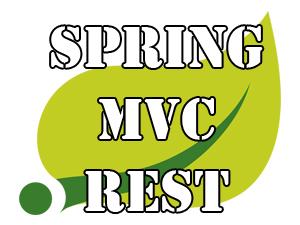
需求和步骤分析
需求:使用 SSM 框架完成对 account 表的增删改查操作。
步骤分析:
- 准备数据库和表记录
- 创建 web 项目
- 编写 MyBatis 在 SSM 环境中可以单独使用
- 编写 Spring 在 SSM 环境中可以单独使用
- Spring 整合 MyBatis
- 编写 SpringMVC 在 SSM 环境中可以单独使用
- Spring 整合 SpringMVC
环境搭建
准备数据库和表记录
CREATE TABLE `account` ( `id` int(11) NOT NULL AUTO_INCREMENT, `name` varchar(32) DEFAULT NULL, `money` double DEFAULT NULL, PRIMARY KEY (`id`)) ENGINE=InnoDB AUTO_INCREMENT=3 DEFAULT CHARSET=utf8;insert into `account`(`id`,`name`,`money`) values (1,'tom',1000),(2,'jerry',1000);
创建 web 项目
使用 Maven 创建名为 ssm 的 web 项目
编写 MyBatis 在 SSM 环境中可以单独使用
需求:基于 MyBatis 先来实现对 account 表的查询
相关坐标
...mysql mysql-connector-java 5.1.47com.alibaba druid 1.1.15org.mybatis mybatis 3.5.1...
Account 实体
public class Account { private Integer id; private String name; private Double money; // getter and setter ... }
AccountDao 接口
public interface AccountDao { public List findAll();}
AccountDao.xml 映射
<?xml version="1.0" encoding="UTF-8" ?> select * from account
MyBatis 核心配置文件
jdbc.properties
jdbc.driverClassName=com.mysql.jdbc.Driverjdbc.url=jdbc:mysql:///spring_db?characterEncoding=utf8&useSSL=falsejdbc.username=rootjdbc.password=password
SqlMapConfig.xml
<?xml version="1.0" encoding="UTF-8" ?>
测试代码
public class MyBatisTest { @Test public void testMybatis() throws IOException { InputStream resourceAsStream = Resources.getResourceAsStream("SqlMapConfig.xml"); SqlSessionFactory sqlSessionFactory = new SqlSessionFactoryBuilder().build(resourceAsStream); SqlSession sqlSession = sqlSessionFactory.openSession(); AccountDao mapper = sqlSession.getMapper(AccountDao.class); List all = mapper.findAll(); for (Account account : all) { System.out.println(account); } sqlSession.close(); }}
编写 Spring 在 SSM 环境中可以单独使用
相关坐标
org.springframework spring-context 5.1.5.RELEASEorg.aspectj aspectjweaver 1.8.13org.springframework spring-jdbc 5.1.5.RELEASEorg.springframework spring-tx 5.1.5.RELEASEorg.springframework spring-test 5.1.5.RELEASEjunit junit 4.12
AccountService 接口
public interface AccountService { List findAll();}
AccountServiceImpl 实现
@Servicepublic class AccountServiceImpl implements AccountService { @Override public List findAll() { System.out.println("findAll 执行了..."); return null; }}
Spring 核心配置文件
applicationContext.xml
<?xml version="1.0" encoding="UTF-8"?>
测试代码
@RunWith(SpringJUnit4ClassRunner.class)@ContextConfiguration("classpath:applicationContext.xml")public class SpringTest { @Autowired private AccountService accountService; @Test public void testSpring() throws Exception { List accountList = accountService.findAll(); System.out.println(accountList); }}
Spring 整合 MyBatis
整合思想
将 MyBatis 接口代理对象的创建权交给 Spring 管理,我们就可以把 Dao 的代理对象注入到 Service 中,此时也就完成了 Spring 与 MyBatis 的整合了。
导入整合包
org.mybatis mybatis-spring 1.3.1
Spring 配置文件管理 MyBatis
此时可以将 MyBatis 主配置文件 SqlMapConfig.xml 删除,在 applicationContext.xml 配置文件中加入 MyBatis:
<?xml version="1.0" encoding="UTF-8"?>
修改 AccountServiceImpl
@Servicepublic class AccountServiceImpl implements AccountService { @Autowired AccountDao accountDao; @Override public List findAll() { return accountDao.findAll(); }}
测试代码
@RunWith(SpringJUnit4ClassRunner.class)@ContextConfiguration("classpath:applicationContext.xml")public class SpringTest { @Autowired private AccountService accountService; @Test public void testSpring() { System.out.println(accountService.findAll()); }}
编写 SpringMVC 在 SSM 环境中可以单独使用
需求:访问到 Controller 里面的方法查询所有账户,并跳转到 list.jsp 页面进行列表展示
相关坐标
org.springframework spring-webmvc 5.1.5.RELEASEjavax.servlet javax.servlet-api 3.1.0providedjavax.servlet.jsp jsp-api 2.2providedjstl jstl 1.2
导入页面资源
在 webapp 目录下导入 JSP 页面相关的资源:add.jsp、index.jsp、list.jsp、update.jsp
index.jsp
...
查询账户信息列表
...
前端控制器 DispatcherServlet
<?xml version="1.0" encoding="UTF-8"?> DispatcherServletorg.springframework.web.servlet.DispatcherServletcontextConfigLocationclasspath:spring-mvc.xml2DispatcherServlet/ CharacterEncodingFilterorg.springframework.web.filter.CharacterEncodingFilterencodingUTF-8CharacterEncodingFilter/*
AccountController 和 list.jsp
@Controller@RequestMapping("/account")public class AccountController { @RequestMapping("/findAll") public String findAll(Model model) { ArrayList arrayList = new ArrayList<>(); arrayList.add(new Account(1, "张人大", 1000d)); arrayList.add(new Account(2, "布莱尔", 2000d)); model.addAttribute("list", arrayList); return "list"; }}... ${account.id} ${account.name} ${account.money} 修改 删除 ...
SpringMVC 核心配置文件
Spring 整合 SpringMVC
整合思想
Spring 和 SpringMVC 本来就已经整合好了,都是属于 Spring 全家桶一部分。
但是需要做到 Spring 和 web 容器整合,让 web 容器启动的时候自动加载 Spring 配置文件,web 容 器销毁的时候 Spring 的 IOC 容器也销毁。
Spring 和 Web 容器整合
ContextLoaderListener 加载
可以使用 spring-web 包中的 ContextLoaderListener 监听器,来监听 servletContext 容器的创建和销毁,来同时创建或销毁 IOC 容器。
web.xml
org.springframework.web.context.ContextLoaderListenercontextConfigLocationclasspath:applicationContext.xml
修改 AccountController
@Controller@RequestMapping("/account")public class AccountController { @Autowired private AccountService accountService; @RequestMapping("/findAll") public String findAll(Model model) { model.addAttribute("list", accountService.findAll()); return "list"; }}
Spring 配置声明式事务
Spring 配置文件加入声明式事务
applicationContext.xml
@Service@Transactionalpublic class AccountServiceImpl implements AccountService { ...}
add.jsp
...
姓名:
余额:
...
AccountDao
void save(Account account);
AccountDao.xml 映射
insert into account (`name`, `money`) values (#{name}, #{money});
AccountService 接口和实现类
void save(Account account);@Overridepublic void save(Account account) { accountDao.save(account);}
AccountController
@RequestMapping("/save")public String save(Account account) { accountService.save(account); // 跳转到 findAll 方法重新查询一次数据库进行数据的遍历展示 return "redirect:/account/findAll";}
修改操作
数据回显
AccountController
@RequestMapping("/findById")public String findById(Integer id,Model model){ // 存到 model 中 model.addAttribute("account", accountService.findById(id)); // 视图跳转 return "update";}
AccountService 接口和实现类
Account findById(Integer id);@Overridepublic Account findById(Integer id) { return accountDao.findById(id);}
AccountDao 接口和 AccountDao.xml 映射文件
Account findById(Integer id); select * from account where id = #{id}
update.jsp
...
姓名:
余额:
...
list.jsp
... 修改 删除...
账户更新
AccountController
@RequestMapping("/update")public String update(Account account) { accountService.update(account); return "redirect:/account/findAll";}
AccountService 接口和实现类
void update(Account account);@Overridepublic void update(Account account) { accountDao.update(account);}
AccountDao 接口和 AccountDao.xml 映射文件
void update(Account account); update account set name = #{name}, money = #{money} where id = #{id}
批量删除
list.jsp
编号 姓名 余额 操作 ${account.id} ${account.name} ${account.money} 修改 删除 添加账户 ......
AccountController
@RequestMapping("/deleteBatch")public String deleteBatch(Integer[] ids) { accountService.deleteBatch(ids); return "redirect:/account/findAll";}
AccountService 接口和实现类
void deleteBatch(Integer[] ids);@Overridepublic void deleteBatch(Integer[] ids) { accountDao.deleteBatch(ids);}
AccountDao 接口和 AccountDao.xml 映射文件
void deleteBatch(Integer[] ids); delete from account #{id}
想了解更多,欢迎关注我的微信公众号:Renda_Zhang