python基础教程
Being a documentarian of what I do, is my new way of learning since reading “Show your work”. It highlights the importance of sharing our work and revealing our creativity…
自从阅读“展示你的作品”以来,成为我所做记录的记录员是我的新学习方式。 它强调了分享我们的工作和展现我们的创造力的重要性。
And since I have recently joined a new internship whose main objective is to be able to apply machine learning algorithms to build predictive models… I started learning Python
而且由于我最近加入了一个新的实习生,其主要目标是能够应用机器学习算法来构建预测模型……我开始学习Python
By going through this article you’ll be able to learn the essential concepts of Python programming such as data types, tuples, lists, dictionaries, functions, regular expressions, Error & Exceptions, scope, Object-Oriented Programming…
通过阅读本文,您将能够学习Python编程的基本概念,例如数据类型,元组,列表,字典,函数,正则表达式,错误和异常,作用域,面向对象的编程…
使用Python进行编程的好处: (Benefits of programming with Python :)
- Python is widely used. Python被广泛使用。
- Python is so easy to work with and read. Python非常易于使用和阅读。
- Can be used in almost all fields. 几乎可以在所有领域中使用。
- Python is a dynamic programming language. Python是一种动态编程语言。
What makes Python a dynamic programming language?
是什么使Python成为动态编程语言?
- Variables are not bound to types. 变量未绑定到类型。
- It states the kind of variable in the runtime of the program. 它指出了程序运行时变量的种类。
第一步 : (First Step :)
Before we start diving, you can just install Python and begin working with the Python Shell installed. But I would recommend installing Anaconda which is an open-source distribution of the Python and R programming languages. It brings many of the tools used in data science and machine learning with just one install.
在开始潜水之前,您只需安装Python并开始使用已安装的Python Shell。 但是我建议安装Anaconda ,它是Python和R编程语言的开源发行版。 只需一次安装,即可带来许多用于数据科学和机器学习的工具。
After installing Anaconda, you can open the Jupyter Notebook to start coding.
安装Anaconda之后,您可以打开Jupyter Notebook开始编码。
Ps: You can consider coding in the In-browser IDE repl.it by creating your python Repl.
附:您可以考虑通过创建python Repl在浏览器内置IDE repl.it中进行编码。
现在该开始学习和编码了: (Now it’s time we start learning and coding :)
The famous “Hello World”:
著名的“ Hello World”:
print("Hello World")
So we use the print() function to print a specified message to the screen, and as you can notice, Python does not require semi-colons to terminate statements.
因此,我们使用print ()函数来打印 指定的消息显示在屏幕上,您会注意到, Python不需要分号来终止语句。
Numbers: Numbers in Python have two main forms:
号码: Python中的数字有两种主要形式:
1. Integers:
1.整数:
exp 12
实验12
2. Floating-point:
2.浮点数:
exp 12.5
经验值12.5
Variable assignment:An assignment sets a value to a variable. In order to assign a variable a value, we use the equals sign (=)
变量分配: 分配将值设置为变量。 为了给变量赋值,我们使用等号(=)
myVariable = 5 # integer
myVariable = "Hola!" # string
# Note that due to the fact that the data types are dynamically typed in python
# you can assign an integer and then a string to the same myVariable variable.
Strings:1. Are used in Python to hold text information and are indicated with the use of single quotes ‘ ‘ or double quotes “ ”.
字串: 1.在Python中保存文本信息和使用单引号'或双引号“”表示。
2. Are a sequence of characters, implying they can be indexed using bracket notation
2.是一个字符序列,表示可以使用方括号表示法对它们进行索引
[].
[] 。
3. Strings are immutable
3.字符串是不可变的
myString = "This is a string." # Assigning a string to myString variable
# Operations on strings
print(myString[0]) # T
print(myString[-1]) # .
# Slicing
print(myString[5:]) # is a string.
print(myString[:3]) # Thi
print(myString[1:6]) # his i
print(myString[::1]) # This is a string.
print(myString[::2]) # Ti sasrn.
# String Methods
print(myString.strip()) # removes any whitespace from the beginning or the end
print(myString.lower()) # this is a string.
print(myString.upper()) # THIS IS A STRING.
print(myString.replace("T", "I")) # Ihis is a string
# Concatenation
a = "he"
b = "llo"
c = a+b
print(c) # hello
# String formatting
x = "Item one: {a} Item two: {b}".format(a="1",b="2")
print(x) # Item one: 1 Item two: 2
# String Length
print(len(myString)) #17
Lists:Lists are Python’s form of Arrays.
清单: 列表是Python的数组形式。
myList =[1,2,"bob"]
print(myList[1]) # 2
myList.append(3) # adds an item to the end of the list
print(myList) # [1,2,"bob",3]
myList.append([4,5])
print(myList) # [1,2,"bob",3,[4,5]]
myList.extend([4,5])
print(myList) # [1,2,"bob",[4,5],4,5]
myList.reverse() # reverses the elements of the list
print(myList) # [5,4,[4,5],"bob",2,1]
myList.pop() # removes the item at the given index from the list
print(myList) # [1,2,"bob",[4,5],4]
list2 = [2,4,1]
list2.sort() #sorts the elements of a list
print(list2) # [1,2,4]
list3 = [1,2,['x','y','z']]
print(list3[2][1]) # y
Dictionary:An unordered, changeable and indexed collection with key-value pairs.
字典: 具有键值对的无序,可变和索引集合。
# empty dictionary declaration
my_dict = {}
# dictionary with integer keys
my_dict = {1: 'apple', 2: 'ball'}
# dictionary with mixed keys
dic ={'1':'a','key2':'b'}
print(dic['key2']) # b
myDic ={'key1':{'123':['a',1,'b']}}
print(myDic['key1']['123'][2]) # b
# It is possible to update a value
myDic['key1'] ='12'
print(myDic) # {'key1': '12'}
Tuple:An ordered and immutable collection of objects that cannot be changed, unlike lists, and use parentheses.
元组: 与列表不同,使用括号的不能更改的对象的有序且不可变的集合。
Set:An unordered collection of unique elements.
组: 独特元素的无序集合。
# tuples
t=(1,2,3)
print(t[0]) # 1
# sets
x=set()
x.add(1)
x.add(2)
print(x) # {1,2}
何时使用列表,字典或集?(When to use List, Dictionary or Set?)

* Use a list when you care about the order because a list keeps order, dict and set don't.
*当您关心订单时,请使用列表,因为列表会保持顺序,字典和设置不会。
* A dict uses the key-value association, while list and set just contains values.
* dict使用键值关联,而list和set仅包含值。
* A set expects items to be hashable, a list doesn't. So for non-hashable items, you must instead use list and cannot use set.
*集合期望项目是可哈希的,列表则不是。 因此,对于非哈希项,您必须改为使用list,而不能使用set 。
*A set bans duplicates, while it is allowed within a list.
*集合禁止重复,而列表中允许重复。
If .. else statements:Python maintains the usual logical conditions of mathematics ( ==, !=, >, ≥ , < , ≤)
如果.. else语句: Python维护数学的通常逻辑条件(==,!=,>,≥,<,≤)
Note that to indicate a block of code, Python uses indentation.
请注意,为了表示代码块, Python使用了indentation 。
# general syntax
if(first_condition):
Indented statement block for first_condition
elif(second_condition):
Indented statement block for second_condition
else:
Catches anything that is not caught by the previous conditions.
# example
a = 5
b = 2
if b > a:
print("b is greater than a")
elif a == b:
print("a and b are equal")
else:
print("a is greater than b")
# Output: a is greater than b
Loops:1.while loop executes a set of statements as long as a condition is true
循环: 1.while循环只要条件为真就执行一组语句
2.for loop executes a set of statements, once for each item in a list, tuple...
2.for循环执行一组语句,对于列表中的每个项目,一次元组...
# for loop
animals = ["cat", "dog", "rabbit"]
for item in animals:
print(item)
# while loop
j = 1
while j < 5:
print(j)
j += 1
It is also possible to use the break, continue, and else statements with while and for loops, each having its own role when used.
也可以将break,continue和else语句与while和for循环一起使用,使用时每个循环都有其自己的作用。
List Comprehension:A neat way to define and create a list in Python
清单理解: 在Python中定义和创建列表的一种巧妙方法
# an ordinary for loop to create the out list
x= [1,2,3,4]
out= []
for el in x:
out.append(el*2)
# output [2,4,6,8]
# same output using list comprehension
out = [ el *2 for el in x]
Functions:A function in Python is defined using the def keyword
功能: 使用def关键字定义Python中的函数
Here’s the general syntax for creating and calling a function
这是创建和调用函数的一般语法
def my_function(arg1):
print(arg1)
my_function("Hello World")
# We use the return statement to let the function retun a value
def addition_function(a,b):
return a+b
print(addition_function(5,6)) # Output: 11
Lambda:A Lambda function is an anonymous function meaning that it has no name. It usually has no more than a line, can take any number of arguments, but can only have one expression.
Lambda: Lambda函数是一个匿名函数,表示它没有名称。 它通常只有一行,可以接受任意数量的参数,但只能有一个表达式。
# syntax
lambda arguments : expression
# example of a lambda function that multiplies two numbers
l = lambda x, y : x * y
print(l(5, 6))
Scope:Python Scope follows the LEGB Rule:
范围: Python Scope遵循LEGB规则:
-
--
Local: names assigned in a function ‘def or lambda’, and not declared global in that function
本地:在函数“ def或lambda”中分配的名称,但未在该函数中声明为全局
-
--
Enclosing Function locals: names in the local scope of all enclosing functions (def or lambda), from inside to outside.
封闭函数的本地变量:所有封闭函数(def或lambda)在本地范围内的名称,从内到外。
-
--
Global: names assigned to the top level of a module file or declared global in a def function within the file.- Built-in: names preassigned in the built-in names module
全局:分配给模块文件顶层或在文件内的def函数中声明为全局的名称。 -内置:内置名称模块中预先分配的名称
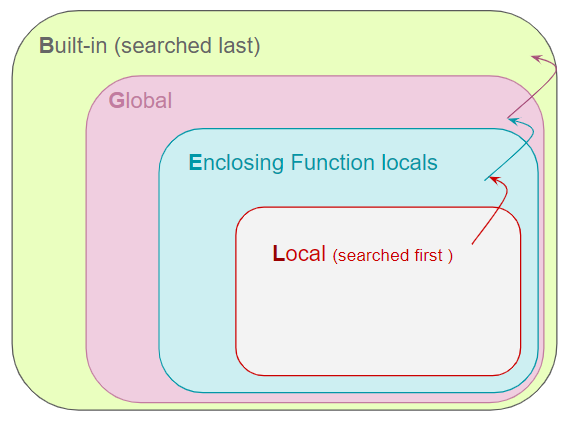
Object-Oriented-programming:Well, this subject is very essential and fundamental to deepen your knowledge of python. I’ll cover the syntactic part of creating a class and instantiating it.
面向对象编程: 好了,这个主题对于加深您对python的了解是非常重要和根本的。 我将介绍创建类并实例化它的语法部分。
# Create a circle class
class Circle():
pi=3.14 # class attriute
def __init__(self,radius): # will be executed when the class is being initiated, this function is present in all classes
self.radius = radius
# self parameter is used to access variables that belongs to the class in our example it's the radius variable
def __str__(self): # a speacial method to be able to print the string representation of an object
return "value of radius {}".format(self.radius)
def area(self): # an object method that will calculate the area
return self.radius*2*Circle.pi
def set_radius(self,new_radius): # an object method that will be used by an object of the class circle to set a new radius value for the object
self.radius=new_radius
# Create Object
c = Circle(2)
print(c.radius) # Output : 2
# delete the c object
del c
Error & Exceptions:Two types of error occur in python:
错误与例外: python中发生两种错误:
1-
1-
Syntax errors for which the program will stop the execution.
程序将停止执行的语法错误。
2-
2
Exceptions that are raised when certain internal events occur and modify the normal flow of the program.
当某些内部事件发生并修改程序的正常流程时引发的异常。
# syntax with an example showing how to open a file and try to write to it
try:
f=open('first.txt','r')
f.write("I'm trying to write in the first file")
except: # this exception will raise if the action of opening the file and/or writing to it fails
print("error, could not write in the file or file not found")
else: # in case of success of the precedent instructions
print("success")
f.close()
finally: # works even if there's an exception
print("I always work no matter")
# output: error, could not write in the file or file not found
# I always work no matter
Regular Expressions:A regular expression is a special sequence of characters that serve to match or find other strings or sets of strings, using a specialized syntax included in a pattern.
常用表达: 正则表达式是特殊的字符序列,可使用模式中包含的特殊语法来匹配或查找其他字符串或字符串集。
import re
# syntax
re.search("the string we're looking for","the sought string")
#retrun the match index
txt = "this is a string with term1 "
match = re.search('term1',txt)
print (march.start()) # the output will indicate the position of the string term1 in txt => 22
# split method returns a list where the string has been split at each match
split_term = '@'
email = 'user@gmail.com'
print(re.split(split_term, email)) # output is ['user', 'gmail.com']
# returns a list containing all matches for the searched term
print(re.findall('match','test match in match')) # print(re.findall('match','test match in match'))
# use of metacharacters
# we'll create a function to be able to see multiple examples at once
def multi_re_find(patterns,phrase):
for pat in patterns:
print(re.findall(pat,phrase))
# examples
phrase ="aba..aab..abababa...aabb"
pattern =['ab*'] # will look for an 'a' followed by 0 or more 'b'
multi_re_find(pattern,phrase) # output: ['ab', 'a', 'a', 'ab', 'ab', 'ab', 'ab', 'a', 'a', 'abb']
pattern =['ab+'] # will look for an 'a' followed by 1 or more 'b'
multi_re_find(pattern,phrase) # output: ['ab', 'ab', 'ab', 'ab', 'ab', 'abb']
pattern =['ab?'] # will look for an 'a' followed by 0 or 1 'b'
multi_re_find(pattern,phrase) # output: ['ab', 'ab', 'ab', 'ab', 'ab', 'abb']
pattern =['ab{1,3}'] # will look for an 'a' followed by 1 or 3 'b'
multi_re_find(pattern,phrase) # output: ['ab', 'ab', 'ab', 'ab', 'abb']
pattern =['a[ab]+'] # will look for an 'a' followed by either 1 or more 'a' or 1 or more 'b'
multi_re_find(pattern,phrase) # output: ['aab', 'abababa', 'aabb']
phrase = 'aa!bb?aba?'
pattern =['[^!.?]+'] # will remove every ? and !
multi_re_find(pattern,phrase) # output: ['aab', 'abababa', 'aabb']
phrase = 'aa123aba'
pattern =[r'\d+'] # will retun the digit part
multi_re_find(pattern,phrase) # output: ['123']
pattern =[r'\D+'] # will return the non digit par
multi_re_find(pattern,phrase) # output: ['aa', 'aba']
The article is finally coming to an end. Hope this covers what you should know, fellow reader, as a newbie to python programming.
这篇文章终于结束了。 希望读者能读到,作为其他的Python编程新手,您应该知道。
I can now say that learning Python is by far a good decision I made, to advance my programming skills. I hope this applies to you too.
我现在可以说,到目前为止,学习Python是提高我的编程技能的一个不错的决定。 我希望这也适用于您。
And keep in mind that the idea here is not to memorize everything at once, but just be able to use this article as a reference.
并且请记住,这里的想法不是立即记住所有内容,而只是可以将本文用作参考。
You can start applying what you learned by practising here. Enjoy it!
您可以通过在这里练习来开始应用所学到的知识。 好好享受!
python基础教程