/*数据库的基本操作--*/
以示例的方式,玩转基本的操作, 熟悉了这些操作, mysql就已经入门了
1. 创建表
create table test1(id int(4) not NULL,age int(4));create table test2(id int(4),t1 int(4) not null comment 'test1中的外键id',`name` varchar(100) not null comment '名称');
2. 插入数据
2.1 从查询数据到插入
insert into test1 select 1, 30;insert into test1 select 2, 31;insert into test1 select 3, 32;
2.2 插入一行数据
insert into test1 (id,age) values(4, 33);insert into test2 (id, t1, `name`) values(1, 1, 'test2');
2.3插入多行数据
insert into test1 (id,age) values(5, 34),(6,35);
3. 修改表结构
如果数据库的中数据量已经比较大,修改表结构会导致锁住全表, 每一行数据列会进行数据类型的转化, 速度很慢
3.1 添加一列
alter table test1 add column note varchar(64) not null comment '备注';
3.2 修改列
alter table test1 modify note varchar(100) not null comment '备注';
3.3 修改列名称
alter table test1 change note remark varchar(100) not null comment '备注';
3.4 删除列
alter table test1 drop column remark;
4. 查询
4.1 查询所有
select * from test1;
4.2 按条件查询
select * from test1 where id = 1;
4.3 查询记录数
select count(*) from test1 where id = 1;
4.4 关联查询
注意:left JOIN, right JOIN, arross JOIN的区别, 写法上没有多大的差别, 那么关联要理解笛卡尔积。
select a.id, a.age, b.name from test1 aLEFT JOIN test2 b on a.id = b.t1where a.id = 1;
4.5 聚合查询(group by)
注意:where 条件与having 条件的位置
select id, age, count(*) as num from test1 where id in(1,2) GROUP BY id having age < 40;
4.6 排序(order by)
select id, age, count(*) as num from test1 where id in(1,2) GROUP BY id having age < 40 order by age desc;
4.7 分页(limit)
注意:limit 是从0开始
select id, age, count(*) as num from test1 where id in(1,2) GROUP BY id having age < 40 order by age desc limit 0,1;
5. 索引
索引可以在创建表时创建, 也可以在已创建表之上修改, 如果是主键, 可以不给名称, 因为一个数据表中只存在一个主键, 其他的索引尽量给出名称, 方便修改.
在mysql中常用的索引有:非空索引,主键索引,唯一索引,全文索引,普通索引。
5.1 添加主键索引
alter table test1 add PRIMARY key(id);
5.2 刪除主鍵索引
alter table test1 drop primary key;
5.3 添加唯一索引
alter table test1 add UNIQUE index_name(id);create unique index index_name ON test1(id);
5.4 刪除索引
alter table test1 DROP index index_name;drop index index_name on test1;
5.5 添加普通索引
alter table test1 add index index_name(id);create index index_name on test1(id);
5.6 添加全文索引
alter table test2 add FULLTEXT index_name(`name`);CREATE FULLTEXT index index_name1 on test2(`name`);drop index index_name1 on test2;
5.7 查看表的索引
show index from test1;
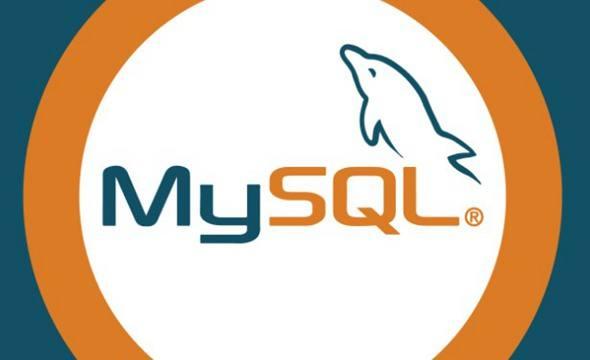