In my previous article, we discussed Java lists, queues, and sets in the Java Collection Interface. If you are not familiar with lists, queues, and sets. Let’s dive into Java Map Interface.
在上一篇文章中 ,我们讨论了Java集合接口中的Java列表,队列和集合。 如果您不熟悉列表,队列和集合。 让我们深入研究Java Map Interface。
什么是Java Map接口? (What is the Java Map Interface?)
The Map interface is present in the java.util package and represents a mapping between a key and a value. The Map interface is not a subtype of the Collection Interface. Therefore it behaves a bit differently from the rest of the collection types.
Map接口存在于java.util包中,它表示键和值之间的映射。 Map接口不是Collection接口的子类型。 因此,它的行为与其余集合类型略有不同。
A Map is an object that maps keys to values. A Map cannot contain duplicate keys: Each key can map to at most one value. It models the mathematical function abstraction. The Map the interface includes methods for basic operations (such as
put
,get
,remove
,containsKey
,containsValue
,size
, andempty
).映射是将键映射到值的对象。 映射不能包含重复的键:每个键最多可以映射到一个值。 它为数学函数抽象建模。 Map接口包含用于基本操作的方法(例如
put
,get
,remove
,containsKey
,containsValue
,size
和empty
)。
Map<Key, Value> numbers = new HashMap<>();
Map<Key, Value> numbers = new LinkedHashMap<>();
Map<Key, Value> numbers = new TreeMap<>();
地图界面的层次结构 (Hierarchy of the Map Interface)
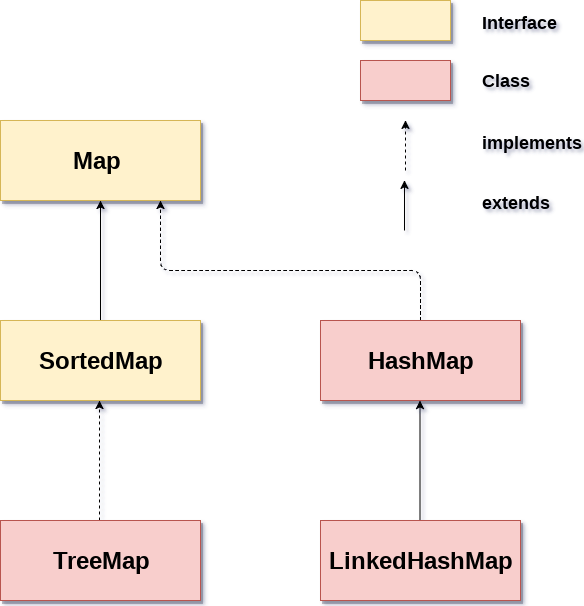
地图界面的属性 (Properties of the Map Interface)
- Given a key and a value we can store the value in a Map object. After the value is stored, you can retrieve it by using its key. 给定键和值,我们可以将值存储在Map对象中。 值存储后,可以使用其键检索它。
Several methods throw a
NoSuchElementException
when no items exist in the invoking map.当调用映射中不存在任何项时,有几种方法会引发
NoSuchElementException
。A
ClassCastException
is thrown when an object is incompatible with the elements in a map.当对象与地图中的元素不兼容时,
ClassCastException
。A
NullPointerException
is thrown if an attempt is made to use a null object and null is not allowed in the map.如果尝试使用null对象并且在映射中不允许null,则抛出
NullPointerException
。An
UnsupportedOperationException
is thrown when an attempt is made to change an unmodifiable map.尝试更改不可修改的映射时,抛出
UnsupportedOperationException
。
哈希图 (HashMap)
Java HashMap class implements the Map interface which allows us to store key and value pairs, where keys should be unique. If you try to insert the duplicate key, it will replace the element of the corresponding key.
Java HashMap类实现了Map接口,该接口允许我们存储键和值对 ,其中键应该是唯一的。 如果尝试插入重复键,它将替换相应键的元素。
HashMap<Key,Value> map1= new HashMap<>();
有关Java哈希图的重要事实 (Important facts about Java Hash Map)
- Contains values based on the key. 包含基于键的值。
- Contains only unique keys. 仅包含唯一键。
- It may have one null key and multiple null values. 它可能具有一个null键和多个null值。
It maintains no order.
它不维护任何顺序。
Let’s go through the following example to get a clear idea of Hash Maps.
让我们来看下面的示例,以清楚地了解Hash Maps。
import java.util.*;
public class HashMp {
public static void main(String[] args) {
// Creating a map using the HashMap
HashMap<String, Integer> numbers = new HashMap<>();
// Insert elements to the map
numbers.put("One", 1);
numbers.put("Two", 2);
numbers.put("Three", 3);
numbers.put("Four", 4);
numbers.put("Five", 5);
System.out.println("Map: " + numbers);
// Access keys of the map
System.out.println("Keys: " + numbers.keySet());
// Access values of the map
System.out.println("Values: " + numbers.values());
// Access entries of the map
System.out.println("Entries: " + numbers.entrySet());
// Get an iterator
Iterator itr = numbers.entrySet().iterator();
while (itr.hasNext()) {
Map.Entry pair = (Map.Entry)itr.next();
System.out.println(pair.getKey() + " => " + pair.getValue());
}
// Remove Elements from the map
int value = numbers.remove("Two");
System.out.println("Removed Value: " + value);
System.out.println("Entries: " + numbers.entrySet());
}
}
/*OUTPUT
Map: {Five=5, One=1, Four=4, Two=2, Three=3}
Keys: [Five, One, Four, Two, Three]
Values: [5, 1, 4, 2, 3]
Entries: [Five=5, One=1, Four=4, Two=2, Three=3]
Five => 5
One => 1
Four => 4
Two => 2
Three => 3
Removed Value: 2
Entries: [Five=5, One=1, Four=4, Three=3]
*/
LinkedHashMap (LinkedHashMap)
Java LinkedHashMap class is Hashtable and Linked list implementation of the Map interface, with predictable iteration order. It inherits the HashMap class and implements the Map interface.
Java LinkedHashMap类是Map接口的Hashtable和Linked list实现,具有可预测的迭代顺序。 它继承了HashMap类并实现了Map接口。
LinkedHashMap<Key,Value> map1= new LinkedHashMap<>();
有关Java LinkedHash Map的重要事实 (Important facts about Java LinkedHash Map)
- Contains values based on the key. 包含基于键的值。
- Contains unique elements. 包含独特的元素。
- May have one null key and multiple null values. 可能有一个null键和多个null值。
Maintains insertion order.
保持插入顺序。
Let’s go through the following example to get a clear idea of LinkedHash Maps.
让我们来看下面的示例,以清楚了解LinkedHash Maps。
import java.util.*;
public class LinkedHMap {
public static void main(String args[]) {
LinkedHashMap<String, Integer> map=new LinkedHashMap<String, Integer>();
// Insert elements to the map
map.put("One", 1);
map.put("Two", 2);
map.put("Three", 3);
map.put("Four", 4);
map.put("Five", 5);
map.put(null,6);
map.put("Seven",null);
map.put("Eight",null);
System.out.println("Map: " + map);
// Access keys of the map
System.out.println("Keys: " + map.keySet());
// Access values of the map
System.out.println("Values: " + map.values());
// Access entries of the map
System.out.println("Entries: " + map.entrySet());
// Get an iterator
Iterator itr = map.entrySet().iterator();
while (itr.hasNext()) {
Map.Entry pair = (Map.Entry)itr.next();
System.out.println(pair.getKey() + " => " + pair.getValue());
}
// Remove Elements from the map
int value = map.remove("Two");
System.out.println("Removed Value: " + value);
System.out.println("Entries: " + map.entrySet());
}
}
/*OUTPUT
Map: {One=1, Two=2, Three=3, Four=4, Five=5, null=6, Seven=null, Eight=null}
Keys: [One, Two, Three, Four, Five, null, Seven, Eight]
Values: [1, 2, 3, 4, 5, 6, null, null]
Entries: [One=1, Two=2, Three=3, Four=4, Five=5, null=6, Seven=null, Eight=null]
One => 1
Two => 2
Three => 3
Four => 4
Five => 5
null => 6
Seven => null
Eight => null
Removed Value: 2
Entries: [One=1, Three=3, Four=4, Five=5, null=6, Seven=null, Eight=null]
*/
树状图 (TreeMap)
Java TreeMap class is a red-black tree-based implementation. It implements the NavigableMap interface and extends AbstractMap class. It provides an efficient means of storing key-value pairs in sorted order.
Java TreeMap类是基于红黑树的实现 。 它实现了NavigableMap接口并扩展了AbstractMap类。 它提供了一种有效的方式来按排序顺序存储键值对。
TreeMap<Key,Value> map1= new TreeMap<>();
有关Java TreeMap的重要事实 (Important facts about Java TreeMap)
- Contains values based on the key. 包含基于键的值。
- Contains only unique elements. 仅包含唯一元素。
Cannot have a null key but can have multiple null values.
不能有一个空键,但可以有多个空值。
Maintains an ascending order.
保持升序。
Let’s go through the following example to get a clear idea of TreeMaps.
让我们来看下面的示例,以清楚了解TreeMaps。
import java.util.*;
public class TreeMp {
public static void main(String[] args) {
// Creating Map using TreeMap
TreeMap<String, Integer> values = new TreeMap<>();
// Insert elements to map
values.put("Second", 24);
values.put("First", 16);
values.put("Fourth", 23);
values.put("Third", 11);
values.put("Fifth", 52);
System.out.println("Map using TreeMap: " + values);
// Replacing the values
values.replace("Third", 3);
values.replace("First", 1);
values.replace("Fourth", 4);
values.replace("Second", 2);
values.replace("Fifth", 5);
System.out.println("New Map: " + values);
// Remove elements from the map
int removedValue = values.remove("First");
System.out.println("Removed Value: " + removedValue);
System.out.println("New Map after removed element: " + values);
}
}
/*OUTPUT
Map using TreeMap: {Fifth=52, First=16, Fourth=23, Second=24, Third=11}
New Map: {Fifth=5, First=1, Fourth=4, Second=2, Third=3}
Removed Value: 1
New Map after removed element: {Fifth=5, Fourth=4, Second=2, Third=3}
*/
摘要 (Summary)
At the end of this article, we learned the basic concepts of Java Map Interface Also, we explored the advantages of the Map Interface in Java. Hope this article will help you to understand these concepts. Let’s meet with another interesting article in the near future.
在本文的最后,我们学习了Java Map Interface的基本概念。此外,我们还探索了Java中Map Interface的优势。 希望本文能帮助您理解这些概念。 让我们在不久的将来见到另一篇有趣的文章。
Thank you!
谢谢!
Happy Learning 🙌😊
快乐学习🙌😊
翻译自: https://levelup.gitconnected.com/lets-learn-java-map-interface-a9a3f191e557