在.net反编译的时候或者是查看网页源码中都能看到类似"\u7f16\u7801\u8f6c\u6362"的编码 ,阅读起来很是不方便,于是从网上搜索编码解码工具,只搜到一个html版本的native2ascii,网页版本的感觉不是很方便,就自己做了一个winform的工具
设计界面如图
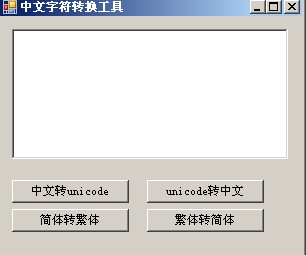
Code
1
using System;
2
using System.Collections.Generic;
3
using System.ComponentModel;
4
using System.Data;
5
using System.Drawing;
6
using System.Text;
7
using System.Windows.Forms;
8
using Microsoft.VisualBasic.Devices;
9
namespace native2ascii
10

{
11
public partial class Form1 : Form
12
{
13
public Form1()
14
{
15
InitializeComponent();
16
}
17
18
private void button1_Click(object sender, EventArgs e)
19
{
20
this.textBox1.Text = native2ascii2(textBox1.Text);
21
}
22
23
//native2ascii method
24
public static String native2ascii(String str)
25
{
26
int code;
27
char[] chars = str.ToCharArray();
28
StringBuilder sb = new StringBuilder(255);
29
for (int i = 0; i < chars.Length; i++)
30
{
31
char c = chars[i];
32
if (c > 255)
33
{
34
sb.Append("\\u");
35
code = (c >> 8);
36
string tmp = code.ToString("X");
37
if (tmp.Length == 1) sb.Append("0");
38
sb.Append(tmp);
39
code = (c & 0xFF);
40
tmp = code.ToString("X");
41
if (tmp.Length == 1) sb.Append("0");
42
sb.Append(tmp);
43
}
44
else
45
{
46
sb.Append(c);
47
}
48
49
}
50
return (sb.ToString());
51
}
52
public static String native2ascii2(String str)
53
{
54
string outStr = "";
55
if (!string.IsNullOrEmpty(str))
56
{
57
for (int i = 0; i < str.Length; i++)
58
{
59
//将中文字符转为10进制整数,然后转为16进制unicode字符
60
outStr += "\\u" + ((int)str[i]).ToString("x");
61
}
62
}
63
return outStr;
64
65
}
66
//end method
67
//ascii2native method
68
public static String ascii2native(String str)
69
{
70
string outStr = "";
71
if (!string.IsNullOrEmpty(str))
72
{
73
string[] strlist = str.Replace("\\", "").Split('u');
74
try
75
{
76
for (int i = 1; i < strlist.Length; i++)
77
{
78
//将unicode字符转为10进制整数,然后转为char中文字符
79
outStr += (char)int.Parse(strlist[i], System.Globalization.NumberStyles.HexNumber);
80
}
81
}
82
catch (FormatException ex)
83
{
84
outStr = ex.Message;
85
}
86
87
}
88
return outStr;
89
90
}
91
//end method
92
93
private void button2_Click(object sender, EventArgs e)
94
{
95
textBox1.Text = ascii2native(textBox1.Text);
96
97
}
98
99
100
/**//// <summary>
101
/// 转换为简体中文
102
/// </summary>
103
public static string ToSChinese(string str)
104
{
105
//return Strings.StrConv(str, VbStrConv.SimplifiedChinese, 0);
106
return Microsoft.VisualBasic.Strings.StrConv(str, Microsoft.VisualBasic.VbStrConv.SimplifiedChinese, 0);
107
}
108
109
/**//// <summary>
110
/// 转换为繁体中文
111
/// </summary>
112
public static string ToTChinese(string str)
113
{
114
return Microsoft.VisualBasic.Strings.StrConv(str, Microsoft.VisualBasic.VbStrConv.TraditionalChinese, 0);
115
}
116
117
private void button3_Click(object sender, EventArgs e)
118
{
119
textBox1.Text = ToTChinese(textBox1.Text);
120
}
121
122
private void button4_Click(object sender, EventArgs e)
123
{
124
textBox1.Text = ToSChinese(textBox1.Text);
125
}
126
127
128
}
129
}
注:代码中的native2ascii 与native2ascii2方法都是实现从中文转换unicode方法
简体转繁体与繁体转简体用到了VB中的函数因此需要引用Visual Basic
引用方法
右击 引用 选择 添加引用 在引用.net库选择 Microsoft Visual Basic
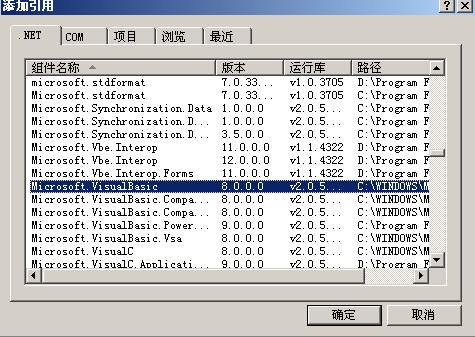