1.效果
调用阿里云的接口 去定位IP地址
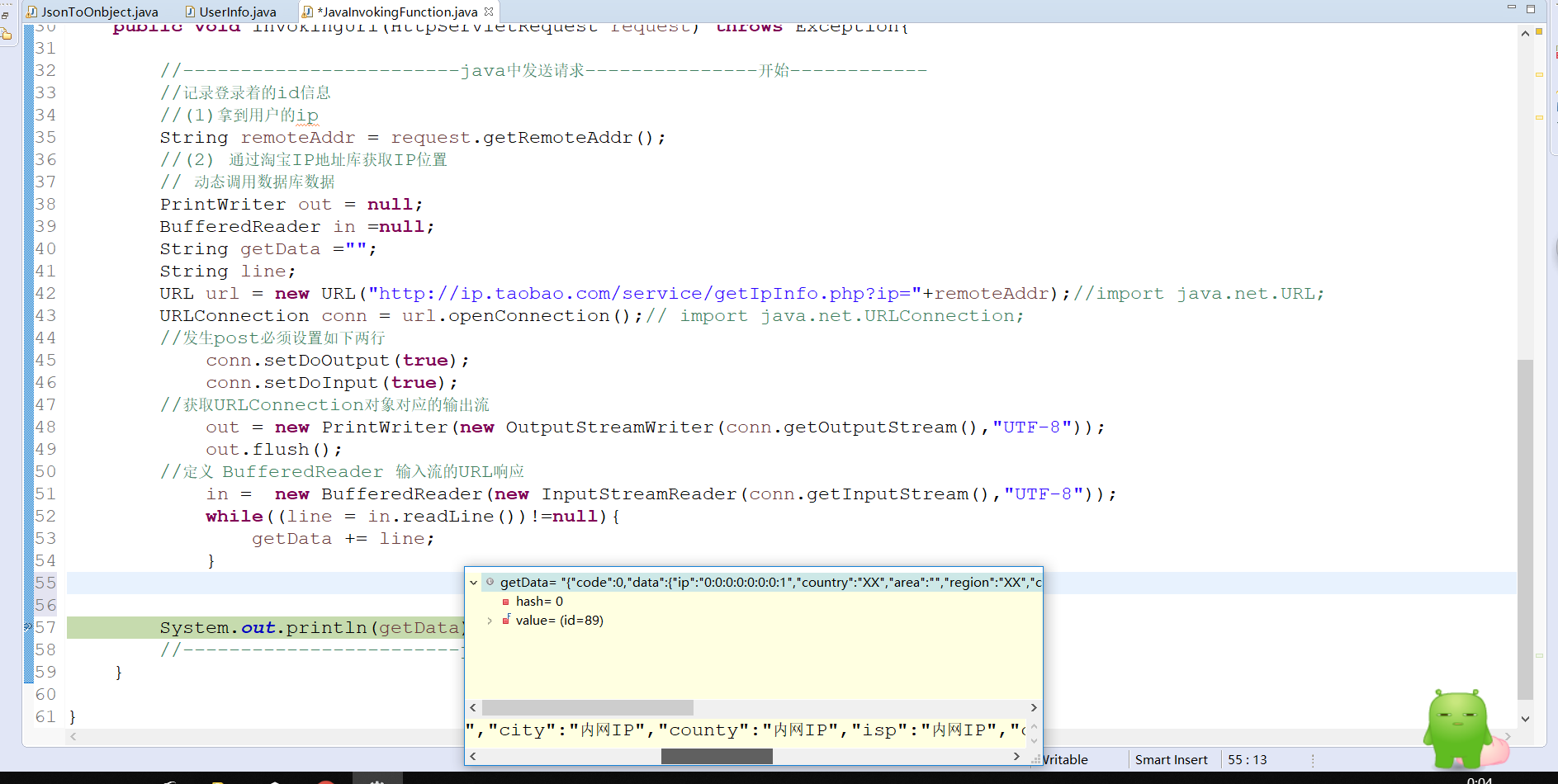
2. 代码
/** * 1. Java中远程调用方法 * http://localhost:8080/mavenssm20180519/invokingUrl.action * @Title: invokingUrl * @Description: * @return void * @throws Exception * @throws @date 2018年7月22日 下午11:58:58 */ @RequestMapping("/invokingUrl.action") public void invokingUrl(HttpServletRequest request) throws Exception{ //------------------------java中发送请求---------------开始------------ //记录登录着的id信息 //(1)拿到用户的ip String remoteAddr = request.getRemoteAddr(); //(2) 通过淘宝IP地址库获取IP位置 // 动态调用数据库数据 PrintWriter out = null; BufferedReader in =null; String getData =""; String line; URL url = new URL("http://ip.taobao.com/service/getIpInfo.php?ip="+remoteAddr);//import java.net.URL; URLConnection conn = url.openConnection();// import java.net.URLConnection; //发生post必须设置如下两行 conn.setDoOutput(true); conn.setDoInput(true); //获取URLConnection对象对应的输出流 out = new PrintWriter(new OutputStreamWriter(conn.getOutputStream(),"UTF-8")); out.flush(); //定义 BufferedReader 输入流的URL响应 in = new BufferedReader(new InputStreamReader(conn.getInputStream(),"UTF-8")); while((line = in.readLine())!=null){ getData += line; } System.out.println(getData); //------------------------java中发送请求---------------结束------------ } |
2. 在java发送get/post的请求
2.1 utils 类
package cn.mg.kindeditor.utils; import java.io.BufferedReader; import java.io.InputStreamReader; import java.io.OutputStreamWriter; import java.io.PrintWriter; import java.net.URL; import java.net.URLConnection; public class sendRequestUtils { /** * 1.post方式发送请求 * @param requestUrl * @return * @throws Exception */ public static String sendPostRequest(String requestUrl) throws Exception{ //------------------------java中发送请求---------------开始------------ //记录登录着的id信息 //(1)拿到用户的ip //(2) 通过淘宝IP地址库获取IP位置 // 动态调用数据库数据 PrintWriter out = null; BufferedReader in =null; String getData =""; String line; URL url = new URL(requestUrl);//import java.net.URL; URLConnection conn = url.openConnection();// import java.net.URLConnection; //发生post必须设置如下两行 conn.setDoOutput(true); conn.setDoInput(true); //获取URLConnection对象对应的输出流 out = new PrintWriter(new OutputStreamWriter(conn.getOutputStream(),"UTF-8")); out.flush(); //定义 BufferedReader 输入流的URL响应 in = new BufferedReader(new InputStreamReader(conn.getInputStream(),"UTF-8")); while((line = in.readLine())!=null){ getData += line; } System.out.println(getData); return getData; } /** * 2.get方式发送请求 * @param url * @return */ public static String sendGetRequest(String url){ String result = ""; String line; StringBuffer sb=new StringBuffer(); BufferedReader in = null; try { URL realUrl = new URL(url); // 打开和URL之间的连接 URLConnection conn = realUrl.openConnection(); // 设置通用的请求属性 设置请求格式 conn.setRequestProperty("contentType", "utf-8"); conn.setRequestProperty("content-type", "application/x-www-form-urlencoded"); //设置超时时间 conn.setConnectTimeout(60); conn.setReadTimeout(60); // 建立实际的连接 conn.connect(); // 定义 BufferedReader输入流来读取URL的响应,设置接收格式 in = new BufferedReader(new InputStreamReader( conn.getInputStream(),"utf-8")); while ((line = in.readLine()) != null) { sb.append(line); } result=sb.toString(); } catch (Exception e) { System.out.println("发送GET请求出现异常!" + e); e.printStackTrace(); } // 使用finally块来关闭输入流 finally { try { if (in != null) { in.close(); } } catch (Exception e2) { e2.printStackTrace(); } } return result; } } |
2.2 调用(应用)
2.2.1 post
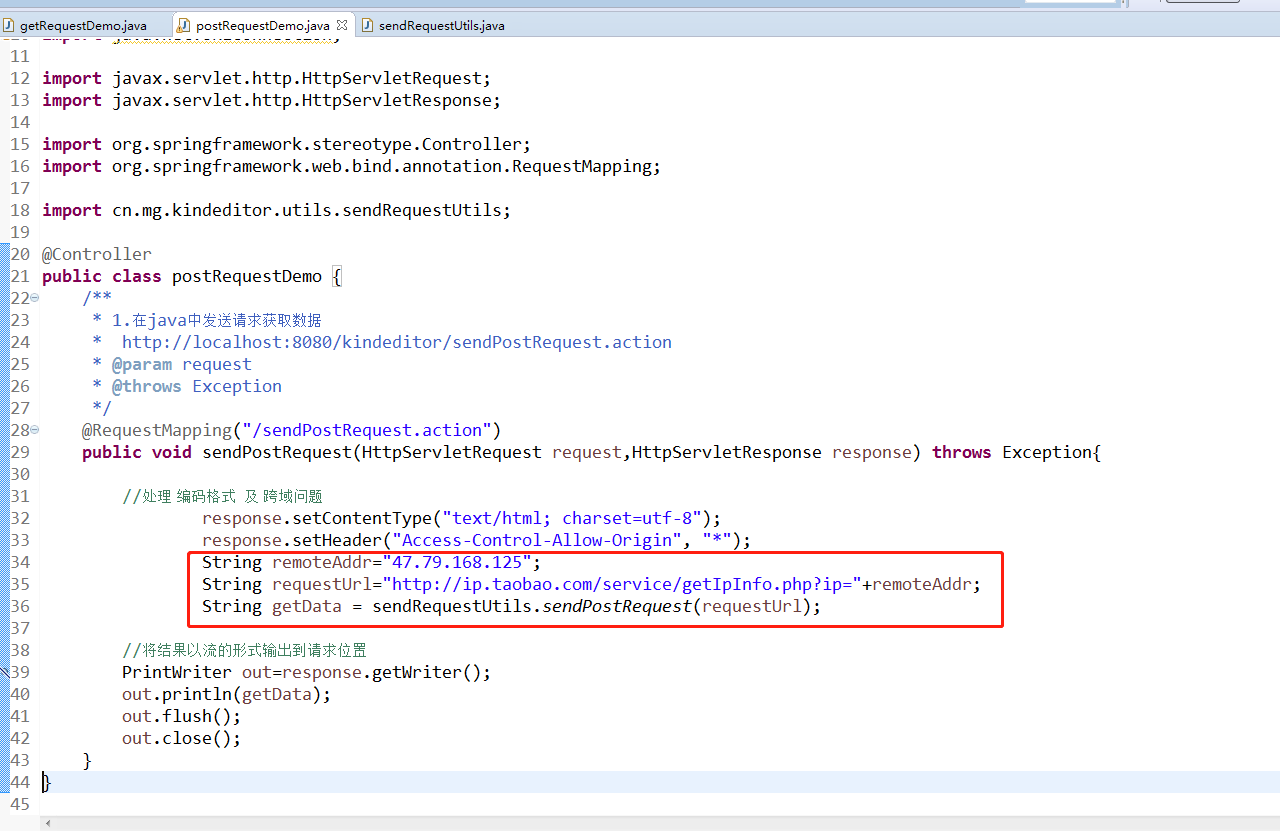
效果:
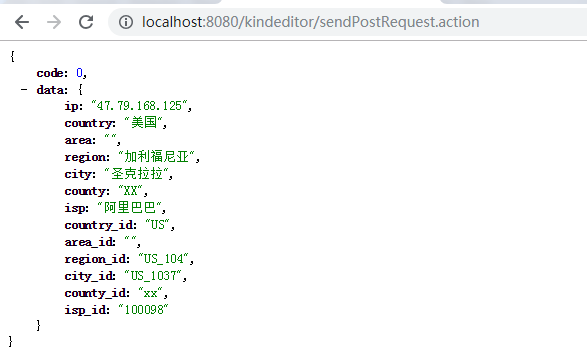
2.2.2 get
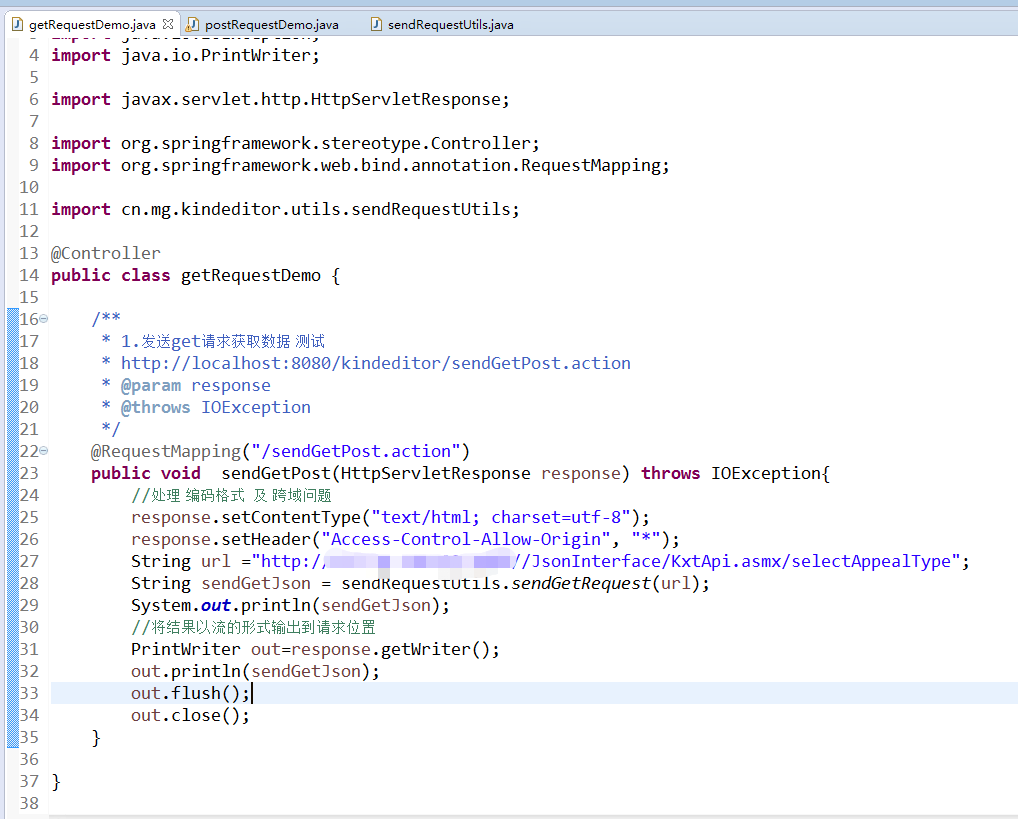
效果:
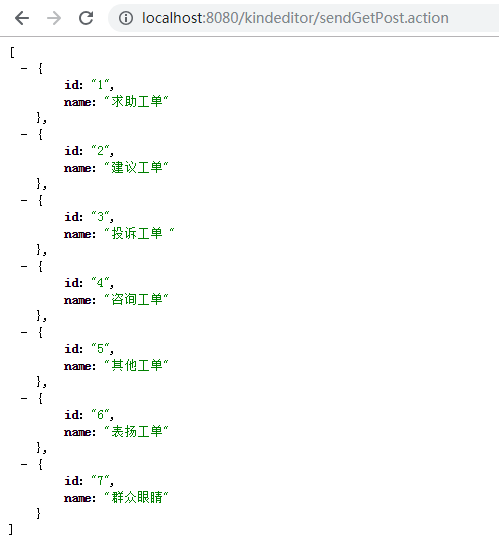
3. 附件
码云:
https://gitee.com/Luck_Me/javaToGetOrPost/tree/master
百度云:
链接:https://pan.baidu.com/s/1FJSngPT72DwNTrgsWV-cLg
提取码:7tv2