1
using
System;
2
using
System.Web;
3
using
System.Web.Services;
4
using
System.Web.Services.Protocols;
5
6
[WebService(Namespace
=
"
http://tempuri.org/
"
)]
7
[WebServiceBinding(ConformsTo
=
WsiProfiles.BasicProfile1_1)]
8
public
class
Service : System.Web.Services.WebService
9
{
10
public Service ()
{
11
12
//Uncomment the following line if using designed components
13
//InitializeComponent();
14
}
15
16
[WebMethod]
17
public string SayHelloTo(string someBody)
{
18
return "Hello "+someBody;
19
}
20
[WebMethod]
21
public string SayWelcomeTo(string someBody)
22
{
23
return "Welcome, " + someBody;
24
}
25
26
}
27

2

3

4

5

6

7

8

9



10



11

12

13

14

15

16

17



18

19

20

21

22



23

24

25

26

27

2. 创建性能计数器,这里用一个简单的 cs 文件替代了 installer 文件,只需用csc命令讲下面的代码编译成exe文件,然后运行exe文件就可以在系统中创建自己的性能计数器。注意:我们并不需要做总访问次数和时间的除法运算来得到每秒的访问次数,PerformanceCounterType枚举类型专门负责这个,详细的信息请查阅msdn.
1
using
System;
2
using
System.Diagnostics;
3
4
namespace
ZZQ.Net.Demo
5
{
6
public class Installer
7
{
8
9
public static void Main()
10
{
11
try
12
{
13
if (PerformanceCounterCategory.Exists("MyPerfCategory"))
14
{
15
PerformanceCounterCategory.Delete("MyPerfCategory");
16
}
17
18
19
CounterCreationDataCollection counterDatas = new CounterCreationDataCollection();
20
21
counterDatas.Add(new CounterCreationData("calls total", "number of service calls",
22
PerformanceCounterType.NumberOfItems64));
23
counterDatas.Add(new CounterCreationData("calls / sec", "number of service calls per second.",
24
PerformanceCounterType.RateOfCountsPerSecond64));
25
counterDatas.Add(new CounterCreationData("errors total",
26
"number of errors returned form service to the client.",
27
PerformanceCounterType.NumberOfItems64));
28
counterDatas.Add(new CounterCreationData("errors / sec",
29
"number of errors returned form service to the client per second.",
30
PerformanceCounterType.RateOfCountsPerSecond64));
31
counterDatas.Add(new CounterCreationData("average processing time",
32
"average call processing time in milliseconds.",
33
PerformanceCounterType.AverageCount64));
34
counterDatas.Add(new CounterCreationData("average processing time base", "",
35
PerformanceCounterType.AverageBase));
36
counterDatas.Add(new CounterCreationData("Processing time latency",
37
"Processing time in milliseconds.",
38
PerformanceCounterType.NumberOfItems32));
39
40
PerformanceCounterCategory.Create("MyPerfCategory", "It is just for demonstration purpose!", PerformanceCounterCategoryType.MultiInstance, counterDatas);
41
42
43
}
44
catch (Exception ex)
45
{
46
Console.WriteLine("Error occurred: " + ex.ToString());
47
}
48
Console.ReadLine();
49
}
50
}
51
}
52

2

3

4

5



6

7



8

9

10



11

12



13

14



15

16

17

18

19

20

21

22

23

24

25

26

27

28

29

30

31

32

33

34

35

36

37

38

39

40

41

42

43

44

45



46

47

48

49

50

51

52

3. 客户端的代码使用两个 timer 在 tick 事件中生成的随机数做循环调用模拟当前的访问量,并且在每次调用的时候更新计数器,代码很简单。
1
using
System;
2
using
System.Collections.Generic;
3
using
System.ComponentModel;
4
using
System.Data;
5
using
System.Drawing;
6
using
System.Text;
7
using
System.Windows.Forms;
8
using
PerformanceCounterDemo.localhost;
9
using
ZZQ.Net.Demo;
10
11
12
namespace
PerformanceCounterDemo
13
{
14
public partial class Demo : Form
15
{
16
17
public Demo()
18
{
19
InitializeComponent();
20
}
21
22
private void btnHello_Click(object sender, EventArgs e)
23
{
24
timer1.Enabled = true;
25
}
26
27
28
29
private void btnWelcome_Click(object sender, EventArgs e)
30
{
31
timer2.Enabled = true;
32
}
33
34
private void btnStop_Click(object sender, EventArgs e)
35
{
36
37
timer1.Enabled = false;
38
timer2.Enabled = false;
39
}
40
private void timer1_Tick(object sender, EventArgs e)
41
{
42
Random r = new Random();
43
int counter = r.Next(30);
44
CallHello(counter);
45
46
}
47
48
private void timer2_Tick(object sender, EventArgs e)
49
{
50
Random r = new Random();
51
int counter = r.Next(30);
52
CallWelcome(counter);
53
}
54
55
56
private void CallHello(int counter)
57
{
58
Service s = new Service();
59
string strResult = string.Empty;
60
for (int i = 0; i < counter; i++)
61
{
62
DateTime start = DateTime.Now;
63
strResult += "The " + i.ToString() + " time,return value is " + s.SayHelloTo("zzq" + i.ToString()) + "\r\n";
64
txtHello.Text = strResult;
65
TimeSpan span = DateTime.Now.Subtract(start);
66
PensPerfCounterManager.UpdatePerfCounters("MyPerfCategory", "SayHelloTo", span, true);
67
68
}
69
}
70
private void CallWelcome(int counter)
71
{
72
Service s = new Service();
73
string strResult = string.Empty;
74
75
for (int i = 0; i < counter; i++)
76
{
77
DateTime start = DateTime.Now;
78
strResult += "The " + i.ToString() + " time,return value is " + s.SayHelloTo("zzq" + i.ToString()) + "\r\n";
79
txtWelcome.Text = strResult;
80
PensPerfCounterManager.UpdatePerfCounters("MyPerfCategory", "SayWelcomeTo", start, true);
81
}
82
}
83
84
}
85
}

2

3

4

5

6

7

8

9

10

11

12

13



14

15



16

17

18



19

20

21

22

23



24

25

26

27

28

29

30



31

32

33

34

35



36

37

38

39

40

41



42

43

44

45

46

47

48

49



50

51

52

53

54

55

56

57



58

59

60

61



62

63

64

65

66

67

68

69

70

71



72

73

74

75

76



77

78

79

80

81

82

83

84

85

我的代码中UpdatePerfCounters方法的最后一个参数总是传的true,因为这两个web method太简单了,基本不会出错。^_^。实际用的时候会针对是否出现异常来决定是否更新和错误相关的计数器。
4.运行客户端的程序然后在运行里键入perfmon打开性能计数器管理窗口,找到MyPerfCategory性能对象,并且添加所有所有计数器的所有实例。
客户端演示效果图:
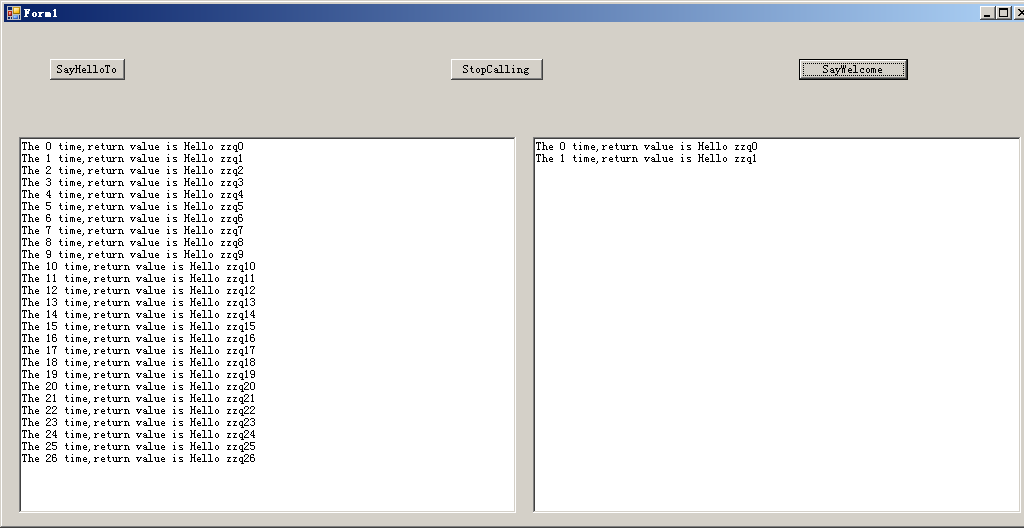
性能计数器效果图:
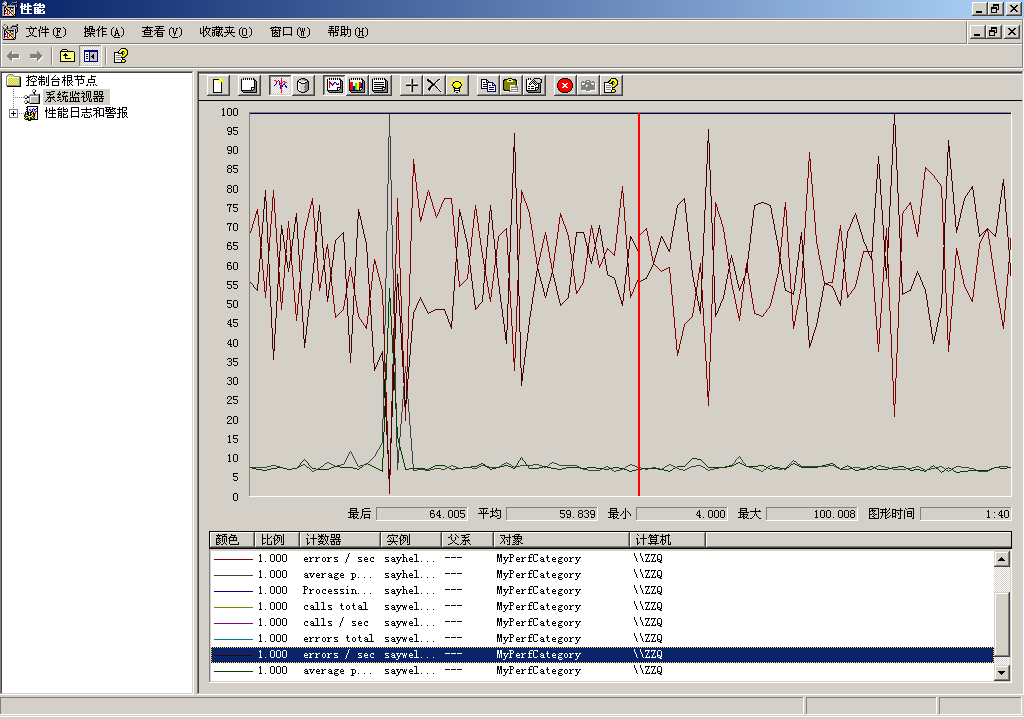