演示效果:
打开txt文件
输入文字,保存
选择保存地址
生成文件
源代码:
View Code
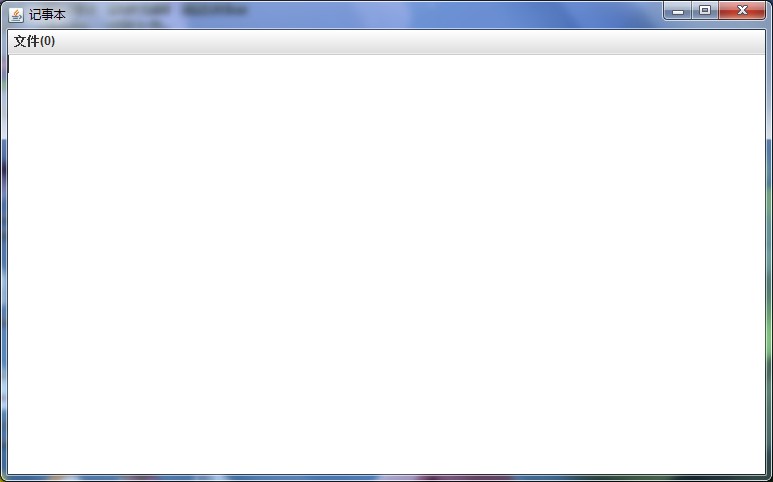
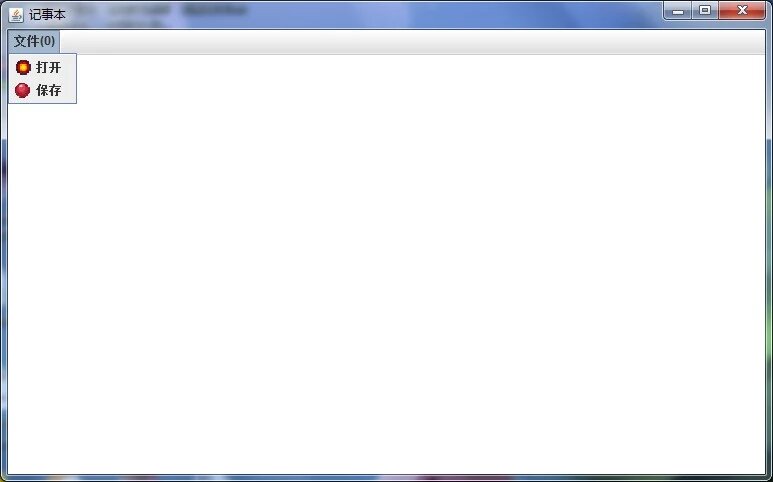
打开txt文件
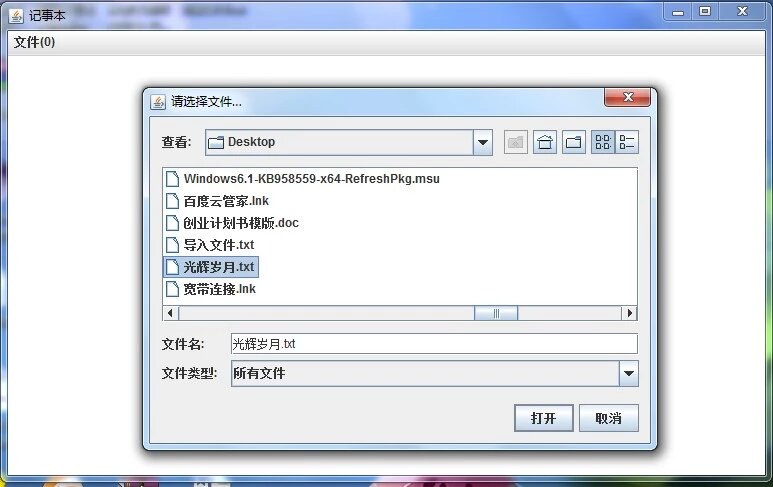
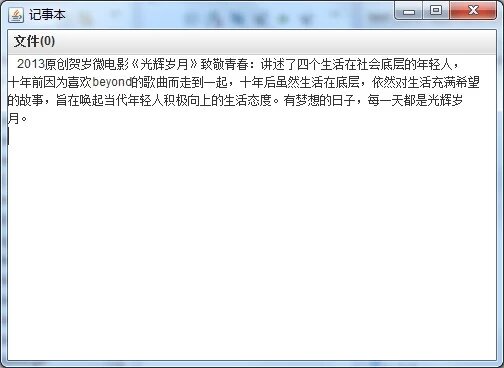
输入文字,保存
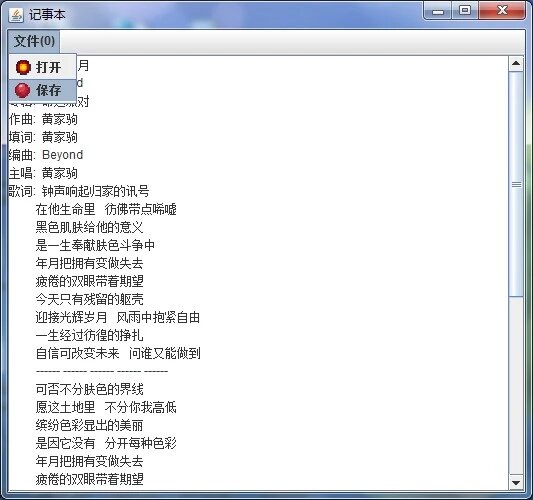
选择保存地址
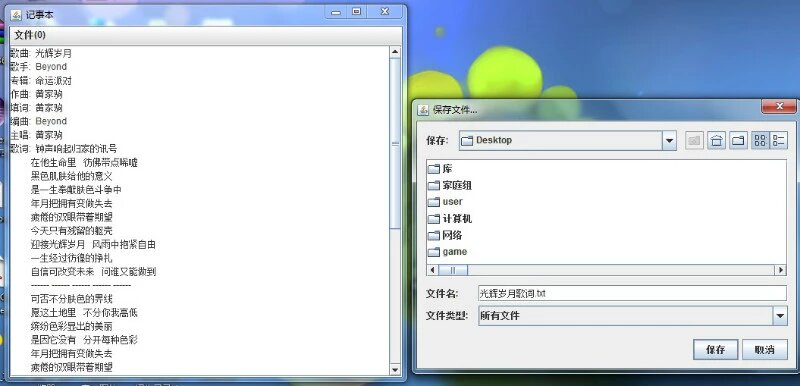
生成文件
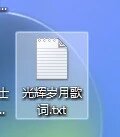
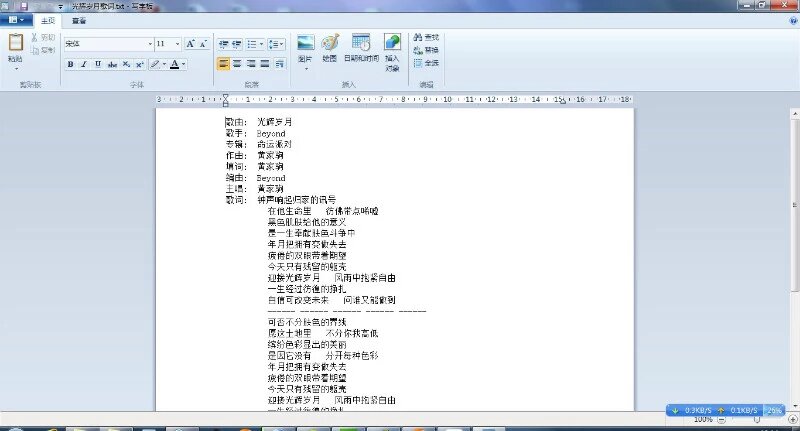
源代码:


1 package io; 2 import java.io.*; 3 import java.awt.*; 4 import java.awt.event.ActionEvent; 5 import java.awt.event.ActionListener; 6 7 import javax.swing.*; 8 9 10 11 public class text extends JFrame implements ActionListener{ 12 13 /** 14 * @param args 15 */ 16 //需要的组件 17 //文本框 18 JTextArea jta1=null; 19 //菜单条 20 JMenuBar jmb1=null; 21 22 //菜单 23 24 JMenu jm1=null; 25 //菜单项 26 JMenuItem jmi1=null; 27 JMenuItem jmi2=null; 28 29 JScrollPane jsp1=null; 30 31 public static void main(String[] args) { 32 // TODO Auto-generated method stub 33 34 text show=new text(); 35 36 } 37 38 public text() 39 { 40 //文本框 41 jta1 =new JTextArea(); 42 43 44 45 //菜单条 46 jmb1=new JMenuBar(); 47 48 //菜单 49 50 jm1=new JMenu("文件(0)"); 51 //助记符 52 jm1.setMnemonic('F'); 53 54 //菜单项 55 jmi1=new JMenuItem("打开",new ImageIcon("src/10.gif")); 56 57 //注册监听 58 59 jmi1.addActionListener(this); 60 jmi1.setActionCommand("open"); 61 62 63 64 65 jmi2=new JMenuItem("保存",new ImageIcon("src/9.gif")); 66 67 //注册监听 68 jmi2.addActionListener(this); 69 jmi2.setActionCommand("save"); 70 71 72 this.setJMenuBar(jmb1); 73 jmb1.add(jm1); 74 jm1.add(jmi1); 75 jm1.add(jmi2); 76 77 78 jsp1=new JScrollPane(jta1); 79 80 this.add(jsp1); 81 82 83 this.setTitle("记事本"); 84 this.setSize(400, 300); 85 this.setLocation(100, 100); 86 this.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); 87 this.setVisible(true); 88 } 89 90 @Override 91 public void actionPerformed(ActionEvent arg0) { 92 // TODO Auto-generated method stub 93 //判断 94 95 if(arg0.getActionCommand().equals("open")) 96 { 97 //文件选择组件 98 JFileChooser jfc1=new JFileChooser(); 99 //设置名字 100 jfc1.setDialogTitle("请选择文件…"); 101 102 //设置组件.null表示默认方式 103 jfc1.showOpenDialog(null); 104 105 106 jfc1.setVisible(true); 107 //得到用户选择的文件路径 108 109 String filename1=jfc1.getSelectedFile().getAbsolutePath(); 110 111 //读取 112 FileReader fr1=null; 113 114 BufferedReader br1=null; 115 116 try { 117 fr1=new FileReader(filename1); 118 br1=new BufferedReader(fr1); 119 120 //读取信息,显示到文本框jta1 121 122 String s=""; 123 String all=""; 124 while((s=br1.readLine())!=null) 125 { 126 all+=s+"\r\n"; 127 //jta1.setText(s+"\r\n"); 128 } 129 130 jta1.setText(all); 131 132 } catch (FileNotFoundException e) { 133 // TODO Auto-generated catch block 134 e.printStackTrace(); 135 } catch (IOException e) { 136 // TODO Auto-generated catch block 137 e.printStackTrace(); 138 } 139 finally{ 140 try { 141 fr1.close(); 142 } catch (IOException e) { 143 // TODO Auto-generated catch block 144 e.printStackTrace(); 145 } 146 try { 147 br1.close(); 148 } catch (IOException e) { 149 // TODO Auto-generated catch block 150 e.printStackTrace(); 151 } 152 } 153 } 154 155 156 else if(arg0.getActionCommand().equals("save")) 157 { 158 //文件选择组件 159 JFileChooser jfc1=new JFileChooser(); 160 //设置名字 161 jfc1.setDialogTitle("保存文件…"); 162 163 //设置组件.null表示默认方式 164 jfc1.showSaveDialog(null); 165 166 167 jfc1.setVisible(true); 168 //得到用户选择的文件路径 169 170 //得到用户选择的文件路径 171 172 String filename1=jfc1.getSelectedFile().getAbsolutePath(); 173 174 //写入 175 FileWriter fw1=null; 176 177 BufferedWriter bw1=null; 178 179 try { 180 fw1=new FileWriter(filename1); 181 182 183 //写入信息,显示到文本框jta1 184 185 186 187 fw1.write(this.jta1.getText()); 188 189 } catch (FileNotFoundException e) { 190 // TODO Auto-generated catch block 191 e.printStackTrace(); 192 } catch (IOException e) { 193 // TODO Auto-generated catch block 194 e.printStackTrace(); 195 } 196 finally{ 197 try { 198 fw1.close(); 199 } catch (IOException e) { 200 // TODO Auto-generated catch block 201 e.printStackTrace(); 202 } 203 try { 204 bw1.close(); 205 } catch (IOException e) { 206 // TODO Auto-generated catch block 207 e.printStackTrace(); 208 } 209 } 210 211 } 212 } 213 214 } 215