[ServiceContract] public interface IPeopleInfo { [OperationContract] int GetAge(string name); }
数据契约:
[DataContract] public class People { public string Name; public int Age; }
服务实现:
public class PeopleInfo : IPeopleInfo { public int GetAge(string name) { List<People> peopleList = DataFactory.GetPeopleList(); return peopleList.Find(t =>t.Name==name).Age ; } }
辅助类:
public class DataFactory { public static List<People> GetPeopleList() { List<People> peopleList = new List<People>(); peopleList.AddRange(new People[] { new People{Name="tjm",Age=18}, new People{Name="lw",Age=20}, new People{Name="tj",Age=22}, }); return peopleList; } }
WCF部署IIS出现“由于扩展配置问题而无法提供您请求的页面。如果该页面是脚本,请添加处理程序。如果应下载文件,请添加 MIME 映射”的解决办法
管理员身份运行C:\Windows\Microsoft.NET\Framework\v3.0\Windows Communication Foundation\ServiceModelReg.exe -i
HTTP 错误 404.3 - Not Found,
使用管理员注册:C:\Windows\system32>"C:\Windows\Microsoft.NET\Framework\v4.0.30319\ServiceModelReg.exe" -r
Microsoft(R) WCF/WF 注册工具版本 4.5.0.0
版权所有(C) Microsoft Corporation。保留所有权利。
用于管理一台计算机上 WCF 和 WF 组件的安装和卸载的管理实用工具。
[错误]此 Windows 版本不支持此工具。管理员应改为使用“打开或关闭 Windows 功能”对话框或 dism 命令行工具来安装/卸载 Windows Communication Foundation 功能。
把我画红线的框内的复选框全部勾选,点击确定,然后再在iis中再进行浏览就能够找到发布后的WCF服务了,原因应该是我没有安装WCF服务的组件而导致的吧。在浏览器中浏览服务如下。
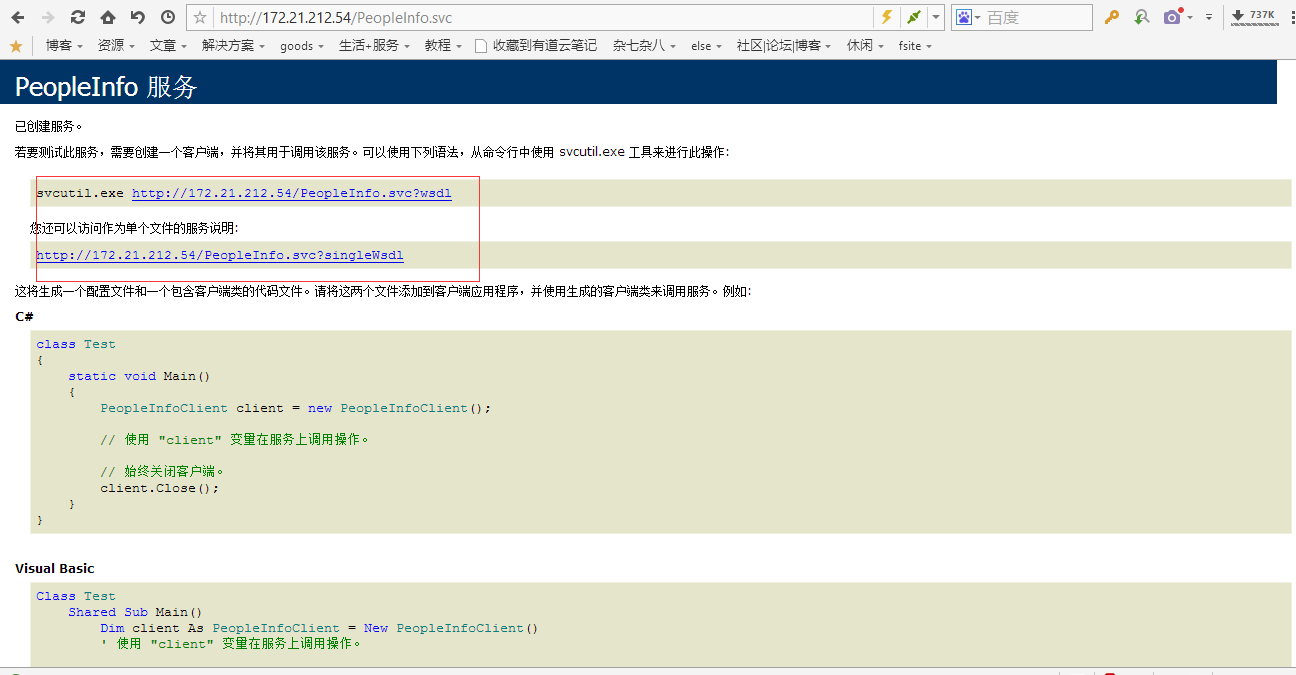
在window功能中卸载iis和WCF服务,然后再重新安装配置。
首先建立一个Winform项目,界面如下。
注意:在部署之前需要把第一步中编写服务的dll引用进来。
ServiceHost host; private void btnStart_Click(object sender, EventArgs e) { //使用代码绑定 Uri tcpa = new Uri("net.tcp://172.21.212.54:8090/peopleinfo"); host = new ServiceHost(typeof(FirstWCFService.PeopleInfo), tcpa); ServiceMetadataBehavior mBehave = new ServiceMetadataBehavior(); NetTcpBinding tcpb = new NetTcpBinding(); host.Description.Behaviors.Add(mBehave); host.AddServiceEndpoint(typeof(IMetadataExchange), MetadataExchangeBindings.CreateMexTcpBinding(), "mex"); host.AddServiceEndpoint(typeof(FirstWCFService.IPeopleInfo), tcpb, tcpa); host.Open();
this.btnStart.Enabled = false;
this.label1.Text = "Service Running"; } private void btnStop_Click(object sender, EventArgs e) { if (host != null) { host.Close(); this.label1.Text = "Service Closed"; this.btnStart.Enabled = true; } }
2)使用配置文件
<?xml version="1.0" encoding="utf-8" ?> <configuration> <system.serviceModel> <services> <service name="FirstWCFService.PeopleInfo"> <!--客户端用来解释服务,这个端点如果缺少服务会发布失败--> <endpoint contract="IMetadataExchange" binding="mexTcpBinding" address ="net.tcp://172.21.212.54:8090/peoleinfo/mex"></endpoint> <!--提供服务的端点--> <endpoint address="net.tcp://172.21.212.54:8090/peoleinfo" binding="netTcpBinding" contract="FirstWCFService.IPeopleInfo"> </endpoint> </service> </services> <behaviors> <serviceBehaviors> <behavior> <serviceMetadata/> </behavior> </serviceBehaviors> </behaviors> </system.serviceModel> </configuration>
虽然是用来配置文件,但是开启服务的代码还是需要写的。代码如下。
ServiceHost host; private void btnStart_Click(object sender, EventArgs e) { host = new ServiceHost(typeof(FirstWCFService.PeopleInfo)); host.Open(); this.btnStart.Enabled = false; this.label1.Text = "Service Running"; } private void btnStop_Click(object sender, EventArgs e) { if (host != null) { host.Close(); this.label1.Text = "Service Closed"; this.btnStart.Enabled = true; } }
运行程序,点击Start按钮,到此使用Winform作为宿主来发布WCF服务已经成功了。
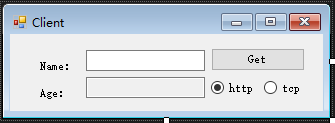
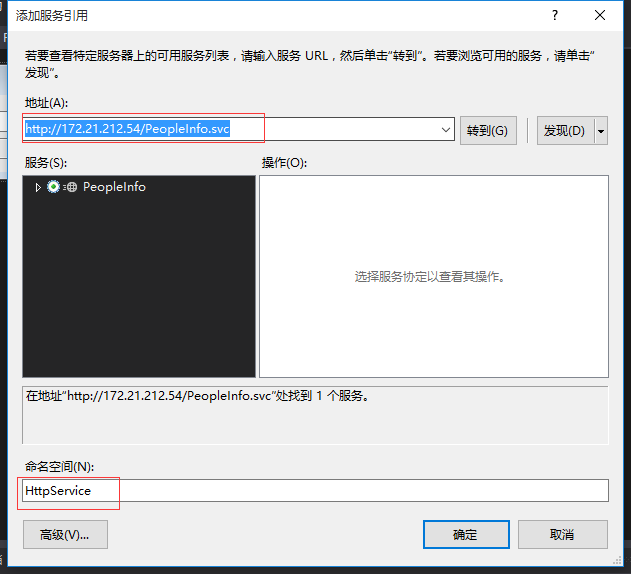
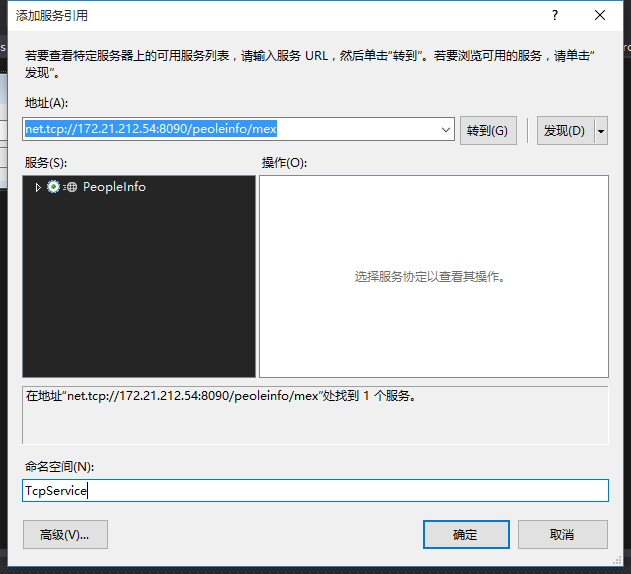
<?xml version="1.0" encoding="utf-8" ?> <configuration> <system.serviceModel> <bindings> <basicHttpBinding> <binding name="BasicHttpBinding_IPeopleInfo" /> </basicHttpBinding> <netTcpBinding> <binding name="NetTcpBinding_IPeopleInfo"> </binding> </netTcpBinding> </bindings> <client> <endpoint address="http://172.21.212.54/PeopleInfo.svc" binding="basicHttpBinding" bindingConfiguration="BasicHttpBinding_IPeopleInfo" contract="HttpService.IPeopleInfo" name="BasicHttpBinding_IPeopleInfo" /> <endpoint address="net.tcp://172.21.212.54:8090/peoleinfo" binding="netTcpBinding" bindingConfiguration="NetTcpBinding_IPeopleInfo" contract="TcpService.IPeopleInfo" name="NetTcpBinding_IPeopleInfo" /> </client> </system.serviceModel> </configuration>
调用服务操作的代码非常简单,就类似于操作本地的类一样,代码如下:
private void bntGet_Click(object sender, EventArgs e) { string name = this.txtName.Text; int age; if (rdbHttp.Checked) { HttpService.PeopleInfoClient httpClient = new HttpService.PeopleInfoClient(); age = httpClient.GetAge(name); } else { TcpService.PeopleInfoClient tcpClient = new TcpService.PeopleInfoClient(); age = tcpClient.GetAge(name); } this.txtAge.Text = age.ToString(); }
到此,wcf的客户端编写完成,结果如下。
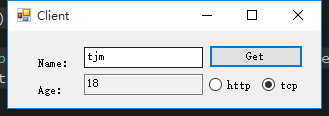
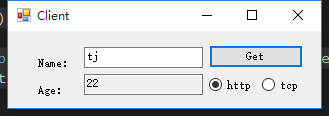
System.ServiceModel.Security.SecurityNegotiationException: 服务器已拒绝客户端凭据。 --->
System.Security.Authentication.InvalidCredentialException: 服务器已拒绝客户端凭据。 --->
System.ComponentModel.Win32Exception: 登录没有成功
<?xml version="1.0" encoding="utf-8" ?> <configuration> <system.serviceModel> <services> <service name="FirstWCFService.PeopleInfo"> <!--客户端用来解释服务,这个端点如果缺少服务会发布失败--> <endpoint contract="IMetadataExchange" binding="mexTcpBinding" address ="net.tcp://172.21.212.54:8090/peoleinfo/mex"></endpoint> <!--提供服务的端点--> <endpoint address="net.tcp://172.21.212.54:8090/peoleinfo" binding="netTcpBinding" contract="FirstWCFService.IPeopleInfo" bindingConfiguration="NoSecurity"> </endpoint> </service> </services> <bindings> <netTcpBinding> <binding name="NoSecurity"> <security mode="None"/> </binding> </netTcpBinding> </bindings> <behaviors> <serviceBehaviors> <behavior> <serviceMetadata/> </behavior> </serviceBehaviors> </behaviors> </system.serviceModel> </configuration>
设置完成之后,重新开启服务。然后在客户端删除winform宿主发布的服务,并且再重新添加,以便在配置文件中重新生成访问tcp方式的服务端点的配置。更新后的配置文件如下,红色加粗部分为更新的。
<?xml version="1.0" encoding="utf-8" ?> <configuration> <system.serviceModel> <bindings> <basicHttpBinding> <binding name="BasicHttpBinding_IPeopleInfo" /> </basicHttpBinding> <netTcpBinding> <binding name="NetTcpBinding_IPeopleInfo"> <security mode="None" /> </binding> </netTcpBinding> </bindings> <client> <endpoint address="http://172.21.212.54/PeopleInfo.svc" binding="basicHttpBinding" bindingConfiguration="BasicHttpBinding_IPeopleInfo" contract="HttpService.IPeopleInfo" name="BasicHttpBinding_IPeopleInfo" /> <endpoint address="net.tcp://172.21.212.54:8090/peoleinfo" binding="netTcpBinding" bindingConfiguration="NetTcpBinding_IPeopleInfo" contract="TcpService.IPeopleInfo" name="NetTcpBinding_IPeopleInfo" /> </client> </system.serviceModel> </configuration>