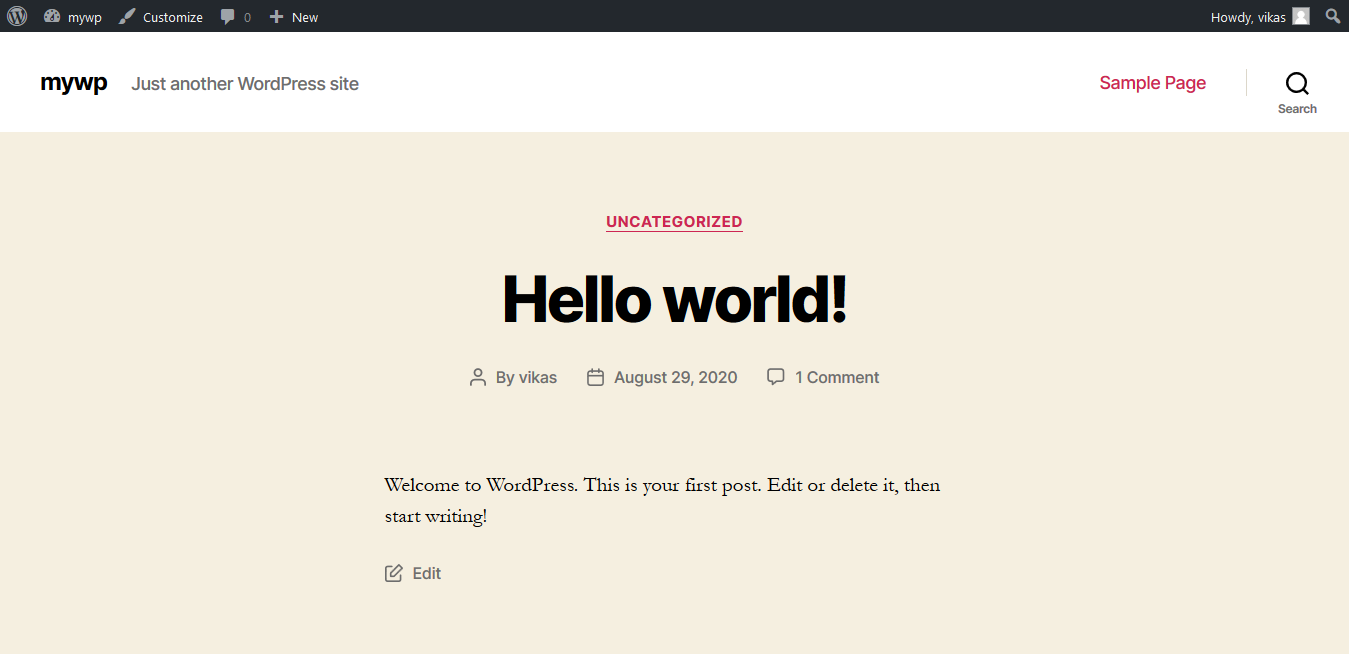
So, after doing everything we can delete all the created resources with terraform destroy command,
因此,完成所有操作后,我们可以使用terraform destroy命令删除所有创建的资源,
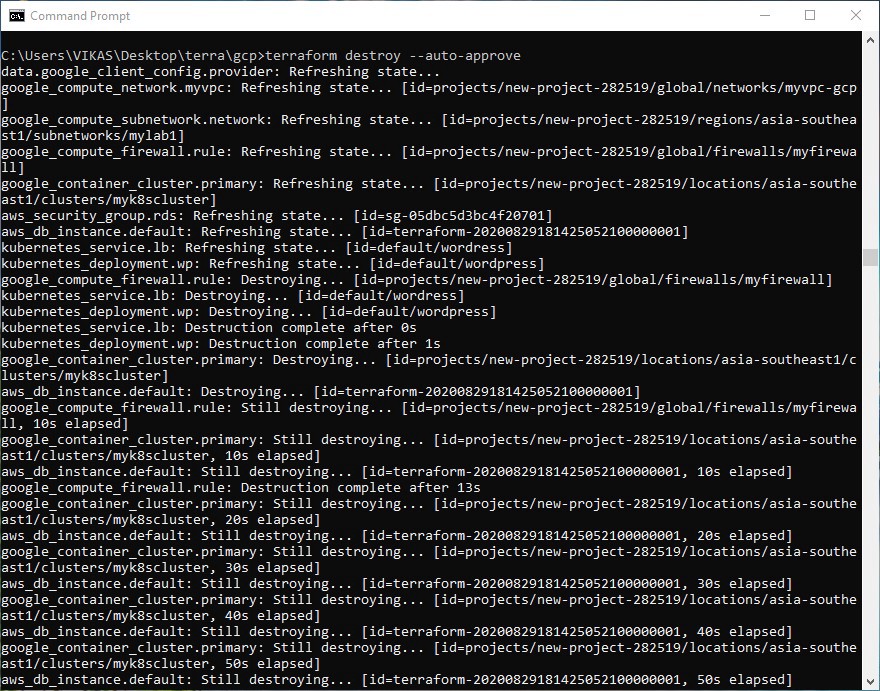
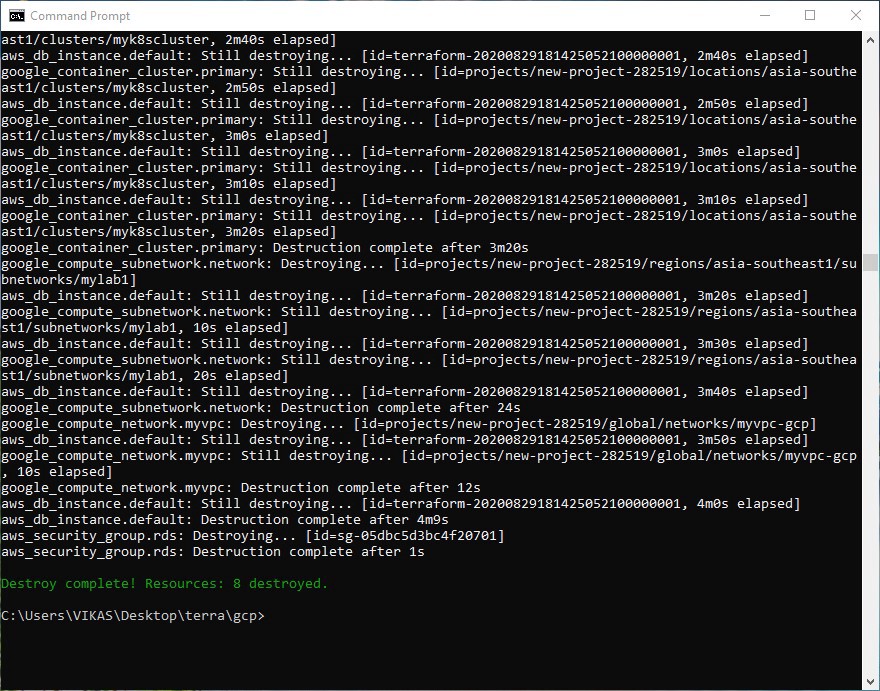
aws terraform