目录结构
引入jQuery:jquery-1.11.1.min.js
html代码
<!DOCTYPE html>
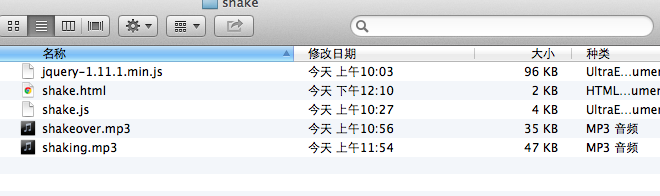
引入jQuery:jquery-1.11.1.min.js
html代码
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>摇一摇功能</title>
<script type="text/javascript" src="jquery-1.11.1.min.js"></script>
<script type="text/javascript" src="shake.js"></script>
<script type="text/javascript">
var count = 1;
window.onload = function() {
var myShakeEvent = new Shake({
threshold: 15
});
myShakeEvent.start();
window.addEventListener('shake', shakeEventDidOccur, false);
function shakeEventDidOccur () {
shakingAutoPlay();
$("#shakeMe").text("第"+count+"次摇晃手机");
count++;
window.setTimeout(shakeoverAutoPlay,1500);
}
};
//摇动时的声音
function shakingAutoPlay(){
var shaking = document.getElementById("shaking");//必须使用 document.getElementById 获取dom而非jQuery对象
shaking.loop = false;//必须设置为false 不然在android手机4.0版本上会一直循环播放该音效
shaking.play();
}
//摇动结束后的声音
function shakeoverAutoPlay(){
var shakeover = document.getElementById("shakeover");
shakeover.loop = false;
shakeover.play();
}
</script>
</head>
<body>
<div id="pro">
<p>使劲晃动您的手机</p>
<h1 id="shakeMe">尚未摇动</h1>
<audio id="shaking" src="shaking.mp3" controls="controls" loop="false" hidden="true"></audio>
<audio id="shakeover" src="shakeover.mp3" controls="controls" loop="false" hidden="true"></audio>
</div>
</body>
</html>
shake.js
shake.js
/*
* Author: Alex Gibson
* https://github.com/alexgibson/shake.js
* License: MIT license
*/
(function(global, factory) {
if (typeof define === 'function' && define.amd) {
define(function() {
return factory(global, global.document);
});
} else if (typeof module !== 'undefined' && module.exports) {
module.exports = factory(global, global.document);
} else {
global.Shake = factory(global, global.document);
}
} (typeof window !== 'undefined' ? window : this, function (window, document) {
'use strict';
function Shake(options) {
//feature detect
this.hasDeviceMotion = 'ondevicemotion' in window;
this.options = {
threshold: 15, //default velocity threshold for shake to register
timeout: 1000 //default interval between events
};
if (typeof options === 'object') {
for (var i in options) {
if (options.hasOwnProperty(i)) {
this.options[i] = options[i];
}
}
}
//use date to prevent multiple shakes firing
this.lastTime = new Date();
//accelerometer values
this.lastX = null;
this.lastY = null;
this.lastZ = null;
//create custom event
if (typeof document.CustomEvent === 'function') {
this.event = new document.CustomEvent('shake', {
bubbles: true,
cancelable: true
});
} else if (typeof document.createEvent === 'function') {
this.event = document.createEvent('Event');
this.event.initEvent('shake', true, true);
} else {
return false;
}
}
//reset timer values
Shake.prototype.reset = function () {
this.lastTime = new Date();
this.lastX = null;
this.lastY = null;
this.lastZ = null;
};
//start listening for devicemotion
Shake.prototype.start = function () {
this.reset();
if (this.hasDeviceMotion) {
window.addEventListener('devicemotion', this, false);
}
};
//stop listening for devicemotion
Shake.prototype.stop = function () {
if (this.hasDeviceMotion) {
window.removeEventListener('devicemotion', this, false);
}
this.reset();
};
//calculates if shake did occur
Shake.prototype.devicemotion = function (e) {
var current = e.accelerationIncludingGravity;
var currentTime;
var timeDifference;
var deltaX = 0;
var deltaY = 0;
var deltaZ = 0;
if ((this.lastX === null) && (this.lastY === null) && (this.lastZ === null)) {
this.lastX = current.x;
this.lastY = current.y;
this.lastZ = current.z;
return;
}
deltaX = Math.abs(this.lastX - current.x);
deltaY = Math.abs(this.lastY - current.y);
deltaZ = Math.abs(this.lastZ - current.z);
if (((deltaX > this.options.threshold) && (deltaY > this.options.threshold)) || ((deltaX > this.options.threshold) && (deltaZ > this.options.threshold)) || ((deltaY > this.options.threshold) && (deltaZ > this.options.threshold))) {
//calculate time in milliseconds since last shake registered
currentTime = new Date();
timeDifference = currentTime.getTime() - this.lastTime.getTime();
if (timeDifference > this.options.timeout) {
window.dispatchEvent(this.event);
this.lastTime = new Date();
}
}
this.lastX = current.x;
this.lastY = current.y;
this.lastZ = current.z;
};
//event handler
Shake.prototype.handleEvent = function (e) {
if (typeof (this[e.type]) === 'function') {
return this[e.type](e);
}
};
return Shake;
}));
demo地址:http://files.cnblogs.com/files/avivaye/shake.zip