ios swift请求框架
We all use a lot of frameworks in our daily development routine. We just type the magical word “import” and it’s all set. But what’s happening behind that import statement? How do you make it possible for your fellow developers to use your classes with just one line of code? Today, I’ll try to show you how to create a binary framework.
在我们的日常开发例程中,我们所有人都使用很多框架。 我们只需要输入神奇的单词“ import”就可以了。 但是该import语句后面发生了什么? 您如何使同等开发人员仅用一行代码即可使用您的类? 今天,我将尝试向您展示如何创建一个二进制框架。
First, it’s better to understand the difference between framework and library, a subject that we commonly come across in iOS developer interviews.
首先,最好了解框架和库之间的区别,这是我们在iOS开发人员访谈中经常遇到的主题。
Library is a file that defines bunch of code; not part of your original target. A library can be dynamic (.dylib) or static (.a)
库是定义一堆代码的文件; 不属于您最初的目标。 库可以是动态(.dylib)或静态(.a)
As you can understand from their names, static libraries are compiled once so the file size can be bigger.
从它们的名称可以理解,静态库只编译一次,因此文件大小可以更大。
Dynamic libraries, on the other hand, are loaded into memory when they are needed. This can be loadtime or runtime. All Apple system libraries are dynamic.
另一方面,动态库在需要时被加载到内存中。 这可以是加载时间或运行时。 所有Apple系统库都是动态的。
A framework is a package that contains resources like dynamic libraries, images, interface builder files, headers etc. It is basically a bundle that ends with .framework extension.
框架是一个包含动态库,图像,界面生成器文件,标头等资源的包。它基本上是一个以.framework扩展名结尾的捆绑包。
We will cover frameworks in this article.
我们将在本文中介绍框架。
Let’s dive into Xcode and see how to create one.
让我们深入研究Xcode,看看如何创建一个。
First, we need to choose Xcode -> New project -> Framework.
首先,我们需要选择Xcode-> New project-> Framework。
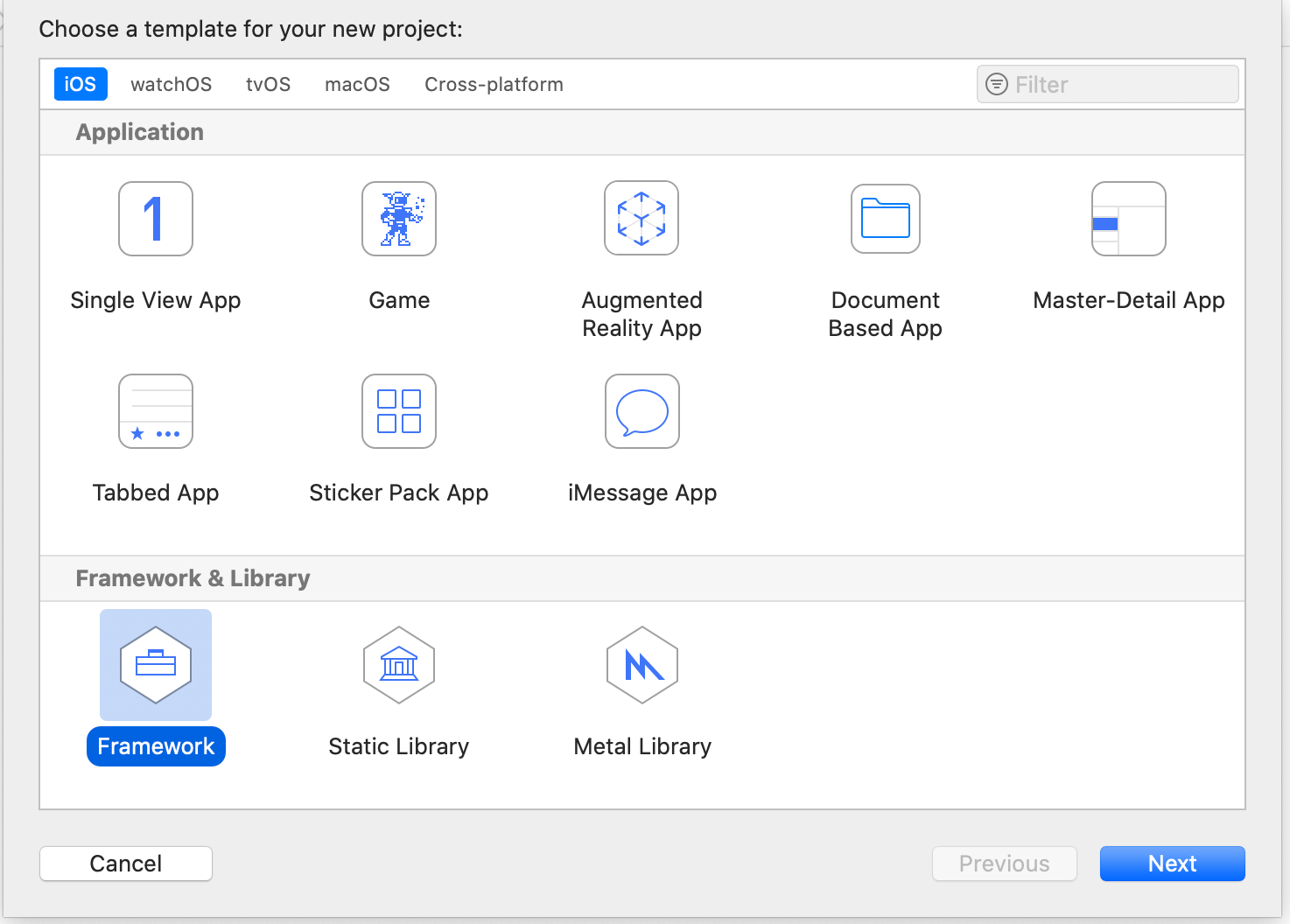
In this demo, we name our project VfkFrameworkDemo. It will be the name of our framework too.
在此演示中,我们将项目命名为VfkFrameworkDemo。 这也将是我们框架的名称。
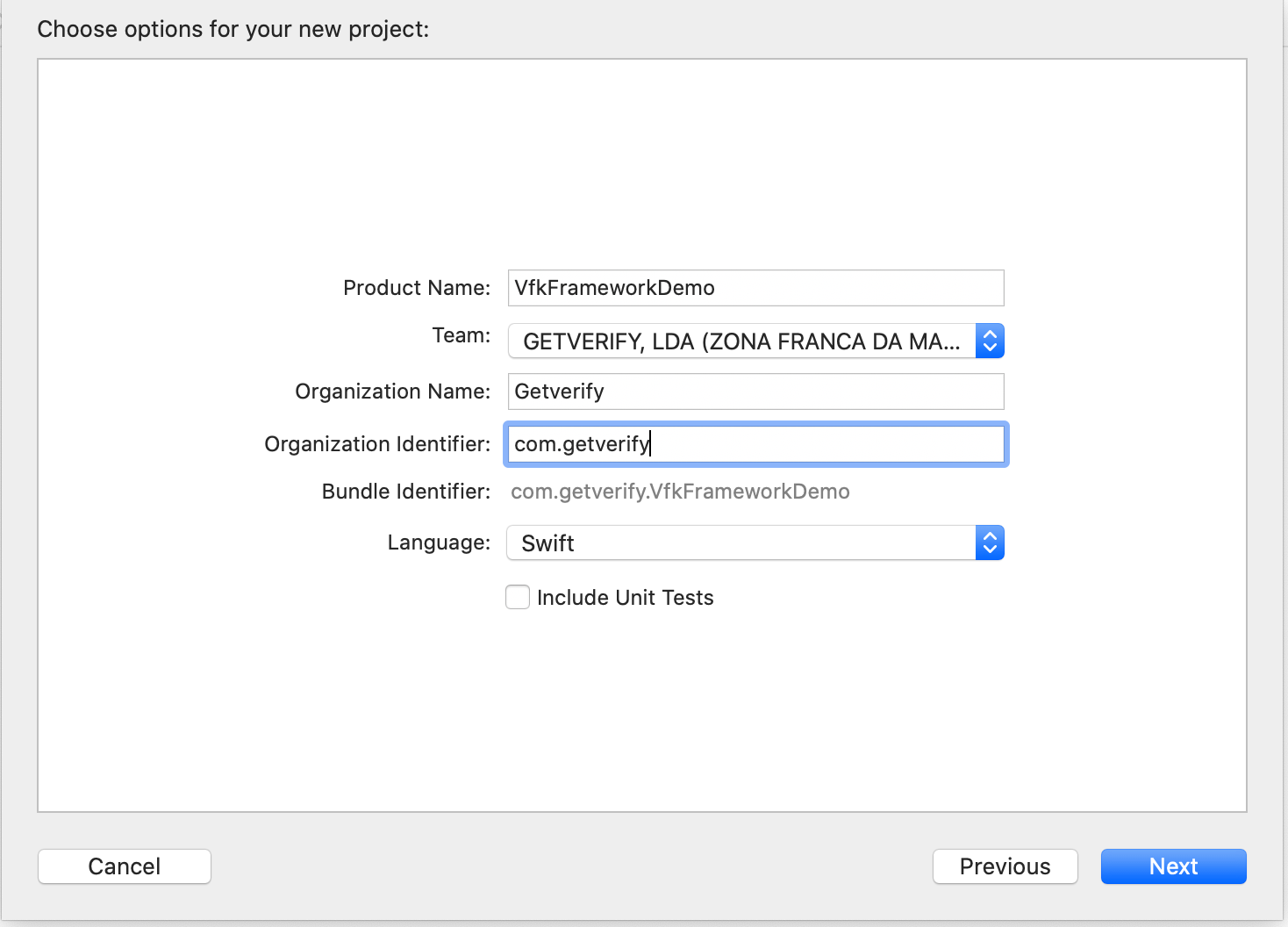
After creating a new framework project, all we see in our bundle is a single .h file.
创建新的框架项目后,我们在捆绑包中看到的只是一个.h文件。
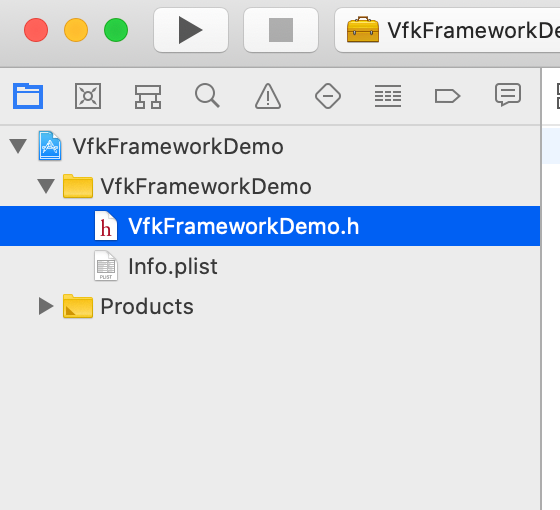
We can leave that .h file as it is, we won’t be needing it after.
我们可以保留该.h文件原样,以后将不再需要它。
Let’s create a new swift file, VfkFrameworkLogger.swift (filename doesn’t matter really) and add the code below.
让我们创建一个新的swift文件VfkFrameworkLogger.swift (文件名并不重要)并添加以下代码。
import Foundation
public class VfkFrameworkDemo {
public init() {
debugPrint("VfkFrameworkDemo initialized.")
}
}
Important side note;Classes and functions which will be used in integration must be marked as public.You can still keep your internal classes; they won’t be available to developer who uses your framework.You can still keep your internal classes; they won’t be available to the developer who uses your framework.That’s the beauty of binary frameworks; people can use your code in background, but they can’t see what you don’t want them to see. Privacy matters.
重要的旁注;将在集成中使用的类和函数必须标记为public 。您仍然可以保留内部类; 使用您的框架的开发人员将无法使用它们。您仍然可以保留内部类; 使用您的框架的开发人员将无法使用它们。 人们可以在后台使用您的代码,但看不到您不希望他们看到的内容。 隐私很重要。
If we select “Generic iOS Device” from device list and build project, we see a file named VfkFrameworkDemo.framework in Bundle, under Products folder.
如果从设备列表中选择“通用iOS设备”并构建项目,则会在“产品”文件夹下的捆绑包中看到一个名为VfkFrameworkDemo.framework的文件。
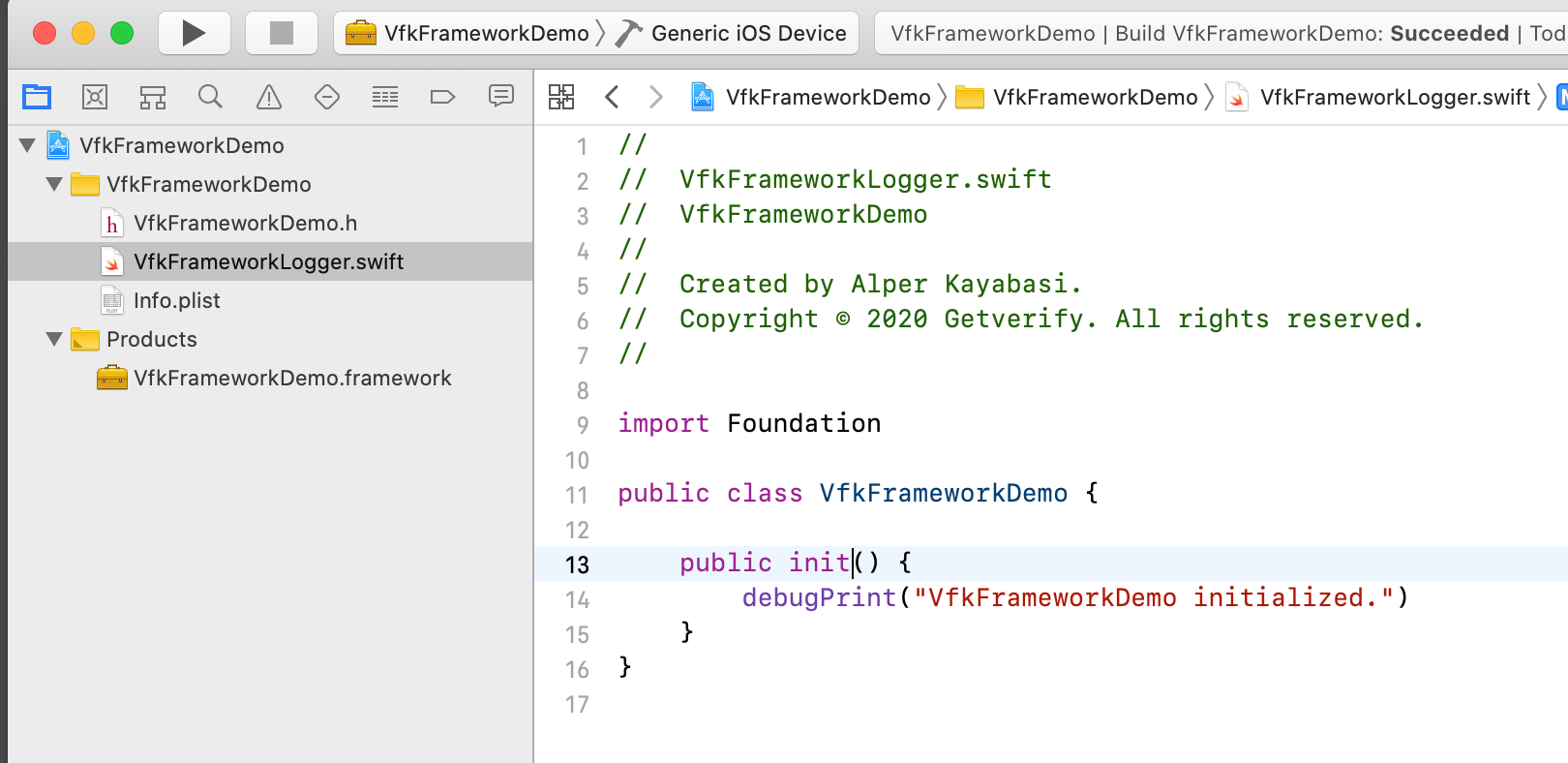
This is our first framework, now we can use it with drag and drop.Well, that was simple. Are we done? Not quite.
这是我们的第一个框架,现在我们可以拖放使用它了,那很简单。 我们完了吗? 不完全的。
The first problem we’ll encounter here is “build targets.” We built our framework for “Generic iOS Device”, that means our framework can only be used on real devices.That’s a bummer. A lot of iOS developers use simulators. What should we do?
我们在这里遇到的第一个问题是“构建目标”。 我们为“通用iOS设备”构建了框架,这意味着我们的框架只能在真实设备上使用。 许多iOS开发人员都使用模拟器。 我们应该做什么?
Let’s change our build target to “iPhone 8 simulator” and build again.
让我们将构建目标更改为“ iPhone 8 Simulator”,然后重新构建。
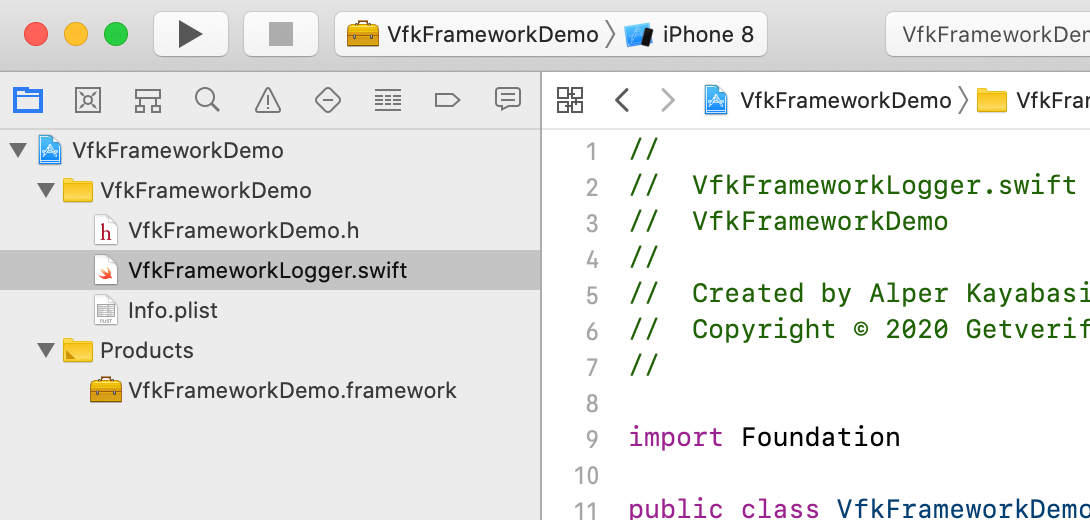
We did it, now our framework can be used on a simulator. But wait…We still need “real devices” too.
我们做到了,现在我们的框架可以在模拟器上使用了。 但是等等……我们仍然需要“真实设备”。
Fortunately, there is a way to combine these two frameworks and create a universal one which will work both on device and simulator.This workaround is generally referred as “aggregate target” and it’s a simple way to create universal framework in Xcode, without the need of using Terminal. (We can still use terminal for this process btw.)
幸运的是,有一种方法可以将这两个框架组合在一起,并创建一个可以在设备和模拟器上运行的通用框架。这种解决方法通常称为“聚合目标”,它是在Xcode中创建通用框架的简单方法,无需使用使用终端。 (顺便说一下,我们仍然可以将终端用于此过程。)
Let’s create a new target with File -> New -> Target -> Aggregate.For the sake of understanding this process, we name our new target “Aggregate”.
让我们通过File-> New-> Target-> Aggregate创建一个新目标。为了理解此过程,我们将新目标命名为“ Aggregate”。
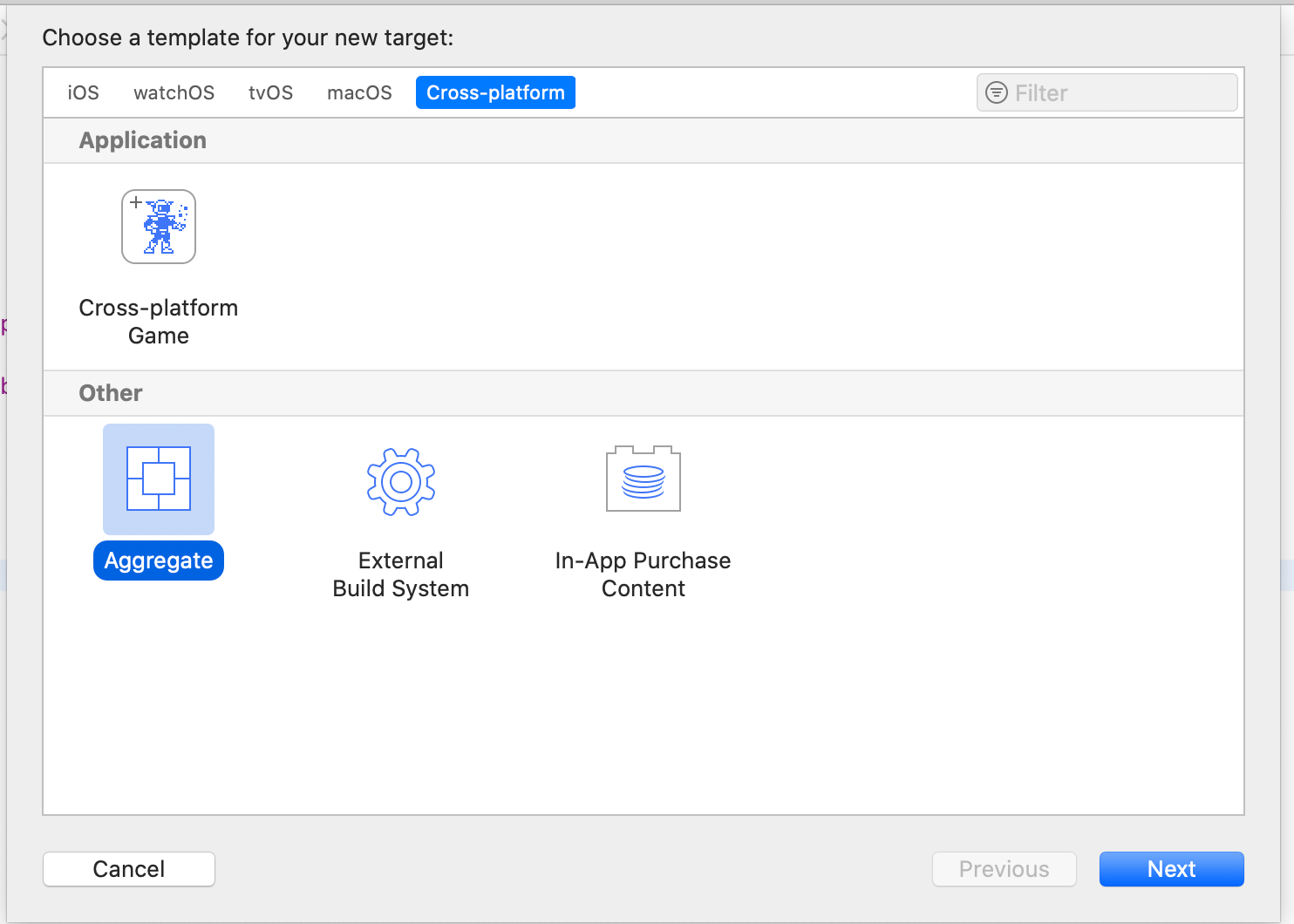
After creating our new target, we need to switch to “Aggregate”, go to “Build Phases”, and create a new “Run Script”
创建新目标后,我们需要切换到“聚合”,转到“构建阶段”,然后创建一个新的“运行脚本”
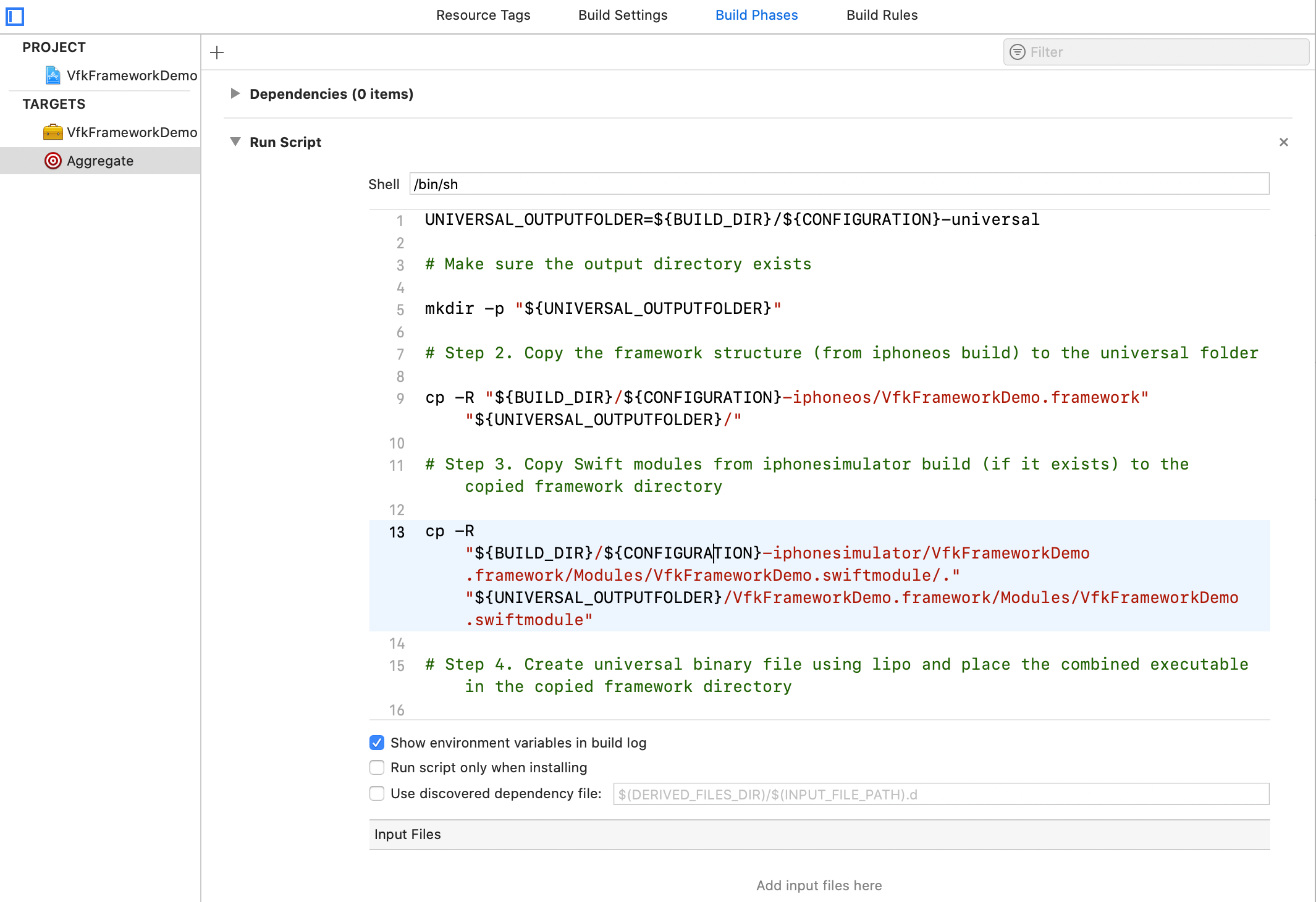
This is the code snippet we’ll use in the script.
这是我们将在脚本中使用的代码片段。
UNIVERSAL_OUTPUTFOLDER=${BUILD_DIR}/${CONFIGURATION}-universal
# Make sure the output directory exists
mkdir -p "${UNIVERSAL_OUTPUTFOLDER}"
# Step 2. Copy the framework structure (from iphoneos build) to the universal folder
cp -R "${BUILD_DIR}/${CONFIGURATION}-iphoneos/VfkFrameworkDemo.framework" "${UNIVERSAL_OUTPUTFOLDER}/"
# Step 3. Copy Swift modules from iphonesimulator build (if it exists) to the copied framework directory
cp -R "${BUILD_DIR}/${CONFIGURATION}-iphonesimulator/VfkFrameworkDemo.framework/Modules/VfkFrameworkDemo.swiftmodule/." "${UNIVERSAL_OUTPUTFOLDER}/VfkFrameworkDemo.framework/Modules/VfkFrameworkDemo.swiftmodule"
# Step 4. Create universal binary file using lipo and place the combined executable in the copied framework directory
lipo -create -output "${UNIVERSAL_OUTPUTFOLDER}/VfkFrameworkDemo.framework/VfkFrameworkDemo" "${BUILD_DIR}/${CONFIGURATION}-iphonesimulator/VfkFrameworkDemo.framework/VfkFrameworkDemo" "${BUILD_DIR}/${CONFIGURATION}-iphoneos/VfkFrameworkDemo.framework/VfkFrameworkDemo"
# Step 5. Convenience step to open the project's directory in Finder
open "${UNIVERSAL_OUTPUTFOLDER}"
The process is simply explained in five steps.First, we need to build our framework for both real device and simulator seperately. After that, the run script will combine these two frameworks into a one universal framework to rule them all.
只需五个步骤即可简单地解释该过程。首先,我们需要分别为真实设备和模拟器构建框架。 之后,运行脚本会将这两个框架组合为一个通用框架,以对它们全部进行统治。
- Choose VfkFrameworkDemo -> Generic iOS Device and build. 选择VfkFrameworkDemo->通用iOS设备并进行构建。
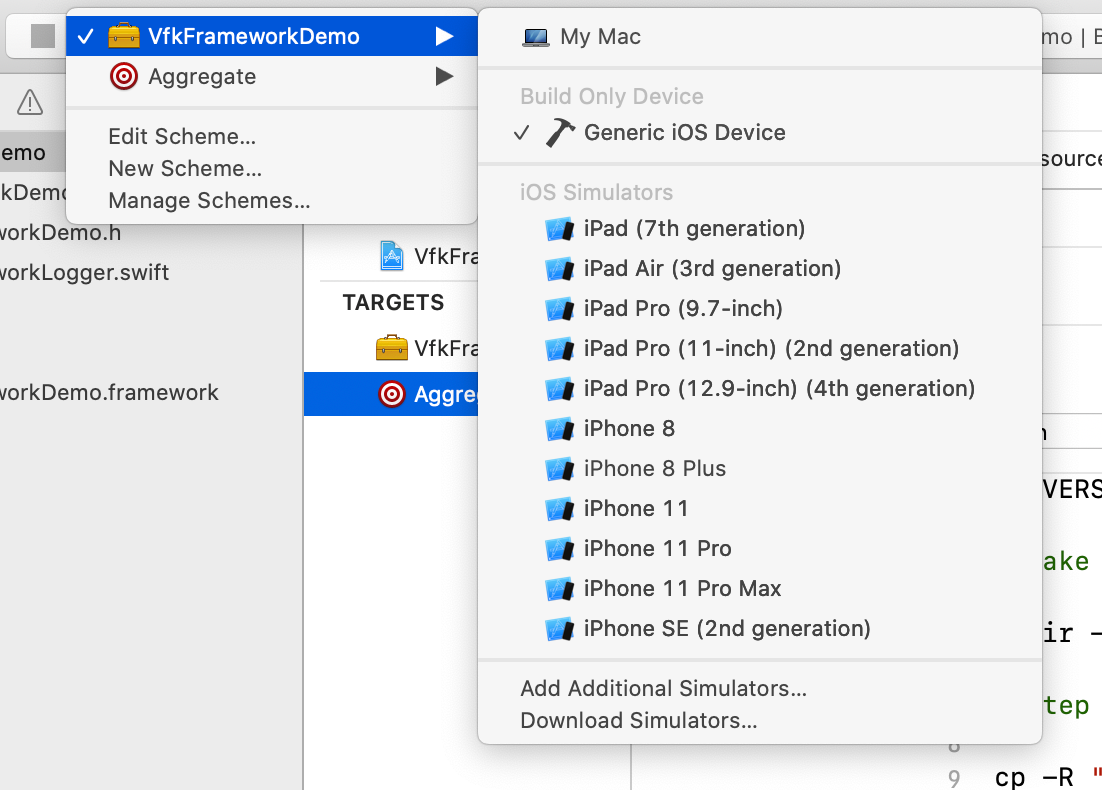
- Choose VfkFrameworkDemo -> iPhone 8 and build again. 选择VfkFrameworkDemo-> iPhone 8并重新构建。
- Choose Aggregate -> Generic iOS Device and build again. 选择Aggregate-> Generic iOS Device并重新构建。
After these steps, Xcode (the run script) opens the finder and shows us our universal framework. This file can be used on both simulator and real devices.
完成这些步骤后,Xcode(运行脚本)将打开查找程序并向我们展示我们的通用框架。 该文件可在模拟器和真实设备上使用。
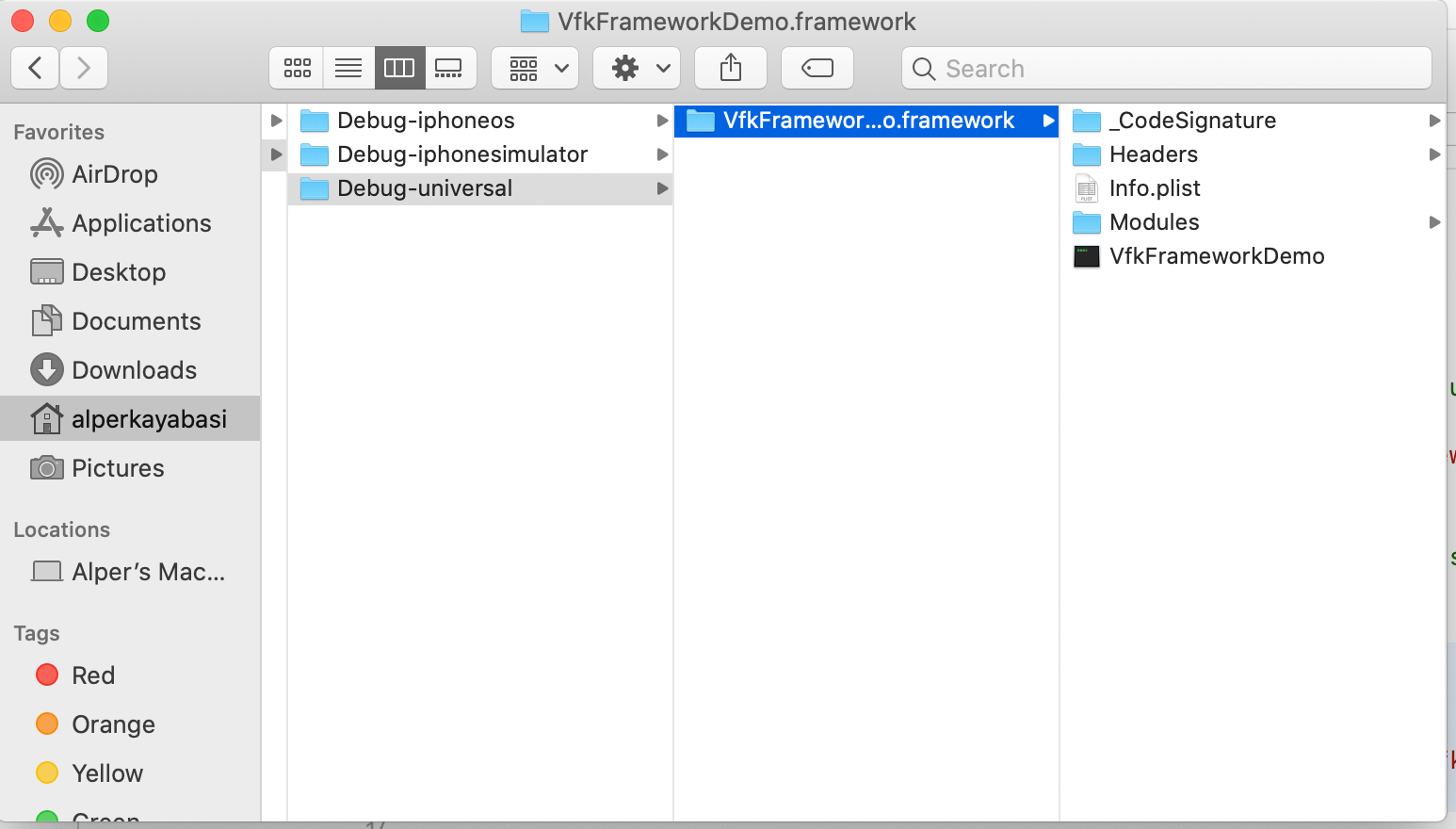
Drag it into Desktop, we’ll use it in a couple of minutes.
将其拖到桌面中,我们将在几分钟后使用它。
Create a new Single-View Xcode project. We don’t need anything special, we just need a clean project to import our framework into.
创建一个新的Single-View Xcode项目。 我们不需要任何特殊的东西,我们只需要一个干净的项目即可将我们的框架导入其中。
Drag VfkFrameworkDemo.framework into the bundle.
将VfkFrameworkDemo.framework拖到包中。
Go to project’s General settings, select Embed & Sign from Frameworks, Libraries and Embedded Content menu.
转到项目的“常规”设置,从“ 框架”,“库”和“嵌入式内容”菜单中选择“ 嵌入和签名” 。
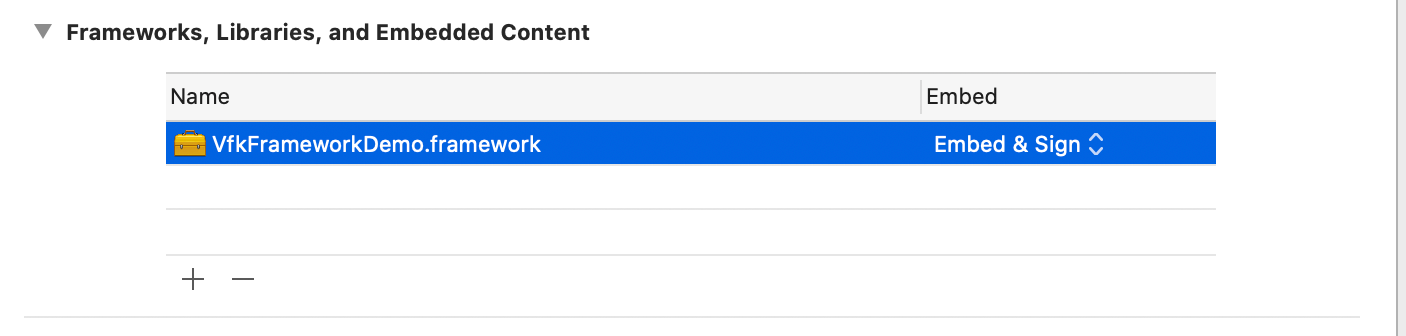
This step is not important at this time but it must be done to use the framework on a real device.
目前,此步骤并不重要,但必须完成此步骤才能在实际设备上使用该框架。
This step is not important at this time but it must be done to use the framework on a real device.PS: If you distribute your framework with Cocoapods, it takes care of that signing issue on its own.
目前,此步骤并不重要,但必须完成此步骤才能在实际设备上使用该框架。 PS:如果您使用Cocoapods分发框架,它将独自解决该签名问题。
This is what our new project looks like.
这就是我们的新项目的样子。
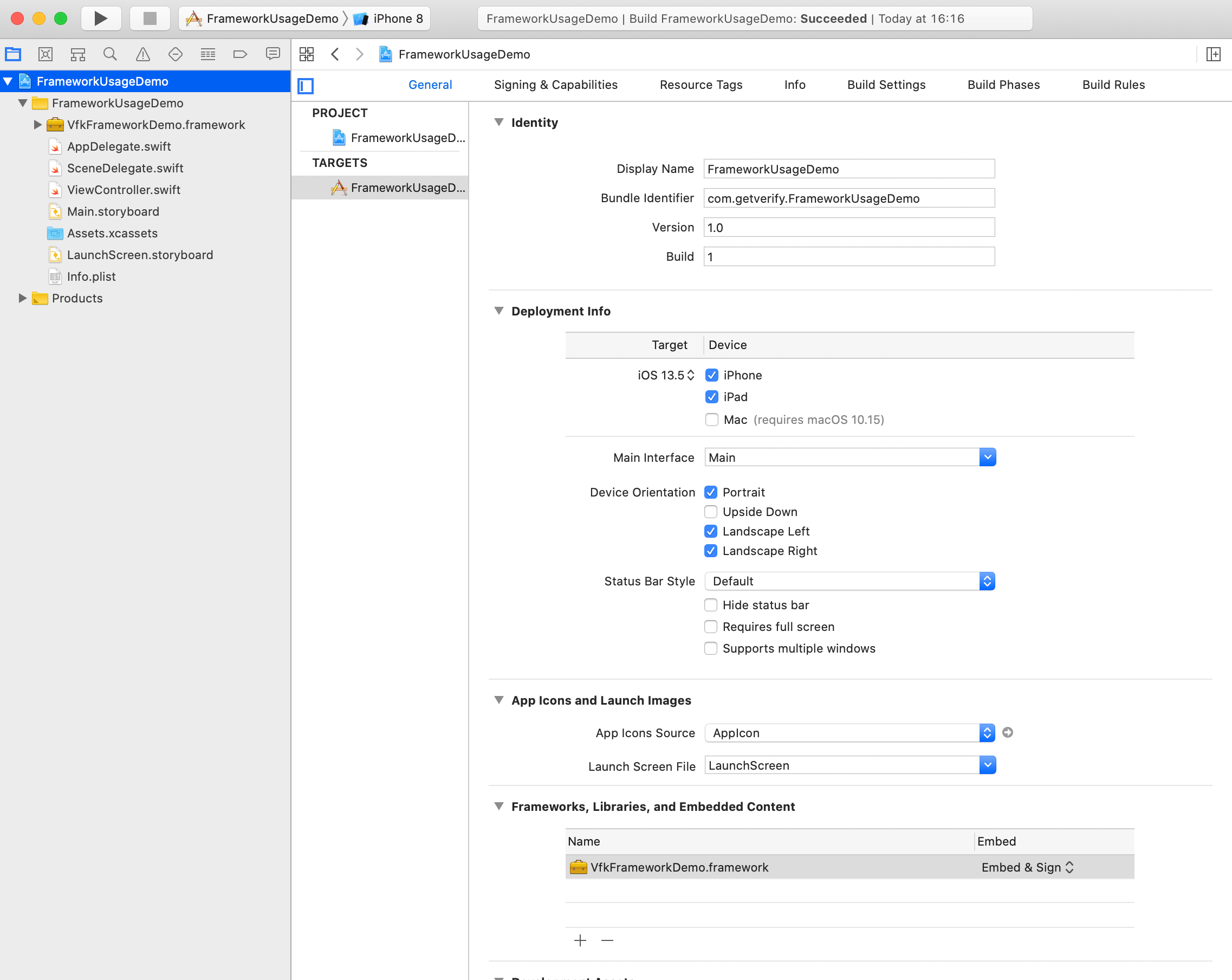
Open ViewController.swift and import VfkFrameworkDemo
打开ViewController.swift并导入VfkFrameworkDemo
- Add the framework’s init code into ViewDidLoad. 将框架的初始化代码添加到ViewDidLoad中。
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view.
_ = VfkFrameworkDemo.init()
}
This is our ViewController.swift file. Now Build and Run the project and have a look at debug console.
这是我们的ViewController.swift文件。 现在构建并运行项目,并查看调试控制台。
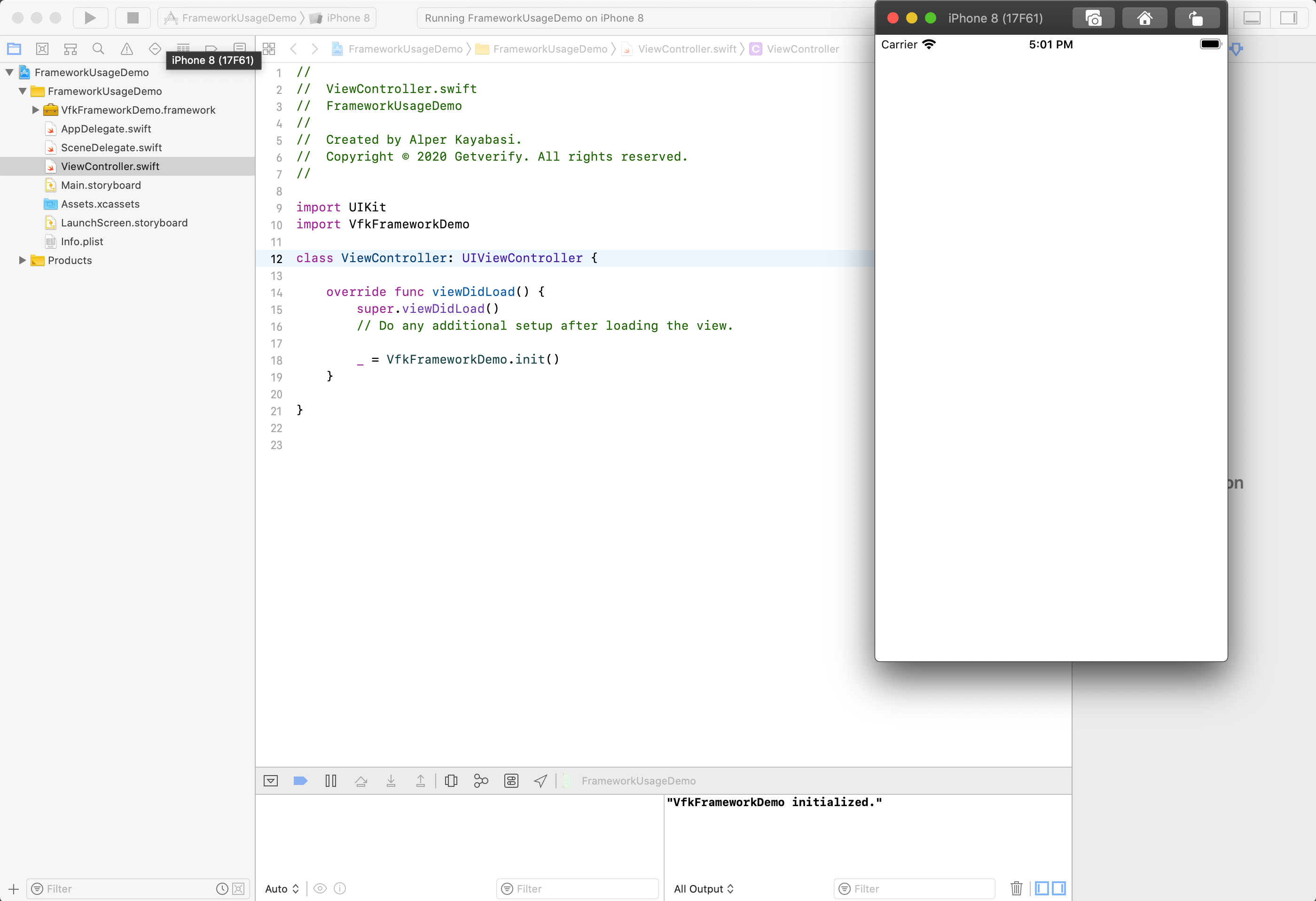
It worked! We just initialized our framework and used it from another project.
有效! 我们只是初始化了我们的框架,并在另一个项目中使用了它。
We didn’t see the framework’s code. We don’t know what happened in the background. With just a single line of code, the framework did its job −in this case, just a debug print− but it can be much more.
我们没有看到框架的代码。 我们不知道背景发生了什么。 仅用一行代码,框架就完成了它的工作-在这种情况下,只是调试打印-但它可以做的更多。
The ABI Stability came with Swift 5.1. That means a binary framework built with Xcode 10.2 or later can now be used on other Swift versions, too. Before that, a framework developer needed to build a new version of a framework for every Swift version. That was a real struggle but now it’s gone, kudos to Apple.
Swift 5.1附带了ABI稳定性 。 这意味着使用Xcode 10.2或更高版本构建的二进制框架现在也可以在其他Swift版本上使用。 在此之前,框架开发人员需要为每个Swift版本构建一个新版本的框架。 那是一次真正的斗争,但现在已经不复存在了,对苹果公司而言是赞誉。
When creating VerifyKit, our development team came across with a strange bug. We can address this as “The corrupted .swiftinterface bug”.
在创建VerifyKit时,我们的开发团队遇到了一个奇怪的错误。 我们可以将其称为“损坏的.swiftinterface错误” 。
We could use our framework on Macbook which built the .framework file without an issue, but when other developer tried to use it on another computer, Xcode gave the error “Failed to load module VfkFrameworkDemo”. (in our case, module name was VerifyKit)
我们可以在Macbook上使用构建.framework文件的框架而没有问题,但是当其他开发人员尝试在另一台计算机上使用它时,Xcode给出了错误“无法加载模块VfkFrameworkDemo” 。 (在我们的示例中,模块名称为VerifyKit)
We realized that Xcode created a corrupted .swiftinterface file and added our module name before every public class.To fix this, you need to go framework’s folder in Terminal and run this line of code.
我们意识到Xcode创建了一个损坏的.swiftinterface文件,并在每个公共类之前添加了我们的模块名称。要解决此问题,您需要在Terminal中进入框架的文件夹并运行以下代码行。
find . -name "*.swiftinterface" -exec sed -i -e 's/VfkFrameworkDemo\.//g' {} \;
This code snippet removes redundant module names (you need to replace VfkFrameworkDemo with your framework module name) in public class definitions, so everyone can use your framework.
此代码段在公共类定义中删除了多余的模块名称(您需要用框架模块名称替换VfkFrameworkDemo) ,以便每个人都可以使用您的框架。
I don’t know if this is a common bug but it gave us a real headache, so I wanted to add this as a side note.
我不知道这是否是一个常见的错误,但它给我们带来了很大的麻烦,所以我想补充一点。
That’s it, we just created a universal binary framework in Swift and used it from another project.We did it with the classic drag&drop, but there are other ways to distribute your framework, like Cocoapods or Carthage.
就是这样,我们刚刚在Swift中创建了一个通用的二进制框架并从另一个项目中使用了它,我们通过经典的拖放操作完成了它,但是还有其他分发框架的方法,例如Cocoapods或Carthage。
As you know, most common way to distribute your framework is Cocoapods. But unfortunately, it’s a subject for our another article.
如您所知,分发框架的最常见方法是Cocoapods。 但不幸的是,这是我们另一篇文章的主题。
Please feel free to comment if you have any questions.
如有任何疑问,请随时发表评论。
Don’t forget to check VerifyKit out!
不要忘记签出VerifyKit !
Happy coding.
快乐的编码。
翻译自: https://medium.com/verifykit/creating-a-binary-framework-in-ios-with-swift-29d1b4a03469
ios swift请求框架