运行效果:
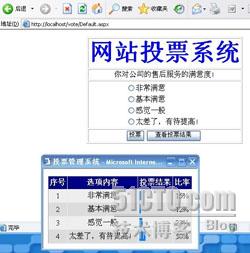
前台代码:Default.aspx
------------------------------------------------------------------------------------------------------------------------------------------------------
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Default.aspx.cs" Inherits="_Default" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "
http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd
">
<html xmlns="
http://www.w3.org/1999/xhtml
">
<head runat="server">
<title>投票管理系统</title>
<style type="text/css">
.style2
{
text-align: center;
color: #0000FF;
font-size: 40pt;
height: 46px;
font-weight: bold;
}
.style3
{
text-align: center;
height: 18px;
}
.style4
{
text-align: center;
height: 20px;
}
.style5
{
text-align: center;
height: 25px;
}
</style>
</head>
<body>
<form id="form1" runat="server">
<table align="center" border ="1">
<tr>
<td class="style2">
网站投票系统</td>
</tr>
<tr>
<td class="style3">
<asp:Label ID="lblTitle" runat="server"></asp:Label>
</td>
</tr>
<tr>
<td class="style4" align="left">
<asp:RadioButtonList ID="rbtnItem" runat="server">
</asp:RadioButtonList>
</td>
</tr>
<tr>
<td class="style5">
<asp:Button ID="btnVote" runat="server" Text="投票"
/>
<asp:Button ID="btnShow" runat="server" Text="查看投票结果" />
</td>
</tr>
</table>
<br />
</form>
</body>
</html>
<head runat="server">
<title>投票管理系统</title>
<style type="text/css">
.style2
{
text-align: center;
color: #0000FF;
font-size: 40pt;
height: 46px;
font-weight: bold;
}
.style3
{
text-align: center;
height: 18px;
}
.style4
{
text-align: center;
height: 20px;
}
.style5
{
text-align: center;
height: 25px;
}
</style>
</head>
<body>
<form id="form1" runat="server">
<table align="center" border ="1">
<tr>
<td class="style2">
网站投票系统</td>
</tr>
<tr>
<td class="style3">
<asp:Label ID="lblTitle" runat="server"></asp:Label>
</td>
</tr>
<tr>
<td class="style4" align="left">
<asp:RadioButtonList ID="rbtnItem" runat="server">
</asp:RadioButtonList>
</td>
</tr>
<tr>
<td class="style5">
<asp:Button ID="btnVote" runat="server" Text="投票"
/>
<asp:Button ID="btnShow" runat="server" Text="查看投票结果" />
</td>
</tr>
</table>
<br />
</form>
</body>
</html>
前台代码:ShowResult.aspx
----------------------------------------------------------------------------------------------------------------------------------------------
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="ShowResult.aspx.cs" Inherits="ShowResult" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "
http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd
">
<html xmlns="
http://www.w3.org/1999/xhtml
">
<head runat="server">
<title>投票管理系统</title>
</head>
<body>
<form id="form1" runat="server">
<div style ="text-align :center ">
<asp:GridView ID="GVResult" runat="server" AutoGenerateColumns="False"
CellPadding="3" GridLines="Vertical" Height="145px" BackColor="White"
BorderColor="#999999" BorderStyle="None" BorderWidth="1px" onrowdatabound="GVResult_RowDataBound"
>
<FooterStyle BackColor="#CCCCCC" ForeColor="Black" />
<RowStyle BorderStyle="Double" HorizontalAlign="Center"
VerticalAlign="Middle" BackColor="#EEEEEE" ForeColor="Black" />
<Columns>
<asp:BoundField DataField="voteItemID" HeaderText="序号"/>
<asp:BoundField DataField="voteItemName" HeaderText="选项内容" />
<asp:TemplateField HeaderText ="投票结果">
<ItemTemplate >
</ItemTemplate>
<ItemStyle HorizontalAlign="Left" />
</asp:TemplateField>
<asp:BoundField HeaderText="比率" />
</Columns>
<PagerStyle BackColor="#999999" ForeColor="Black" HorizontalAlign="Center" />
<SelectedRowStyle BackColor="#008A8C" Font-Bold="True" ForeColor="White" />
<HeaderStyle BackColor="#000084" Font-Bold="True" ForeColor="White" />
<AlternatingRowStyle BackColor="#DCDCDC" />
</asp:GridView>
</div>
</form>
</body>
</html>
<head runat="server">
<title>投票管理系统</title>
</head>
<body>
<form id="form1" runat="server">
<div style ="text-align :center ">
<asp:GridView ID="GVResult" runat="server" AutoGenerateColumns="False"
CellPadding="3" GridLines="Vertical" Height="145px" BackColor="White"
BorderColor="#999999" BorderStyle="None" BorderWidth="1px" onrowdatabound="GVResult_RowDataBound"
>
<FooterStyle BackColor="#CCCCCC" ForeColor="Black" />
<RowStyle BorderStyle="Double" HorizontalAlign="Center"
VerticalAlign="Middle" BackColor="#EEEEEE" ForeColor="Black" />
<Columns>
<asp:BoundField DataField="voteItemID" HeaderText="序号"/>
<asp:BoundField DataField="voteItemName" HeaderText="选项内容" />
<asp:TemplateField HeaderText ="投票结果">
<ItemTemplate >
</ItemTemplate>
<ItemStyle HorizontalAlign="Left" />
</asp:TemplateField>
<asp:BoundField HeaderText="比率" />
</Columns>
<PagerStyle BackColor="#999999" ForeColor="Black" HorizontalAlign="Center" />
<SelectedRowStyle BackColor="#008A8C" Font-Bold="True" ForeColor="White" />
<HeaderStyle BackColor="#000084" Font-Bold="True" ForeColor="White" />
<AlternatingRowStyle BackColor="#DCDCDC" />
</asp:GridView>
</div>
</form>
</body>
</html>
后台代码:Default.aspx
--------------------------------------------------------------------------------------------------------------------------------------------------
using System;
using System.Configuration;
using System.Data;
using System.Linq;
using System.Web;
using System.Web.Security;
using System.Web.UI;
using System.Web.UI.HtmlControls;
using System.Web.UI.WebControls;
using System.Web.UI.WebControls.WebParts;
using System.Xml.Linq;
using System.Data.SqlClient;
using System.Configuration;
using System.Data;
using System.Linq;
using System.Web;
using System.Web.Security;
using System.Web.UI;
using System.Web.UI.HtmlControls;
using System.Web.UI.WebControls;
using System.Web.UI.WebControls.WebParts;
using System.Xml.Linq;
using System.Data.SqlClient;
public partial class _Default : System.Web.UI.Page
{
int voteID = 1;
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
{
//连接
SqlConnection con = new SqlConnection(System.Configuration.ConfigurationSettings.AppSettings["con"]);
con.Open();
{
int voteID = 1;
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
{
//连接
SqlConnection con = new SqlConnection(System.Configuration.ConfigurationSettings.AppSettings["con"]);
con.Open();
//显示投票的标题
SqlCommand Titlecmd = new SqlCommand("select voteTitle from voteTitle where voteID=" + voteID, con);
this.lblTitle.Text = Convert.ToString(Titlecmd.ExecuteScalar());
SqlCommand Titlecmd = new SqlCommand("select voteTitle from voteTitle where voteID=" + voteID, con);
this.lblTitle.Text = Convert.ToString(Titlecmd.ExecuteScalar());
//显示投票的内容
SqlCommand Itemcmd = new SqlCommand("select voteItemID,voteItemName from voteItem where voteID=" + voteID, con);
SqlDataReader sdr = Itemcmd.ExecuteReader();
this.rbtnItem.DataSource = sdr;
this.rbtnItem.DataValueField = "voteItemID";
this.rbtnItem.DataTextField = "voteItemName";
this.rbtnItem.DataBind();
sdr.Close();
con.Close();
}
SqlCommand Itemcmd = new SqlCommand("select voteItemID,voteItemName from voteItem where voteID=" + voteID, con);
SqlDataReader sdr = Itemcmd.ExecuteReader();
this.rbtnItem.DataSource = sdr;
this.rbtnItem.DataValueField = "voteItemID";
this.rbtnItem.DataTextField = "voteItemName";
this.rbtnItem.DataBind();
sdr.Close();
con.Close();
}
}
protected void btnVote_Click(object sender, EventArgs e)
{
//发出请求的远程主机的IP地址
string UserIP = Request.UserHostAddress.ToString();
Response.Write("IP是:" + UserIP.ToString() + "<br>");
//获取当前时间
Response.Write("现在时间是:" + DateTime.Now + "<br><br>");
//选择的项
Response.Write("已选择第" + Convert.ToInt32(Convert.ToInt32(this.rbtnItem.SelectedIndex) + 1) + "项");
Response.Write("已选择第" + Convert.ToInt32(Convert.ToInt32(this.rbtnItem.SelectedIndex) + 1) + "项");
//连接DB
SqlConnection con = new SqlConnection(System.Configuration.ConfigurationSettings.AppSettings["con"]);
con.Open();
SqlConnection con = new SqlConnection(System.Configuration.ConfigurationSettings.AppSettings["con"]);
con.Open();
SqlCommand IPcmd = new SqlCommand("select count(userIP) from voteUser where userIP='" + UserIP.ToString() + "'", con);
int count = Convert.ToInt32(IPcmd.ExecuteScalar()) - 1;
//未投票情况
if (count < 0)
{
SqlCommand insertcmd = new SqlCommand("insert into voteUser values('" + UserIP.ToString() + "','" + Request .UserHostName .ToString() + "','" + DateTime .Now + "')", con);
insertcmd.ExecuteNonQuery();
SqlCommand updatacmd = new SqlCommand("update voteItem set voteItemCount = voteItemCount + 1 where voteID=" + voteID + "and voteItemID=" +Convert.ToInt32(Convert.ToInt32(this.rbtnItem.SelectedIndex) + 1),con);
updatacmd.ExecuteNonQuery();
Response .Write ("<script>alert('投票成功,谢谢您的参与!')</script>");
}
//已投过票情况
else
{
SqlCommand loginTimecmd = new SqlCommand("select loginTime from voteUser where userIP='" + UserIP.ToString() + "'", con);
DateTime dbTime=Convert .ToDateTime (loginTimecmd .ExecuteScalar ());
Response.Write("<br>数据库当前时间是:" + dbTime + "<br>");
//通过失效时间,在月份上加数,以防止同一IP地址重复投票
Response.Write("<br>数据库失效时间是:" + dbTime.AddMonths(5) + "<br>");
if(dbTime.AddMonths(5) > DateTime.Now)
{
Response.Write("<script>alert('你已投过票了,谢谢您的参与!')</script>");
}
else
{
try
{
SqlCommand updatacmd = new SqlCommand("update voteItem set voteItemCount = voteItemCount + 1 where voteID=" + voteID + "and voteItemID=" + Convert.ToInt32(Convert.ToInt32(this.rbtnItem.SelectedIndex) + 1), con);
updatacmd.ExecuteNonQuery();
Response.Write("<script>alert('投票成功,谢谢您的参与!')</script>");
}
catch
{
Response.Write("<script>alert("+ e.ToString () +")</script>");
int count = Convert.ToInt32(IPcmd.ExecuteScalar()) - 1;
//未投票情况
if (count < 0)
{
SqlCommand insertcmd = new SqlCommand("insert into voteUser values('" + UserIP.ToString() + "','" + Request .UserHostName .ToString() + "','" + DateTime .Now + "')", con);
insertcmd.ExecuteNonQuery();
SqlCommand updatacmd = new SqlCommand("update voteItem set voteItemCount = voteItemCount + 1 where voteID=" + voteID + "and voteItemID=" +Convert.ToInt32(Convert.ToInt32(this.rbtnItem.SelectedIndex) + 1),con);
updatacmd.ExecuteNonQuery();
Response .Write ("<script>alert('投票成功,谢谢您的参与!')</script>");
}
//已投过票情况
else
{
SqlCommand loginTimecmd = new SqlCommand("select loginTime from voteUser where userIP='" + UserIP.ToString() + "'", con);
DateTime dbTime=Convert .ToDateTime (loginTimecmd .ExecuteScalar ());
Response.Write("<br>数据库当前时间是:" + dbTime + "<br>");
//通过失效时间,在月份上加数,以防止同一IP地址重复投票
Response.Write("<br>数据库失效时间是:" + dbTime.AddMonths(5) + "<br>");
if(dbTime.AddMonths(5) > DateTime.Now)
{
Response.Write("<script>alert('你已投过票了,谢谢您的参与!')</script>");
}
else
{
try
{
SqlCommand updatacmd = new SqlCommand("update voteItem set voteItemCount = voteItemCount + 1 where voteID=" + voteID + "and voteItemID=" + Convert.ToInt32(Convert.ToInt32(this.rbtnItem.SelectedIndex) + 1), con);
updatacmd.ExecuteNonQuery();
Response.Write("<script>alert('投票成功,谢谢您的参与!')</script>");
}
catch
{
Response.Write("<script>alert("+ e.ToString () +")</script>");
}
}
}
}
}
}
protected void btnShow_Click(object sender, EventArgs e)
{
//Response.Write ("<script>window.open('ShowResult.aspx','投票管理系统','height=170,width=330')</script>");
Response.Redirect("ShowResult.aspx");
}
}
后台代码:ShowResult.aspx
----------------------------------------------------------------------------------------------------------------------------------------------------------
using System;
using System.Collections;
using System.Configuration;
using System.Data;
using System.Linq;
using System.Web;
using System.Web.Security;
using System.Web.UI;
using System.Web.UI.HtmlControls;
using System.Web.UI.WebControls;
using System.Web.UI.WebControls.WebParts;
using System.Xml.Linq;
using System.Data.SqlClient;
using System.Collections;
using System.Configuration;
using System.Data;
using System.Linq;
using System.Web;
using System.Web.Security;
using System.Web.UI;
using System.Web.UI.HtmlControls;
using System.Web.UI.WebControls;
using System.Web.UI.WebControls.WebParts;
using System.Xml.Linq;
using System.Data.SqlClient;
public partial class ShowResult : System.Web.UI.Page
{ int voteID=1;
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
{
//连接DB
SqlConnection con = new SqlConnection(System.Configuration.ConfigurationSettings.AppSettings["con"]);
con.Open();
{ int voteID=1;
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
{
//连接DB
SqlConnection con = new SqlConnection(System.Configuration.ConfigurationSettings.AppSettings["con"]);
con.Open();
//显示投票结果数据
SqlDataAdapter sda = new SqlDataAdapter();
sda.SelectCommand = new SqlCommand("select voteItemID,voteItemName,voteItemCount from voteItem where voteID=" + voteID, con);
DataSet ds = new DataSet();
sda.Fill(ds, "voteItem");
GVResult.DataSource = ds.Tables[0];
GVResult.DataBind();
}
SqlDataAdapter sda = new SqlDataAdapter();
sda.SelectCommand = new SqlCommand("select voteItemID,voteItemName,voteItemCount from voteItem where voteID=" + voteID, con);
DataSet ds = new DataSet();
sda.Fill(ds, "voteItem");
GVResult.DataSource = ds.Tables[0];
GVResult.DataBind();
}
}
public float ItemCount()
{
SqlConnection con = new SqlConnection(System.Configuration.ConfigurationSettings.AppSettings["con"]);
con.Open();
//计算总票数
SqlCommand CountCmd = new SqlCommand("select sum(voteItemCount) from voteItem where voteID=" + voteID, con);
float ALLcount = Convert.ToInt32(CountCmd.ExecuteScalar());
return ALLcount;
}
protected void GVResult_RowDataBound(object sender, GridViewRowEventArgs e)
{
if(e.Row.RowType == DataControlRowType .DataRow )
{
DataRowView drv =(DataRowView) e.Row.DataItem;
//找出某列的各个值
int voteItemCount = Convert .ToInt32 ( drv.Row["voteItemCount"]);
//求出各项目所占的比率
float ALLcount = ItemCount();
int percent =Convert .ToInt32 (( voteItemCount / ALLcount)*100);
//显示所占的比率图片
Image voteItemImage = new Image();
voteItemImage.ImageUrl = "~/pic/vote.jpg";
voteItemImage.Width = Unit.Parse(voteItemCount + "px");
voteItemImage.Height = Unit.Parse("20px");
e.Row.Cells[2].Controls.Add(voteItemImage);
//显示比率值
e.Row.Cells[3].Text = percent.ToString ()+"%";
}
}
}
}
}
转载于:https://blog.51cto.com/djw8088/324004