图片是由许多不一样的RGB的像素按照一定的顺序排列而成的,利用python相关处理库可以读取到图片的每一个像素的RGB值。
图片的RGB按照从左到右,从上到下从新生成一张图片,这张图片就是跟原来的图片是一模一样的,如果想实现图片的反转,那图片的RGB必须从右到左,从上到下从新生成图片!
经过测试,已经实现图片的反转,剪切功能。
# 反转图片 def flipPic(self,imgFlip): im = Image.new("RGB", (self.width, self.height)) txt = open(self.txt) num = random.randint(50,100) for i in range(self.width-1,-1,-1): for r in range(0,self.height): line = txt.readline() rgb = line.split(", ") red = int(rgb[0]) + 0 if self.isRandomColor else rgb[0] green = int(rgb[1]) + num if self.isRandomColor else rgb[1] blue = int(rgb[2]) + 0 if self.isRandomColor else rgb[2] im.putpixel((i, r), (int(red), int(green), int(blue))) # print(i,r,red,green,blue) im.save(imgFlip) # im.show() im.close()
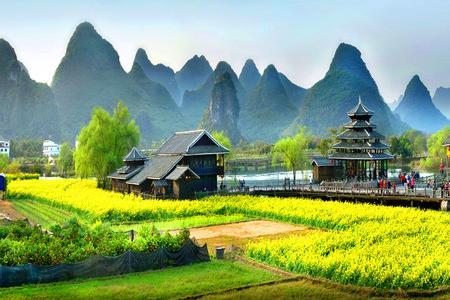
原图
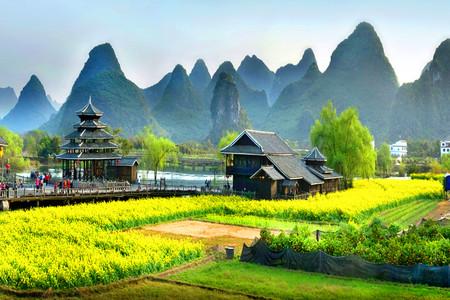
左右反转图
下面是实现从原图片的左上角剪切图片的功能:
# 截取图片 def cutPic(self,imgCut,width,height): self.setRgbToTxt(width,height) im = Image.new("RGB", (width, height)) txt = open(self.txt) num = random.randint(50,100) for i in range(0,width): for r in range(0,height): line = txt.readline() rgb = line.split(", ") red = int(rgb[0]) + 0 if self.isRandomColor else rgb[0] green = int(rgb[1]) + 0 if self.isRandomColor else rgb[1] blue = int(rgb[2]) + num if self.isRandomColor else rgb[2] im.putpixel((i, r), (int(red), int(green), int(blue))) im.save(imgCut) # im.show() im.close()
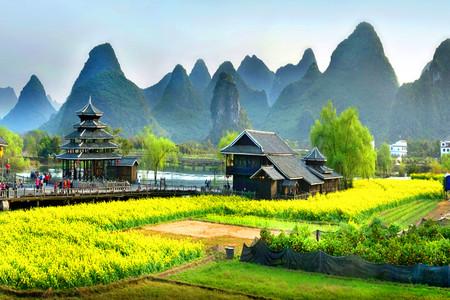
原图
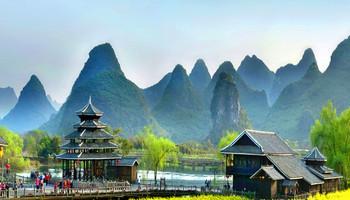
以原图左上角开始剪切350px * 200px的新图片
全部代码展示区:
from PIL import Imageimport osimport randomimport timeclass Photo(): def __init__(self,img,txt,isRandomColor): self.img = img self.txt = txt self.isRandomColor = isRandomColor # 获取图片宽高 def getImgInfo(self): self.im = Image.open(self.img) self.width = self.im.size[0] self.height = self.im.size[1] # 取得图片RGB信息并保存到txt文件 def setRgbToTxt(self,width=0,height=0): width = int(width) if width > 0 else self.width height = int(height) if height > 0 else self.height txt = open(self.txt,"w") for i in range(0,width): for r in range(0,height): v = self.im.getpixel((i,r)) txt.write(v.__str__().replace('(','').replace(")","") + "") # print(i,r,v) txt.close() #创建新图片 def createNewPicByRgb(self,imgNew): im = Image.new("RGB", (self.width, self.height)) txt = open(self.txt) num = random.randint(50,100) for i in range(0,self.width): for r in range(0,self.height): line = txt.readline() rgb = line.split(", ") red = int(rgb[0]) + num if self.isRandomColor else rgb[0] green = int(rgb[1]) + 0 if self.isRandomColor else rgb[1] blue = int(rgb[2]) + 0 if self.isRandomColor else rgb[2] im.putpixel((i, r), (int(red), int(green), int(blue))) im.save(imgNew) # im.show() im.close() # 反转图片 def flipPic(self,imgFlip): im = Image.new("RGB", (self.width, self.height)) txt = open(self.txt) num = random.randint(50,100) for i in range(self.width-1,-1,-1): for r in range(0,self.height): line = txt.readline() rgb = line.split(", ") red = int(rgb[0]) + 0 if self.isRandomColor else rgb[0] green = int(rgb[1]) + num if self.isRandomColor else rgb[1] blue = int(rgb[2]) + 0 if self.isRandomColor else rgb[2] im.putpixel((i, r), (int(red), int(green), int(blue))) # print(i,r,red,green,blue) im.save(imgFlip) # im.show() im.close() # 截取图片 def cutPic(self,imgCut,width,height): self.setRgbToTxt(width,height) im = Image.new("RGB", (width, height)) txt = open(self.txt) num = random.randint(50,100) for i in range(0,width): for r in range(0,height): line = txt.readline() rgb = line.split(", ") red = int(rgb[0]) + 0 if self.isRandomColor else rgb[0] green = int(rgb[1]) + 0 if self.isRandomColor else rgb[1] blue = int(rgb[2]) + num if self.isRandomColor else rgb[2] im.putpixel((i, r), (int(red), int(green), int(blue))) im.save(imgCut) # im.show() im.close() # 删除多余文件 def deleteTxt(self): if os.path.exists(self.txt): os.remove(self.txt) def __del__(self): print("操作完成")if __name__ == "__main__": PATH = os.getcwd() PATH_DATA = os.getcwd() + r"data" # print(PATH_DATA) # exit() fileName = "t1" img = PATH_DATA + r"images%s.jpg" %(fileName) txt = PATH_DATA + r"images%s.txt" %(fileName) imgNew = PATH + r"dataimages%s.jpg" %(fileName+"_imgNew") imgFlip = PATH + r"dataimages%s.jpg" %(fileName+"_imgFlip") imgCut = PATH + r"dataimages%s.jpg" %(fileName+"_imgCut") isRandomColor = False photo = Photo(img,txt,isRandomColor) photo.getImgInfo() photo.cutPic(imgCut,350,200) photo.deleteTxt() time.sleep(2) print("延迟 2 秒") photo.setRgbToTxt() photo.createNewPicByRgb(imgNew) photo.flipPic(imgFlip) photo.deleteTxt()