laravel 类不存在
Laravel 8 was released yesterday with a ton of new features and changes. One of those changes was the removal of the default route namespacing.
Laravel 8昨天发布,具有大量新功能和更改。 这些更改之一是删除了默认路由的命名空间。
Although this change is backward-compatible, meaning that older projects that used Laravel 7.x can easily migrate to Laravel 8.x without having to change anything, new projects created in Laravel 8 (starting 8/Sep) have to take this into account.
尽管此更改是向后兼容的,这意味着使用Laravel 7.x的较旧项目可以轻松迁移到Laravel 8.x,而无需进行任何更改,但是在Laravel 8中创建的新项目(从8月9日开始)必须考虑到这一点。
Many developers have been facing an issue in their newly created Laravel 8 apps where they try to load their routes and they run into an Exception that says something like:
许多开发人员在他们新创建的Laravel 8应用程序中都遇到了一个问题,他们尝试加载其路由,并且遇到了类似以下内容的Exception:
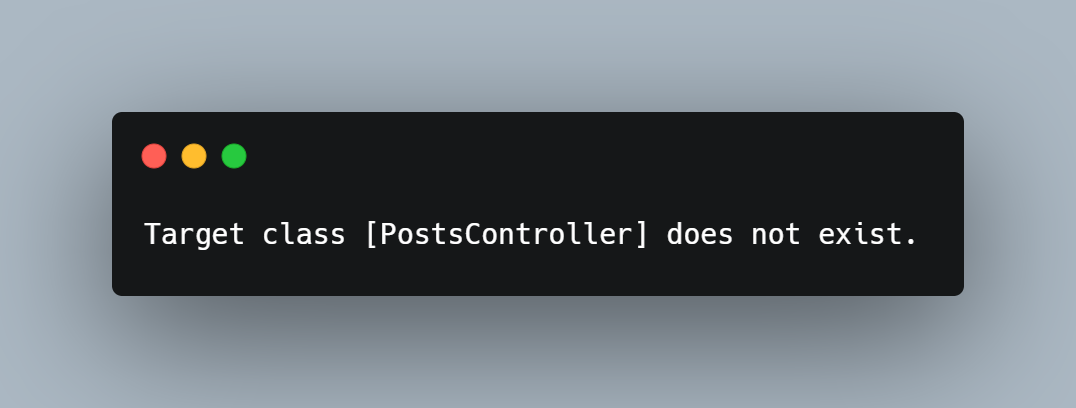
The issue is not that the code is broken, but that 99.9% of Laravel tutorials are now broken in this department because most of them relied on this default namespace for the string syntax.
问题不在于代码已被破坏,而是该部门现在已破坏了99.9%的Laravel教程,因为它们大多数都依赖于此默认名称空间来表示字符串语法。
改变(The change)
Up until Laravel 7, the RouteServiceProvider.php file had the following code:
直到Laravel 7,RouteServiceProvider.php文件具有以下代码:
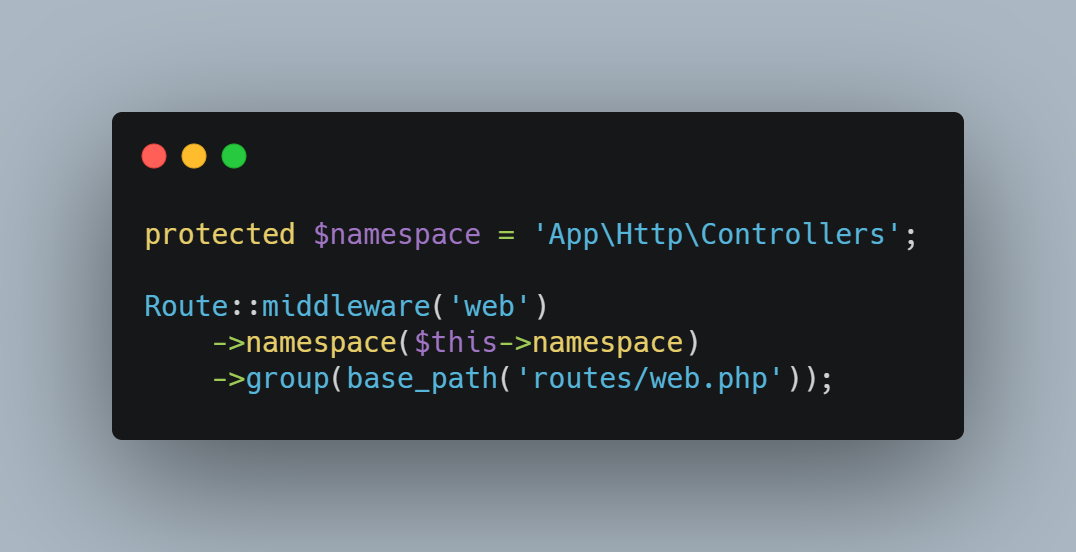
What this does is tell Laravel to load the routes in routes/web.php, using the web middleware and the App\Http\Controllers namespace. This, in turn, means that whenever you declared a route using the string-syntax, Laravel would look for that controller in the App\Http\Controllers folder:
这样做是告诉Laravel使用Web中间件和App \ Http \ Controllers命名空间将路由加载到route / web.php中。 反过来,这意味着每当您使用字符串语法声明路由时,Laravel都会在App \ Http \ Controllers文件夹中查找该控制器:
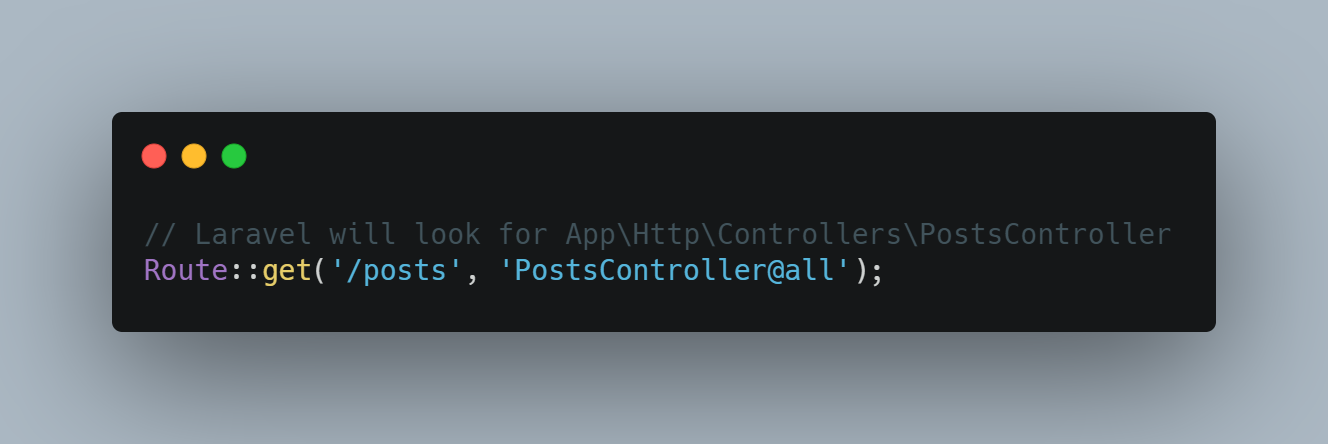
In Laravel 8, the $namespace variable was removed and the Route declaration was changed to:
在Laravel 8中,删除了$ namespace变量,并且Route声明更改为:
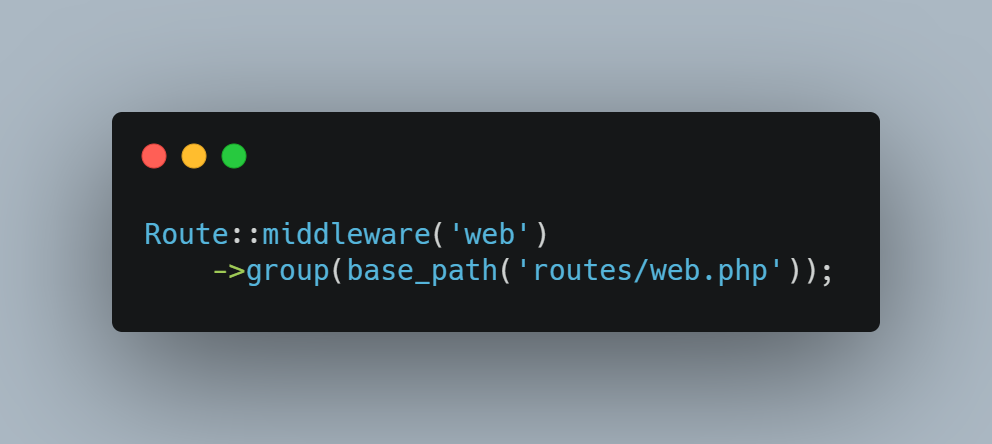
Now, Laravel is looking for routes inside your web.php file, as always. It’s also applying the web middleware, as always. But notice that it’s no longer using the previous namespace.
现在,Laravel一如既往地在web.php文件中寻找路由。 一如既往,它也在应用Web中间件。 但是请注意,它不再使用以前的名称空间。
This means that starting in Laravel 8, when you declare your routes using the string-syntax, Laravel isn’t going to look for your controller inside App\Http\Controllers.
这意味着从Laravel 8开始,当您使用字符串语法声明路由时,Laravel不会在App \ Http \ Controllers中寻找控制器。
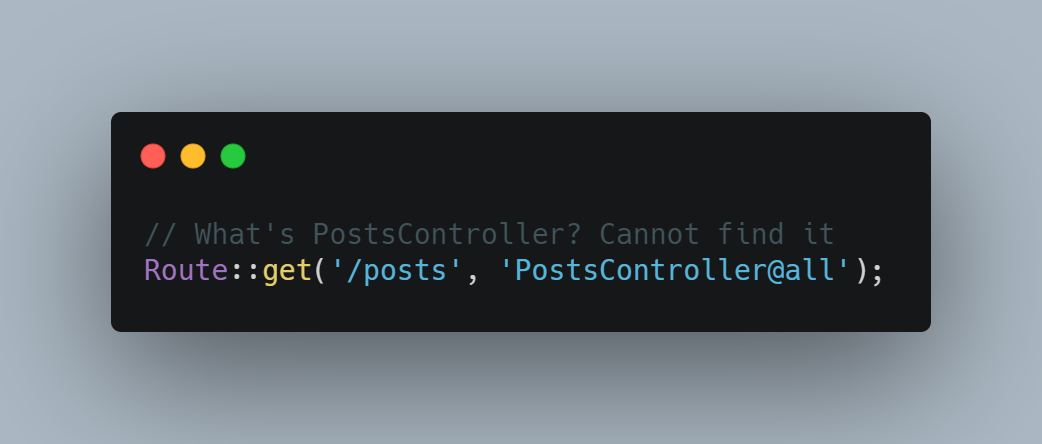
我该如何解决? (How do I fix this?)
The problem here is that Laravel has no idea where to look for your controller, so all we have to do is let it know! There are 3 ways you can accomplish this:
这里的问题是Laravel不知道在哪里寻找您的控制器,所以我们要做的就是让它知道! 您可以通过3种方式完成此操作:
- Add the namespace back manually so you can use it as you did in Laravel 7.x and before 手动重新添加名称空间,以便您可以像在Laravel 7.x及之前版本中一样使用它
- Use the full namespace in your route files when using the string-syntax使用字符串语法时,请在路由文件中使用完整的名称空间
- Use the action syntax (recommended)使用动作语法(推荐)
手动添加名称空间 (Adding the namespace manually)
This is fairly simple. Go into your RoutesServiceProvider.php file and you’ll see the following:
这很简单。 进入RoutesServiceProvider.php文件,您将看到以下内容:
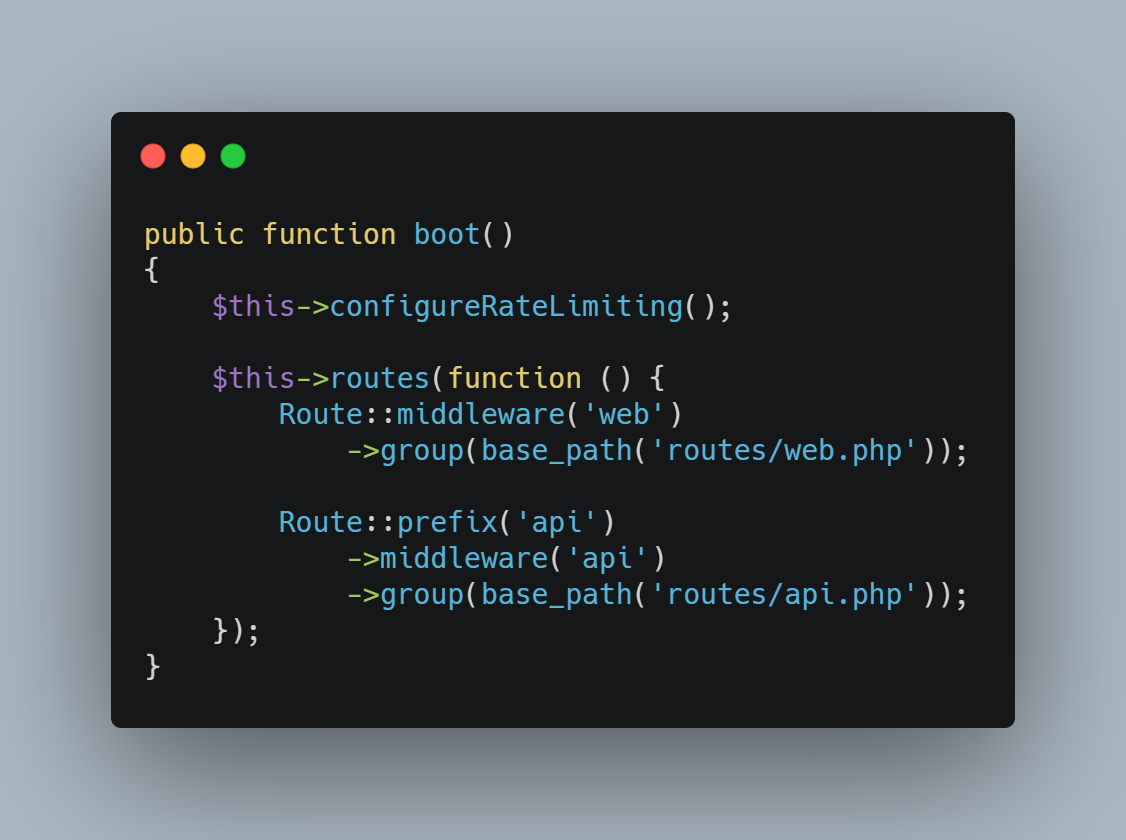
All you need to do is add the following three lines to this file and Laravel will go back to using the default namespace as in Laravel 7.x:
您需要做的就是向该文件添加以下三行,Laravel将回到使用默认名称空间的方式,如Laravel 7.x:
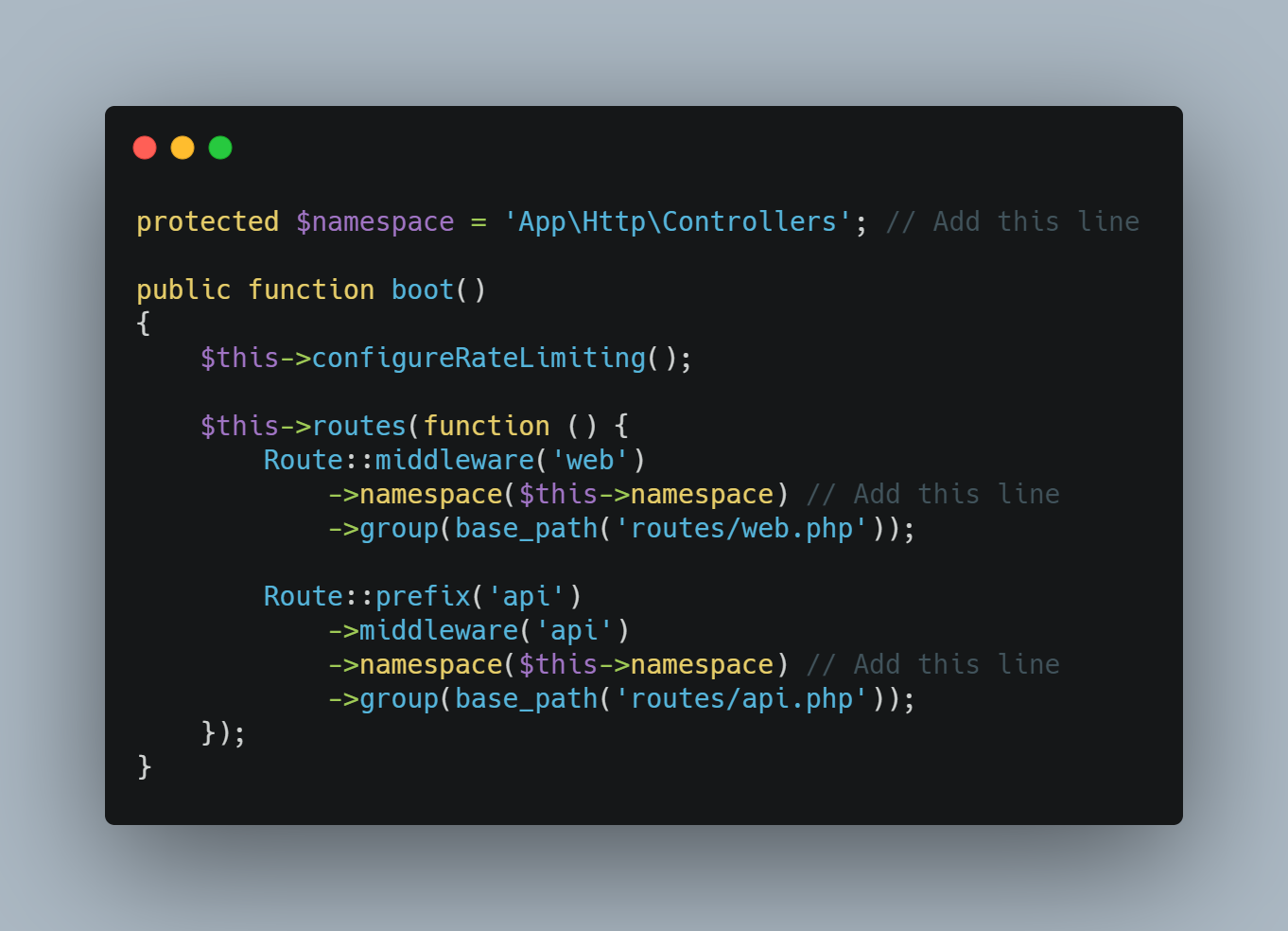
What did we just do? We declared the $namespace variable with the default Namespace for our controllers and told laravel to use that for our web and api routes.
我们刚刚做了什么? 我们为控制器声明了具有默认命名空间的$ namespace变量,并告诉laravel将其用于我们的Web和api路由。
If you try to run your app again, everything should be working.
如果您尝试再次运行您的应用程序,那么一切应该正常。
使用完整的名称空间 (Using the full namespace)
This one involves changing all your route declarations, but the idea is simple: prepend your controller names with their namespace. See the following example for our PostsController inside the app/Http/Controllers folder.
这涉及到更改所有路由声明,但是想法很简单:在控制器名称前添加名称空间。 请参阅以下示例,其中包含app / Http / Controllers文件夹中的PostsController。
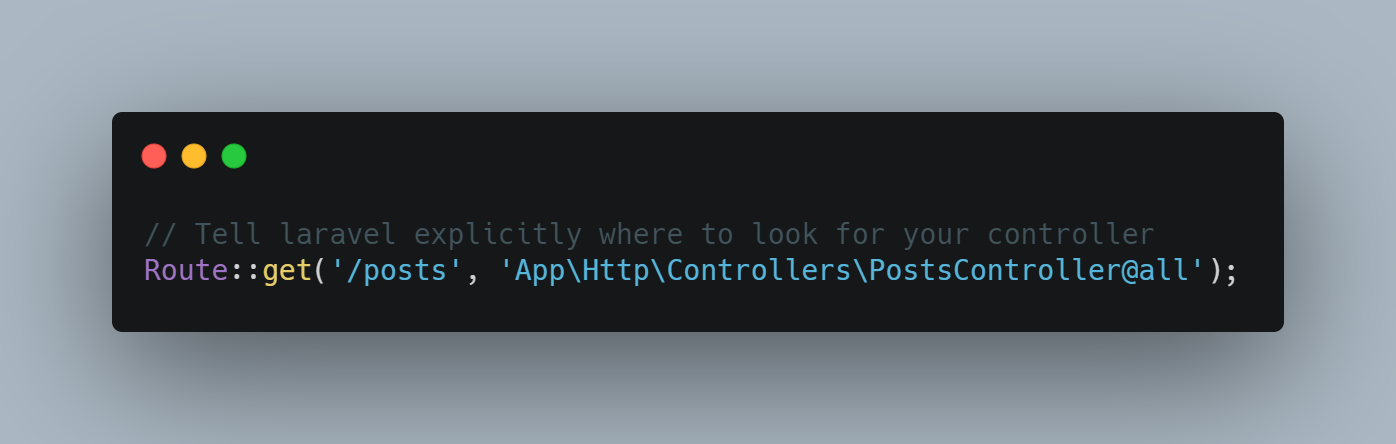
If you try again, everything should be running smoothly.
如果重试,一切应该运行顺利。
使用动作语法 (Using the Action Syntax)
This is the alternative I personally recommend as I find it more typo-proof and in my experience provides better IDE support as we are explicitly telling the code which class to use. Instead of using our usual string syntax, we can use the action syntax where we specify the class and method to use in an array:
这是我个人推荐的替代方法,因为我发现它具有更好的防错字功能,并且根据我的经验,当我们明确告诉代码要使用哪个类时,它可以提供更好的IDE支持。 除了使用通常的字符串语法,我们还可以使用动作语法,在其中指定要在数组中使用的类和方法:
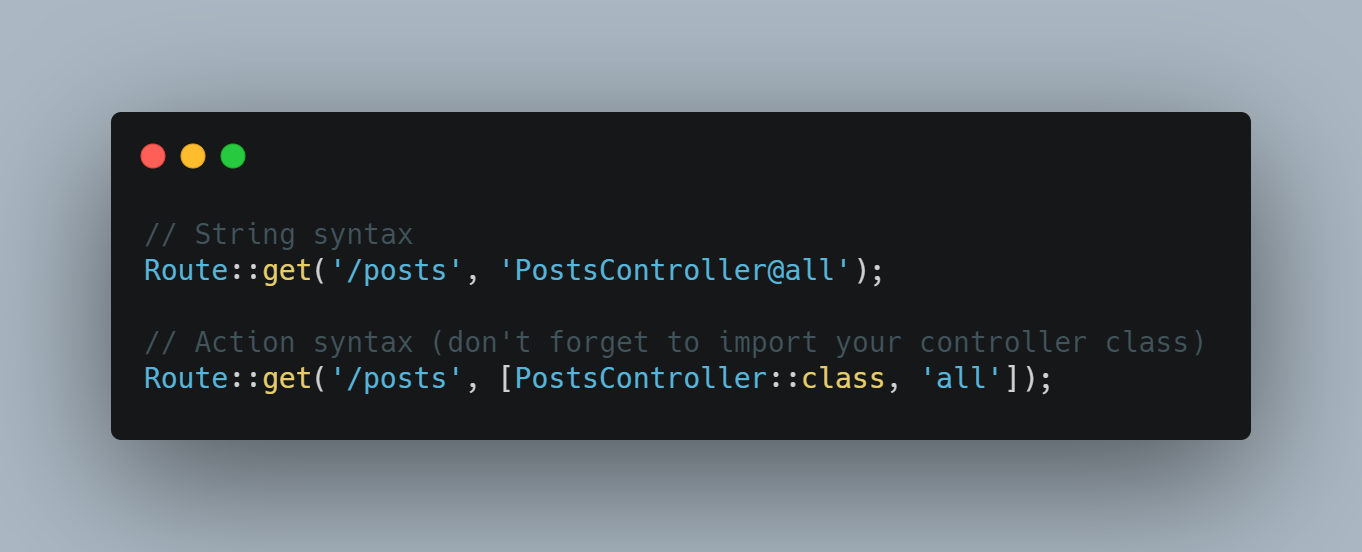
Notice here we are not passing the PostsController within quotes but rather PostsController::class, which internally will return ‘App\Http\Controllers\PostsController’. The second value in the array is the method to call within that controller, meaning: “In PostsController.php call the ‘all’ method.
请注意,此处我们不是在引号中传递PostsController,而是传递PostsController :: class,该内部将返回“ App \ Http \ Controllers \ PostsController”。 数组中的第二个值是在该控制器内调用的方法,表示:“在PostsController.php中,调用'all'方法。
Again, if you try to run your app again, everything should be up and running.
同样,如果您尝试再次运行您的应用程序,则所有内容都应已启动并正在运行。
结束语 (Closing Remarks)
By now, your app should be up and running again. If not, please feel free to ask for help. Everyone in the community is eager to give a hand.
现在,您的应用程序应该已重新启动并运行。 如果没有,请随时寻求帮助。 社区中的每个人都渴望伸出援助之手。
Whether you added the namespace manually, specified the full namespace in your routes, or went with the action syntax, what you just did is tell Laravel in which namespace your controllers actually are, so now it actually knows where to look.
无论您是手动添加名称空间,在路由中指定完整的名称空间,还是使用动作语法,您所做的只是告诉Laravel控制器实际位于哪个名称空间,因此现在它实际上知道在哪里查找。
If you liked what you read or want to learn more cool stuff related to Laravel, you can follow me on Twitter, where I post about coding, entrepreneurship, and living a better life.
如果您喜欢阅读的书籍或想学习与Laravel相关的更多有趣的知识,可以在Twitter上关注我,我在那儿发布了有关编码,企业家精神和更好生活的信息。
翻译自: https://medium.com/@litvinjuan/how-to-fix-target-class-does-not-exist-in-laravel-8-f9e28b79f8b4
laravel 类不存在