WebAPI 2参数绑定方法
简单类型参数
Example 1: Sending a simple parameter in the Url
01
02
03
04
05
06
07
08
09
10
11
|
[RoutePrefix(
"api/values"
)]
public
class
ValuesController : ApiController
{
// http://localhost:49407/api/values/example1?id=2
[Route(
"example1"
)]
[HttpGet]
public
string
Get(
int
id)
{
return
"value"
;
}
}
|
Example 2: Sending simple parameters in the Url
01
02
03
04
05
06
07
|
// http://localhost:49407/api/values/example2?id1=1&id2=2&id3=3
[Route(
"example2"
)]
[HttpGet]
public
string
GetWith3Parameters(
int
id1,
long
id2,
double
id3)
{
return
"value"
;
}
|
Example 3: Sending simple parameters using attribute routing
01
02
03
04
05
06
07
|
// http://localhost:49407/api/values/example3/2/3/4
[Route(
"example3/{id1}/{id2}/{id3}"
)]
[HttpGet]
public
string
GetWith3ParametersAttributeRouting(
int
id1,
long
id2,
double
id3)
{
return
"value"
;
}
|
Example 4: Sending an object in the Url
// http://localhost:49407/api/values/example4?id1=1&id2=2&id3=3 [Route("example4")] [HttpGet] public string GetWithUri([FromUri] ParamsObject paramsObject) { return "value:" + paramsObject.Id1; }
Example 5: Sending an object in the Request body
[Route("example5")] [HttpPost] public string GetWithBody([FromBody] ParamsObject paramsObject) { return "value:" + paramsObject.Id1; }
注意 [FromBody] 只能用一次,多于一次将不能正常工作
Calling the method using Urlencoded in the body:
01
02
03
04
05
06
|
User-Agent: Fiddler
Host: localhost:49407
Content-Length: 32
Content-Type: application/x-www-form-urlencoded
id1=1&id2=2&id3=3
|
Calling the method using Json in the body
01
02
03
04
05
06
|
User-Agent: Fiddler
Host: localhost:49407
Content-Length: 32
Content-Type: application/json
{
"Id1"
: 2,
"Id2"
: 2,
"Id3"
: 3}
|
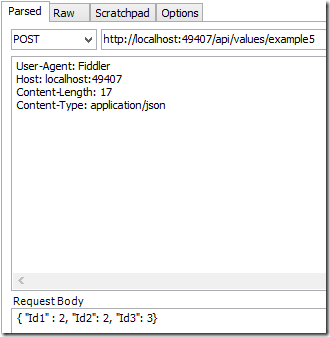
Calling the method using XML in the body
This requires extra code in the Global.asax
01
02
03
04
05
06
07
08
09
10
11
12
|
protected
void
Application_Start()
{
var
xml = GlobalConfiguration.Configuration.Formatters.XmlFormatter;
xml.UseXmlSerializer =
true
;
The client request
is
as
follows:
User-Agent: Fiddler
Content-Type: application/xml
Host: localhost:49407
Content-Length: 65
<ParamsObject><Id1>7</Id1><Id2>8</Id2><Id3>9</Id3></ParamsObject>
|
数组和列表(Array,List)
Example 6: Sending a simple list in the Url
01
02
03
04
05
06
07
08
09
10
11
12
|
// http://localhost:49407/api/values/example6?paramsObject=2,paramsObject=4,paramsObject=9
[Route(
"example6"
)]
[HttpGet]
public
string
GetListFromUri([FromUri] List<
int
> paramsObject)
{
if
(paramsObject !=
null
)
{
return
"recieved a list with length:"
+ paramsObject.Count;
}
return
"NOTHING RECIEVED..."
;
}
|
Example 7: Sending an object list in the Body
01
02
03
04
05
06
07
08
09
10
11
12
|
// http://localhost:49407/api/values/example8
[Route(
"example8"
)]
[HttpPost]
public
string
GetListFromBody([FromBody] List<ParamsObject> paramsList)
{
if
(paramsList !=
null
)
{
return
"recieved a list with length:"
+ paramsList.Count;
}
return
"NOTHING RECIEVED..."
;
}
|
Calling with Json:
01
02
03
04
05
06
|
User-Agent: Fiddler
Content-Type: application/json
Host: localhost:49407
Content-Length: 91
[{
"Id1"
:3,
"Id2"
:76,
"Id3"
:19},{
"Id1"
:56,
"Id2"
:87,
"Id3"
:94},{
"Id1"
:976,
"Id2"
:345,
"Id3"
:7554}]
|
Calling with XML:
01
02
03
04
05
06
07
08
09
10
|
User-Agent: Fiddler
Content-Type: application/xml
Host: localhost:49407
Content-Length: 258
<ArrayOfParamsObject>
<ParamsObject><Id1>3</Id1><Id2>76</Id2><Id3>19</Id3></ParamsObject>
<ParamsObject><Id1>56</Id1><Id2>87</Id2><Id3>94</Id3></ParamsObject>
<ParamsObject><Id1>976</Id1><Id2>345</Id2><Id3>7554</Id3></ParamsObject>
</ArrayOfParamsObject>
|
Example 8: Sending object lists in the Body
01
02
03
04
05
06
07
08
09
10
11
|
[Route(
"example8"
)]
[HttpPost]
public
string
GetListsFromBody([FromBody] List<List<ParamsObject>> paramsList)
{
if
(paramsList !=
null
)
{
return
"recieved a list with length:"
+ paramsList.Count;
}
return
"NOTHING RECIEVED..."
;
}
|
This is a little bit different to the previous examples. The body can only send one single object to Web API. Because of this, the lists of objects are wrapped in a list or a parent object.
01
02
03
04
05
06
07
08
09
10
11
12
13
14
15
16
17
18
|
POST http:
//localhost:49407/api/values/example8 HTTP/1.1
User-Agent: Fiddler
Content-Type: application/json
Host: localhost:49407
Content-Length: 185
[
[
{
"Id1"
:3,
"Id2"
:76,
"Id3"
:19},
{
"Id1"
:56,
"Id2"
:87,
"Id3"
:94},
{
"Id1"
:976,
"Id2"
:345,
"Id3"
:7554}
],
[
{
"Id1"
:3,
"Id2"
:76,
"Id3"
:19},
{
"Id1"
:56,
"Id2"
:87,
"Id3"
:94},
{
"Id1"
:976,
"Id2"
:345,
"Id3"
:7554}
]
]
|