accumulate
reduce()vs累加 (reduce() vs accumulate)
减少() (reduce())
The functools
module is for higher-order functions. Functions that act on or return other functions. In general, any callable object can be treated as a function for the purposes of this module.
functools
模块用于高阶函数。 作用于或返回其他功能的功能。 通常,就此模块而言,任何可调用对象都可以视为函数。
The functools module provides the following function functools.reduce()
functools模块提供以下功能functools.reduce()
functools.reduce()
functools.reduce()
Apply function of two arguments cumulatively to the items of iterable, from left to right, so as to reduce the iterable to a single value.
将两个参数的函数从左到右累计应用于iterable的项,以将iterable减少为单个值。
functools.reduce(function, iterable,initializer)
functools. reduce ( function , iterable,initializer )
If the optional initializer
is present, it is placed before the items of the iterable in the calculation, and serves as a default when the iterable is empty.
如果存在可选的initializer
值设定项,则将其放在计算中可迭代项的前面,并在可迭代项为空时用作默认值。
If initializer
is not given and iterable contains only one item, the first item is returned.
如果未给出initializer
值设定项,并且iterable仅包含一项,则返回第一项。
Example :reduce(lambda x, y: x+y, [1, 2, 3, 4, 5])
示例: reduce(lambda x, y: x+y, [1, 2, 3, 4, 5])

Example 1: Find the product of the list elements using reduce()
示例1:使用reduce()查找列表元素的乘积
from functools import reduce
l1=[1,2,3,4,5]
l2=reduce(lambda x,y:x*y,l1)
print (l2)#Output:120
Example 2:Find the largest number in the iterable using reduce()
示例2:使用reduce()查找可迭代的最大数字
from functools import reduce
l1=[15,12,30,4,5]
l2=reduce(lambda x,y: x if x>y else y,l1)
print (l2)#Output:30
图示: (Pictorial representation:)
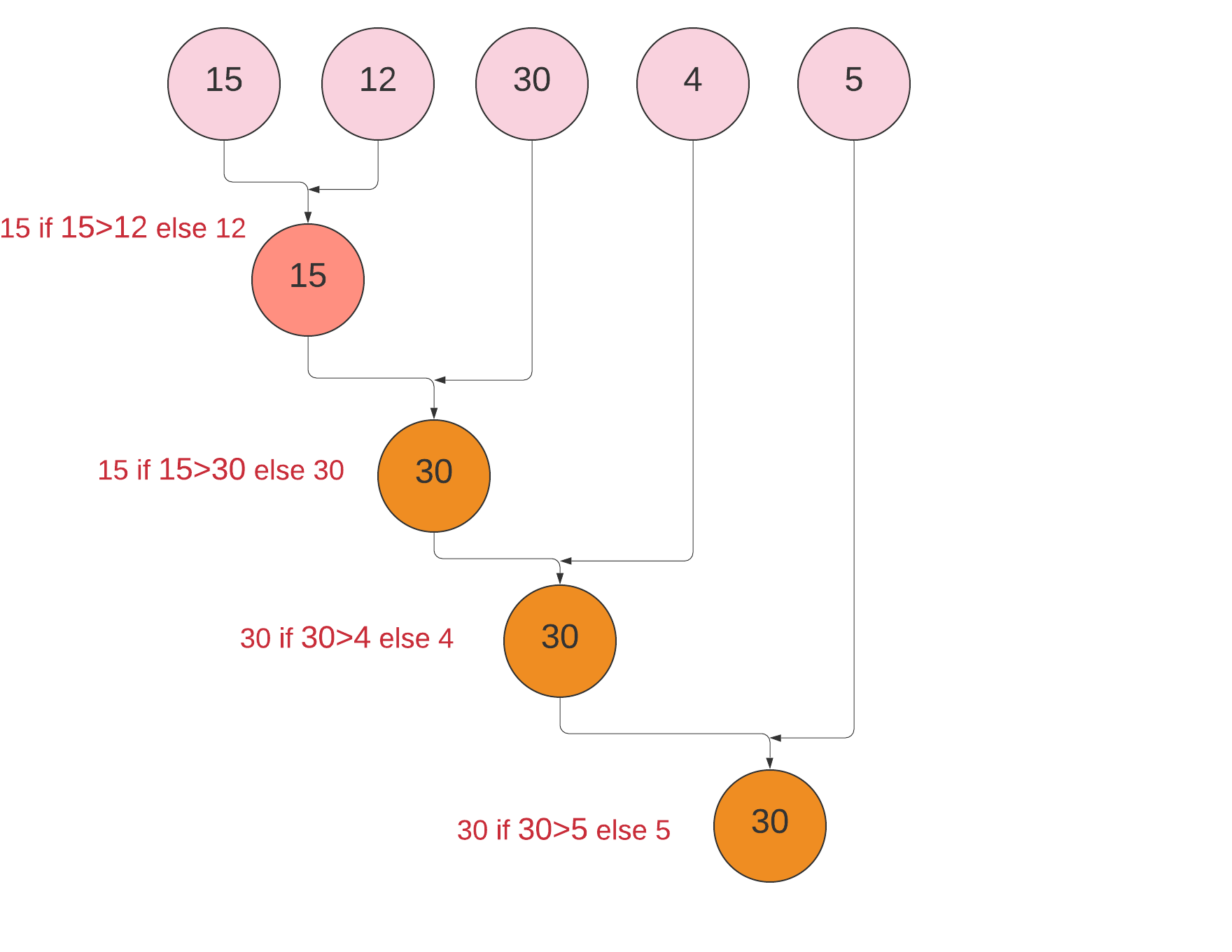
Example 3:Using User-defined function in reduce()
示例3:在reduce()中使用用户定义的函数
from functools import reducedef sum1(x,y):
return x+y
l1=[15,12,30,4,5]
l2=reduce(sum1,l1)
print (l2)#Output:66
Example 4: Initializer is mentioned.
示例4:提到初始化程序。
If the optional initializer
is present, it is placed before the items of the iterable in the calculation.
如果存在可选的initializer
程序,则将其放在计算中可迭代项的前面。
from functools import reducedef sum1(x,y):
return x+y
l1=[1,2,3,4,5]
l2=reduce(sum1,l1,10)
print (l2)#Output:25
Example 5:Iterable contains only one item, reduce() will return that item.
示例5:Iterable仅包含一项,reduce()将返回该项。
from functools import reducedef sum1(x,y):
return x+y
l1=[5]
l2=reduce(sum1,l1)
print (l2)#Output:5from functools import reduce
l1=[15]
l2=reduce(lambda x,y: x if x>y else y,l1)
print (l2)#Output:15
Example 6: If iterable is empty and the initializer is given, reduce() will return the initializer.
示例6:如果iterable为空,并且给出了初始化程序,则reduce()将返回初始化程序。
from functools import reducedef sum1(x,y):
return x+y
l1=[]
l2=reduce(sum1,l1,10)
print (l2)#Output:10
itertools.accumulate() (itertools.accumulate())
Makes an iterator that returns accumulated sum or accumulated results of other binary functions which is mentioned in func-parameter.If func is supplied, it should be a function of two arguments. Elements of the input iterable may be any type that can be accepted as arguments to func.-Python documentation
生成一个迭代器,该迭代器返回func-parameter中提到的其他二进制函数的累加和或累加结果。如果提供了func ,则它应该是两个参数的函数。 可迭代输入的元素可以是可以接受为func参数的任何类型。- Python文档
itertools.accumulate(iterable[, func, *, initial=None])
itertools. accumulate ( iterable [, func , * , initial=None ])
Example 1:By using itertools.accumulate(), we can find the running product of an iterable. The function argument is given as operator.mul.
示例1:通过使用itertools.accumulate(),我们可以找到iterable的运行产品。 函数参数作为operator.mul给出。
It will return an iterator that yields all intermediate values. We can convert to list by using a list() constructor.
它将返回产生所有中间值的迭代器。 我们可以使用list()构造函数转换为list。
from itertools import accumulateimport operator
l2=accumulate([1,2,3,4,5],operator.mul)
print (list(l2))#Output:[1, 2, 6, 24, 120]
Pictorial Representation.
绘画作品。

Example 2: If the function parameter is not mentioned, by default it will perform an addition operation
示例2:如果未提及功能参数,则默认情况下它将执行加法运算
It will return an iterator that yields all intermediate values. We can convert to list by using list() constructor.
它将返回产生所有中间值的迭代器。 我们可以使用list()构造函数转换为列表。
import itertools
#using add operator,so importing operator moduleimport operator
l1=itertools.accumulate([1,2,3,4,5])
print (l1)#Output:<itertools.accumulate object at 0x02CD94C8>
#Converting iterator to list object.
print (list(l1))#Output:[1, 3, 6, 10, 15]#using reduce() for same functionfrom functools import reduce
r1=reduce(operator.add,[1,2,3,4,5])
print (r1)#Output:15
Example 3:Function argument is given as max(), to find a running maximum
示例3:函数参数以max()的形式给出,以查找运行最大值
from itertools import accumulate
l4=accumulate([2,4,6,3,1],max)
print (list(l4))#Output:[2, 4, 6, 6, 6]
Example 4: If the initial value is mentioned, it will start accumulating from the initial value.
例4:如果提到了初始值,它将从初始值开始累加。
from itertools import accumulateimport operator
#If initial parameter is mentioned, it will start accumulating from the initial value.
#It will contain more than one element in the ouptut iterable.
l2=accumulate([1,2,3,4,5],operator.add,initial=10)
print (list(l2))#Output:[10, 11, 13, 16, 20, 25]
Example 5: If the iterable is empty and the initial parameter is mentioned, it will return the initial value.
示例5:如果iterable为空,并且提到了初始参数,它将返回初始值。
from itertools import accumulateimport operatorl2=accumulate([],operator.add,initial=10)
print (list(l2))#Output:[10]
Example 6: If iterable contains one element and the initial parameter is not mentioned, it will return that element.
示例6:如果iterable包含一个元素,并且未提及初始参数,它将返回该元素。
from itertools import accumulate
l2=accumulate([5],lambda x,y:x+y)
print (list(l2))#Output:[5]
Example 7: Iterating through the iterator using for loop
示例7:使用for循环遍历迭代器
Return type is an iterator. We can iterate through iterator using for loop also.
返回类型是一个迭代器。 我们也可以使用for循环遍历迭代器。
from itertools import accumulate
l2=accumulate([5,6,7],lambda x,y:x+y)for i in l2:
print (i)
'''
Output:
5
11
18
'''
reduce()和accumulate()之间的区别 (Differences between reduce() and accumulate())
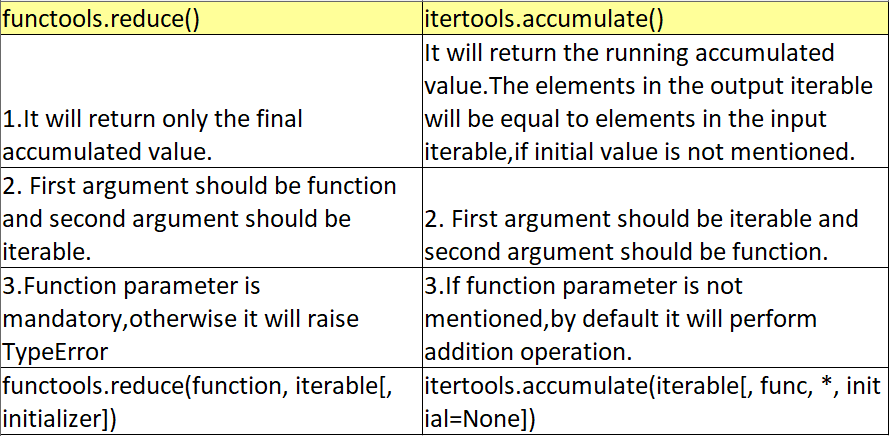
结论: (Conclusion:)
reduce() function is supported by the functools module.
functools模块支持reduce()函数。
accumulate() function is supported by the itertools module.
itertools模块支持accumulate()函数。
reduce() will return only an accumulated value.
reduce()将仅返回一个累加值 。
accumulate() will return the running accumulated value. Like we can find running max, running product, running sum of an iterable using the accumulate() function.
accumulate()将返回运行的累积值 。 就像我们可以使用accumulate()函数找到运行的最大值,运行的产品,可迭代的运行总和。
accumulate() returns an iterator.
accumulate()返回一个迭代器。
Thank you for reading my article, I hope you found it helpful!
感谢您阅读我的文章,希望对您有所帮助!
资源(Python文档): (Resources(Python Documentation):)
翻译自: https://codeburst.io/reduce-vs-accumulate-in-python-3ecee4ee8094
accumulate