## 1. 引入php-jwt包
composer require firebase/php-jwt
## 2. 生成token
```
//生成token
public function createJwt($userId = 'zq')
{
$key = md5('zq8876!@!'); //jwt的签发密钥,验证token的时候需要用到
$time = time(); //签发时间
$expire = $time + 14400; //过期时间
$token = array(
"user_id" => $userId,
"iss" => "http://www.najingquan.com/",//签发组织
"aud" => "zhangqi", //签发作者
"iat" => $time,
"nbf" => $time,
"exp" => $expire
);
$jwt = JWTUtil::encode($token, $key);
return $jwt;
}
```
## 3. 验证token
```
//校验jwt权限API
public function verifyJwt($jwt = '')
{
$key = md5('zq8876!@!');
try {
$jwtAuth = json_encode(JWTUtil::decode($jwt, $key, array('HS256')));
$authInfo = json_decode($jwtAuth, true);
$msg = [];
if (!empty($authInfo['user_id'])) {
$msg = [
'status' => 1001,
'msg' => 'Token验证通过'
];
} else {
$msg = [
'status' => 1002,
'msg' => 'Token验证不通过,用户不存在'
];
}
return $msg;
} catch (\Firebase\JWT\ExpiredException $e) {
echo json_encode([
'status' => 1003,
'msg' => 'Token过期'
]);
exit;
} catch (\Exception $e) {
echo json_encode([
'status' => 1002,
'msg' => 'Token无效'
]);
exit;
}
}
```
## 4. 测试
生成token
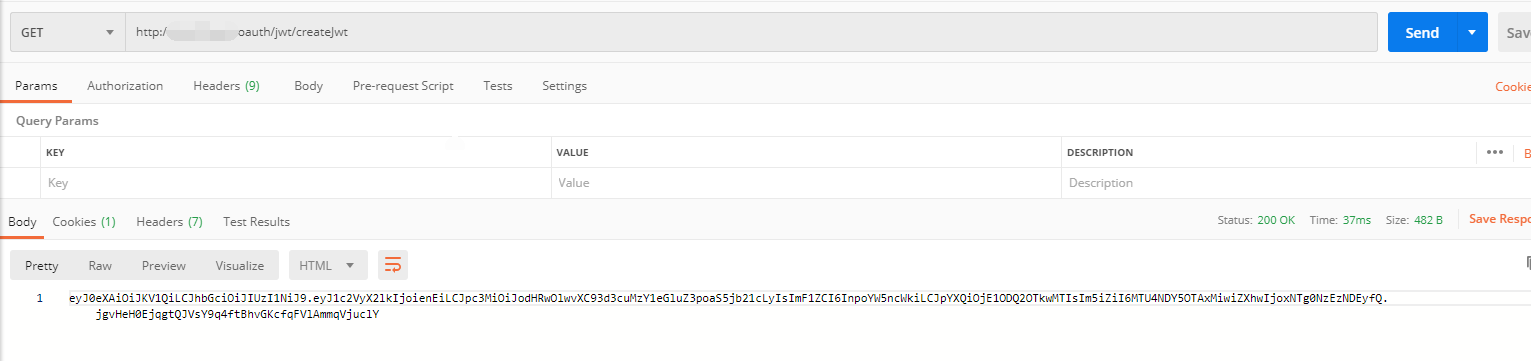
验证token
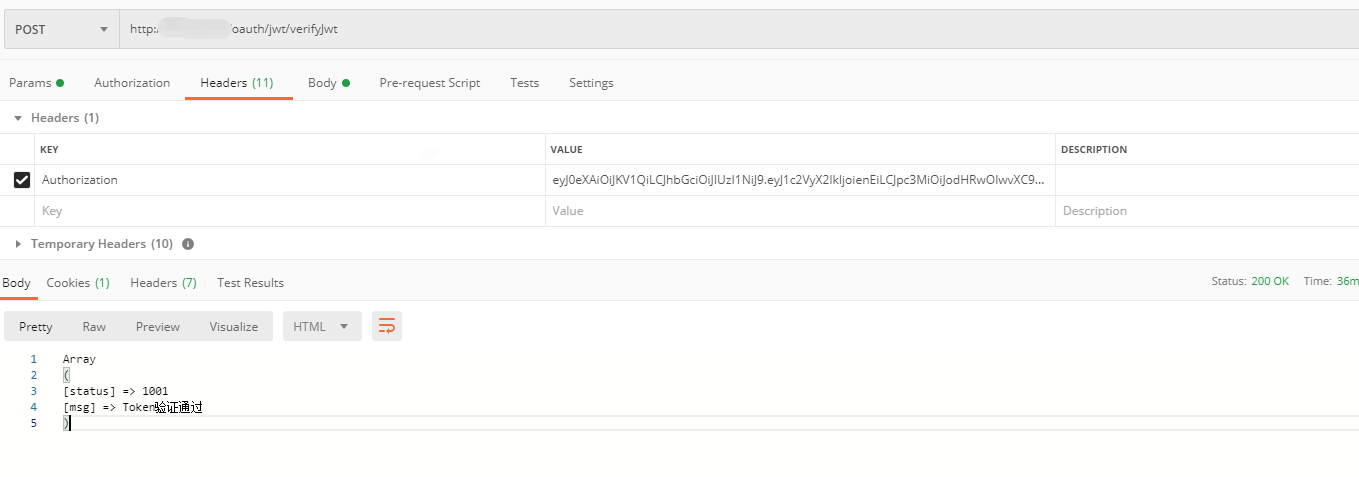