When Javascript developers think of ECMA, they reference the changes made in 2015 that improved syntax and added new features to the language. However, ECMA still updates the language! And earlier this year, they released ES11, with two of my new favorite tools: Optional Chaining and Null Coalescing!
当Javascript开发人员想到ECMA时,他们会引用2015年所做的更改,这些更改改进了语法并为该语言添加了新功能。 但是,ECMA仍会更新语言! 今年早些时候,他们发布了ES11,其中包含我最喜欢的两个工具:可选链接和Null合并!
空的简史 (A Brief History of null)
null is a very interesting datatype. It is a primitive type, along with String, Number, Boolean, Symbol, and Undefined. (Side note: as of ES11, BigInt is now another primitive type!) Primitive types are immutable, while objects (including arrays), can be mutated.
null是一个非常有趣的数据类型。 它是原始类型,以及字符串,数字,布尔值,符号和未定义。 (附带说明:从ES11开始,BigInt现在是另一个原始类型!)原始类型是不可变的,而对象(包括数组)则可以被突变。
However, null is the odd primitive out. When you use typeof
on any datatype, you are given a reasonable and expected response: typeof 3
is a Number
, typeof {foo: "bar"}
is an Object
, etc. Due to the way Javascript was developed, null is considered an Object
when typeof
is applied to it. Check out this brilliant article by Dr. Axel Rauschmayer for more detail!
但是, null是奇数基元。 在任何数据类型上使用typeof
时,都会得到合理且预期的响应: typeof 3
是Number
, typeof {foo: "bar"}
是Object
,等等。由于开发Javascript的方式,将null视为Object
当将typeof
应用于它时。 请查看Axel Rauschmayer博士的精彩文章,了解更多详细信息!
null is a falsey value, is loosely equal to undefined (undefined == null // TRUE
), and, most importantly represents the intentional absence of any object value. When a value is not defined, it will always receive the value of undefined. When Javascript developers declare a variable but want it to be empty, they will set the value to null. null is never assigned by Javascript, but only by developers; it is a useful tool to give a value of null if it does not have any meaningful data. Getting a value of null means that your variable is declared and does exist, automatically informing you that you don’t have to adjust variable declaration, hoisting, or scope issues: the value is usable, just void of any information.
null是一个虚假值,大致等于undefined( undefined == null // TRUE
),并且最重要的是,它表示有意缺少任何对象值。 当未定义值时,它将始终接收undefined的值。 当Javascript开发人员声明变量但希望它为空时,他们会将值设置为null 。 null永远不会由Javascript分配,而只能由开发人员分配; 如果它没有任何有意义的数据,则它是一个提供null值的有用工具。 获得null值表示变量已声明且确实存在,并自动通知您不必调整变量声明,提升或范围问题:该值是可用的,几乎没有任何信息。
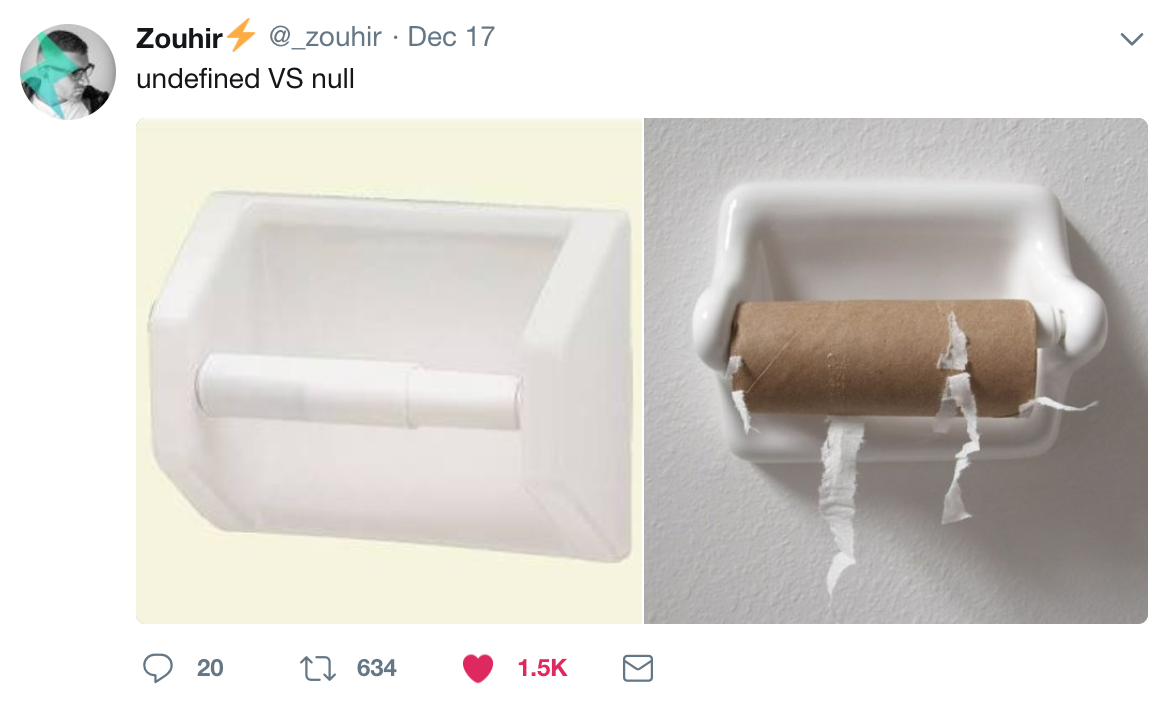
可选链接(Optional Chaining)
Has this ever happened to you?!
你曾经发生过这些事情吗?!
const friendData = props.user.friends.map(callback)Uncaught TypeError: cannot read property 'friends' of undefined
Your application needs to access some data, and you rely on a chain of methods or properties to evaluate the data you really want. Optional Chaining is here to rescue you!
您的应用程序需要访问一些数据,并且您依赖于一系列方法或属性来评估您真正想要的数据。 可选链条可以为您解救!
Optional Chaining lets you keep your nested chain without having to add a whole bunch of conditionals. If the property cannot be accessed, the remaining methods or properties do not evaluate, and the return value is undefined. All you have to do is add a ?
just after the value that could be empty, and JS will do the rest
可选的链接使您可以保留嵌套链,而不必添加一堆条件。 如果无法访问该属性,则剩余的方法或属性将不求值,并且返回值是undefined 。 您所要做的就是添加一个?
在可能为空的值之后,其余的将由JS完成
const friendData = props.user?.friends.map(callback)console.log(friendData)
// undefined
However, this can be misleading; my value declared, and I don’t want it to be undefined. That’s where Nullish Coalescing comes to the rescue!
但是,这可能会引起误解。 我声明了我的值,但我不希望它被不确定。 那就是Nullish合并救援的地方!
空位合并 (Nullish Coalescing)
Nullish Coalescing will check if a value is nullish (that is, either null or undefined), and allow some other evaluation to occur. To use the above example, if the chain of methods becomes undefined, the new nullish coalescing operator ??
will change the value.
Nullish Coalescing将检查值是否为空(即null或undefined ),并允许进行其他评估。 使用上面的示例,如果方法链变得不确定,则新的空值合并运算符??
将更改值。
const friendData = props.user?.friends.map(callback) ?? []console.log(friendData)
// []
I could make this chain evaluate to an array, or null, depending on what the subsequent data needs. This new operator also works for conditionals!
我可以使此链评估为数组,或者为null ,具体取决于后续数据的需求。 此新运算符也适用于条件句!
const value = previousVariable ?? false
The nullish coalescing operator will not trigger if the value on the left side is a falsey value like "", false, 0
, but only if it is null or undefined.
如果左侧的值为"", false, 0
值(例如"", false, 0
,则只有在null或undefined的情况下"", false, 0
合并运算符才会触发。
Always be on the lookout for new ECMAScript features; ES6 is not the final form of JavaScript! I hope you find some use with these new ES11 features!
始终在寻找新的ECMAScript功能; ES6不是JavaScript的最终形式! 希望您能从这些新的ES11功能中找到一些用处!