在第一篇开始篇中,我们需要完成的工作非常简单,就是定义一个数据源,并把它绑定到我们用户端进行展示。
效果如下图:
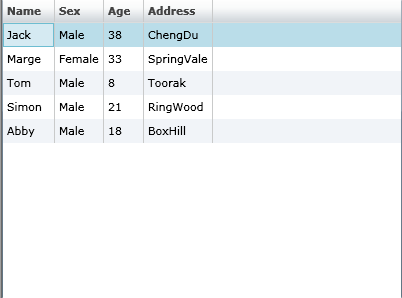
下面开始我们的工作:
1、新建一个Silverlight应用程序,命名为:SLApplicationExample。
2、新添加两个类,分别命名为 Person和People。
Person类的定义代码如下:


using System;
using System.Net;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Documents;
using System.Windows.Ink;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Animation;
using System.Windows.Shapes;
using System.ComponentModel; //因为要用到INotifyPropertyChanged接口
namespace SLApplicationExample
{
public class Person:INotifyPropertyChanged
{
private string _name;
private string _sex;
private int _age;
private string _address;
#region Constructors
public Person() { }
public Person(string NameStr, string SexStr, int AgeInt, string AddressStr)
{
this._name = NameStr;
this._sex = SexStr;
this._age = AgeInt;
this._address = AddressStr;
}
#endregion
#region Properties
public string Name
{
get { return _name; }
set
{
if (value == _name) return;
_name = value;
OnPropertyChanged("Name");
}
}
public string Sex
{
get { return _sex; }
set
{
if (value == _sex) return;
_sex = value;
OnPropertyChanged("Sex");
}
}
public int Age
{
get { return _age; }
set
{
if (value == _age) return;
_age = value;
OnPropertyChanged("Age");
}
}
public string Address
{
get { return _address; }
set
{
if (value == _address) return;
_address = value;
OnPropertyChanged("Address");
}
}
#endregion
#region INotifyPropertyChanged 接口实现
public event PropertyChangedEventHandler PropertyChanged;
protected void OnPropertyChanged(string name)
{
if (PropertyChanged != null)
PropertyChanged(this, new PropertyChangedEventArgs(name));
}
#endregion
}
}


using System;
using System.Net;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Documents;
using System.Windows.Ink;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Animation;
using System.Windows.Shapes;
using System.Collections.ObjectModel; //引入此空间,因为要用到ObservableCollection类
namespace SLApplicationExample
{
//ObservableCollection表示一个动态数据集合,在添加项、移除项或刷新整个列表时,此集合将提供通知。
public class People : ObservableCollection<Person>
{
public static People GetTestData()
{
People peopleData = new People()
{
new Person("Jack", "Male", 38, "ChengDu"),
new Person("Marge", "Female", 33, "SpringVale"),
new Person("Tom", "Male", 8, "Toorak"),
new Person("Simon", "Male", 21, "RingWood"),
new Person("Abby", "Male", 18, "BoxHill")
};
return peopleData;
}
}
}
<
UserControl xmlns:data
=
"
clr-namespace:System.Windows.Controls;assembly=System.Windows.Controls.Data
"
x:Class
=
"
SLApplicationExample.Page
"
xmlns = " http://schemas.microsoft.com/winfx/2006/xaml/presentation "
xmlns:x = " http://schemas.microsoft.com/winfx/2006/xaml "
Width = " 400 " Height = " 300 " >
< Grid x:Name = " LayoutRoot " Background = " White " >
< data:DataGrid x:Name = " dgPeople " />
</ Grid >
</ UserControl >
此用户界面非常简单,就是加入了一个DataGrid控件,我们将在会把我们定义的People类数据源绑定到此控件中进行展示。
xmlns = " http://schemas.microsoft.com/winfx/2006/xaml/presentation "
xmlns:x = " http://schemas.microsoft.com/winfx/2006/xaml "
Width = " 400 " Height = " 300 " >
< Grid x:Name = " LayoutRoot " Background = " White " >
< data:DataGrid x:Name = " dgPeople " />
</ Grid >
</ UserControl >
至此,应用程序如下图:
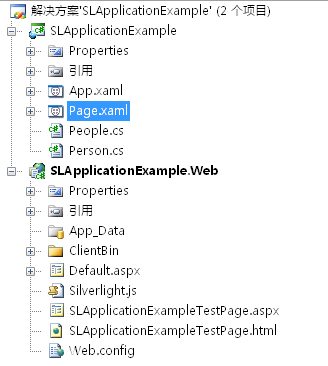
4、后台代码取数据并完成数据绑定,Page.xaml.cs代码如下:
using
System;
using System.Collections.Generic;
using System.Linq;
using System.Net;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Animation;
using System.Windows.Shapes;
namespace SLApplicationExample
{
public partial class Page : UserControl
{
People mypeople;
public Page()
{
InitializeComponent();
Loaded += new RoutedEventHandler(Page_Loaded);
}
private void Page_Loaded( object sender, RoutedEventArgs e)
{
mypeople = People.GetTestData();
this .dgPeople.ItemsSource = mypeople;
}
}
}
生成项目后运行程序即可看到我们前面展示的效果图。
using System.Collections.Generic;
using System.Linq;
using System.Net;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Animation;
using System.Windows.Shapes;
namespace SLApplicationExample
{
public partial class Page : UserControl
{
People mypeople;
public Page()
{
InitializeComponent();
Loaded += new RoutedEventHandler(Page_Loaded);
}
private void Page_Loaded( object sender, RoutedEventArgs e)
{
mypeople = People.GetTestData();
this .dgPeople.ItemsSource = mypeople;
}
}
}
前往:Silverlight学习笔记清单
本文程序在Silverlight2.0和VS2008环境中调试通过。本文参照了部分网络资料,希望能够抛砖引玉,大家共同学习。
(转载本文请注明出处)