Computer program: A list of instructions(code) meant to be followed by a computer
Executing a program: Programs must first be converted into machine code that the computer can understand and execute
Compiler: A program that translates a programming language into machine code is called a compiler
Program -> compiler -> machine code -> operating system(platform)
In JAVA
The Java compiler produces an intermediate format called bytecode.
Java program -> compiler -> Java Bytecode (not machine code for real computer, is machine code for a model computer, called Java Virtual Machine(JVM))
Java Interpreter: convert the bytecode into machine code(Simulates the execution of the JVM on the real computer)
Can run bytecode on any computer that has a Java Interpreter (JRE - Java Runtime Environment - Java 8, Java 9...download from Oracle website) installed
Advantages of Using Java:
- Once a Java program is compiled, you can run the bytecode on any machine with a Java Interpreter. Because you do not have to recompile the program for each machine, Java is platform independent.
- Java is safe. The Java language and compiler restrict certain operations to prevent errors.
- Java standardizes many useful structures and operations such as lists, managing network connections, and providing graphical user interfaces
Disadvantages of Using Java:
- Running bytecode through an interpreter is not as fast as running machine code But this disadvantage is slowly disappearing
- Using platform-specific features (e.g., Windows taskbar) is difficult in Java because Java is platform-independent.
- In order to run a Java program on multiple machines, you must install a Java Interpreter on each machine
Programming methodology:
- Specify and analyze the problem (remove ambiguity Decide on inputs/outputs and algorithms)
- Design the program solution (organize problem into smaller pieces Identify existing code to reuse)
- Implementation (programming)
- Test and verify implementation
- Maintain and update program
Program structure
/* The HelloWorld class prints “Hello, World!” to the screen */ public class HelloWorld { public static void main(String[] args) { // Prints “Hello, World!” System.out.println("Hello, World!"); } }
Every executable Java program consists of a class that contains a method named main
Variables in computer program represent data
Variables in Java have a type. The type defines what kinds of values a variable is allowed to store. (allocate the memory space according to the type)
Integer Types:
- int: +-231 (32 bits)
- long: +-263 (64 bits)
- short: +-215 (16 bits)
- byte: +- 128 (8 bits)
Floating Point (Decimal) Types:
- float: Single-precision decimal numbers. 32 bits
- double: Double-precision decimal numbers. 64 bits
-
double z = 1/3; // z = 0.0 /* * 1 and 3 are all integers, so the result is 0 * The following method can get the correct answer */ double t = 1d/3; // t = 0.333333... double t = (double)1/3 double t = 1.0/3
Other Types:
- String: Letters, words, or sentences. enclosed in double quotes.
- boolean: True or false.
- char: Single Latin Alphanumeric Characters. enclosed in single quotes.
Type
- Variables must be declared before they can be used.
- Variables are a symbolic name given to a memory location.
- All variables have a type which is enforced by the compiler
- Although Java provides ALL variables with an initial value, variables should be initialized before being used. It is considered a good practice to initialize variables upon declaration.
- Variables declared within a method must be initialized before use or the compiler with issue an error.
- Constant values: including the keyword final in its declaration. the names of variables defined as final are UPPERCASE. Final variables must be initialized upon declaration.
Expressions can be combinations of variables, primitives and operators that result in a value
Operators are special symbols used for:
- mathematical functions
- assignment statements
- logical comparisons
5 different groups of operators:
- Arithmetic Operators
- + - * / %(modulo/remainder)
- Order of Operations: Parentheses > Exponent > * / > + -
- Assignment Operator
- = name = value
- += -= *= /= Eg. x *= y+5 is equal to x = x * (y+5)
- Increment / Decrement Operators
- i++ or ++i (++i means increase i before operate the expression, i++ means operate the expression forst and then increase i)
- i-- or --i
- Relational Operators
- > >= == != <= <
- Conditional Operators
- &&(AND) ||(OR) !(NOT)
- short-circuit evaluation
Type casting
int a = 2 //a = 2 double a =2 //a = 2.0 (Implicit) int a = 18.7 //ERROR int a = (int)18.7 //a = 18
char a = 'A'
byte b = (byte)a; //b = 65
Methods
Methods are a way of organizing a sequence of statements into a named unit
Methods can accept inputs (called arguments) and can output a value (called a return value) that is the result of the computations
Hiding the internal workings of a method and providing the correct answer is known as abstraction
Methods can also return nothing in which case they are declared void.
Method consists of Return type, Name, Arguments, Body.
The return type and arguments may be either primitive data types (i.e. int) or complex data types (i.e. Objects),
double divide(double a, double b){ double answer; answer = a / b; return answer; }
Recursive method
int factorial(int n) { if (n==0) //base case/stopping point return 1; else return n * factorial (n-1); //recursive call }
Static method
static double divide(double a, double b) { return a / b; }
Main method: main is a special Java method which the java interpreter looks for when you try to run a class file
class SayHi { public static void main(String[] args) { System.out.println("Hi, " + args[0]); } } java SayHi Charon output: Hi, Charon
Control Structure
Use pre-established code structures:
- block statements (anything contained within curly brackets)
- decision statements ( If, If-Else, Switch )
- Loops ( For, While )
If-Else
int price = 2; if (price > 3) { System.out.println(“Too expensive”); } else { System.out.println(“Good deal”); }
switch
switch(grade){ case 'A': System.out.println('You got an A'); break; case 'B': System.out.println('You got a B'); break; case 'C': System.out.println('You got a C'); break; default: System.out.println('You got a F'); }
while
int limit = 4; int sum = 0; int i = 1; while (i < limit){ sum += i; i++; }
do-while
boolean test = false; do{ System.out.println(“Hey!”) } while(test)
for
int limit = 4; int sum = 0; for(int i = 1; i<=limit; i++ ) { sum += i; }
break continue
Nested loop
for (int i=1; i<=5; i++){ for (int j=1; j<=i; j++){ System.out.println(“*”); } }
Arrays
An array is a series of compartments to store data.
Arrays have a type, name, and size.
We refer to each item in an array as an element
The position of each element is known as its index
Declaration:
int[] price; or int price[];
We need to allocate memory to store arrays
Use the new keyword to allocate memory: price = new int[3];
Combin declaration and allocation: int[] price = new int[3];
Using an Array: price[0] = 60; price[1] = 120; price[2] = 240;
Initializing Arrays: int[] price = {60, 120, 240}
Lesgth of Array: int cnt = price.length; cnt=3
String[] people = {“Gleb”, “Lawrence”, “Michael”, “Stephanie”, “Zawadi”}; for(int i=0; i<names.length; i++) System.out.println(names[i]+”!");
2-Dimensional Arrays - can be thought of as a grid (or matrix) of values - an array of arrays
double[][] heights; heights = new double[20][55]; //This 2-D array has 20 rows and 55 columns //To access the acre at row index 11 and column index 23: heights[11][23]
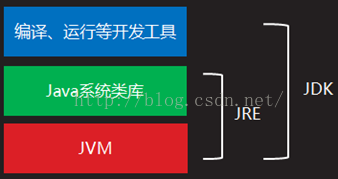
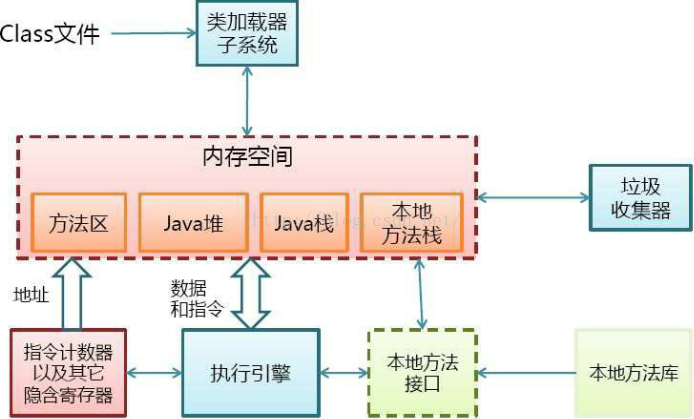
1. 装载
装载过程负责找到二进制字节码并加载至JVM中,JVM通过类名、类所在的包名通过ClassLoader来完成类的加载,同样,也采用以上三个元素来标识一个被加载了的类:类名+包名+ClassLoader实例ID。
2. 链接
链接过程负责对二进制字节码的格式进行校验、初始化装载类中的静态变量以及解析类中调用的接口、类。
完成校验后,JVM初始化类中的静态变量,并将其值赋为默认值。
最后对类中的所有属性、方法进行验证,以确保其需要调用的属性、方法存在,以及具备应的权限(例如public、private域权限等),会造成NoSuchMethodError、NoSuchFieldError等错误信息。
3. 初始化
初始化过程即为执行类中的静态初始化代码、构造器代码以及静态属性的初始化,在四种情况下初始化过程会被触发执行:
调用了new;
反射调用了类中的方法;
子类调用了初始化;
JVM启动过程中指定的初始化类。
ClassLoader抽象类的几个关键方法:
(1) loadClass
此方法负责加载指定名字的类,ClassLoader的实现方法为先从已经加载的类中寻找,如没有则继续从parent ClassLoader中寻找,如仍然没找到,则从System ClassLoader中寻找,最后再调用findClass方法来寻找,如要改变类的加载顺序,则可覆盖此方法
(2) findLoadedClass
此方法负责从当前ClassLoader实例对象的缓存中寻找已加载的类,调用的为native的方法。
(3) findClass
此方法直接抛出ClassNotFoundException,因此需要通过覆盖loadClass或此方法来以自定义的方式加载相应的类。
(4) findSystemClass
此方法负责从System ClassLoader中寻找类,如未找到,则继续从Bootstrap ClassLoader中寻找,如仍然为找到,则返回null。
(5) defineClass
此方法负责将二进制的字节码转换为Class对象
(6) resolveClass
此方法负责完成Class对象的链接,如已链接过,则会直接返回。
JVM运行时数据区:第一块:PC寄存器
PC寄存器是用于存储每个线程下一步将执行的JVM指令,如该方法为native的,则PC寄存器中不存储任何信息。
第二块:JVM栈
JVM栈是线程私有的,每个线程创建的同时都会创建JVM栈,JVM栈中存放的为当前线程中局部基本类型的变量(java中定义的八种基本类型:boolean、char、byte、short、int、long、float、double)、部分的返回结果以及Stack Frame,非基本类型的对象在JVM栈上仅存放一个指向堆上的地址
第三块:堆(Heap)
它是JVM用来存储对象实例以及数组值的区域,可以认为Java中所有通过new创建的对象的内存都在此分配,Heap中的对象的内存需要等待GC进行回收。
第四块:方法区域(Method Area)
(1)在Sun JDK中这块区域对应的为PermanetGeneration,又称为持久代。
(2)方法区域存放了所加载的类的信息(名称、修饰符等)、类中的静态变量、类中定义为final类型的常量、类中的Field信息、类中的方法信息,当开发人员在程序中通过Class对象中的getName、isInterface等方法来获取信息时,这些数据都来源于方法区域,同时方法区域也是全局共享的,在一定的条件下它也会被GC,当方法区域需要使用的内存超过其允许的大小时,会抛出OutOfMemory的错误信息。
第五块:运行时常量池(Runtime Constant Pool)
存放的为类中的固定的常量信息、方法和Field的引用信息等,其空间从方法区域中分配。
第六块:本地方法堆栈(Native Method Stacks)
JVM采用本地方法堆栈来支持native方法的执行,此区域用于存储每个native方法调用的状态