方法一:sql分页
思路:使用数据库进行分页
前端使用element-ui的分页组件,往后台传第几页的起始行offest 以及每页多少行pageSize,后台根据起始行数和每页的行数可以算出该页的最后一行,随后对数据库中的数据先进行排序,算出总共多少行,然后使用 limit 关键字进行限定查询所需要的数据,另外还要把总行数返回,不然前端页面没法显示总条数;(注:下方方法中的file_type为业务参数,请忽略)
vue:
<template>:
<el-pagination @size-change="handleSizeChange" @current-change="handleCurrentChange" :current-page="currentPage" :page-sizes="[10,20,30,40]" :page-size="pageSize" layout="total, sizes, prev, pager, next, jumper" :total="totalSize"> </el-pagination>
data:
//总数据条数
totalSize: 0,
//当前页码
currentPage: 1,
//每页条数
pageSize: 10,
//起始行数
offset: 0
methods:


//改变每页显示的数据条数 handleSizeChange(val) { //设置每页显示的条数 this.pageSize = val; if(!this.currentType) { this.$message({ showClose: true, message: '查询失败!', type: 'error' }); return; } this.acquireInfo(this.currentType); }, //改变页码 handleCurrentChange(val) { //offset为分页后的起始页码 this.offset = (val - 1) * this.pageSize; this.currentPage = val; if(!this.currentType) { this.$message({ showClose: true, message: '查询失败!', type: 'error' }); return; } this.acquireInfo(this.currentType); }, //往后台传offset,和每页多少行,返回的是当前页的pageSize行数据 let params = { "offset": this.offset, "pageSize": this.pageSize, "file_type": type //业务所需,ignore }; resourcesApi.queryFileList(params).then(res =>{ if(res.status === 200 && res.data) { this.fileInfoList = res.data; .....xxx..... } }).catch(error => { .............. }); }); },
api:
queryFileList(param) { return httpSender({ url: '/resources/documentList/getFileListByFileType', method: 'get', params:param }); }
spring boot:
controller:
/** * 根据文件类型查询文件 * @param offset * @param pageSize * @param file_type * @return */ @ApiOperation(value="/getFileListByFileType",notes = "根据文件类型获取文件列表") @GetMapping(value = "/getFileListByFileType") public List<Map> queryFileListByFileType(@RequestParam("offset") @ApiParam(value = "当前页显示的起始数据") int offset, @RequestParam("pageSize") @ApiParam(value = "每页显示的条数") int pageSize, @RequestParam("file_type") @ApiParam(value = "文件类型") String file_type) { try{ int startRow = offset; return documentService.queryFileListByFileType(startRow,pageSize, file_type); }catch(Exception e) { throw e; } }
iservice:
/** * 根据文件类型获取分页后的文件 * @param offest * @param pageSize * @param file_type * @return */ List<Map> queryFileListByFileType(int startRow, int pageSize, String file_type);
impl:
/** * 根据文件类型查询文件 * * @param startRow * @param pageSize * @param file_type * @return */ @Override public List<Map> queryFileListByFileType(int startRow, int pageSize, String file_type) { if (file_type == "" || file_type == null) { return null; } List<Map> list = resourceStorageMapper.queryFileListByFileType(startRow,pageSize, file_type); //获取该文件类型的总条数 int num = resourceStorageMapper.getCountByFileType(file_type); for (Map i : list) { i.put("num", num); } return list; }
mapper:
/** * 根据文件类型、分页条件查询文件列表 * @param startRow * @param endRow * @param file_type * @return */ @Select("SELECT a.file_name,DATE_FORMAT(a.update_datetime,'%Y-%m-%d %T') " + "as update_datetime,a.file_size,a.file_path FROM (SELECT * FROM " + "tab_resources_storage WHERE file_type = #{file_type}) a " + "Left JOIN tab_resources_filetype b ON a.file_type = b.code " + "ORDER BY a.update_datetime DESC LIMIT #{startRow}, #{pageSize};") List<Map> queryFileListByFileType(@Param("startRow") int startRow,@Param("pageSize") intpageSize,@Param("file_type") String file_type); /** * 获取该文件类型的总条数 * @param file_type * @return */ @Select("SELECT COUNT(*) FROM tab_resources_storage WHERE file_type = #{file_type}") int getCountByFileType(@Param("file_type") String file_type);
方法二:PageHelper 分页
思路:使用PageInfo 方法,一步到位,简单粗暴
前端使用element-ui的分页组件,往后台传当前页码currentPage以及每页显示的行数pageSize,后台使用 PageHelper 分页插件实现分页(注:下放代码中的queryCondition为业务数据,请忽略)
vue:
<template>:
<el-pagination @size-change="handleSizeChange" @current-change="handleCurrentChange" :current-page="currentPage" :page-sizes="[10,20,30,40]" :page-size="pageSize" layout="total, sizes, prev, pager, next, jumper" :total="totalSize"> </el-pagination>
data:
//总数据条数 totalSize: 0, //当前页码 currentPage: 1, //每页条数 pageSize: 10,
methods:
let params = { "currentPage": this.currentPage, "pageSize": this.pageSize, "queryCondition": this.dictfilterstr }; //发起请求 dictApi.getDict(params).then(res =>{ let data = res.data; if(data.total) { this.totalSize = data.total; } this.tableData = tData; } }).catch(error =>{})
api:
getDict(param) { return httpSender({ url: '/dict/list', method: 'get', params: param }); },
spring boot:
controller:
@ApiOperation(value = "获取项信息", notes = "获取项信息") @GetMapping(value = "/list") @ResponseBody public PageInfo dictList(@RequestParam("currentPage") @ApiParam(value = "当前页码") int currentPage, @RequestParam("pageSize") @ApiParam(value = "每页显示的条数") int pageSize, @RequestParam(value = "queryCondition", required = false) @ApiParam(value = "查询条件") String queryCondition){ if(StringUtils.isBlank(queryCondition)) { queryCondition = ""; } PageInfo page = null; try{ page = dictService.list(currentPage, pageSize, queryCondition); }catch (Exception e) { logger.error(e.getMessage(), e); throw new DictException(GET_DATA_INFO_FAILED); } return page; }
iservice:
** * 获取项信息并分页 * @param currentPage * @param * @param queryCondition * @return */ PageInfo list(int currentPage, int pageSize, String queryCondition);
impl:
/** * 查询所有字典项信息 * @return */ @Override public PageInfo list(int currentPage, int pageSize, String queryCondition) { PageInfo page = PageHelper.startPage(currentPage, pageSize).doSelectPageInfo(()-> dictMapper.selectDict(queryCondition)); return page; }
mapper:
/** * 根据查询条件查询列表 * * @param queryCondition * @return */ @Select("<script>" + "SELECT * FROM vsai_dict <if test='queryCondition != null and queryCondition != \"\"'> " + "WHERE dict_code LIKE concat(concat(\'%\',#{queryCondition}),\'%\')</if><if test='queryCondition == null or queryCondition == \"\"'>" + "WHERE pid = '0'</if> ORDER BY update_time DESC " + "</script>") List<DictEntity> selectDict(@Param("queryCondition") String queryCondition);
page的返回值:
其中list是查询的参数列表;total为总条数;pages为总页数;
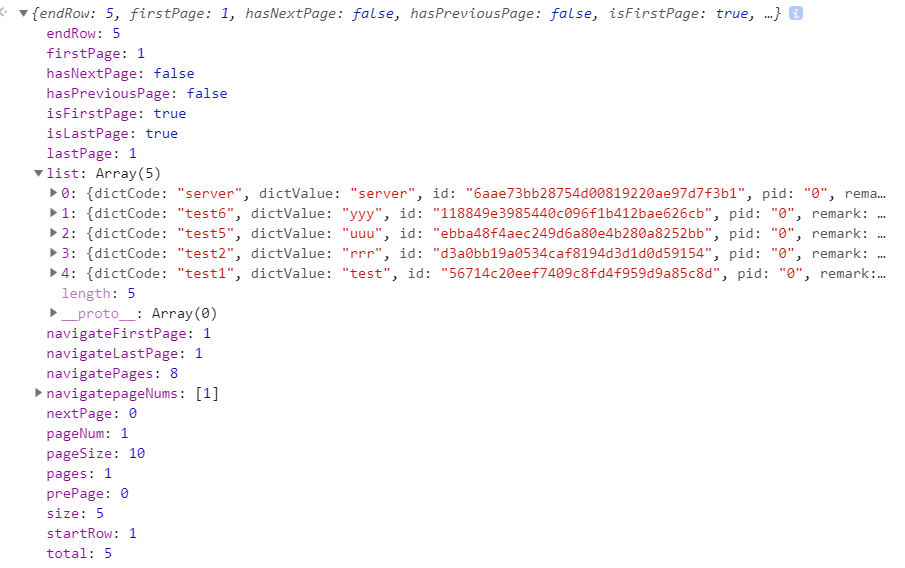
效果图: