个人做题总结,希望能够帮助到未来的学弟学妹们的学习!
永远爱你们的
————新宝宝
1:
题目描述
Your task is to Calculate a + b. Too easy?! Of course! I specially designed the problem for acm beginners. You must have found that some problems have the same titles with this one, yes, all these problems were designed for the same aim
输入
The input will consist of a series of pairs of integers a and b, separated by a space, one pair of integers per line.
输出
For each pair of input integers a and b you should output the sum of a and b in one line, and with one line of output for each line in input.
样例输入
1 5
10 20
样例输出
6
30
可运行的代码:
#include<stdio.h>
int main()
{
int a,b;
while(scanf("%d%d",&a,&b)==2)
printf("%d\n",a+b);
}
#include<stdio.h>
int main()
{
int a,b;
while(scanf("%d%d",&a,&b)!=EOF)
printf("%d\n",a+b);
return 0;
}
2:
题目描述
The first line integer means the number of input integer a and b. Your task is to Calculate a + b.
输入
Your task is to Calculate a + b. The first line integer means the numbers of pairs of input integers.
输出
For each pair of input integers a and b you should output the sum of a and b in one line, and with one line of output for each line in input.
样例输入
2
1 5
10 20
样例输出
6
30
可运行的代码:
#include<stdio.h>
int main()
{
int a,b ;
int n,sum=0;
scanf("%d",&n);
while(n--)
{
scanf("%d%d",&a,&b);
sum=a+b;
printf("%d\n",sum);
}
return 0;
}
//
#include<stdio.h>
int main()
{
int a,b;
while(~scanf("%d%d", &a, &b))printf("%d\n",a+b);
return 0;
}
3:
题目描述
Your task is to Calculate a + b.
输入
Input contains multiple test cases. Each test case contains a pair of integers a and b, one pair of integers per line. A test case containing 0 0 terminates the input and this test case is not to be processed.
输出
For each pair of input integers a and b you should output the sum of a and b in one line, and with one line of output for each line in input.
样例输入
1 5
10 20
0 0
样例输出
6
30
可以运行的代码:
#include<stdio.h>
int main()
{
int a,b;
while(scanf("%d%d",&a,&b)==2)
{
if(a== 0 && b==0)
break;
printf("%d\n",a+b);
}
return 0;
}
4:
题目描述
Your task is to Calculate the sum of some integers.
输入
Input contains multiple test cases. Each test case contains a integer N, and then N integers follow in the same line. A test case starting with 0 terminates the input and this test case is not to be processed.
输出
For each group of input integers you should output their sum in one line, and with one line of output for each line in input.
样例输入
4 1 2 3 4
5 1 2 3 4 5
0
样例输出
10
15
可运行代码:
#include<stdio.h>
int main()
{
int n,a,i=0,sum=0;
while(scanf("%d",&n)&&n!=0)
{
for(i=0;i<n;i++)
{scanf("%d",&a);sum+=a;}
printf("%d\n",sum);
sum=0;
}
return 0;
}
#include<stdio.h>
int main()
{
int N,i,a;
int sum=0;
while(scanf("%d",&N)==1)
{
if(N==0)
break;
sum=0;
while(N--)
{
scanf("%d",&a);
sum=sum+a;
}
printf("%d\n",sum);
}
return 0;
}
5:
题目描述
Your task is to calculate the sum of some integers.
输入
Input contains an integer N in the first line, and then N lines follow. Each line starts with a integer M, and then M integers follow in the same line.
输出
For each group of input integers you should output their sum in one line, and with one line of output for each line in input.
样例输入
2
4 1 2 3 4
5 1 2 3 4 5
样例输出
10
15
可运行代码:
#include<stdio.h>
int main()
{
int N,a,M;
int sum=0;
scanf("%d",&N);
while(N--)
{
sum=0;
scanf("%d",&M);
while(M--)
{
scanf("%d",&a);
sum=sum+a;
}
printf("%d\n",sum);
}
return 0;
}
6:
题目描述
Your task is to calculate the sum of some integers.
输入
Input contains multiple test cases, and one case one line. Each case starts with an integer N, and then N integers follow in the same line.
输出
For each test case you should output the sum of N integers in one line, and with one line of output for each line in input.
样例输入
4 1 2 3 4
5 1 2 3 4 5
样例输出
10
15
可运行代码:
#include<stdio.h>
int main()
{
int n,a,sum=0;
while(scanf("%d",&n)!=EOF)
{ int i=0;
while(i<n)
{scanf("%d",&a);sum+=a;i++;}
printf("%d\n",sum);
sum=0;
}
return 0;
}
7;
题目描述
Your task is to Calculate a + b.
输入
The input will consist of a series of pairs of integers a and b, separated by a space, one pair of integers per line.
输出
For each pair of input integers a and b you should output the sum of a and b, and followed by a blank line.
样例输入
1 5
10 20
样例输出
6
30
可运行代码:
#include<stdio.h>
int main()
{
int a,b;
while(scanf("%d%d",&a,&b)!=EOF)
{
printf("%d\n",a+b);
printf("\n");
}
return 0;
}
///
#include<stdio.h>
int main()
{
int a,b;
while(scanf("%d%d",&a,&b)==2)
{
printf("%d\n",a+b);
printf("\n");
}
return 0;
}
8:
题目描述
Your task is to calculate the sum of some integers
输入
Input contains an integer N in the first line, and then N lines follow. Each line starts with a integer M, and then M integers follow in the same line
输出
For each group of input integers you should output their sum in one line, and you must note that there is a blank line between outputs.
样例输入
3
4 1 2 3 4
5 1 2 3 4 5
3 1 2 3
样例输出
10
15
6
可运行代码:
#include<stdio.h>
int main()
{
int n,n1,a,sum=0;
scanf("%d",&n);
for(int i=0;i<n;i++)
{
scanf("%d",&n1);
for(int j=0;j<n1;j++)
{
scanf("%d",&a);
sum+=a;
}
printf("%d\n",sum);
printf("\n");
sum=0;
}
return 0;
}
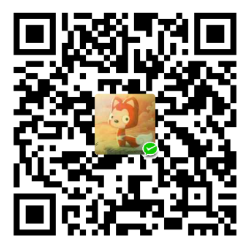
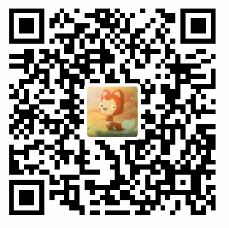