1.搭个简易上传测试服务器
比如:python flask写简单服务器
from flask import Flask, url_for, request,redirect,send_from_directory
import os
app = Flask(__name__)
app.config['UPLOAD_FOLDER'] = 'uploads/' # 保存文件位置
ALLOWED_EXTENSIONS = set(['txt', 'pdf', 'png', 'jpg', 'jpeg', 'gif'])
@app.route('/uploads/<filename>')
def uploaded_file(filename):
return send_from_directory(app.config['UPLOAD_FOLDER'], filename)
@app.route('/', methods=['GET', 'POST'])
def upload_file():
if request.method == 'POST':
file = request.files['file']
if file :
file.save(os.path.join(app.config['UPLOAD_FOLDER'], file.filename))
return redirect(url_for('uploaded_file',
filename=file.filename))
return '''
<html><head><title>上传测试</title>
</head><body><h1>上传测试</h1>
<script>
function upl(){
var form = new FormData();
form.append("file", document.getElementsByName("file")[0].files[0]);
var oReq = new XMLHttpRequest();
oReq.open("POST", "/");
oReq.send(form);
}
</script>
<form action="" method="post" enctype="multipart/form-data">
<p><input type="file" name="file">
<input type="submit" value="表单提交">
</p></form>
<input type="button" value="ajax提交" onclick="upl()">
</body> </html>
'''
with app.test_request_context(): # 输出url
pass
if __name__ == '__main__':
# app.debug = True
app.run()
2.观察上传头标识
运行服务器后打开浏览器访问,地址打开控制台,上传任意文件,进行观察
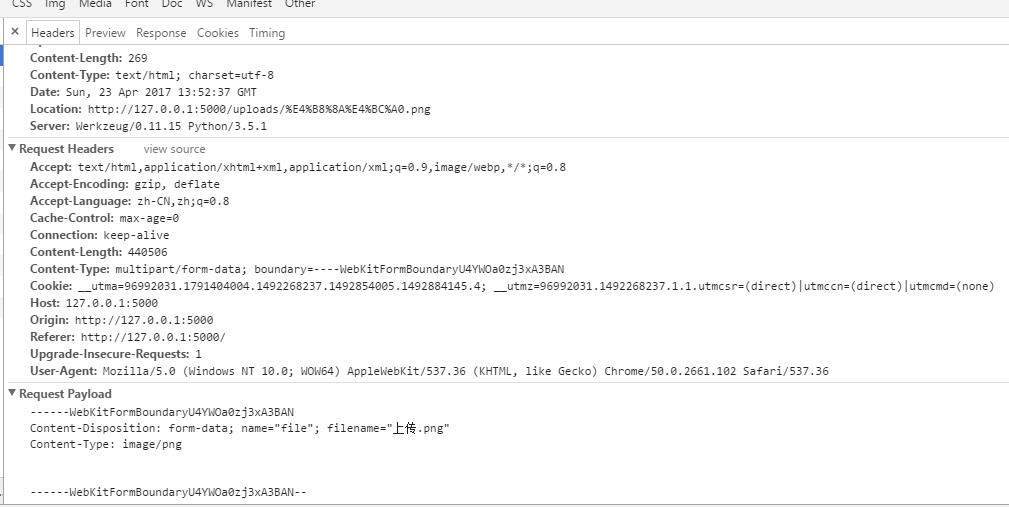
截图
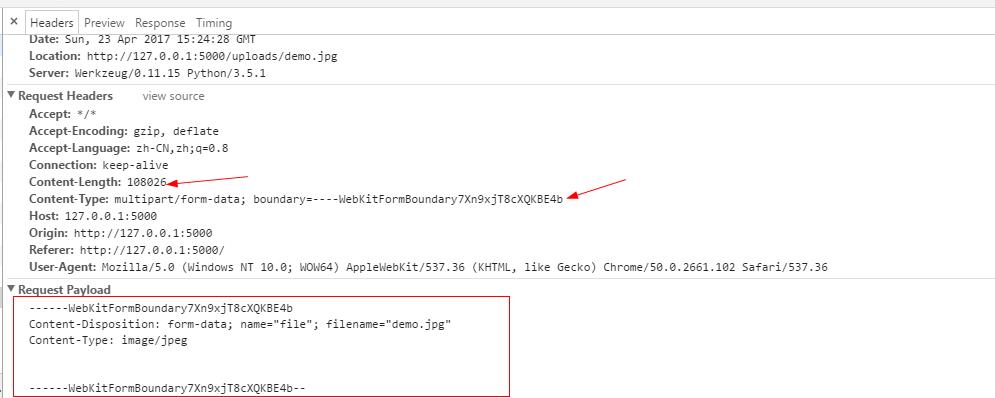
截图
3.nodejs编写上传测试客户端
var http = require('http');
var querystring = require('querystring');
var fs = require('fs');
var post_data = { };//post提交数据
var content = querystring.stringify(post_data);#将对象转换成字符串,字符串里多个参数将用 ‘&' 分隔,将用 ‘=' 赋值
var boundaryKey = new Date().getTime();//创建随机切割标识字 你可以百度 'multipart form-data boundary'了解
//var boundaryKey =Math.random().toString(16);
var options = {
hostname: '127.0.0.1',
port: 5000,
path: '/',
method: 'POST',
headers: {
// 'Accept': '*/*',
// 'Accept-Encoding': 'gzip, deflate',
// 'Connection': 'keep-alive',
'Content-Type':'multipart/form-data; boundary=----'+boundaryKey,//文件上传标识与切割标识
// 'Host':'127.0.0.1:5000',
// 'Origin':'http://127.0.0.1:5000',
// 'Referer':'http://127.0.0.1:5000/',
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; WOW64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/50.0.2661.102 Safari/537.36'
}
};
var req = http.request(options, function (res) {
res.setEncoding('utf8');
console.log('STATUS: ' + res.statusCode);
console.log('HEADERS: ' + JSON.stringify(res.headers));
res.on('data', function (chunk) {
console.log('BODY: ' + chunk);
});
res.on('end', function () {
console.log('res end');
});
});
var payload ='\r\n------'+boundaryKey+'\r\n' +
'Content-Disposition: form-data; name="file"; filename="test.png"\r\n' +
'Content-Type: image/png\r\n\r\n';
var enddata = '\r\n------'+boundaryKey+'--';
req.setHeader('Content-Length', Buffer.byteLength(payload) + Buffer.byteLength(enddata) + fs.statSync("./test.png").size);
req.write(payload);
var fileStream = fs.createReadStream("./test.png", { bufferSize: 4 * 1024 });
fileStream.pipe(req, { end: false });
fileStream.on('end', function () {
req.end(enddata);
});
req.on('error', function (e) {
console.log('problem with request: ' + e.message);
});
req.write(content);
// req.end();
还有种写法:
var http = require('http');
var querystring = require('querystring');
var fs = require('fs');
var boundaryKey = new Date().getTime()//Math.random().toString(16); //创建随机切割标识字
var options = {
hostname: '127.0.0.1',
port: 5000,
path: '/',
method: 'POST',
headers: {
// 'Accept': '*/*',
// 'Accept-Encoding': 'gzip, deflate',
// 'Connection': 'keep-alive',
'Content-Type': 'multipart/form-data; boundary=----' + boundaryKey,
// 'Host':'127.0.0.1:5000',
// 'Origin':'http://127.0.0.1:5000',
// 'Referer':'http://127.0.0.1:5000/',
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; WOW64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/50.0.2661.102 Safari/537.36'
}
};
var req = http.request(options, function (res) {
res.setEncoding('utf8');
console.log('STATUS: ' + res.statusCode);
console.log('HEADERS: ' + JSON.stringify(res.headers));
res.on('data', function (chunk) {
console.log('BODY: ' + chunk);
});
res.on('end', function () {
console.log('res end');
});
});
var payload = '\r\n------' + boundaryKey + '\r\n' +
'Content-Disposition: form-data; name="file"; filename="test.png"\r\n' +
'Content-Type: image/png\r\n\r\n';
var enddata = '\r\n------' + boundaryKey + '--';
var fileStream=fs.readFileSync("./test.png");
req.setHeader('Content-Length', Buffer.byteLength(payload) + Buffer.byteLength(enddata) + fs.statSync("./test.png").size);
console.log(payload);
req.write(payload);
console.log(fileStream);
req.write(fileStream);
console.log(enddata);
req.end(enddata);
req.on('error', function (e) {
console.log('problem with request: ' + e.message);
});