不多bibi,直接建表
DROP TABLE IF EXISTS `user`;CREATE TABLE `user` ( `id` bigint(20) NULL DEFAULT NULL COMMENT '主键', `name` varchar(32) CHARACTER SET utf8 COLLATE utf8_general_ci NULL DEFAULT NULL COMMENT '姓名', `age` int(11) NULL DEFAULT NULL COMMENT '年龄', `email` varchar(64) CHARACTER SET utf8 COLLATE utf8_general_ci NULL DEFAULT NULL COMMENT '邮箱', `manager_id` bigint(20) NULL DEFAULT NULL COMMENT '直属上级id', `create_time` datetime(0) NULL DEFAULT NULL COMMENT '创建时间', `update_time` datetime(0) NULL DEFAULT NULL COMMENT '修改时间', `version` int(11) NULL DEFAULT 1 COMMENT '版本', `deleted` int(1) NULL DEFAULT 0 COMMENT '逻辑删除标识(0,未删除;1,已删除)') ENGINE = InnoDB CHARACTER SET = utf8 COLLATE = utf8_general_ci ROW_FORMAT = Dynamic;INSERT INTO `user` VALUES (1234, '大boss', 40, 'boss@163.com', NULL, '2019-10-02 10:08:02', '2019-10-02 10:08:05', 1, 0);INSERT INTO `user` VALUES (2345, '王天风', 25, 'wtf@163.com', 1234, '2019-10-02 10:09:07', '2019-10-02 10:09:10', 1, 0);INSERT INTO `user` VALUES (2346, '李艺伟', 28, 'lyw@163.com', 2345, '2019-10-02 10:10:09', '2019-10-02 10:10:12', 1, 0);INSERT INTO `user` VALUES (3456, '张雨绮', 31, 'zyq@163.com', 2345, '2019-10-02 10:10:54', '2019-10-02 10:10:58', 1, 0);INSERT INTO `user` VALUES (4566, '刘雨红', 32, 'lyh@163.com', 2345, '2019-10-02 10:11:51', '2019-10-02 10:11:55', 1, 0);SET FOREIGN_KEY_CHECKS = 1;
项目延用MyBatisPlus学习整理(一)https://github.com/xiao-ren-wu/notebook/tree/master/mybatis-plus-demo
逻辑删除
- 设定逻辑删除规则
在配置文件中配置逻辑删除和逻辑未删除的值
mybatis-plus: global-config: logic-not-delete-value: 0 logic-delete-value: 1
- 在pojo类中在逻辑删除的字段加注解@TableLogic
@Data@EqualsAndHashCode(callSuper = false)public class User extends Model { @TableId(type = IdType.AUTO) private Long id; @TableField(condition = SqlCondition.LIKE) private String name; private Integer age; private String email; private Long managerId; private LocalDateTime createTime; private LocalDateTime updateTime; private Integer version; @TableLogic private Integer deleted;}
- 通过id逻辑删除
@Test public void deleteById(){ userMapper.deleteById(4566L); }

- 查询中排除删除标识字段及注意事项
逻辑删除字段只是为了标识数据是否被逻辑删除,在查询的时候,并不想也将该字段查询出来。
我们只需要在delete字段上增加@TableField(select = false)mybatisplus在查询的时候就会自动忽略该字段。
@Test public void selectIgnoreDeleteTest(){ userMapper.selectById(3456L); }

自定义sql,MybatisPlus不会忽略deleted属性,需要我们手动忽略
自动填充
MybaitsPlus在我们插入数据或者更新数据的时候,为我们提供了自动填充功能。类似MySQL提供的默认值一样。 如果我们需要使用自动填充功能,我们需要在实体类的相应属性上加@TableField注解,并指定什么时候进行自动填充。mybatisPlus为我们提供了三种填充时机,在FieldFill枚举中
public enum FieldFill { /** * 默认不处理 */ DEFAULT, /** * 插入时填充字段 */ INSERT, /** * 更新时填充字段 */ UPDATE, /** * 插入和更新时填充字段 */ INSERT_UPDATE}
设置好之后,我们还需要编写具体的填充规则,具体是编写一个填充类并交给Spring管理,然后实现MetaObjectHandler接口中的insertFill和updateFill方法。 eg:
- 插入User对象的时候自动填充插入时间,更新User对象的时候自动填充更新时间。
- 指定实体类中需要自动填充的字段,并设置填充时机
@Data@EqualsAndHashCode(callSuper = false)public class User extends Model { ... @TableField(fill = INSERT) private LocalDateTime createTime; @TableField(fill = UPDATE) private LocalDateTime updateTime; ...}
- 编写填充规则
@Componentpublic class MyMetaObjHandler implements MetaObjectHandler { @Override public void insertFill(MetaObject metaObject) { if(metaObject.hasSetter("createTime")){ setInsertFieldValByName("createTime", LocalDateTime.now(),metaObject); } } @Override public void updateFill(MetaObject metaObject) { if(metaObject.hasSetter("updateTime")){ setUpdateFieldValByName("updateTime",LocalDateTime.now(),metaObject); } }}
解释一下为什么要用if判断是否有对应的属性 mybatisPlus在执行插入或者更新操作的时候,每次都会执行该方法,有些表中是没有设置自动填充字段的,而且有些自动填充字段的值得获取比较消耗系统性能,所以为了不必要的消耗,进行if判断,决定是否需要填充。
有些时候我们已经设置了属性的值。不想让mybatisPlus再自动填充,也就是说我们没有设置属性的值,mybatisPlus进行填充,如果设置了那么就用我们设置的值。这种情况我们只需要在填充类中提前获取默认值,然后使用该默认值就可以了。
@Override public void updateFill(MetaObject metaObject) { if(metaObject.hasSetter("updateTime")){ Object updateTime = getFieldValByName("updateTime", metaObject); if(Objects.nonNull(updateTime)){ setUpdateFieldValByName("updateTime",updateTime,metaObject); }else{ setUpdateFieldValByName("updateTime",LocalDateTime.now(),metaObject); } } }
乐观锁
乐观锁适用于读多写少的情况,更新数据的时候不使用“锁“而是使用版本号来判断是否可以更新数据。通过不加锁来减小数据更新时间和系统的性能消耗,进而提高数据库的吞吐量。CAS机制就是一种典型的乐观锁的形式。 乐观锁是逻辑存在的一种概念,我们如果使用乐观锁需要手动在表的加上version字段。
- mysql使用乐观锁伪代码示例:
update user set balabala....where balabala... and version = xxx
乐观锁
1.配置类中注入乐观锁插件
@Bean public OptimisticLockerInterceptor optimisticLockerInterceptor(){ return new OptimisticLockerInterceptor(); }
- 实体类中的版本字段增加@version注解
@Data@EqualsAndHashCode(callSuper = false)public class User extends Model { ... @Version private Integer version; ...}
- test
更新王天风的年龄
@Test public void testLock(){ int version = 1; User user = new User(); user.setEmail("wtf@163.com"); user.setAge(34); user.setId(2345L); user.setManagerId(1234L); user.setVersion(1); userMapper.updateById(user); }

image.png
数据库中的version已经变成2

image.png
注意事项:
- 支持的类型只有:int,Integer,long,Long,Date,Timestamp,LocalDateTime
- 整数类型下newVerison = oldVersion+1
- newVersion会写到entity中
- 仅支持updateById(id)与update(entity,wrapper)方法
- 在update(entiry,wrapper)方法下,wrapper不能复用
性能分析
- 配置类中注入性能分析插件
@Bean // @Profile({"dev,test"}) public PerformanceInterceptor performanceInterceptor() { PerformanceInterceptor performanceInterceptor = new PerformanceInterceptor(); // 格式化sql输出 performanceInterceptor.setFormat(true); // 设置sql执行最大时间,单位(ms) performanceInterceptor.setMaxTime(5L); return performanceInterceptor; }
执行sql就可以打印sql执行的信息了
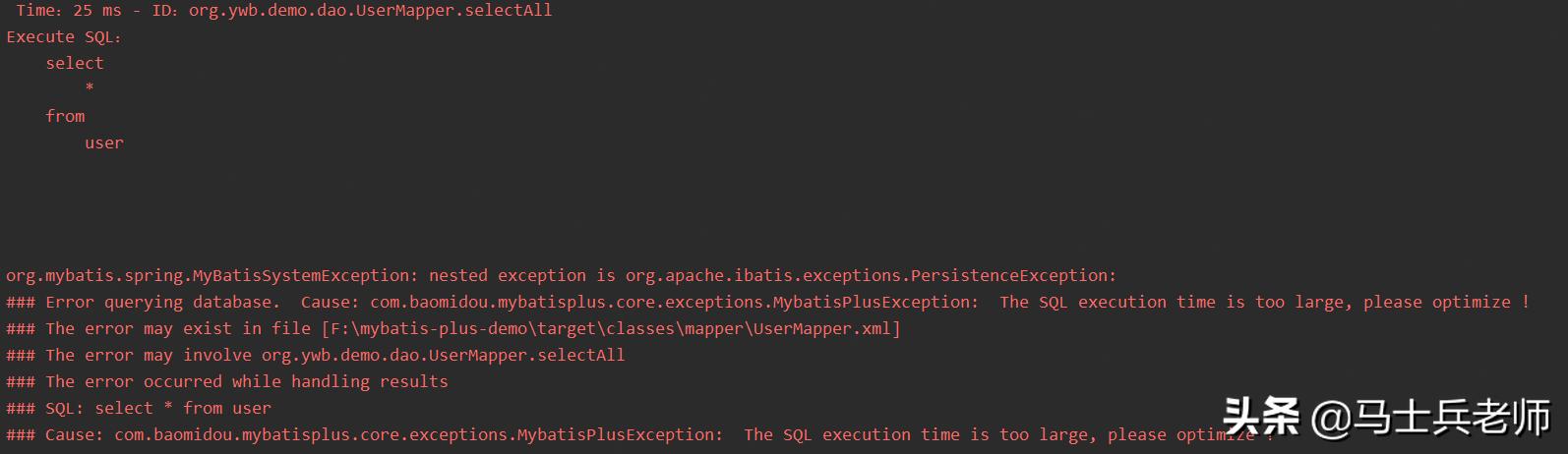
image.png
依靠第三方插件美化sql输出
https://mp.baomidou.com/guide/p6spy.html
- 第三方依赖
p6spy p6spy 3.8.5
- 更改配置文件中的dirver和url
spring: datasource:# driver-class-name: com.mysql.cj.jdbc.Driver username: root password: root# url: jdbc:mysql://localhost:3306/test?serverTimezone=CTT&useUnicode=true&characterEncoding=utf-8&allowMultiQueries=true driver-class-name: com.p6spy.engine.spy.P6SpyDriver url: jdbc:p6spy:mysql://localhost:3306/test?serverTimezone=CTT&useUnicode=true&characterEncoding=utf-8&allowMultiQueries=true
- 增加spy.properties配置文件
module.log=com.p6spy.engine.logging.P6LogFactory,com.p6spy.engine.outage.P6OutageFactory# 自定义日志打印logMessageFormat=com.baomidou.mybatisplus.extension.p6spy.P6SpyLogger#日志输出到控制台appender=com.baomidou.mybatisplus.extension.p6spy.StdoutLogger# 使用日志系统记录 sql#appender=com.p6spy.engine.spy.appender.Slf4JLogger# 设置 p6spy driver 代理deregisterdrivers=true# 取消JDBC URL前缀useprefix=true# 配置记录 Log 例外,可去掉的结果集有error,info,batch,debug,statement,commit,rollback,result,resultset.excludecategories=info,debug,result,batch,resultset# 日期格式dateformat=yyyy-MM-dd HH:mm:ss# 实际驱动可多个#driverlist=org.h2.Driver# 是否开启慢SQL记录outagedetection=true# 慢SQL记录标准 2 秒outagedetectioninterval=2
- test
注意
开启性能分析会消耗系统的性能,所以性能分析插件要配合@Profile注解执行使用的环境。
SQL注入器 ->_-> 封装自定义通用SQL
实现步骤:
- 创建定义方法的类
- 创建注入器
- 在mapper中加入自定义方法
eg: 编写一个删除表所有数据的方法
- 创建定义方法的类
public class DeleteAllMethod extends AbstractMethod { @Override public MappedStatement injectMappedStatement(Class> mapperClass, Class> modelClass, TableInfo tableInfo) { // 执行的sql String sql = "delete from " + tableInfo.getTableName(); // mapper接口方法名 String method = "deleteAll"; SqlSource sqlSource = languageDriver.createSqlSource(configuration, sql, mapperClass); return addDeleteMappedStatement(mapperClass, method, sqlSource); }}
- 创建注入器。添加自己的方法
@Componentpublic class MySqlInject extends DefaultSqlInjector { @Override public List getMethodList(Class> mapperClass) { List methodList = super.getMethodList(mapperClass); methodList.add(new DeleteAllMethod()); return methodList; }}
- 在mapper中加入自定义方法
public interface UserMapper extends BaseMapper { /** * 删除所有表数据 * * @return 影响行数 */ int deleteAll();}
- test
@Test public void deleteAll(){ userMapper.deleteAll(); }
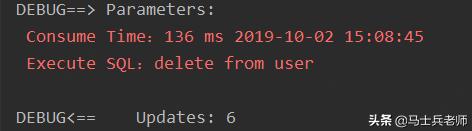
作者:茶还是咖啡
链接:https://www.jianshu.com/p/5c9e6acf9a70