1、后台获取商品详情接口:
在上一篇文章所新建的ProudctManageController
类中新建下面方法:*Controller
:
//获取商品详情接口
@RequestMapping("get_detail.do")
@ResponseBody
public ServerResponse getDetail(HttpSession session, Integer productId){
User user=(User) session.getAttribute(Const.CURRENT_USER);
if(user==null){
return ServerResponse.createByErrorCodeMessage(ResponseCode.NEED_LOGIN.getCode(),"未登录,请先登录");
}
if(iUserService.checkAdminRole(user).isSuccess()){
//增加商品的逻辑方法
return iProductService.manageProductDetail(productId);
}else {
return ServerResponse.createByErrorMessage("当前登录者不是管理员,无权限操作");
}
}
*Service
:
//查询商品的详细信息
ServerResponse<ProductDetailVo> manageProductDetail(Integer productId);
*ServiceImpl
:
//查询商品的详细信息
public ServerResponse<ProductDetailVo> manageProductDetail(Integer productId){
if(productId==null){
return ServerResponse.createByErrorCodeMessage(ResponseCode.ILLEGAL_ARGUMENT.getCode(),ResponseCode.ILLEGAL_ARGUMENT.getDesc());
}
Product product=productMapper.selectByPrimaryKey(productId);
if(product==null){
return ServerResponse.createByErrorMessage("商品已下架或者删除");
}
ProductDetailVo productDetailVo=assembleProductDetailVo(product);
return ServerResponse.createBySuccess(productDetailVo);
}
其中用到了selectByPrimaryKey
和assembleProductDetailVo
这两个方法,其中selectByPrimaryKey
是使用Mybaties逆向工程生产的方法,根据productId
来查询Product
的信息。
下面介绍下assembleProductDetailVo
这个方法
这个方法是自己封装的,用户返回给前端一个商品详情类,那么为什么要用到这个封装类呢?因为我们返回给前端的不仅仅是一个数据表里面的信息,我们返回的多个表综合的信息。所以使用的是多表查询,我们自己封装了一个ProductDetailVo
类,来定义我们需要返回给前端定义的字段。
新建ProductDetailVo
类
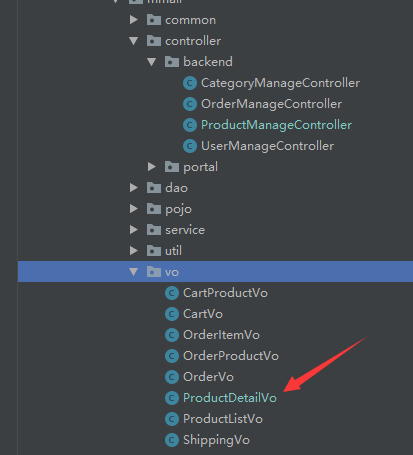
ProductDetailVo
内容如下:
package com.mmall.vo;
import java.math.BigDecimal;
public class ProductDetailVo {
private Integer id;
private Integer categoryId;
private String name;
private String subtitle;
private String mainImage;
private String subImages;
private String detail;
private BigDecimal price;
private Integer stock;
private Integer status;
private String createTime;
private String updateTime;
private String imageHost;
private Integer parentCategoryId;
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public Integer getCategoryId() {
return categoryId;
}
public void setCategoryId(Integer categoryId) {
this.categoryId = categoryId;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getSubtitle() {
return subtitle;
}
public void setSubtitle(String subtitle) {
this.subtitle = subtitle;
}
public String getMainImage() {
return mainImage;
}
public void setMainImage(String mainImage) {
this.mainImage = mainImage;
}
public String getSubImages() {
return subImages;
}
public void setSubImages(String subImages) {
this.subImages = subImages;
}
public String getDetail() {
return detail;
}
public void setDetail(String detail) {
this.detail = detail;
}
public BigDecimal getPrice() {
return price;
}
public void setPrice(BigDecimal price) {
this.price = price;
}
public Integer getStock() {
return stock;
}
public void setStock(Integer stock) {
this.stock = stock;
}
public Integer getStatus() {
return status;
}
public void setStatus(Integer status) {
this.status = status;
}
public String getCreateTime() {
return createTime;
}
public void setCreateTime(String createTime) {
this.createTime = createTime;
}
public String getUpdateTime() {
return updateTime;
}
public void setUpdateTime(String updateTime) {
this.updateTime = updateTime;
}
public String getImageHost() {
return imageHost;
}
public void setImageHost(String imageHost) {
this.imageHost = imageHost;
}
public Integer getParentCategoryId() {
return parentCategoryId;
}
public void setParentCategoryId(Integer parentCategoryId) {
this.parentCategoryId = parentCategoryId;
}
}
assembleProductDetailVo
:封装的方法
//对返回的商品详细信息进行封装
private ProductDetailVo assembleProductDetailVo(Product product){
ProductDetailVo productDetailVo=new ProductDetailVo();
productDetailVo.setId(product.getId());
productDetailVo.setSubtitle(product.getSubtitle());
productDetailVo.setPrice(product.getPrice());
productDetailVo.setMainImage(product.getMainImage());
productDetailVo.setSubImages(product.getSubImages());
productDetailVo.setCategoryId(product.getCategoryId());
productDetailVo.setDetail(product.getDetail());
productDetailVo.setName(product.getName());
productDetailVo.setStatus(product.getStatus());
productDetailVo.setStock(product.getStock());
productDetailVo.setImageHost(PropertiesUtil.getProperty("ftp.server.http.prefix","http://img.happymmall.com/"));
Category category=categoryMapper.selectByPrimaryKey(product.getCategoryId());
if(category==null){
productDetailVo.setParentCategoryId(0);//默认根节点
}else{
productDetailVo.setParentCategoryId(category.getParentId());
}
productDetailVo.setCreateTime(DateTimeUtil.dateToStr(product.getCreateTime()));
productDetailVo.setUpdateTime(DateTimeUtil.dateToStr(product.getUpdateTime()));
return productDetailVo;
}
这一行代码设置的是对应的图片服务器:下面会对这个作介绍:
productDetailVo.setImageHost(PropertiesUtil.getProperty("ftp.server.http.prefix","http://img.happymmall.com/"));
selectByPrimaryKey
方法使用的是逆向工程自动生成的方法。
2、PropertiesUtil工具的开发:
1、在util包下新建PropertiesUtil
类
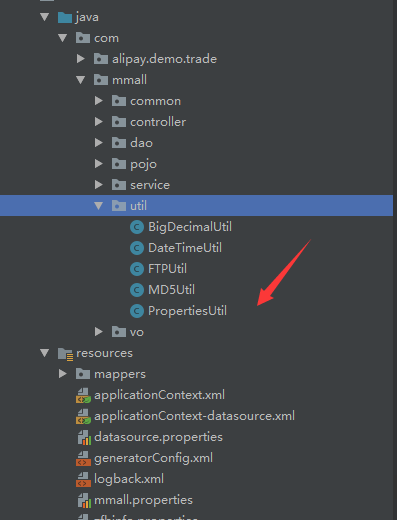
PropertiesUtil
:
package com.mmall.util;
import org.apache.commons.lang3.StringUtils;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.IOException;
import java.io.InputStreamReader;
import java.util.Properties;
/**
* Created by geely
*/
public class PropertiesUtil {
private static Logger logger = LoggerFactory.getLogger(PropertiesUtil.class);
private static Properties props;
static {
String fileName = "mmall.properties";
props = new Properties();
try {
props.load(new InputStreamReader(PropertiesUtil.class.getClassLoader().getResourceAsStream(fileName),"UTF-8"));
} catch (IOException e) {
logger.error("配置文件读取异常",e);
}
}
public static String getProperty(String key){
String value = props.getProperty(key.trim());
if(StringUtils.isBlank(value)){
return null;
}
return value.trim();
}
public static String getProperty(String key,String defaultValue){
String value = props.getProperty(key.trim());
if(StringUtils.isBlank(value)){
value = defaultValue;
}
return value.trim();
}
}
String fileName = "mmall.properties";
这行代码所指的就是下文中新建的mmal.properties
配置文件 。
2、resouces目录下新建mmal.properties
配置文件
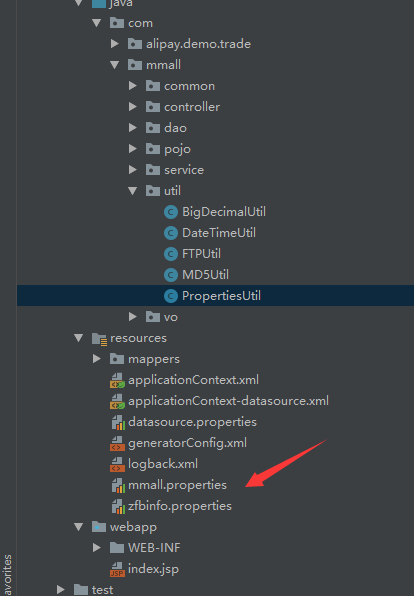
mmal.properties
:
//对应的ftp文件服务器的相关配置
ftp.server.ip=127.0.0.1
ftp.user=admin
ftp.pass=admin
ftp.server.http.prefix=http://image.imooc.com/
alipay.callback.url=http://erjq8u.natappfree.cc/order/alipay_callback.do
password.salt = geelysdafaqj23ou89ZXcj@#$@#$#@KJdjklj;D../dSF.,
3、DateTimeUtil时间处理工具
1、新建DateTimeUtil
工具类
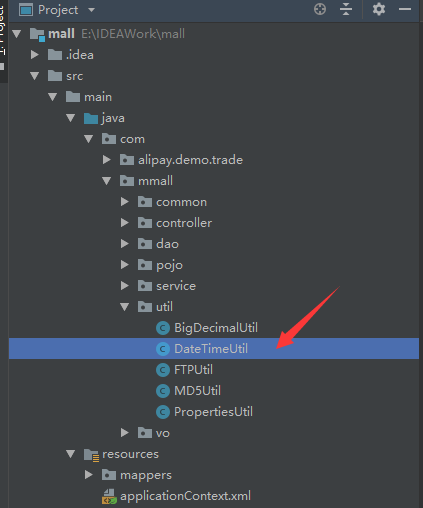
DateTimeUtil
:
package com.mmall.util;
import org.apache.commons.lang3.StringUtils;
import org.joda.time.DateTime;
import org.joda.time.format.DateTimeFormat;
import org.joda.time.format.DateTimeFormatter;
import java.util.Date;
public class DateTimeUtil {
//joda-time
//str->date
//date->str
public static final String STANDARD_FORMAT="yyyy-MM-dd HH:mm:ss";
public static Date strToDate(String dateTimeStr,String formatStr){
DateTimeFormatter dateTimeFormatter=DateTimeFormat.forPattern(formatStr);
DateTime dateTime=dateTimeFormatter.parseDateTime(dateTimeStr);
return dateTime.toDate();
}
public static String dateToStr(Date date,String formatStr){
if(date==null){
return StringUtils.EMPTY;
}
DateTime dateTime=new DateTime(date);
return dateTime.toString(formatStr);
}
/**
*
* @param dateTimeStr 具体时间
* @param STANDARD_FORMAT 时间格式
* @return
*/
public static Date strToDate(String dateTimeStr){
DateTimeFormatter dateTimeFormatter=DateTimeFormat.forPattern(STANDARD_FORMAT);
DateTime dateTime=dateTimeFormatter.parseDateTime(dateTimeStr);
return dateTime.toDate();
}
public static String dateToStr(Date date){
if(date==null){
return StringUtils.EMPTY;
}
DateTime dateTime=new DateTime(date);
return dateTime.toString(STANDARD_FORMAT);
}
/* public static void main(String[] args) {
System.out.println(DateTimeUtil.dateToStr(new Date(),"yyyy-MM-dd HH:mm:ss"));
System.out.println(DateTimeUtil.strToDate("2016-02-09 11:45:28","yyyy-MM-dd HH:mm:ss"));
}*/
}
4、接口测试
后台获取商品详情接口测试
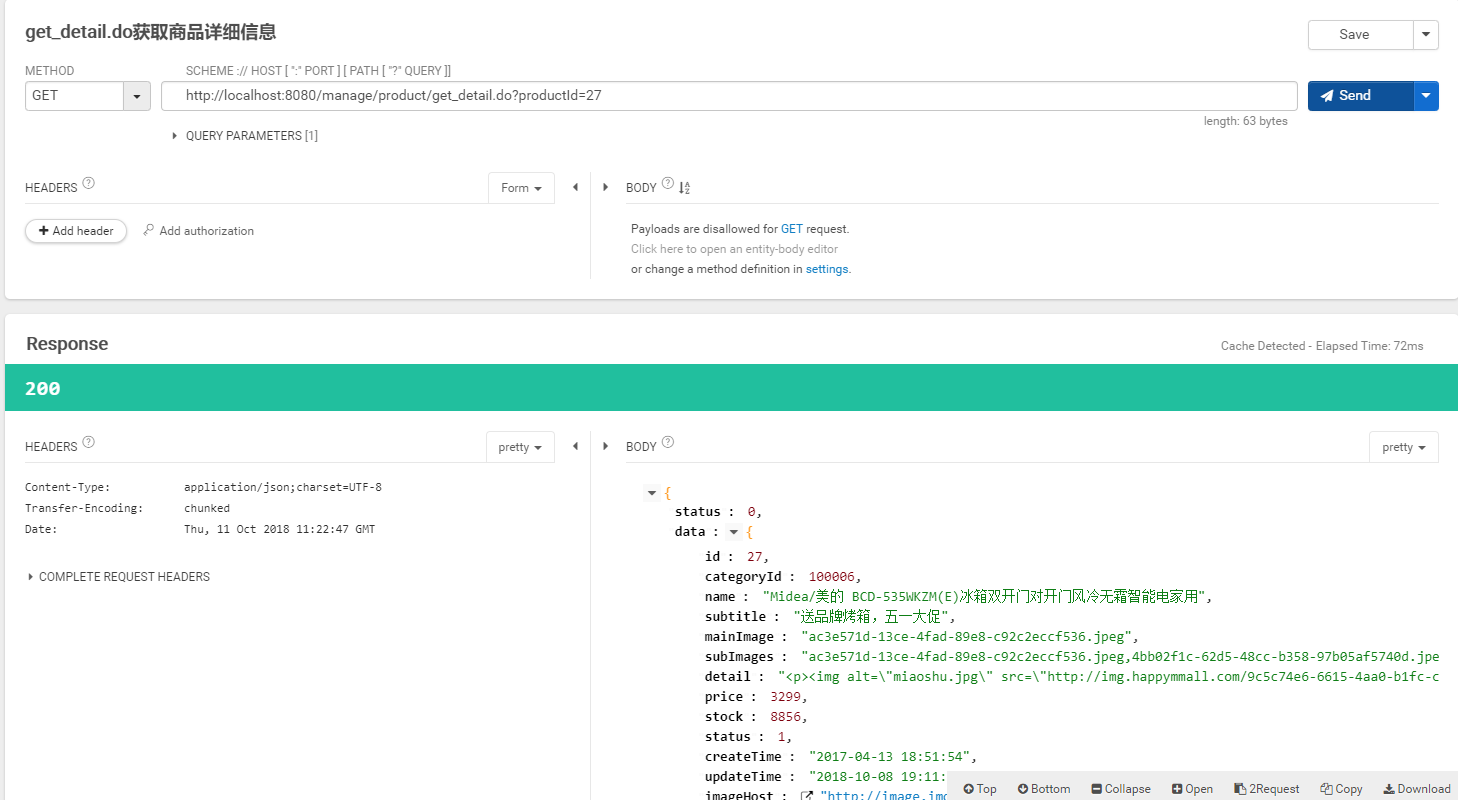