python实习
编程,PYTHON (PROGRAMMING, PYTHON)
There are different Python implementations out there such as CPython, Jython, IronPython, etc. The optimization techniques we are going to discuss in this article are related to CPython which is standard Python implementation.
那里有不同的Python实现,例如CPython , Jython , IronPython等。我们将在本文中讨论的优化技术与标准Python实现CPython有关。
实习生 (Interning)
Interning is re-using the objects on-demand instead of creating the new objects. What does this mean? Let’s try to understand Integer and String interning with examples.
实习是按需重新使用对象,而不是创建新对象。 这是什么意思? 让我们尝试通过示例来理解Integer和String实习。
is — this is used to compare the memory location of two python objects.id — this returns memory location in base-10.
is —用于比较两个python对象的内存位置。 id —返回以10为基数的内存位置。
整数实习 (Integer interning)
At startup, Python pre-loads/caches a list of integers into the memory. These are in the range -5 to +256
. Any time when we try to create an integer object within this range, Python automatically refer to these objects in the memory instead of creating new integer objects.
在启动时,Python将整数列表预加载/缓存到内存中。 它们的范围是-5 to +256
。 每当我们尝试在此范围内创建整数对象时,Python都会自动在内存中引用这些对象,而不是创建新的整数对象。
The reason behind this optimization strategy is simple that integers in the -5 to 256
are used more often. So it makes sense to store them in the main memory. So, Python pre-loads them in the memory at the startup so that speed and memory are optimized.
此优化策略背后的原因很简单, -5 to 256
整数被更频繁地使用。 因此将它们存储在主存储器中很有意义。 因此,Python在启动时将它们预先加载到内存中,以便优化速度和内存。
Example 1:
范例1:
In this example, both a
and b
are assigned to value 100. Since it is within the range -5 to +256
, Python uses interning so that b
will also reference the same memory location instead of creating another integer object with value 100.
在此示例中, a
和b
都分配了值100。由于它在-5 to +256
范围内,因此Python使用interning,因此b
也将引用相同的内存位置,而不是创建另一个值为100的整数对象。
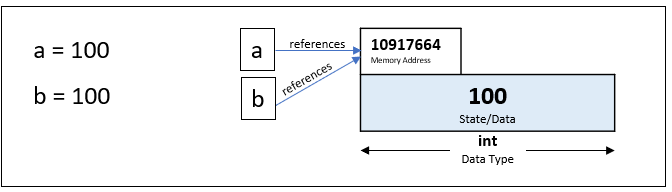
As we can see from the code below, both a
and b
are referencing the same object in the memory. Python will not create a new object but instead references to a
’s memory location. This is all due to integer interning.
从下面的代码中可以看到, a
和b
都引用了内存中的同一对象。 Python将不会创建新的对象,而是引用a
的内存位置。 这都是由于整数实习。

Example 2:
范例2:
In this example, both a
and b
are assigned with value 1000. Since it is outside the range -5 to +256, Python will create two integer objects. So both a and b will be stored in different locations in the memory.
在此示例中, a
和b
都分配了值1000。由于它在-5到+256范围之外,因此Python将创建两个整数对象。 因此,a和b都将存储在内存中的不同位置。

As we can see from the code below, both a
and b
are stored in different locations in the memory.
从下面的代码可以看到, a
和b
都存储在内存中的不同位置。

字符串实习 (String interning)
Like integers, some of the strings also get interned. Generally, any string that satisfies the identifier naming convention will get interned. Sometimes there will be exceptions. So, don’t rely on it.
像整数一样,某些字符串也会被固定。 通常,满足标识符命名约定的任何字符串都会被中断。 有时会有例外。 因此,请不要依赖它。
Example 1:
范例1:
The string “Data” is a valid identifier, Python interns the string so both the variables will point to the same memory locations.
字符串“ Data”是有效的标识符,Python会在字符串中插入一个空格,以便两个变量都指向相同的内存位置。

Example 2:
范例2:
The string “Data Science” is not a valid identifier. Hence string interning is not applied here so both a and b will point to two different memory locations.
字符串“ Data Science”不是有效的标识符。 因此,这里不应用字符串实习,因此a和b都将指向两个不同的存储位置。

All the above examples are from Google Colab which has Python version 3.6.9
以上所有示例均来自具有Python 3.6.9版的Google Colab。
In Python 3.6, any valid string with length ≤ 20 will get interned. But in Python 3.7, this has been changed to 4096. So as I mentioned earlier, these things will keep changing for different Python versions.
在Python 3.6中,长度小于或等于20的任何有效字符串都会被屏蔽。 但是在Python 3.7中,此值已更改为4096。因此,正如我之前提到的,这些情况将针对不同的Python版本不断变化。
Since not all strings are interned, Python provides the option force the string to be interned using sys.intern()
. This should not be used unless there is a need. Refer the sample code below.
由于并非所有字符串都被sys.intern()
,因此Python提供了使用sys.intern()
强制字符串被sys.intern()
的选项。 除非有必要,否则不应使用此功能。 请参考下面的示例代码。

为什么字符串实习很重要? (Why string interning is important?)
Let’s assume that you have an application where a lot of string operations are happening. If we were to use equality operator ==
for comparing long strings Python tries to compare it character by character and obviously it will take some time. But if these long strings can be interned then we know that they point to the same memory location. In such a case we can use is
keyword for comparing memory locations as it works much faster.
假设您有一个正在执行许多字符串操作的应用程序。 如果我们使用equality operator ==
来比较长字符串,Python会尝试逐个字符地比较它,这显然会花费一些时间。 但是,如果可以插入这些长字符串,那么我们知道它们指向相同的存储位置。 在这种情况下,我们可以使用is
关键字来比较内存位置,因为它的工作速度更快。
结论 (Conclusion)
Hope that you have understood the concept of interning for optimization in Python.
希望您已经了解了Python 实习优化的概念。
If you are interested in learning more about optimization go through the Peephole optimization.
如果您有兴趣了解有关优化的更多信息,请进行“ 窥Kong优化” 。
To understand Mutability and Immutability in Python, please click here to read the article.
要了解Python中的可变性和不变性 ,请单击此处阅读本文。
Follow me if you would like to read more such articles on Python and Data Science.
如果您想阅读更多有关Python和数据科学的文章,请关注我。
Thank you so much for taking out time to read this article. You can reach me at https://www.linkedin.com/in/chetanambi/
非常感谢您抽出宝贵的时间阅读本文。 您可以通过https://www.linkedin.com/in/chetanambi/与我联系
翻译自: https://towardsdatascience.com/optimization-in-python-interning-805be5e9fd3e
python实习