援引:https://www.jianshu.com/p/1ee2619137f9
文章之前,我们先来看下本文的知识大纲:
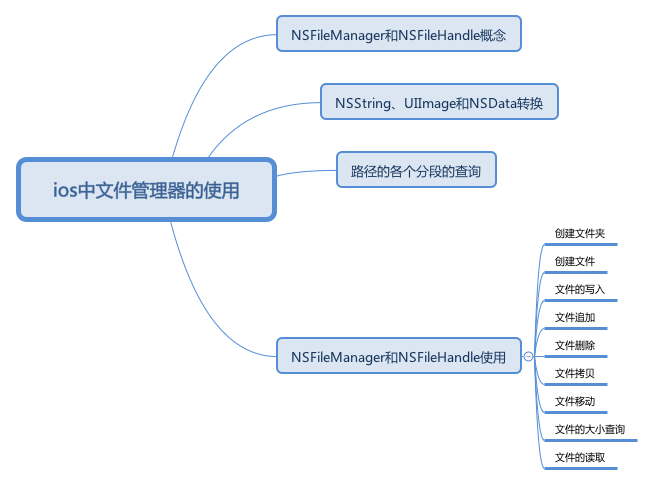
ios中文件管理器的使用.png
1.NSFileManager和NSFilehandle的概念 1.NSFileManager 是 Foundation 框架中用来管理和操作文件、目录等文件系统相关联内容的类。 2.NSFileHandle类:它需要配合NSFileManager文件管理类,对文件内容进行操作,写入数据、读取数据。 2.NSFileManager和NSFileHandle的配合使用,实现文件的增删改查等功能 2.1 拿到沙盒的Document路径(通常默认放在这个路径下) // 拿到沙盒路径 -(NSString*)getDocumentPath { // @expandTilde 是否覆盖 NSArray *paths = NSSearchPathForDirectoriesInDomains(NSDocumentDirectory, NSUserDomainMask, YES); NSString *documentsDirectory = [paths objectAtIndex:0]; return documentsDirectory; } 2.2 创建文件夹,并且判断是否创建成功 NSString *documentsPath =[self getDocumentPath]; NSFileManager *fileManager = [NSFileManager defaultManager];// NSFileManager 是 Foundation 框架中用来管理和操作文件、目录等文件系统相关联内容的类。 NSString * testDirectory = [documentsPath stringByAppendingPathComponent:@"日记"]; BOOL res = [fileManager createDirectoryAtPath:testDirectory withIntermediateDirectories:YES attributes:nil error:nil]; if (res) { NSLog(@"文件夹创建成功"); }else { NSLog(@"文件夹创建失败"); } 2.3 创建文件,并判断文件是否创建 NSString *testPath = [testDirectory stringByAppendingPathComponent:@"test.txt"]; BOOL res1 = [fileManager createFileAtPath:testPath contents:nil attributes:nil]; if (res1) { NSLog(@"文件创建成功: %@" ,testPath); }else { NSLog(@"文件创建失败"); } 2.4 写入文件,并判断文件是否存在 NSString * str = @"你是一位好同志"; BOOL res2 = [str writeToFile:testPath atomically:YES encoding:NSUTF8StringEncoding error:nil]; if (res2) { NSLog(@"文件写入成功"); }else { NSLog(@"文件写入失败"); } // 判断文件是否存在 if ([fileManager fileExistsAtPath:testPath]) { NSLog(@" 是否存在 "); } 2.5 追加写入内容 // 追加内容 NSFileHandle * handFile = [NSFileHandle fileHandleForUpdatingAtPath:testPath]; //NSFileHandle类:它需要配合NSFileManager文件管理类,对文件内容进行操作,写入数据、读取数据。 [handFile seekToEndOfFile];// 文件光标移动末尾 NSString * str1 = @"追加内容";// 追加内容 NSData * data = [str1 dataUsingEncoding:NSUTF8StringEncoding];// 转换文件格式 [handFile writeData:data];// 写入文件 [handFile closeFile];// 关闭 2.6 删除文件,并判断文件是否删除 // 删除文件 BOOL isOK = [fileManager removeItemAtPath:testPath error:nil]; if (isOK) { NSLog(@"-- 成功删除---"); } 2.7 拷贝文件 // 拷贝文件 NSString *targetPath = [testDirectory stringByAppendingPathComponent:@"test1.txt"]; //目标文件路径 [fileManager copyItemAtPath:testPath toPath:targetPath error:nil]; // 拷贝文件 2.8 移动文件 [fileManager moveItemAtPath:testPath toPath:targetPath error:nil]; 2.9 读取文件 NSString * content = [NSString stringWithContentsOfFile:testPath encoding:NSUTF8StringEncoding error:nil]; NSLog(@"拿到文章的内容 -- %@",content); 2.10 计算文件大小 // 计算文件大小 - (unsigned long long)fileSizeAtPath:(NSString *)filePath { NSFileManager *fileManager = [NSFileManager defaultManager]; BOOL isExist = [fileManager fileExistsAtPath:filePath]; if (isExist) { unsigned long long fileSize = [[fileManager attributesOfItemAtPath:filePath error:nil] fileSize]; return fileSize; } else { NSLog(@"file is not exist"); return 0; } } 2.11 计算文件夹大小 // 计算整个文件夹中所有文件大小 - (unsigned long long)folderSizeAtPath:(NSString*)folderPath { NSFileManager *fileManager = [NSFileManager defaultManager]; BOOL isExist = [fileManager fileExistsAtPath:folderPath]; if (isExist) { NSEnumerator *childFileEnumerator = [[fileManager subpathsAtPath:folderPath] objectEnumerator]; unsigned long long folderSize = 0; NSString *fileName = @""; while ((fileName = [childFileEnumerator nextObject]) != nil){ NSString* fileAbsolutePath = [folderPath stringByAppendingPathComponent:fileName]; folderSize += [self fileSizeAtPath:fileAbsolutePath]; } return folderSize / (1024.0 * 1024.0); } else { NSLog(@"file is not exist"); return 0; } } 3.NSString、UIImage 和 NSData的相互转换 3.1 NSString 和 NSData转换 NSString * string = @"123456"; NSData * data = [string dataUsingEncoding:NSUTF8StringEncoding]; //NSString 转换为NSData NSString * str = [[NSString alloc]initWithData:data encoding:NSUTF8StringEncoding];////NSData 转换为NSString 3.2 UIImage 和 NSData转换 //NSData转换为UIImage NSString * imagePath = @"/Users/yyf/Desktop/ios中文件管理器的使用.png"; NSData *imageData = [NSData dataWithContentsOfFile: imagePath]; UIImage *image = [UIImage imageWithData: imageData]; //UIImage转换为NSData NSData *imageData1 = UIImagePNGRepresentation(image); 3.路径各个分段的查询 NSString * path = @"/Doc/work/diary"; NSArray * array = [path pathComponents];//文件的各个部分 NSString * lastName = [path lastPathComponent];//文件的最后一个部分 NSString * deleteName = [path stringByDeletingLastPathComponent];// 文件删除最后一个部分 NSString * appendName = [path stringByAppendingPathComponent:@"cy.jpg"];// 文件追加一个部分 NSLog(@"获得所有文件%@\n 获得最后文件%@\n 删除之后的名称%@\n追加名称%@\n",array,lastName,deleteName,appendName);
附上代码地址:
Demo地址
[end]
关于ios中文件管理器的使用介绍到这里。