1.Automation Test Intruduction
Framework
2.Build Test Environment
2.1Add New Project
2.1.1Add Related Jar files:(build path>Labraries)
selenium-server-standalone-3.4.0.jar
2.1.2Add required projects:(build path>projects)
2.1.3Install TestNG plugin
Help>Eclipse marketplace>search 'TestNG' and install
Help>Install New software>Input http://beust.com/eclipse to install
3.Test Scripts
Test Execution:
TestType->Build Test Environment--->TEst Execution
Test Execution Result Analysis:
Idea-->Retest-->log-->analysis result
4.Start up Selenium Web Driver
WebDriver driver= new InternetExplorerDriver();
WebDriver driver= new FirefoxDriver();
WebDriver driver= new ChromeDriver();
Navigate To URL
driver.get("http://www.baidu.com");
driver.navigate().to("http://www.baidu.com");
5.Design Test Script
TestNg Test step:
OPeration Flow: Validatuin Point:
STEP1 Verify(){}
STEP2
STEP3
****
If exception is cathched, it is better to handle it immediately
If the test points are parallel, throw excetion at end of all check point
6.Find Element
Tool:firebug
firebug is a development of the plug-in under web browser Mozilla Firefox. It sets the HTML view and edit, and Javascript console, network status monitor at a whole,
and is tool that to develop Javascript,CSS,HTML and Ajax
获取元素的方法
1. by.name()
<button id="gbqfba" aria-label="Google Search" name="btnK" class="gbqfba"><span id="gbqfsa">Google Search</span></button>
复制代码
1 public class SearchButtonByName {
2 public static void main(String[] args){
3 WebDriver driver = new FirefoxDriver();
4 driver.get("http://www.forexample.com");
5 WebElement searchBox = driver.findElement(By.name("btnK"));
6 searchBox.click();
7 }
8 }
2.by.id()
<button id="gbqfba" aria-label="Google Search" name="btnK" class="gbqfba"><span id="gbqfsa">Google Search</span></button>
public class SearchButtonById {
public static void main(String[] args){
WebDriver driver = new FirefoxDriver();
driver.get("http://www.forexample.com");
WebElement searchBox = driver.findElement(By.id("gbqfba"));
searchBox.click();
}
}
3.By.tagName()
这个方法搜索到的元素通常不止一个,所以一般结合findElements方法来使用。
public class SearchPageByTagName{
public static void main(String[] args){
WebDriver driver = new FirefoxDriver();
driver.get("http://www.forexample.com");
List<WebElement> buttons = driver.findElements(By.tagName("button"));
System.out.println(buttons.size()); //打印出button的个数
}
}
如果无法准确地得到我们想要的元素就需要结合bype属性才能过滤出来想要的元素
public class SearchElementsByTagName{
2
3 public static void main(String[] args){
4
5 WebDriver driver = new FirefoxDriver();
6
7 driver.get("http://www.forexample.com");
8
9 List<WebElement> allInputs = driver.findElements(By.tagName("input"));
10
11 //只打印所有文本框的值
12
13 for(WebElement e: allInputs){
14
15 if (e.getAttribute(“type”).equals(“text”)){
16
17 System.out.println(e.getText().toString()); //打印出每个文本框里的值
18
19 }
20
21 }
22
23 }
24
25 }
4.By.className()
className属性是利用元素的CSS样式表所引用的 伪类名称 来进行元素的查找
对于任何HTML页面的元素来说,一般程序员或者页面设计师会给元素直接赋予一个样式属性或者利用css文件的伪类来定义元素样式,使元素在页面上显示时能够更加美观
1 .buttonStyle{
2
3 width: 50px;
4
5 height: 50px;
6
7 border-radius: 50%;
8
9 margin: 0% 2%;
10
11 }
<button name="sampleBtnName" id="sampleBtnId" class="buttonStyle">I'm Button</button>
1 public class SearchElementsByClassName{
2
3 public static void main(String[] args){
4
5 WebDriver driver = new FirefoxDriver();
6
7 driver.get("http://www.forexample.com");
8
9 WebElement searchBox = driver.findElement(By.className("buttonStyle"));
10
11 searchBox.sendKeys("Hello, world");
12
13 }
14
15 }
使用className来进行元素定位时有时会碰到一个元素指定了若干个class属性值的复合样式, 如 <button id="J_sidebar_login" class="btn btn_big btn_submit" type="submit">登录</button>。
这个button元素指定了三个不同的CSS伪类名作为它的样式属性值,此时就必须结合后面要介绍的cssSelector方法来定位
5.By.linkText()
通过超文本链接上的文字信息来定位
<a href="/intl/en/about.html">About Google</a>
1 public class SearchElementsByLinkText{
2
3 public static void main(String[] args){
4
5 WebDriver driver = new FirefoxDriver();
6
7 driver.get("http://www.forexample.com");
8
9 WebElement aboutLink = driver.findElement(By.linkText("About Google"));
10
11 aboutLink.click();
12
13 }
14
15 }
6.By.partialLinkTest()
*一般为<a /a>,如果标签中含有其他元素不能采用LinkTest(),可以用partialLinkTest()
当不能准确知道超链接上的文本信息或者只通过一些关键字进行匹配,通过使用这个方法来通过 部分链接文字 进行匹配
1 public class SearchElementsByPartialLinkText{
2
3 public static void main(String[] args){
4
5 WebDriver driver = new FirefoxDriver();
6
7 driver.get("http://www.forexample.com");
8
9 WebElement aboutLink = driver.findElement(By.partialLinkText("About"));
10
11 aboutLink.click();
12
13 }
14
15 }
7. By.xpath()
Expression | Description |
nodename | Select all child nodes of this node |
/ | Select from the root node |
// | Select the nodes in the document from the current node of the matching selection, regardless of their location |
. | Select the current node |
.. | Select the parent node of the current node |
@ | Select attribute |
绝对路径
xpath以/开头时表示让xpath解析引擎从文档的根节点开始解析, 表示寻找父节点的直接子节点
xpath以//开头时表示xpath引擎从文档的任意符合的元素节点开始进行解析,表示寻找父节点下任意符合条件的子节点
a.用Contains关键字
driver.findElement(By.xpath(“//a[contains(@href, ‘logout’)]”));//寻找herf属性值中包含logout的元素a
b.start-with
driver.findElement(By.xpath(“//a[starts-with(@rel, ‘nofo’)]));
c.Text关键字
driver.findElement(By.xpath(“//*[text()=’退出’]));
driver.findElement(By.xpath(“//a[contains(text(), ’退出’)]));
''//input[contains(@class,'inputID']''查找class里inputid
"//div[(@class,' ')]""//span[(@class), ' ']"
利用属性定位
"//input[@id='su']" 查找ID为'su'的input
利用层级关系
"//form[@class='by_s_ipt_wr'/span/input]" 需要找//form[@class='by_s_ipt_wr',根据父亲//form[@class='by_s_ipt_wr'/span,爷爷//form[@class='by_s_ipt_wr'/span/input
利用逻辑运算符
"//input[@id='kw' and @class='su']/span/input"
//tr/td/span--->//tr//span
//tbody/tr[1]--->第一行
//tbody/tr[last()]--->最后一行
//tbody/tr[postion()<3]--->前两行
//tbody/tr[@class=""]//td[@id=""]//a[text()=""]---->tbody tr[class=""] td[id=""] a[text()=""]
//a[title=""]/ancestor::tr[id=""]/label/i--->
8.By.cssSelector()
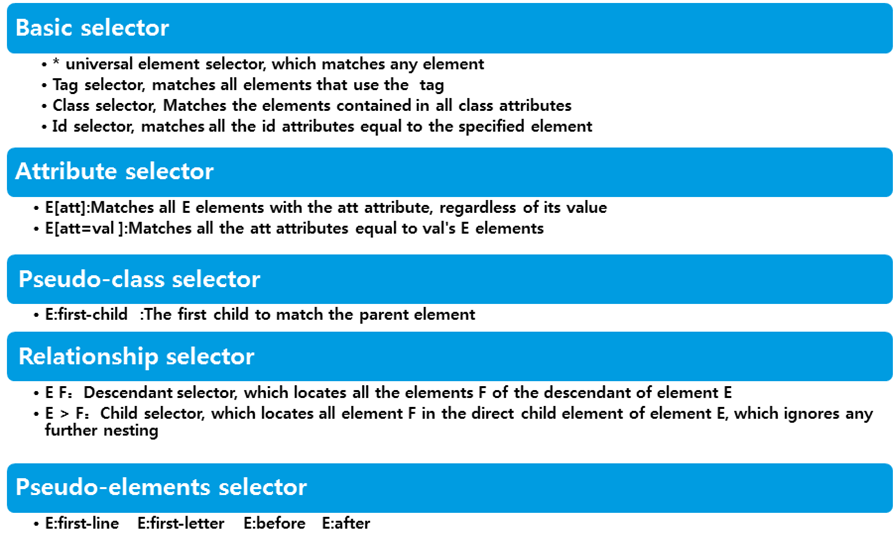
通过class定位
<span class='bg s_ipt_wr'>
find_element_by_CSS_selector(".s_ipt")
通过ID定位
("#kw")
tr:first-child
tr:first-of-type
tr:nth-child(2)
:nth-of-type(2)-->找到第二行的节点
:last-of-type--->最后一个节点
tr td
tr>td
定位id为flrs的div元素,可以写成:#flrs
定位id为flrs下的A元素,可以写成: #flrs > a
定位id为flrs下的href属性值为/forexample/about.html的元素,可以写成: #flrs > a[href=”/forexample/about.html”]
如果需要指定多个属性值时,可以逐一加在后面,如#flrs > input[name=”username”][type=”text”]。
匹配一个有id属性,并且id属性是以”id_prefix_”开头的超链接元素:a[id^='id_prefix_']
匹配一个有id属性,并且id属性是以”_id_sufix”结尾的超链接元素:a[id$='_id_sufix']
匹配一个有id属性,并且id属性中包含”id_pattern”字符的超链接元素:a[id*='id_pattern']
<div class="formdiv">
<form name="fnfn">
<input name="username" type="text"></input>
<input name="password" type="text"></input>
<input name="continue" type="button"></input>
<input name="cancel" type="button"></input>
<input value="SYS123456" name="vid" type="text">
<input value="ks10cf6d6" name="cid" type="text">
</form>
<div class="subdiv">
<ul id="recordlist">
<p>Heading</p>
<li>Cat</li>
<li>Dog</li>
<li>Car</li>
<li>Goat</li>
</ul>
</div>
</div>
Locator matching
div.formdiv <div class="formdiv">
#recordlist
ul#recordlist <ul id="recordlist">
div.subdiv p
div.subdiv>ul>p <p>Heding</p>
form+div <divclass="subdiv">
p+li P~li <li>Cat</li> The former is 1,the latter is 4
form> input[name=username] <input name="username">
input[name$=id][value^=SYS] <input value="SYS123456" name="vid" type="hidden">
input:not([name$=id][value^=SYS]) <input name="username" type="text"></input>
li:contains('Goa') <li>Goat</li>
li:not(contains('Goa')) <li>cat</li>
eg:
<button id="ext-eng-1026" class="x-right-button">OK</button>
css=button.x-right-button:contains(OK"")
9.DOM
7.Opreration
7.1Findelement:
driver.findelement(by.id(" "));
element.clear();
element.sendKeys(value);
8.切换Frame
public void switchTo_FrameDyf(String frame) throws Exception {
try {
testDriver.switchTo().frame(frame);
Thread.sleep(5000);
Logger.i(TAG, "The " + frame + " frame was loaded");
} catch (Exception e) {
Logger.e(TAG, "The " + frame + " frame don't exist");
}
}
9.切换Window
public void switchTo_WindowDyf(String expectedWindowTitle) {
try {
for (String windowName : testDriver.getWindowHandles()) {
if (testDriver.switchTo().window(windowName).getTitle().contains(expectedWindowTitle)) {
testDriver.switchTo().window(windowName);
Logger.i(TAG, "Move to Window: " + testDriver.switchTo().window(windowName).getTitle());
break;
}
}
Thread.sleep(5000);
} catch (Exception e) {
// TODO: handle exception
Logger.e(TAG, "Can not find this Window");
}
}
10.Wait
public WebElement waitElementDisplayedDyf(By by) throws Exception {
StopWatch time = new StopWatch();
time.start();
while (true) {
try {
WebElement element = testDriver.findElement(by);
if (element.isDisplayed()) {
return element;
} else if (time.getTime() > TimeOut) {
Logger.e(TAG, "Waitting for element: " + by.toString() + " timeout!");
throw new Exception("Waitting for element is timeout.");
}
} catch (Exception e) {
if (time.getTime() > TimeOut) {
throw e;
}
}
}
}
public void waitElementsPresentDyf(By by) throws Exception {
try {
new WebDriverWait(testDriver, 10).until(ExpectedConditions.visibilityOfElementLocated(by));
} catch (Exception e) {
Logger.i(TAG, e.getMessage() + "\n");
}
}
public List<WebElement> waitElementsDisplayedDyf(By by) throws Exception {
StopWatch time = new StopWatch();
time.start();
while (true) {
try {
List<WebElement> elements = testDriver.findElements(by);
if (elements != null) {
return elements;
} else if (time.getTime() > TimeOut) {
Logger.e(TAG, "Waitting for element: " + by.toString() + " timeout!");
throw new Exception("Waitting for element is timeout.");
}
} catch (Exception e) {
if (time.getTime() > TimeOut) {
throw e;
}
}
}
}
public boolean WaitforJQueryProcessingDyf() {
boolean JQcondition = false;
try {
new WebDriverWait(testDriver,10){
}.until(new ExpectedCondition<Boolean>(){
public Boolean apply(WebDriver driverObject){
return (Boolean) ((JavascriptExecutor) testDriver).executeScript("return jQuery.active == 0");
}
});
JQcondition =(Boolean) ((JavascriptExecutor) testDriver).executeScript("return jQuery.active == 0");
} catch(Exception e){
Logger.e(TAG, e.getMessage());
}
return JQcondition;
}
public boolean WaitDataLoadingDyf(final By selector) {
boolean loaded = false;
WebDriverWait wait = new WebDriverWait(testDriver,10);
try {
ExpectedCondition<Boolean> ec= new ExpectedCondition<Boolean>(){
public Boolean apply(WebDriver driver){
try{
String status= testDriver.findElement(selector).getAttribute("style");
Logger.i(TAG, "The data loading status is: " +(status.contains("none")? "Loading finised" : "loading"));
return (status.contains("none"));
}catch(NoSuchElementException e){
return true;
}
}
};
loaded= wait.until(ec);
}catch(Exception e){
Logger.e(TAG, "This page is loading");
throw e;
}
return loaded;
}
7.JS 操作
7.1Click事件
public void Jsclick_buttonDyf(WebElement button) {
try {
JavascriptExecutor js = (JavascriptExecutor) testDriver;
js.executeScript("arguments[0].click()", button);
} catch (Exception e) {
Logger.e(TAG, "This button click Failed");
}
}