golang测试
If you come from other programming language, you might be bracing yourself for a number of modules and libraries. Fortunately with Go, simplicity is one of the core guiding principle. So, when it comes to testing in GO, we are provided with full-featured testing and profiling tools. That means, when you install GO in your device, you are also going to get full-featured testing and profiling tools. We are also gonna be presented with complete API for controlling test execution.
如果您来自其他编程语言,则可能需要准备许多模块和库。 幸运的是,对于Go来说,简单是其核心指导原则之一。 因此,在GO中进行测试时,我们将提供功能齐全的测试和性能分析工具。 这意味着,当您在设备中安装GO时,您还将获得功能齐全的测试和性能分析工具。 我们还将获得用于控制测试执行的完整API。
There is a testing package include in standard library and it includes everything we need to start writing our own tests and controlling how hour tests run. In this article, we will be using simple, logical test to determine if our test passed or not.
标准库中包含一个测试包,其中包含我们开始编写自己的测试并控制小时测试的运行方式所需的一切。 在本文中,我们将使用简单的逻辑测试来确定测试是否通过。
What type of Test can we write in Go language?
我们可以用Go语言编写哪种类型的测试?
There are three types of tests that we can perform using Go tooling.
我们可以使用Go工具执行三种类型的测试。
- Test: This is the basic test type. Test type is used for: Unit test, Integration test and End to End test. 测试:这是基本测试类型。 测试类型用于:单元测试,集成测试和端到端测试。
2. Benchmark Test: This test is designed for performance profiling
2.基准测试:此测试旨在进行性能分析
3. Example Test: Example test are primarily used for documentation.
3.示例测试:示例测试主要用于文档。
Testing related package
测试相关包
Go provides us with the parts of standard library that are specifically designed to help us write our test. Some of them are:
Go向我们提供了标准库的各个部分,这些部分专门用于帮助我们编写测试。 他们之中有一些是:
- testing: This is sort of a main package for writing our tests. Almost all of the API for creating our test are included in this package. 测试:这是用于编写测试的主要程序包。 该软件包中包含几乎所有用于创建测试的API。
- testing/quick: This is designed to simplify black- box testing. 测试/快速:这旨在简化黑盒测试。
- testing/iotest: iotest package is the sub package of the testing package. This package contains several readers and writers that are designed to exercise very specific aspects of reader and writer. testing / iotest:iotest软件包是测试软件包的子软件包。 该软件包包含一些读者和作家,旨在锻炼读者和作家非常特定的方面。
- net/http/httptest: Inside net/http package there is a sub package called httptest, which contains very valuable APIs for allowing us to simulate requests that we can into our services, response recorders that we can to record the response out and allows us to set up test servers, which allows us to perform end to end test using real http messages to our end points. net / http / httptest:net / http包中有一个名为httptest的子包,其中包含非常有价值的API,这些API可让我们模拟我们可以进入服务的请求,响应记录器,我们可以记录响应并允许我们设置测试服务器,这使我们能够使用真实的http消息到端点来执行端到端测试。
Naming Conventions
命名约定
We don’t have much flexibility in GO for naming conventions. As the conventions used are not only to help other developers, engineers to identify our test, but they are also used by the tooling to identify where our tests suite are and what other test are contained within that test suite.
GO在命名约定方面没有太多灵活性。 由于使用的约定不仅可以帮助其他开发人员,工程师识别我们的测试,而且工具也可以使用它们来识别测试套件的位置以及该测试套件中包含的其他测试。
///File name main_test.gopackage main ||| package main_testfunc TestFoo(t *testing.T) {
......
.......
}
Now, lets break it through. We should name our file with “_test” suffix i.e. <any_name>_test.go . This is used by testing tool to identify where the test are located. Also, while in production these files are excluded when binary are being created.
现在,让我们突破。 我们应使用“ _test”后缀命名文件,即<any_name> _test.go。 测试工具使用它来识别测试的位置。 同样,在生产中,当创建二进制文件时,将排除这些文件。
Next, we take a look at the function name. Every test function name should start with “Test” and note that its a capital T, followed by uppercase later for the name. We do need to accept one parameter, which is the pointer to testing.T object. Important thing is, it does have to be a pointer to testing.T object, and that’s going give our test access to the test runner letting it know that different situation and different things that are going on within our test.
接下来,我们来看一下函数名。 每个测试函数名称都应以“ Test”开头,并注意其大写字母T,其后为大写字母。 我们确实需要接受一个参数,它是指向testing.T对象的指针。 重要的是,它必须是指向testing.T对象的指针,这将使我们的测试可以访问测试运行程序,从而使其知道测试中正在发生的不同情况和不同情况。
We can also see that, package name has been given the same name as our “main” package. This allows us to perform white box testing. When we use the same package name, we are the part of the same package and we’ve access to all of the package level variables within our task. Alternatively, we can add “_test” suffix in our package name, hence making our test a black box test. Since we have a different package name, we no have no longer access to package level entities.
我们还可以看到,软件包名称与“主”软件包的名称相同。 这使我们能够执行白盒测试。 当我们使用相同的程序包名称时,我们就是同一程序包的一部分,并且可以访问任务中的所有程序包级别变量。 或者,我们可以在包名称中添加“ _test”后缀,从而使我们的测试成为黑盒测试。 由于我们使用不同的软件包名称,因此我们不再有权访问软件包级别的实体。
So which one should we? Generally, it’s best practice to try and write black box test because that means your test are actually testing the external APIs of your package and they are not worried about internal implementation details. That’s gonna make our test more robust and representative.
那么我们应该选哪一个呢? 通常,最佳实践是尝试编写黑盒测试,因为这意味着您的测试实际上是在测试包的外部API,并且他们并不担心内部实现细节。 这将使我们的测试更强大和更具代表性。
Demo: Writing a test
演示:编写测试
That’s the basics one should know for writing tests in Go. Now, lets dive in to writing our first test in Go. This is a very simple test program, which will provide the basic understanding and methodologies required for writing a test. First of all create a create a Go project and initiate a .mod file. No additional libraries are needed to install as well.
这是在Go中编写测试的基本知识。 现在,让我们开始编写Go中的第一个测试。 这是一个非常简单的测试程序,它将提供编写测试所需的基本知识和方法。 首先创建一个创建Go项目并启动一个.mod文件。 也不需要安装其他库。
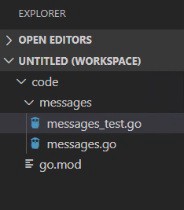
Now, lets take a look at the codes inside the file.
现在,让我们看一下文件中的代码。
“message.go” contains two functions Greet() and Depart().
“ message.go”包含两个函数Greet()和Depart()。
package messagesimport ("fmt")func Greet(name string) string {return fmt.Sprintf("Hello, %v!\n", name)}func Depart(name string) string {return fmt.Sprintf("GoodBye, %v\n", name)}
Greet function greet the user and Depart function takes a departure from the user. Both the functions are quite simple.
迎宾功能向用户致意,而离开功能则使用户离开。 这两个功能都非常简单。
Now let’s take a look at “message_test” file. It consist of following sets of code:
现在让我们看一下“ message_test”文件。 它由以下几组代码组成:
package messagesimport "testing"func TestGreet(t *testing.T) {got := Greet("Flash")expected := "Hello, Flash!\n"if got != expected {t.Errorf("Did not get expected result. Wanted %q, got %q\n", expected, got)}}func TestDepart(t *testing.T) {got := depart("Flash")expected := "GoodBye, Flash\n"if got != expected {t.Errorf("Did not get expected result. Wanted %q, got %q\n", expected, got)}}
Here, you may have noticed that we have declared the package name as messages. It is done so that we can get access to the entities of other files within the package.
在这里,您可能已经注意到我们已经将包名称声明为message。 这样做是为了使我们能够访问包中其他文件的实体。
We can name the package as messages_test, but we also have to import the messages package so that we can get access to the functions in message.go file.
我们可以将包命名为messages_test ,但是我们还必须导入messages包,以便可以访问message.go文件中的函数。
Running the test
运行测试
The most important command to run a test is “go test” command. It runs all the test in current directory. If you want to test specific packages, then you can add package name directly after the “test” keyword, i.e, go test {pkg1}, {pkg2},….{pkgn}.
运行测试最重要的命令是“ go test”命令。 它在当前目录中运行所有测试。 如果要测试特定的软件包,则可以在“ test”关键字之后直接添加软件包名称,即进行测试{pkg1},{pkg2},…。{pkgn} 。
We can also use syntax similar to go “test ./….”. This command is going to find out and run tests in current directory and descendant directories. Similarly, we can use “go test -v” command for generating verbose output and “go test-run {regexp}” command to run only test matching the {regexp}.
我们还可以使用类似的语法来“测试./…。”。 此命令将查找并在当前目录和后代目录中运行测试。 同样,我们可以使用“ go test -v”命令生成详细的输出,并使用“ go test-run {regexp}”命令仅运行与{regexp}匹配的测试。
Now, lets run our test functions that we have created above. To run the test we should open the terminal in the folder where we have created our project. Here in my device, I have open my message directory in the terminal.
现在,让我们运行上面创建的测试功能。 要运行测试,我们应该在创建项目的文件夹中打开终端。 在我的设备中,我已经在终端中打开了消息目录。
go test
When the above command is executed, we get a message as:
当执行以上命令时,我们得到的消息为:

But, if we changed the name from “Flash” to “Batman”, then we get message such as :
但是,如果我们将名称从“ Flash”更改为“ Batman”,则会收到诸如以下消息:

Hence, we are shown the message that our test has failed.
因此,向我们显示了测试失败的消息。
Failure Methods in Go
Go中的失败方法
In the above example, I have directly used the methods as t.Errorf(), which is used for reporting the failures.
在上面的示例中,我直接将这些方法用作t.Errorf() ,用于报告故障。
Generally, there are two types of failure: Immediate failure and Non-immediate failure.
通常,有两种类型的故障: 立即故障和非立即故障 。
Immediate failure are going to exit that test immediately and non-immediate failure are going to indicate that an error have occurred, but your test function is going to continue executing.
立即失败将立即退出该测试, 非立即失败将指示已发生错误,但是您的测试功能将继续执行。
Methods for Immediate Failure:
立即失败的方法:
- t.FailNow() t.FailNow()
- t.Fatal(args…interface{}) t.Fatal(args ... interface {})
- t.Fatalf(format string, args…interface{}) t.Fatalf(格式字符串,args…interface {})
Methods for Non-immediate Failure:
非立即失败的方法:
- t.Fail() t.Fail()
- t.Error(args…interface{}) t.Error(args ... interface {})
- t.Errorf(format string, args…interface{}) t.Errorf(格式字符串,args…interface {})
This is the basic things one should know for creating and executing test in Go. I have tried my best to keep things simple and short as possible. Software testing is really required to point out the defects and errors that were made during the development phases. So, we as a software engineer/programmer should have a practice of writing tests for our applications.
这是在Go中创建和执行测试的基本知识。 我已尽力使事情简单而简短。 确实需要进行软件测试,以指出在开发阶段进行的缺陷和错误。 因此,我们作为软件工程师/程序员,应具有为应用程序编写测试的实践。
I hope this article would be of help for anyone who is trying to learn about the testing in Go. Feel free to provide response and feedbacks. Thank You!!
我希望本文对尝试了解Go中的测试的人有所帮助。 随时提供回应和反馈。 谢谢!!
Keep Learning!! Keep Learning!! Stay Safe!! 😊
保持学习!! 保持学习!! 注意安全!! 😊
翻译自: https://medium.com/wesionary-team/testing-in-go-ac2ec07979cb
golang测试