页面置换算法最佳置换算法
This week I have been doing a lot of algorithm questions in binarysearch.io. For some of you who don’t know binarysearch.io, It is a new platform where you can collaborate with strangers or friends across the country to do algorithm questions together. It is more fun and engaging to do algorithms together than to do it alone. At the same time, you can improve your pair programming skills and help out other debugging codes. If you are still unsure what the difference is between bs .io than any other coding platform, think of Slack and Leetcode having a baby.
本周,我在binarysearch.io中做了很多算法问题。 对于某些不了解binarysearch.io的人来说,这是一个新平台,您可以在其中与全国各地的陌生人或朋友合作,共同解决算法问题。 一起做算法比单独做算法更有趣和更吸引人。 同时,您可以提高结对编程技能并帮助其他调试代码。 如果您仍然不确定bs .io与其他任何编码平台之间有什么区别,请考虑Slack和Leetcode有一个婴儿。
Okay, all joking aside, I am here to talk about best practice for solving algorithms. I found this best practice when I was answering an easy question in bs.io. So here is the question:
好吧,除了开个玩笑,我在这里谈论解决算法的最佳实践。 当我在bs.io中回答一个简单的问题时,我发现了这种最佳实践。 所以这是一个问题 :

了解问题 (Understand the Problem)
Before you try to solve any algorithm question, it is okay not to write code for the first 5–7 mins. Try to understand the problem in the first place. In this example: the question is asking to delete last occurrences for the number of the duplicate. The highlighted words here are duplicates and last occurrences, so as long as there are the same numbers in the array, all we need to do is to delete the last occurrences of that same number in the array.
在尝试解决任何算法问题之前,最好不要在前5到7分钟内编写代码。 首先尝试理解问题。 在此示例中:问题是要求删除重复 出现次数为的最后一次出现 。 此处突出显示的词是重复项和最后出现的词,因此只要数组中存在相同的数字,我们要做的就是删除数组中相同数字的最后出现。
计划 (Plan)
- Plan on how you will solve the question, pseudocode it first if that makes your life easier. We need to worry about any constraints along the way, apply what kind of data structure to solve the problem, different ideas/perspectives to solve the problem such as looping from the back, etc. 计划如何解决问题,如果这样可以使您的生活更轻松,请首先对其进行伪编码。 我们需要担心过程中的任何约束,应用什么样的数据结构来解决问题,使用不同的想法/观点来解决问题,例如从背面循环等等。
- Since we need to keep track of all the duplicate numbers in the array, we can utilize a hash table to track the number frequency. Once we get each of the number frequencies, we can start looping from the back of the array by deleting the last occurrences duplicate number with the hash references. 由于我们需要跟踪数组中所有重复的数字,因此我们可以利用哈希表来跟踪数字频率。 一旦获得每个数字频率,就可以通过删除带有哈希引用的最后出现的重复数字,从数组的背面开始循环。
行动 (Action)
- Now we start writing code and use the plan to approach the problem. One thing to keep in mind, It is okay to solve the question in a brute force way first or not in the best optimal approach yet. Sometimes it is easier to address the problem first, and you can always reverse engineering later to optimize your solution. 现在,我们开始编写代码,并使用该计划解决问题。 要记住的一件事是,可以首先采用蛮力方式解决问题,也可以不采用最佳方法来解决问题。 有时,首先解决问题更容易,并且以后总是可以进行逆向工程以优化解决方案。
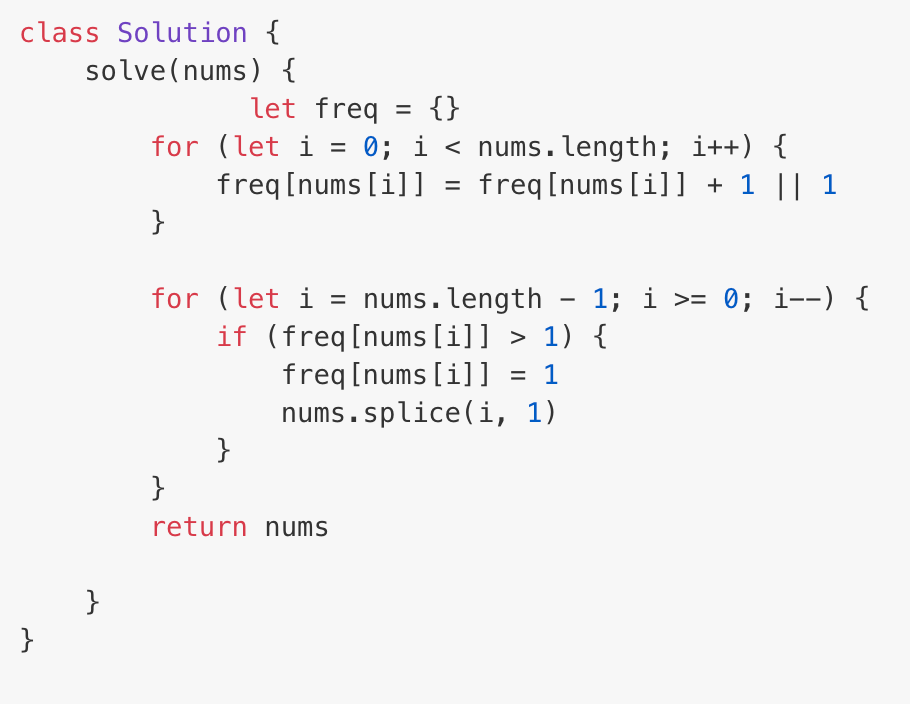
遍历 (Iterate through)
- Before you run the test cases or submit button, make sure you understand what your codes are doing. What value are we returning? Do we hit all the requirements? Any other additional constraints that we need to think of? Iterate your codes once again to understand the bigger picture. 在运行测试用例或提交按钮之前,请确保您了解代码在做什么。 我们返回什么价值? 我们达到所有要求了吗? 我们需要考虑其他任何其他约束吗? 再次迭代您的代码以了解大局。
恭喜,您解决了问题! 怎么办? (Congrats, you solve the problem! Now what?)
- This practice is a crucial step; now, we can reverse engineering to find a better optimal solution. 这种做法是至关重要的一步; 现在,我们可以进行逆向工程以找到更好的最佳解决方案。
- The built-in .splice() method might make the time complexity O(n²) with for loop. 内置的.splice()方法可能会使for循环的时间复杂度为O(n²)。
- Let’s try a different approach with two hash tables and avoid the splice method. 让我们尝试使用具有两个哈希表的另一种方法,并避免使用splice方法。
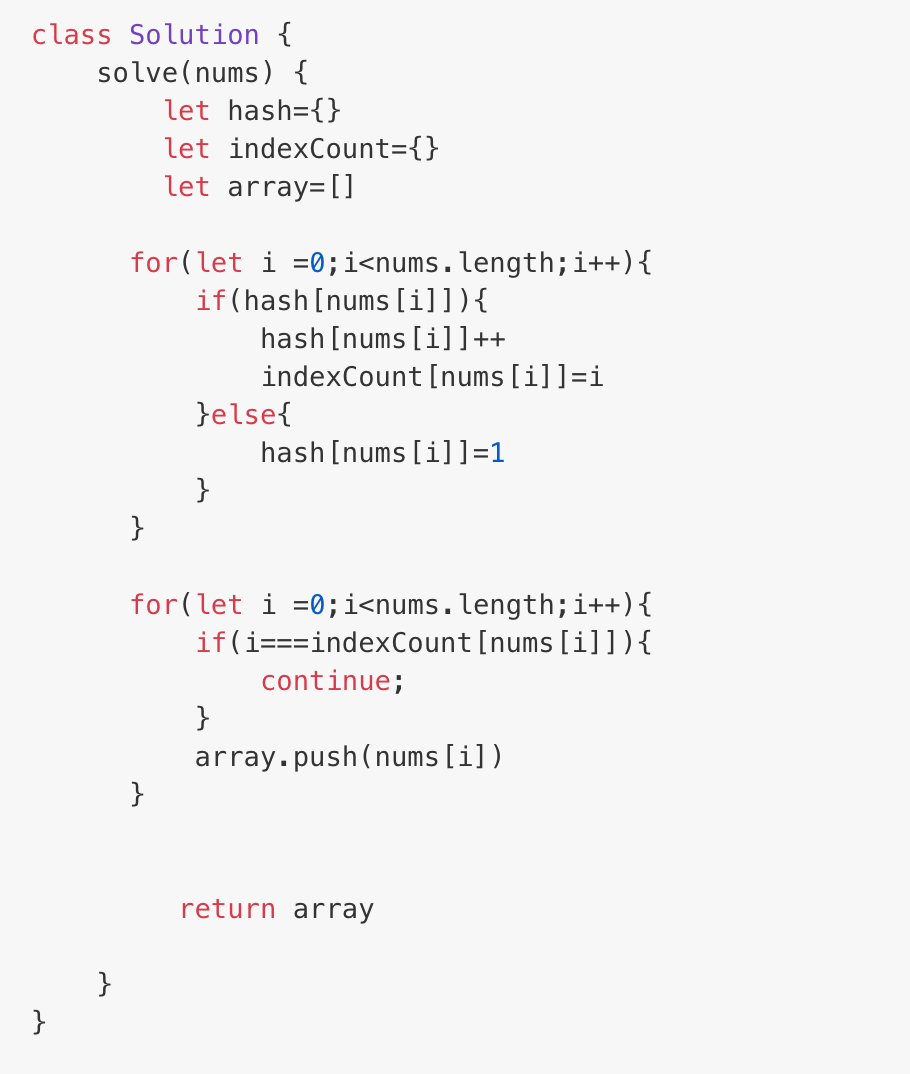
The first hash table would be the same as the previous approach, getting all the frequency numbers in the array. The other hash table indexCount={} keeps track of the duplicates number index of the array. From there, we know the last index occurrence for the duplicate numbers. Lastly, we can create an array (array=[]), loop through the original nums array, and if those duplicates number indexes of the array are not met(in indexCount={}) push the number into the new array we just created.
第一个哈希表将与以前的方法相同,获得数组中的所有频率编号。 另一个哈希表indexCount = {}跟踪数组的重复编号索引。 从那里,我们知道重复编号的最后一次索引出现。 最后,我们可以创建一个数组(array = []),循环遍历原始的nums数组,如果这些重复数 不满足数组的索引(在indexCount = {}中)将数字推入我们刚刚创建的新数组中。
- As you can see, here the time complexity is O(n) instead of O(n²) 如您所见,这里的时间复杂度是O(n)而不是O(n²)
摘要 (Summary)
- Try to understand the question first before writing out any code. Take your time to understand what the problem is asking. 编写任何代码之前,请先尝试理解问题。 花点时间了解问题所在。
- Check to see if you can find any pattern, constraint, and different data structures to solve the question. 检查是否可以找到任何模式,约束和不同的数据结构来解决该问题。
- Solve the question first with brute force if you think it makes your life easier and reverse engineering later. 如果您认为这可以使您的生活更轻松,请先用蛮力解决问题,然后再进行逆向工程。
- Before submitting codes, make sure you hit all the constraints that the question asks, run through your thought process of each line of your codes again, check if you hit all the goals that the questions ask. 在提交代码之前,请确保您达到问题所要求的所有限制,再次遍历代码每一行的思考过程,检查是否达到问题所要达到的所有目标。
- One of the best and essential practices to scale your algorithm skill is to solve the problem, optimize your solution, or use different approaches to address the question. You can use different data structures or other programming languages to solve. Perhaps, you can see other people’s solutions, and discover a new way of approaching the problem. There is nothing to be ashamed of after optimizing your solution and look at other people’s answers on how they approach the problem, as long as you learn something new. 扩展算法技能的最佳和必要实践之一是解决问题,优化解决方案或使用其他方法来解决问题。 您可以使用不同的数据结构或其他编程语言来解决。 也许,您可以看到其他人的解决方案,并找到解决问题的新方法。 优化您的解决方案并查看其他人关于他们如何解决问题的答案后,只要您学到了新的东西,就不会感到羞耻。
Thanks for taking the time to read, and I hope this post can help the way you practice your problem-solving skills. If you want to practice algorithm questions together, don’t be afraid to add me(@the_goodone) in binarysearch.io!
感谢您抽出宝贵的时间阅读本文,希望这篇文章对您练习解决问题的能力有所帮助。 如果您想一起练习算法问题,请不要害怕在binarysearch.io中加我(@the_goodone)!
翻译自: https://medium.com/the-innovation/best-practice-of-solving-algorithms-a2759b8d690
页面置换算法最佳置换算法