这篇学习笔记主要介绍了ActiveRecord如何处理One-To-One映射。本文涉及两个实体类User(用户)和NativePalce(祖籍),两个类是一对一的关系:
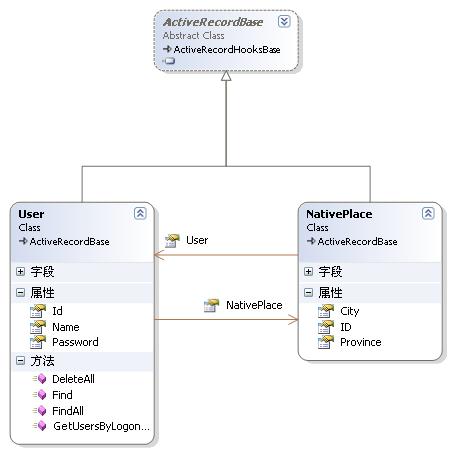
主要内容:
1.编写数据库脚本
2.OneToOne属性说明
3.编写实体类
4.编写表示层调用代码
一、编写数据库脚本















在编写数据库时需要注意的是:
1.副表NativePalce的主键不能为自增类型;
2.主表Users和副表NativePalce的主键名必须一致。
二、OneToOne属性说明:
在Castle.ActiveRecord中,用OneToOne属性代替NHibernate配置文件中的<OneToOne></OneToOne>标签。具有以下子属性:
a.cascade:(可选) 表明操作是否从父对象级联到被关联的对象
b.constrained:(可选) 表明该类对应的表对应的数据库表,和被关联的对象所对应的数据库表之间,通过一个外键引用对主键进行约束。这个选项影响
Save()
和
Delete()
在级联执行时的先后顺序(也在schema export tool中被使用)
c.outer-join:(可选 - 默认为
auto
):当设置
hibernate.use_outer_join
的时候,对这个关联允许外连接抓取
d.access:(可选 - defaults to
property
): 用来访问属性的策略
e.CustomAccess:还不知道干嘛滴
三、编写实体类
本文使用主键关联处理一对一的关系。
主键关联不需要额外的表字段;两行是通过这种一对一关系相关联的,那么这两行就共享同样的主关键字值。所以如果你希望两个对象通过主键一对一关联,你必须确认它们被赋予同样的标识值!
1.在上篇文章中的User类中增加属性NativePlace和字段objNativePlace
















































































































































3.配置文件:以上篇文章的一样
四、编写表示层调用代码(只列出新增User的过程):











































