下面的代码就演示了为JTextArea、JList增加滚动条的代码:
package com.cownew.Char19;
import javax.swing.SwingUtilities;
import java.awt.BorderLayout;
import javax.swing.DefaultListModel;
import javax.swing.JPanel;
import javax.swing.JFrame;
import javax.swing.JTextArea;
import javax.swing.ListModel;
import java.awt.Rectangle;
import javax.swing.JList;
import javax.swing.JScrollPane;
public class ScrollPaneTest1 extends JFrame
{
private JPanel jContentPane = null;
private JTextArea jTextArea = null;
private JList jList = null;
private JScrollPane jScrollPane = null;
private JScrollPane jScrollPane1 = null;
private JList jList1 = null;
private JTextArea jTextArea1 = null;
private JTextArea getJTextArea()
{
if (jTextArea == null)
{
jTextArea = new JTextArea();
jTextArea.setBounds(new Rectangle(12, 7, 95, 71));
}
return jTextArea;
}
private JList getJList()
{
if (jList == null)
{
jList = new JList();
jList.setBounds(new Rectangle(8, 92, 106, 71));
DefaultListModel listModel = new DefaultListModel();
listModel.addElement("22222");
listModel.addElement("33333333");
listModel.addElement("55555555555555");
listModel.addElement("8888888888");
listModel.addElement("88888888");
listModel.addElement("999999999");
jList.setModel(listModel);
}
return jList;
}
private JScrollPane getJScrollPane()
{
if (jScrollPane == null)
{
jScrollPane = new JScrollPane();
jScrollPane.setBounds(new Rectangle(143, 7, 122, 75));
jScrollPane.setViewportView(getJTextArea1());
}
return jScrollPane;
}
private JScrollPane getJScrollPane1()
{
if (jScrollPane1 == null)
{
jScrollPane1 = new JScrollPane();
jScrollPane1.setBounds(new Rectangle(142, 96, 128, 68));
jScrollPane1.setViewportView(getJList1());
}
return jScrollPane1;
}
private JList getJList1()
{
if (jList1 == null)
{
jList1 = new JList();
DefaultListModel listModel = new DefaultListModel();
listModel.addElement("22222");
listModel.addElement("33333333");
listModel.addElement("8888888888888888888888888888");
listModel.addElement("8888888888");
listModel.addElement("88888888");
listModel.addElement("999999999");
jList1.setModel(listModel);
}
return jList1;
}
private JTextArea getJTextArea1()
{
if (jTextArea1 == null)
{
jTextArea1 = new JTextArea();
}
return jTextArea1;
}
public static void main(String[] args)
{
SwingUtilities.invokeLater(new Runnable() {
public void run()
{
ScrollPaneTest1 thisClass = new ScrollPaneTest1();
thisClass.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
thisClass.setVisible(true);
}
});
}
public ScrollPaneTest1()
{
super();
initialize();
}
private void initialize()
{
this.setSize(300, 200);
this.setContentPane(getJContentPane());
this.setTitle("JFrame");
}
private JPanel getJContentPane()
{
if (jContentPane == null)
{
jContentPane = new JPanel();
jContentPane.setLayout(null);
jContentPane.add(getJTextArea(), null);
jContentPane.add(getJList(), null);
jContentPane.add(getJScrollPane(), null);
jContentPane.add(getJScrollPane1(), null);
}
return jContentPane;
}
}
运行效果图:
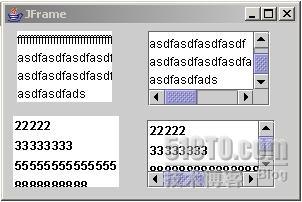
图 17.9
JScrollPane还能为组合界面增加滚动条:
package com.cownew.Char19;
import java.awt.Dimension;
import java.awt.Rectangle;
import javax.swing.JButton;
import javax.swing.JCheckBox;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.JScrollPane;
import javax.swing.JSlider;
import javax.swing.JTextField;
import javax.swing.SwingUtilities;
public class ScrollPaneTest2 extends JFrame
{
private JPanel jContentPane = null;
private JScrollPane jScrollPane = null;
private JPanel jPanel = null;
private JButton jButton = null;
private JButton jButton1 = null;
private JCheckBox jCheckBox = null;
private JTextField jTextField = null;
private JSlider jSlider = null;
private JScrollPane getJScrollPane()
{
if (jScrollPane == null)
{
jScrollPane = new JScrollPane();
jScrollPane.setBounds(new Rectangle(28, 17, 142, 114));
jScrollPane.setViewportView(getJPanel());
}
return jScrollPane;
}
private JPanel getJPanel()
{
if (jPanel == null)
{
jPanel = new JPanel();
jPanel.setLayout(null);
jPanel.add(getJButton(), null);
jPanel.add(getJButton1(), null);
jPanel.add(getJCheckBox(), null);
jPanel.add(getJTextField(), null);
jPanel.add(getJSlider(), null);
jPanel.setPreferredSize(new Dimension(300,200));
}
return jPanel;
}
private JButton getJButton()
{
if (jButton == null)
{
jButton = new JButton();
jButton.setBounds(new Rectangle(6, 10, 74, 28));
}
return jButton;
}
private JButton getJButton1()
{
if (jButton1 == null)
{
jButton1 = new JButton();
jButton1.setBounds(new Rectangle(102, 9, 82, 30));
}
return jButton1;
}
private JCheckBox getJCheckBox()
{
if (jCheckBox == null)
{
jCheckBox = new JCheckBox();
jCheckBox.setBounds(new Rectangle(17, 56, 93, 21));
jCheckBox.setText("aaaaabbb");
}
return jCheckBox;
}
private JTextField getJTextField()
{
if (jTextField == null)
{
jTextField = new JTextField();
jTextField.setBounds(new Rectangle(126, 57, 99, 22));
}
return jTextField;
}
private JSlider getJSlider()
{
if (jSlider == null)
{
jSlider = new JSlider();
jSlider.setBounds(new Rectangle(20, 111, 205, 25));
}
return jSlider;
}
public static void main(String[] args)
{
SwingUtilities.invokeLater(new Runnable() {
public void run()
{
ScrollPaneTest2 thisClass = new ScrollPaneTest2();
thisClass.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
thisClass.setVisible(true);
}
});
}
public ScrollPaneTest2()
{
super();
initialize();
}
private void initialize()
{
this.setSize(221, 177);
this.setContentPane(getJContentPane());
this.setTitle("JFrame");
}
private JPanel getJContentPane()