第一步:配置web.xml,设置servlet的DispatcherServlet,设置
servlet启动的配置文件
spring_back
<servlet> <servlet-name>springmvc-back</servlet-name> <servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class> <init-param> <param-name>contextConfigLocation</param-name> <param-value>classpath:springmvc-back.xml</param-value> </init-param> </servlet> <servlet-mapping> <servlet-name>springmvc-back</servlet-name> <!-- <url-pattern>*.jsp</url-pattern> --> <url-pattern>*.do</url-pattern> </servlet-mapping>
第二步:配置springmvc-back.xml,设置
jsp视图解析类
<!-- springmvc 扫包 @Controller @Service .....--> <context:component-scan base-package="com.mybatis" use-default-filters="false"> <context:include-filter type="annotation" expression="org.springframework.stereotype.Controller"/> </context:component-scan> <!-- jsp视图 --> <bean id="jspViewResolver" class="org.springframework.web.servlet.view.InternalResourceViewResolver"> <property name="prefix" value="/"/> <property name="suffix" value=".jsp"/> </bean>
第三步:创建BaseQuery条件对象类,设置分页和查询可选字段属性


package com.mybatis.core.query; import java.io.Serializable; /** * 条件对象公用对象 * @author liangms */ public class BaseQuery implements Serializable{ private static final long serialVersionUID = 1L; //定义常量每页数 private static int DEFUALT_SIZE=10; //每页的记录数 protected Integer pageSize=10; //起始行 protected Integer startRow; //起始页码 protected Integer pageNo=1; //sql查询字段 protected String fields; public Integer getPageSize() { return pageSize; } public void setPageSize(int pageSize) { this.pageSize = pageSize; } public Integer getStartRow() { return startRow; } public void setStartRow(int startRow) { this.startRow = startRow; } public Integer getPageNo() { return pageNo; } /* *页码 * */ public void setPageNo(int pageNo) { this.pageNo = pageNo; this.startRow=(pageNo-1)*this.pageSize; } public String getFields() { return fields; } public void setFields(String fields) { this.fields = fields; } }
第四步:BrandQuery继承
BaseQuery
public
class
BrandQuery
extends
BaseQuery
第五步:BrandDao创建getBrandListCount,getBrandListWithPage接口
public int getBrandListCount(BrandQuery bQuery);
public List<Brand> getBrandListWithPage(BrandQuery bQuery);
第六步:
BrandDao.xml 配置
getBrandListWithPage
分页sql语句
<sql id="brandListLimit"> <if test="startRow != null"> limit #{startRow},#{pageSize} </if> </sql> <!-- 数据总数 --> <select id="getBrandListCount" parameterType="BrandQuery" resultType="int"> SELECT count(1) FROM bbs_brand <!-- <include refid="brandListLimit" /> --> </select> <select id="getBrandListWithPage" parameterType="BrandQuery" resultMap="brand"> <include refid="brandSelectorByFields" /> <include refid="brandWhere" /> <include refid="brandListOrder" /> <include refid="brandListLimit" /> </select>
第七步:
BrandServiceImpl实现
getBrandListWithPage接口服务
public Pagination getBrandListWithPage(BrandQuery bQuery) { Pagination p = new Pagination(bQuery.getPageNo(),bQuery.getPageSize(),brandDao.getBrandListCount(bQuery)); List<Brand> brands=brandDao.getBrandListWithPage(bQuery); p.setList(brands); return p; } public int getBrandListCount(BrandQuery bQuery) { return brandDao.getBrandListCount(bQuery); }
第八步:创建
BrandControl类


package com.mybatis.core.controller; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Controller; import org.springframework.ui.ModelMap; import org.springframework.web.bind.annotation.RequestMapping; import com.mybatis.core.query.BrandQuery; import com.mybatis.core.service.BrandService; import cn.itcast.common.page.Pagination; @Controller public class BrandController { @Autowired private BrandService brandService; @RequestMapping("/index.do") public String index(Integer pageNo, ModelMap model) { BrandQuery bQuery=new BrandQuery(); //设置过滤字段 //bQuery.setFields("id,name"); //设置like 条件 /*bQuery.setNameLike(true); bQuery.setName("莲");*/ //设置order bQuery.orderById(true); //每页三条记录 bQuery.setPageSize(3); if(pageNo==null) pageNo=1; //起始页为1 bQuery.setPageNo(pageNo.intValue()); Pagination pagination=brandService.getBrandListWithPage(bQuery); pagination.pageView("index.do", ""); model.addAttribute("pagination",pagination); return "index"; } }
第九步:实现index.jsp


<%@ page language="java" import="java.util.*" pageEncoding="UTF-8"%> <%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c"%> <%@ taglib uri="http://java.sun.com/jsp/jstl/fmt" prefix="fmt"%> <%@ taglib uri="http://java.sun.com/jsp/jstl/functions" prefix="fn"%> <html> <body> <table> <thead> <tr> <th>品牌id</th> <th>品牌名称</th> <th>品牌图片</th> <th>品牌描述</th> <th>排序</th> <th>是否可用</th> </tr> <tbody> <c:forEach items="${pagination.list}" var="entry"> <tr> <td>${entry.id}</td> <td>${entry.name}</td> <td>${entry.name}</td> <td>${entry.name}</td> <td>${entry.name}</td> <td>${entry.name}</td> </tr> </c:forEach> </tbody> </thead> <tbody> </tbody> </table> <span class="r inb_a page_b"> <c:forEach items="${pagination.pageView }" var="page"> ${page } </c:forEach> </span> </body> </html>
第十步:浏览器测试分页,http://localhost/MybatisTest/index.do
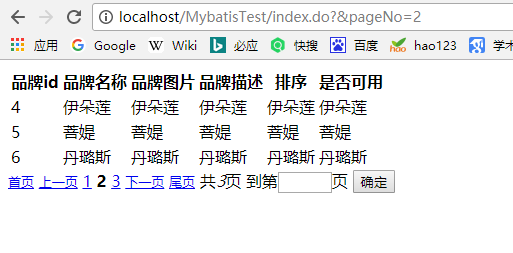