本文涉及两个实体类:基类(User)、子类(Employee)。以下是类图:
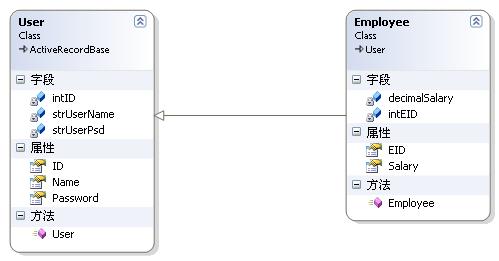
本文主要内容:
1.编写数据库脚本
2.JoinedBase和JoinedKey属性说明
3.编写实体类
4.编写调用代码
一、编写数据库脚本
其实本文涉及的数据表在前面的笔记中都出现过!












二、JoinedBase和JoinedKey属性说明
JoinedBase属性:该属性是ActiveRecord属性子属性,用于基类中;
JoinedKey性性:该属性用在子类中,用于代替PrimaryKey的位置;
三、编写实体类
基类:User.cs


































































































子类:Employee.cs









































































四、编写调用代码(只列出添加Employee的代码)




















