想要给图片添加文字水印或者注释,我们需要实现在UIImage上写字的功能。
1,效果图如下:
(在图片左上角和右下角都添加了文字。)
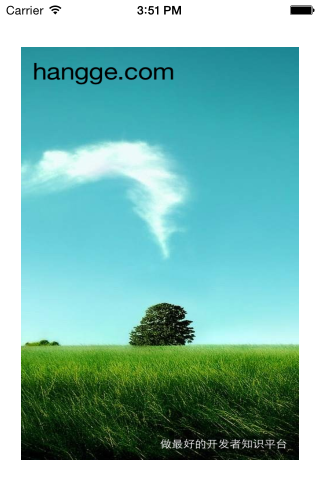
2,为方便使用,我们通过扩展UIImage类来实现添加水印功能
(文字大小,文字颜色,背景色,位置,边距都可以设置)
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
|
//--- UIImageExtension.swift ---
import
UIKit
extension
UIImage
{
//水印位置枚举
enum
WaterMarkCorner
{
case
TopLeft
case
TopRight
case
BottomLeft
case
BottomRight
}
//添加水印方法
func
waterMarkedImage(waterMarkText:
String
, corner:
WaterMarkCorner
= .
BottomRight
,
margin:
CGPoint
=
CGPoint
(x: 20, y: 20), waterMarkTextColor:
UIColor
=
UIColor
.whiteColor(),
waterMarkTextFont:
UIFont
=
UIFont
.systemFontOfSize(20),
backgroundColor:
UIColor
=
UIColor
.clearColor()) ->
UIImage
{
let
textAttributes = [
NSForegroundColorAttributeName
:waterMarkTextColor,
NSFontAttributeName
:waterMarkTextFont]
let
textSize =
NSString
(string: waterMarkText).sizeWithAttributes(textAttributes)
var
textFrame =
CGRectMake
(0, 0, textSize.width, textSize.height)
let
imageSize =
self
.size
switch
corner{
case
.
TopLeft
:
textFrame.origin = margin
case
.
TopRight
:
textFrame.origin =
CGPoint
(x: imageSize.width - textSize.width - margin.x, y: margin.y)
case
.
BottomLeft
:
textFrame.origin =
CGPoint
(x: margin.x, y: imageSize.height - textSize.height - margin.y)
case
.
BottomRight
:
textFrame.origin =
CGPoint
(x: imageSize.width - textSize.width - margin.x,
y: imageSize.height - textSize.height - margin.y)
}
// 开始给图片添加文字水印
UIGraphicsBeginImageContext
(imageSize)
self
.drawInRect(
CGRectMake
(0, 0, imageSize.width, imageSize.height))
NSString
(string: waterMarkText).drawInRect(textFrame, withAttributes: textAttributes)
let
waterMarkedImage =
UIGraphicsGetImageFromCurrentImageContext
()
UIGraphicsEndImageContext
()
return
waterMarkedImage
}
}
|
3,使用样例
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
|
import
UIKit
class
ViewController
:
UIViewController
{
@IBOutlet
weak
var
imageView:
UIImageView
!
override
func
viewDidLoad() {
super
.viewDidLoad()
//使用链式调用方法,给图片添加两条水印
imageView.image =
UIImage
(named:
"bg"
)?
.waterMarkedImage(
"做最好的开发者知识平台"
)
.waterMarkedImage(
"hangge.com"
, corner: .
TopLeft
,
margin:
CGPoint
(x: 20, y: 20), waterMarkTextColor:
UIColor
.blackColor(),
waterMarkTextFont:
UIFont
.systemFontOfSize(45), backgroundColor:
UIColor
.clearColor())
}
override
func
didReceiveMemoryWarning() {
super
.didReceiveMemoryWarning()
}
}
|