什么是Python列表?
列表的确切含义是一个包含不同Python对象的容器,该对象可能是整数,单词,值等。它等效于其他编程语言中的数组。 它由方括号表示(这是将其与元组(用圆括号分隔的元组)区分开的属性之一)。 它也是可变的,即可以修改或更新。 与不可变的元组不同。
Python列表的例子:
Python列表可以是同类的,这意味着它们可以包含相同类型的对象。 或异构的,包括不同类型的对象。
同类列表的示例包括:
list of integers = [1, 2, 3, 8, 33]list of animals = ['dog', 'cat', 'goat']list of names = ['John', 'Travis', 'Sheila']list of floating numbers = [2.2, 4.5, 9.8, 10.4]
异构列表的示例包括:
[2, 'cat', 34.33, 'Travis'][2.22, 33, 'pen']
访问列表中的值
要访问列表内的值,可以使用列表内对象的索引。 Python列表中的索引是指元素在有序列表中的位置。 例如:
list = [3, 22, 30, 5.3, 20]
- 上面列表中的第一个值3的索引为0
- 第二个值22的索引为1
- 第三个值30的索引为2
等等。 要访问列表中的每个值,您可以使用:
list[0] to access 3list[1] to access 22list[2] to access 30list[3] to access 5.3list[4] to access 20
也可以使用索引-1来访问列表的最后一个成员。 例如,
list[-1] = 20
列表切片(List slicing)
列表切片是一种拆分列表子集的方法,列表对象的索引也用于此目的。 例如,使用上面的相同列表示例;
list[:] = [3, 22, 30, 5.3, 20] (all the members of the list];list[1:3] = [22, 30] (members of the list from index 1 to index 3, without the member at index 3);list[:4] = [3, 22, 30, 5.3] (members of the list from index 0 to index 4, without the member at index 4)list[2:-1] = [30, 5.3] (members of the list from index 2, which is the third element, to the second to the last element in the list, which is 5.3).
Python列表是上限排他的,这意味着列表切片期间的最后一个索引通常被忽略。
这就是为什么
list[2:-1] = [30, 5.3]
,而不是[30,5.3,20]。 上面给出的所有其他列表切片示例也是如此。
更新列表
假设您有一个列表= [物理学,化学,数学],并且想要将该列表更改为[生物学,化学,数学],从而有效地更改了索引为0的成员。可以通过将该索引分配给 您想要的新成员。
那是,
list = [physics, chemistry, mathematics] list[0] = biology print(list)
Output: [biology, chemistry, mathematics]
这将用所需的新值(化学)替换索引0(物理)处的成员。 可以对要更改的列表的任何成员或子集执行此操作。
再举一个例子: 假设您有一个名为整数的列表,其中包含数字[2、5、9、20、27]。 要将列表中的5替换为10,可以执行以下操作:
integers = [2, 5, 9, 20, 27] integers[1] = 10 print(integers)>>> [2, 10, 9, 20, 27]
要将整数列表的最后一个成员27替换为30.5等自由数字,可以使用:
integers = [2, 5, 9, 20, 27] integers[-1] = 30.5 print(integers)>>> [2, 5, 9, 20, 30.5]
删除列表元素
删除列表元素的Python方法有3种:list.remove(),list.pop()和del运算符。 Remove方法将要删除的特定元素作为参数,而pop和del则将要删除的元素的索引作为参数。 例如:
list= [3、5、7、8、9、20]
要从列表中删除3(第一个元素),可以使用:
list.remove(3) orlist.pop[0], ordel list[0]要从列表中删除索引为3的项目8,您可以使用:
list.remove(8), orlist.pop[3]添加列表元素
要将元素追加到列表,请使用append方法,这会将元素添加到列表的末尾。
例如:
list_1 = [3, 5, 7, 8, 9, 20] list_1.append(3.33) print(list_1) >>> list_1 = [3, 5, 7, 8, 9, 20, 3.33] list_1.append("cats") print(list_1) >>> list_1 = [3, 5, 7, 8, 9, 20, 3.33, "cats"]
List 内置函数(方法)
以下是列表内置函数和方法及其说明的列表:
len(list):给出列表的长度作为输出。 例如:
numbers = [2, 5, 7, 9]print(len(numbers))>>> 4
max(list): 返回列表中具有最大值的项目。 例如:
numbers = [2, 5, 7, 9]print(max(numbers))>>> 9
min(list): 返回列表中具有最小值的项目。 例如:
numbers = [2, 5, 7, 9]print(min(numbers))>>> 2
list(tuple): 将元组对象转换为列表。 例如;
animals = (cat, dog, fish, cow)print(list(animals))>>> [cat, dog, fish, cow]
list.append(element): 将元素追加到列表。 例如;
numbers = [2, 5, 7, 9]numbers.append(15)print(numbers)>>> [2, 5, 7, 9, 15]
list.pop(index): 从列表中删除指定索引处的元素。 例如;
numbers = [2, 5, 7, 9, 15]numbers.pop(2)print(numbers)>>> [2, 5, 9, 15]
list.remove(element):从列表中删除元素。
values = [2, 5, 7, 9]values.remove(2)print(values)>>> [5, 7, 9]
list.reverse(): 反转列表的对象。 例如;
values = [2, 5, 7, 10]values.reverse()print(values)>>> [10, 7, 5, 2]
list.index(element): 获取列表中元素的索引值。 例如;
animals = ['cat', 'dog', 'fish', 'cow', 'goat']fish_index = animals.index('fish')print(fish_index)>>> 2
sum(list): 如果值是所有数字(整数或小数),则获取列表中所有值的总和。 例如;
values = [2, 5, 10]sum_of_values = sum(values)print(sum_of_values)>>> 17
如果列表包含不是数字的任何元素(例如字符串),sum方法将不起作用。 您会收到一条错误消息:“ TypeError:+不支持的操作数类型:'int'和'str'”
list.sort(): 以升序或降序排列整数,浮点数或字符串的列表。 例如:values = [1, 7, 9, 3, 5]# To sort the values in ascending order:values.sort()print(values)>>> [1, 3, 5, 7, 9]
另一个例子:
values = [2, 10, 7, 14, 50]# To sort the values in descending order:values.sort(reverse = True)print(values)>>> [50, 14, 10, 7, 2]
字符串列表也可以按字母顺序或字符串长度排序。 例如;
# to sort the list by length of the elementsstrings = ['cat', 'mammal', 'goat', 'is']sort_by_alphabet = strings.sort()sort_by_length = strings.sort(key = len)print(sort_by_alphabet)print(sort_by_length)>>> ['cat', 'goat', 'is', 'mammal'] ['is', 'cat', 'goat', 'mammal']
我们可以使用'strings按字母顺序对同一列表进行排序。
遍历列表
遍历列表可以与Python中的任何其他循环函数完全相同。 这样,可以同时对列表的多个元素执行一种方法。 例如:
list = [10, 20, 30, 40, 50, 60, 70].
要遍历此列表的所有元素,比方说,向每个元素添加10:
for elem in list: elem = elem + 5 print(elem) >>>>15 25 35 45 55 65 75
遍历列表的前三个元素,并将其全部删除;
for elem in list[:3]: list.remove(elem) >>>list = [40, 50, 60, 70]
要遍历列表的第三个元素(索引2),并将它们附加到名为new_list的新列表中,请执行以下操作:
new_list = [] for elem in list[2:]: new_list.append(elem) print(“New List: {}”.format(new_list)) Output:New List: [30, 40, 50, 60, 70]
这样,可以将任何方法或方法或功能应用于列表的成员以执行特定操作。 您可以遍历列表的所有成员,也可以使用列表切片遍历列表的子集。
List Comprehensions列表推导
列表推导是Python函数,用于使用已经创建的序列来创建新序列(例如列表,字典等)。 它们有助于减少较长的循环,并使您的代码更易于阅读和维护。
例如; 假设您要创建一个列表,其中包含从1到9的所有数字的平方:
list_of squares = [] for int in range(1, 10): square = int ** 2 list_of_squares.append(square) print(list_of_squares)List_of_squares using for loop: [1, 4, 9, 16, 25, 36, 49, 64, 81]
要对列表推导执行相同的操作:
list_of_squares_2 = [int**2 for int in range(1, 10)] print('List of squares using list comprehension: {}'.format(list_of_squares_2))Output using list comprehension: [1, 4, 9, 16, 25, 36, 49, 64, 81]
如上所示,使用列表推导编写代码比使用传统的for循环要短得多,而且速度也更快。 这只是使用列表推导代替for循环的一个示例,但是可以在很多也可以使用for循环的地方复制和使用它。 有时,使用for循环是更好的选择,尤其是在代码复杂的情况下,但是在许多情况下,列表理解将使您的编码更加轻松快捷。
下表是包含一些列表函数和方法及其说明的表。
Built-in Functions
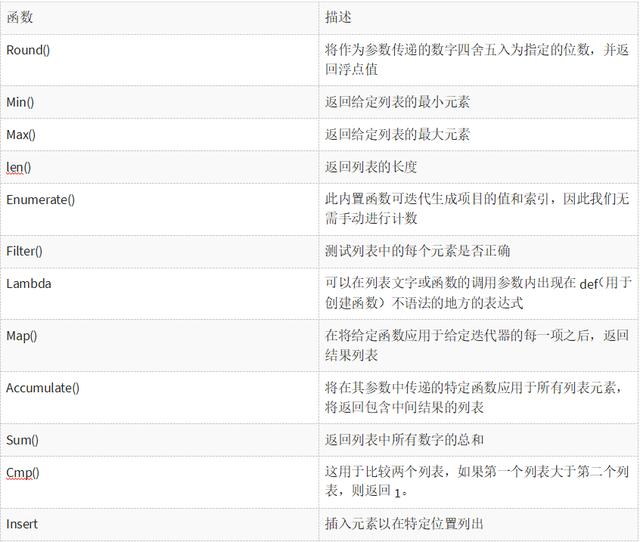
List Methods
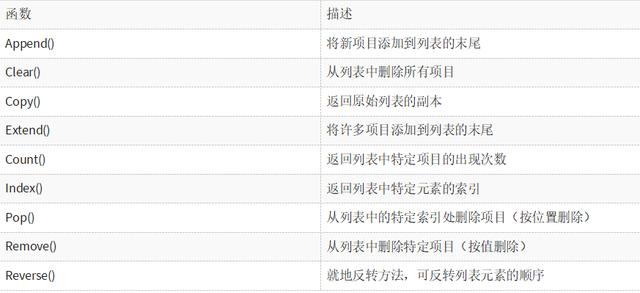
摘要
- 列表的确切含义是一个包含不同Python对象的容器,该对象可能是整数,单词,值等。
- Python列表可以是同类的,这意味着它们可以包含相同类型的对象。 或异构的,包含不同类型的对象。
- 要访问列表内的值,可以使用列表内对象的索引。
- 列表切片是一种拆分列表子集的方法,列表对象的索引也用于此目的。
- 删除列表元素的三种方法是:1)list.remove(),2)list.pop()和3)del运算符
- Append方法用于附加元素。 这会将元素添加到列表的末尾。
- Python程序的循环方法可以在数据列表的多个元素上同时执行。
- 列表推导是Python函数,用于使用已经创建的序列来创建新序列(例如列表,字典等)。