ok,继续。现在我有了一个窗口,我要在窗口上画张图片。有很多办法可以在winform上来张图片,但是这次要用DirectX来做。
Step1:添加对Directx程序集的引用(在.net 卡片下,如果没有 C:\WINDOWS\Microsoft.NET\DirectX for Managed Code\ 找找。
Microsoft.DirectX
Microsoft.DirectX.Direct3D
Microsoft.DirectX.Direct3DX
先添这三个就够了,Dirext3DX是个工具集,有很多个版本,这东西现在还用不到。
Step2:用到的东西,使用DirectX来完成这次绘图我需要三个主要的对象 Device(设备),Sprite(精灵),Texture(纹理)。
简单点理解,Device是“墙”,Sprite是“笔”,Texture是“油漆或图纸”,现在可以开始画了。
初始化“墙面”(Device)
DirextX9 Doc:A Microsoft Direct3D device is the rendering component of Direct3D。因此做任何绘制工作前必须创建该对象。
它的创建方法:Device(Int32,DeviceType,IntPtr,CreateFlags,PresentParameters[]) ,看下面(来自DirectX9 sample)。
//
初始化显示设备
public
bool
InitializeGraphics()

{
try

{
//参数集
PresentParameters presentParams = new PresentParameters();
presentParams.Windowed = true;
presentParams.SwapEffect = SwapEffect.Discard;

device = new Device(0, DeviceType.Hardware, this, CreateFlags.SoftwareVertexProcessing, presentParams);
return true;
}
catch (DirectXException)

{
return false;
}
}
参数一(int32),为显示设备的索引值,当你有多快显示卡的时候通过该参数来确定使用哪块。
参数二(DeviceType),加速的类型。其他的选项先不管,先用Hardware来完成。
参数三(CreateFlags),一个标签,先不去管它。
参数四(PresentParameters[])显示参数集,通过这个参数的集合我们可以得到我们想要的窗体显示效果。Windowed表示是否为窗口显示,否为全屏。SwapEffect,交换效果,回头再说,照抄。
Step3: 创建一个图纸,这里需要一个纹理(Texture)
DirectX9 Doc:Textures are a powerful tool for creating realism in computer-generated 3-D images. Texture是个强大的工具,他来处理所有的图像素材。
它的重要方法就是Load(载入),使用一个TextureLoader对象来载入各种来源的素材,我现在需要一个来自图片文件的素材,看代码。
Texture _texture
=
TextureLoader.FromFile(Device,fileName)
Step4:用Sprite把这个纹理画到Device上
Sprite 可以将一些素材绘制出来,目前只需要知道这些,我要使用它的Draw2D方法来绘制纹理,看代码。
sprite
=
new
Sprite(device);

sprite.Begin(SpriteFlags.AlphaBlend);
sprite.Draw2D(p.Texture,
new
PointF(
0
,
0
),
0
,
new
PointF(
0
,
0
), Color.FromArgb(
255
,
255
,
255
,
255
));
device.Transform.World
=
Matrix.Identity;
sprite.End();
关于device.Transform 是用于变形设置的,要知道在2维的现实屏中显示3D的效果它可使不可缺少的,现在这个设置是告诉它别动(不变)。运行一下,bingo!(图)
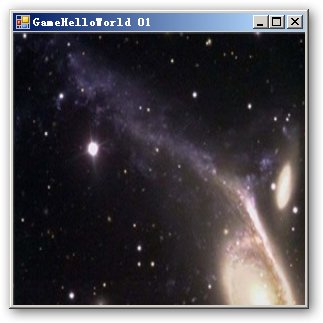
看起来不错哦。现在,我需要让这些图像动起来,让他们丰富起来...
整体的代码如下:
using
System.Collections.Generic;
using
System.ComponentModel;
using
System.Data;
using
System.Drawing;
using
System.Text;
using
System.Windows.Forms;

using
Microsoft.DirectX;
using
Microsoft.DirectX.Direct3D;

namespace
GameHello01

{
public class GameWindow : Form

{
//需要的东西
Device device = null;
Sprite sprite = null;
Texture texture = null;

public GameWindow()

{
this.Text = "GameHelloWorld 01";
this.Paint += new PaintEventHandler(GameWindow_Paint);
}


-- 初始化 Device --#region -- 初始化 Device --
public bool InitializeGraphics()

{
try

{
PresentParameters presentParams = new PresentParameters();
presentParams.Windowed = true;
presentParams.SwapEffect = SwapEffect.Discard;
device = new Device(0, DeviceType.Hardware, this, CreateFlags.SoftwareVertexProcessing, presentParams);
return true;
}
catch (DirectXException)

{
return false;
}
}
#endregion


-- 初始化 Texture --#region -- 初始化 Texture --
public bool InitializeTexture()

{
try

{
texture = TextureLoader.FromFile(device, Application.StartupPath + "\\back.jpg");
return true;
}
catch (DirectXException)

{
return false;
}
}
#endregion


-- 初始化 Sprite --#region -- 初始化 Sprite --
public bool InitializeSprite()

{
try

{
sprite = new Sprite(device);
return true;
}
catch (DirectXException)

{
return false;
}
}
#endregion


-- 画图 Render() --#region -- 画图 Render() --
void Render()

{
if (device == null || sprite == null || texture == null)
return;

device.Clear(ClearFlags.Target, System.Drawing.Color.Black, 1.0f, 0);
device.BeginScene();

sprite.Begin(SpriteFlags.AlphaBlend);

sprite.Draw2D(texture, new PointF(0, 0), 0, new PointF(0, 0), Color.FromArgb(255, 255, 255, 255));
device.Transform.World = Matrix.Identity;

sprite.End();

device.EndScene();
device.Present();
}
#endregion

void GameWindow_Paint(object sender, PaintEventArgs e)

{
this.Render();
}

static void Main()

{
using (GameWindow window = new GameWindow())

{
if (!window.InitializeGraphics())

{
MessageBox.Show("Direct3D初始化错误!");
return;
}
if (!window.InitializeTexture())

{
MessageBox.Show("Texture初始化错误!");
return;
}
if (!window.InitializeSprite())

{
MessageBox.Show("Sprite初始化错误!");
return;
}

window.Show();

while (window.Created)

{
window.Render();
Application.DoEvents();
}
}
}
}