对于一组数据array,平均分配成m组,要求每组的数量差不多。
思路:先对array数据进行排序,计算出可以分成n组。
然后将array数据分成n组已经排序好的数据集合。
将N组数据分正向赋值到M中,再反向赋值到M中,如此反复到结束。
代码:


1 public class Person
2 {
3 public Person(string Name, int Capability)
4 {
5 this._name = Name;
6 this._capability = Capability;
7 }
8 private string _name;
9 private int _capability;
10 /// <summary>
11 ///
12 /// </summary>
13 public string Name
14 {
15 get { return _name; }
16 set { _name = value; }
17 }
18 public int Capability
19 {
20 get { return _capability; }
21 set { _capability = value; }
22 }
23 }


1 static void Main(string[] args)
2 {
3 Person p1 = new Person("NAME1", 201);
4 Person p2 = new Person("NAME2", 233);
5 Person p3 = new Person("NAME3", 189);
6 Person p4 = new Person("NAME4", 198);
7 Person p5 = new Person("NAME5", 164);
8 Person p6 = new Person("NAME6", 181);
9 Person p7 = new Person("NAME7", 212);
10 Person p8 = new Person("NAME8", 205);
11 Person p9 = new Person("NAME9", 192);
12 Person p10 = new Person("NAME10", 241);
13 Person p11 = new Person("NAME11", 136);
14 Person p12 = new Person("NAME12", 201);
15 Person p13 = new Person("NAME13", 142);
16 Person p14 = new Person("NAME14", 127);
17 Person p15 = new Person("NAME15", 189);
18 Person p16 = new Person("NAME16", 221);
19 List<Person> personList = new List<Person>();
20 personList.Add(p1);
21 personList.Add(p2);
22 personList.Add(p3);
23 personList.Add(p4);
24 personList.Add(p5);
25 personList.Add(p6);
26 personList.Add(p7);
27 personList.Add(p8);
28 personList.Add(p9);
29 personList.Add(p10);
30 personList.Add(p11);
31 personList.Add(p12);
32 personList.Add(p13);
33 personList.Add(p14);
34 personList.Add(p15);
35 // personList.Add(p16);
36 Sort(personList, 3);
37 }
38
39 /// <summary>
40 /// 表示分组的作用
41 /// </summary>
42 /// <param name="personList">总人数列表</param>
43 /// <param name="GroupCount">分组数量</param>
44 static void Sort(List<Person> personList, int GroupCount)
45 {
46 if (GroupCount <= personList.Count)
47 {
48 //先排序
49 personList.Sort(Compare);
50 //可以取的次数
51 int QU = Convert.ToInt32(personList.Count / GroupCount) + 1;
52 List<List<Person>> pList = new List<List<Person>>();
53 //排序后的数量
54 List<List<Person>> sortList = new List<List<Person>>();
55 //分组排序后的数据
56 for (int j = 0; j < QU; j++)
57 {
58 List<Person> getPerson = new List<Person>();
59 for (int i = 0; i < GroupCount; i++)
60 {
61 int index = 0;
62 if (j == 0)
63 {
64 index = i + j;
65 }
66 else
67 {
68 index = (j) * GroupCount + i;
69 }
70 if (index < personList.Count)
71 {
72 getPerson.Add(personList[index]);
73 }
74 }
75 if (getPerson.Count > 0)
76 {
77 sortList.Add(getPerson);
78 }
79 }
80 //开始分配
81 for (int j = 0; j < GroupCount; j++)
82 {
83 List<Person> listPerson = new List<Person>();
84 bool sort = true;
85 for (int i = 0; i < sortList.Count; i++)
86 {
87 //正向分
88 if (sort)
89 {
90 if (j < sortList[i].Count)
91 {
92 listPerson.Add(sortList[i][j]);
93 }
94 sort = false;
95 }
96 else//反向分
97 {
98 if (GroupCount - (j + 1) < sortList[i].Count && GroupCount - (j + 1) >= 0)
99 {
100 listPerson.Add(sortList[i][GroupCount - (j + 1)]);
101 }
102 sort = true;
103 }
104 }
105 if (listPerson.Count > 0)
106 {
107 pList.Add(listPerson);
108 }
109 }
110 int m = 0;
111 foreach (List<Person> lp in pList)
112 {
113 m++;
114 Console.Write("当前第" + m.ToString() + "组\r\n");
115 int totalCa = 0;
116 foreach (Person pp in lp)
117 {
118 totalCa = totalCa + pp.Capability;
119 Console.Write("能力:" + pp.Capability + "名字:" + pp.Name + "\r\n");
120 }
121 Console.Write("总能力:" + totalCa.ToString() + "\r\n");
122 Console.Write("结束\r\n");
123 }
124 }
125 else
126 {
127 Console.Write("无法分组,分组数量大于总人数\r\n");
128 }
129 }
130 public static int Compare(Person oPerson, Person nPerson)
131 {
132 if (oPerson == null)
133 {
134 if (nPerson == null)
135 {
136 return 0;
137 }
138 else
139 {
140 return -1;
141 }
142 }
143 else
144 {
145 if (nPerson == null)
146 {
147 return 1;
148 }
149 else
150 {
151 if (oPerson.Capability > nPerson.Capability)
152 {
153 return 1;
154 }
155 else if (oPerson.Capability == nPerson.Capability)
156 {
157 return 0;
158 }
159 else
160 {
161 return -1;
162 }
163 }
164 }
165 }
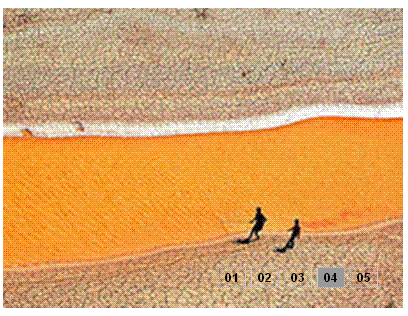